In the capture mode, basically only the reading process is used. There is a shadow register in STM8, but it is invisible to us. We only need to operate the preload register. And it should be noted that whether it is the counter or the capture/compare register, the high 8 bits are read/written first, and then the low 8 bits are read/written.
The document gives a process for input capture mode
According to this process, we can complete our input capture
The document first mentions writing 01 to the CC1S bit of the TIM1_CCMR1 register to configure the port as an input. However, the TIM1_CCMR1 register states that the CC1S bit can only be written when the channel is closed (CC1E=0 in the TIM1_CCER1 register).
Therefore, in the configuration, first write the CC1E bit of the TIM1_CCER1 register to 0, and then write the CC1S bit of the TIM1_CCMR1 to 01.
TIM1_CCER1 &= (unsigned char)~0x01; //Clear CC1E bit in TIM1_CCER1 before configuring TIM1_CCMR1 TIM1_CCMR1 = 0x01; //Configure CC1S bit in TIM1_CCMR1 to 1, CC1 channel is configured as input, IC1 is mapped to TI1FP1 //No filter, no prescaler (each edge detected on the capture input port triggers a capture)
The TIM1_CCMR1 register has two functions, corresponding to capture mode and comparison mode, only the capture mode is needed.
The filter is used to avoid frequency fluctuations, so just write 0. If there is no filter, we can also write 00 for the divider without using a divider. Of course, you can also use a divider to improve accuracy.
Next is to set the trigger mode. We choose rising edge trigger
TIM1_CCER1 &= (unsigned char)~0x02; //Rising edge or high level trigger
Finally, enable the capture function and set the CC1E bit of the TIM1_CCER1 register to 1. Since we use the interrupt mode, we also set the CC1IE bit of the TIM1_IER register to 1 to allow interrupt requests.
The complete initialization code is as follows
void signal_capture_Init(void) { TIM1_CNTRH = 0x00; // Clear the high 8 bits of the counter TIM1_CNTRL = 0x00; // Clear the lower 8 bits of the counter TIM1_PSCRH = 0x00; //Counter clock division high 8 bits TIM1_PSCRL = 0x10; //Counter clock frequency division low 8 bits 16 frequency division TIM1_CCER1 &= (unsigned char)~0x01; //Clear CC1E bit in TIM1_CCER1 before configuring TIM1_CCMR1 TIM1_CCMR1 = 0x01; //Configure CC1S bit in TIM1_CCMR1 to 1, CC1 channel is configured as input, IC1 is mapped to TI1FP1 //No filter, no prescaler (each edge detected on the capture input port triggers a capture) TIM1_CCER1 &= (unsigned char)~0x02; //Rising edge or high level trigger TIM1_IER |= 0x02; //CC1IE=1, enable capture/compare 1 interrupt TIM1_CCER1 |= 0x01; //Capture enable TIM1_CR1 |= 0x01; // Enable timer/counter }
When an input capture occurs, the counter value is transferred to the TIM1_CCR1 register. The clock source of the timer is set to 16 division in the program.
After the frequency division, the frequency of the counter is 1MHz. The frequency division is used here mainly to avoid the counter overflow, which also reduces the accuracy. At the same time, the initial value of the counter is set to 0. The default counting mode of the counter is to count up. After reaching the maximum value, it starts counting from 0 again.
The interrupt handling code is as follows
@far @interrupt void signal_capture_irq (void) { if(TIM1_SR1&0x02) { TIM1_SR1 &= (unsigned char)~0x02; //Clear CC1IF flag if(vsync_cap_data_old == 0x00) {//The first capture interrupt comes vsync_cap_data_old = TIM1_CCR1H; //Read the high 8 bits first vsync_cap_data_old = (unsigned int)(vsync_cap_data_old<<8) + TIM1_CCR1L; // read the lower 8 bits of data again } else { //The second capture interrupt comes vsync_cap_data_new = TIM1_CCR1H; //Read the high 8 bits first vsync_cap_data_new = (unsigned int)(vsync_cap_data_new<<8) + TIM1_CCR1L; // read the lower 8 bits of data again TIM1_IER &= (unsigned char)~0x02; //Disable channel 1 capture/compare interrupt TIM1_CR1 &= (unsigned char)~0x01; //Stop the counter if(vsync_cap_data_new > vsync_cap_data_old) vsync_period = (vsync_cap_data_new - vsync_cap_data_old); else vsync_period = 0xFFFF + vsync_cap_data_new - vsync_cap_data_old; vsync_cap_data_old = 0x00; isCaptureOver = 1; } } }
We capture two interrupts and calculate the time difference.
if(isCaptureOver) { //If the capture is complete, process the data cmd_puts("period:"); cmd_hex((unsigned char)(vsync_period>>8)); cmd_hex((unsigned char)vsync_period); TIM1_CNTRH = 0x00; // Clear the high 8 bits of the counter TIM1_CNTRL = 0x00; // Clear the lower 8 bits of the counter TIM1_IER |= 0x02; //CC1IE=1, enable capture/compare 1 interrupt TIM1_CR1 |= 0x01; // Enable timer/counter isCaptureOver = 0; }Here only the cycle is output from the serial port, the results are as follows
It can be seen that the period fluctuates within a range. We take a value 0x79ED to calculate. The corresponding frequency f=1000000/0x79ED=32.0379Hz is still relatively close to our actual input frequency of 30Hz. The error is a bit large, and it can be further improved through the code
Previous article:STC MCU timer2 capture mode frequency measurement
Next article:Design of data acquisition and processing system for p87c591
Recommended ReadingLatest update time:2024-11-16 23:55
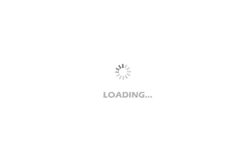
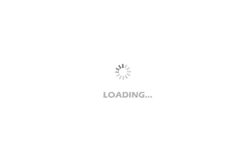
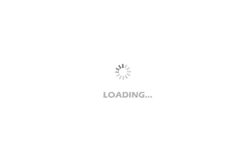
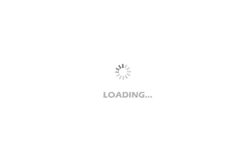
- Popular Resources
- Popular amplifiers
-
STM8 C language programming (1) - basic program and startup code analysis
-
Description of the BLDC back-EMF sampling method based on the STM8 official library
-
STM32 MCU project example: Smart watch design based on TouchGFX (8) Associating the underlying driver with the UI
-
uCOS-III Kernel Implementation and Application Development Practical Guide - Based on STM32 (Wildfire)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- I'm begging for the full datasheet of the Marvell 88e6171/6175 switch chip
- [Vote] Is it harder to read code or write code? Let’s talk about it
- 【GD32307E-START】DAC+ADC test
- EZ-BLE PRoC Low Power Bluetooth Module Introduction
- Can someone help me analyze what this circuit does?
- Op amp circuit waveform analysis problem
- [GD32E231 DIY Contest]——08. Solution to using JLINK to debug and download GD32E231
- Recruiting hardware test engineers Annual salary: 200,000-500,000 | Experience: more than 5 years | Work location: Shenzhen
- Supercapacitor balancing circuit
- When should you choose DC/DC and when should you choose LDO?