Host: WIN8
Development environment: MDK5.13
mcu: stm32f103RB
illustrate:
The observer pattern was previously used in Java, and now the idea of this pattern is applied to microcontroller programming
Android Programming: Observer Pattern Design:
http://blog.csdn.net/jdh99/article/details/41821295
The essence of the observer pattern:
There are two modules A and B. A is the target and B is the observer. Then B can observe the changes of A.
In the program implementation process is:
1.A Generate data
2. A notifies B
3.B Processing Data
Method implemented in single chip microcomputer:
In Java, this idea is implemented through interfaces. If the microcontroller is programmed in C language, it can be implemented through function pointers.
The dw1000 communication module in the source code provides two observable targets: receive data and send data complete.
source code:
dw1000.h
/* * dw1000 communication module header file * (c) copyright 2015, jdh * All Rights Reserved *New creation time: 2015/1/5 by jdh *Modified on: 2015/1/6 by jdh / #ifndef _DW1000_H_ #define _DW1000_H_ /* * head File / #include "world.h" /* * Macro definition / /* * Maximum length of receive data buffer / #define LEN_DW1000_BUF_RX 128 /* * data structure / /* * dw1000 time structure / union _Dw1000_Time { uint8_t pt[8]; uint64_t value; }; /* * Receive data structure / struct _Dw1000_Rx { //Receive data uint8_t buf[LEN_DW1000_BUF_RX]; //dw1000 time to receive data union _Dw1000_Time time; }; /* * Observer mode: receiving data processing function pointer / typedef void (*T_Dw1000_Deal_Rx)(struct _Dw1000_Rx); /* * Observer mode: Send data to complete the processing function pointer / typedef void (*T_Dw1000_Deal_Tx_End)(union _Dw1000_Time); /* * Receive buffer / struct _Rx_Buf_CC1100 { //Receive time T_Time time; //Source ID uint16_t src_id; //function code uint8_t cmd; //data uint8_t data[3]; //rssi int rssi; //lqi uint8_t lqi; }; /* * Functions / /* * Interface function: module loading / void dw1000_load(void); /* * Interface function: module operation / void dw1000_run(void); /* * Interface function: interrupt processing function / void dw1000_irq_handler(void); /* * Interface function: Determine whether it can be sent *Return: 0: cannot be sent, 1: can be sent / uint8_t cc1100_judge_tx(void); /* * Interface function: send data *Parameter: cmd: function code * id: target id * data: 3 bytes of data / void cc1100_tx(uint8_t cmd,uint16_t id,uint8_t *data); /* * Interface function: get received data * Return: receive data / struct _Rx_Buf_CC1100 cc1100_get_rx_buf(void); /* * Interface function: set frequency *Parameter: freq: the frequency point to be set / void cc1100_set_freq(uint8_t freq); /* * Interface function: Register observer: Receive data / void dw1000_register_observer_rx(T_Dw1000_Deal_Rx function); /* * Interface function: Register observer: Send completed / void dw1000_register_observer_tx_end(T_Dw1000_Deal_Tx_End function); #endif
dw1000.c
/* * dw1000 communication module main file * (c) copyright 2015, jdh * All Rights Reserved *New creation time: 2015/1/5 by jdh *Modified on: 2015/1/6 by jdh / /* * head File / #include "dw1000.h" /* * Macro definition / /* * Maximum number of observers / #define MAX_OBSERVER 10 /* * Static variables / /* * Receive data observer list / static T_Dw1000_Deal_Rx Observer_Rx[MAX_OBSERVER]; static uint8_t Len_Observer_Rx = 0; /* * Send the completed observer list / static T_Dw1000_Deal_Tx_End Observer_Tx_End[MAX_OBSERVER]; static uint8_t Len_Observer_Tx_End = 0; /* * Static functions / /* * Receive and process / static void deal_rx(void); /* * Send end processing / static void deal_tx_end(void); /* * Functions / /* * Interface function: module loading / void dw1000_load(void) { } /* * Interface function: module operation / void dw1000_run(void) { } /* * Interface function: Register observer: Receive data / void dw1000_register_observer_rx(T_Dw1000_Deal_Rx function) { Observer_Rx[Len_Observer_Rx++] = function; } /* * Interface function: Register observer: Send completed / void dw1000_register_observer_tx_end(T_Dw1000_Deal_Tx_End function) { Observer_Tx_End[Len_Observer_Tx_End++] = function; } /* * Interface function: interrupt processing function / void dw1000_irq_handler(void) { uint32_t status = 0; uint32_t clear = 0; // will clear any events seen uint8_t resetrx; status = dwt_read32bitreg(SYS_STATUS_ID); // read status register low 32bit if(status & SYS_STATUS_LDEDONE) { if((status & (SYS_STATUS_LDEDONE | SYS_STATUS_RXPHD | SYS_STATUS_RXSFDD)) != (SYS_STATUS_LDEDONE | SYS_STATUS_RXPHD | SYS_STATUS_RXSFDD)) { resetrx = 0xe0; //got LDE done but other flags SFD and PHR are clear - this is a bad frame - reset the transceiver dwt_forcetrxoff(); //this will clear all events //set rx reset dwt_writetodevice(PMSC_ID, 0x3, 1, &resetrx); resetrx = 0xf0; //clear RX reset dwt_writetodevice(PMSC_ID, 0x3, 1, &resetrx); // dwt_write16bitoffsetreg(SYS_CTRL_ID,0,(uint16)SYS_CTRL_RXENAB); } } if((status & SYS_STATUS_RXFCG) && (status & SYS_STATUS_LDEDONE)) // Receiver FCS Good { //clear all receive status bits (as we are finished with this receive event) clear |= status & CLEAR_ALLRXGOOD_EVENTS ; dwt_write32bitreg(SYS_STATUS_ID,clear); // write status register to clear event bits we have seen //Receive and process deal_rx(); } else { if (status & SYS_STATUS_TXFRS) // Transmit Frame Sent { clear |= CLEAR_ALLTX_EVENTS; //clear TX event bits dwt_write32bitreg(SYS_STATUS_ID,clear); // write status register to clear event bits we have seen //Send end processing deal_tx_end(); } else { if (status & SYS_STATUS_RXRFTO) { //Receive timeout clear |= status & SYS_STATUS_RXRFTO ; dwt_write32bitreg(SYS_STATUS_ID,clear); // write status register to clear event bits we have seen dwt_setrxtimeout(0); dwt_rxenable(0); } else { // Clear all flags when exception occurs clear |= CLEAR_ALLRXERROR_EVENTS; dwt_write32bitreg(SYS_STATUS_ID,clear); // write status register to clear event bits we have seen dwt_forcetrxoff(); //this will clear all events //set rx reset dwt_writetodevice(PMSC_ID, 0x3, 1, &resetrx); resetrx = 0xf0; //clear RX reset dwt_writetodevice(PMSC_ID, 0x3, 1, &resetrx); dwt_rxenable(0); } } } } /* * Receive and process / static void deal_rx(void) { struct _Dw1000_Rx rx; uint16_t len; uint8_t i = 0; len = dwt_read16bitoffsetreg(RX_FINFO_ID, 0) & 0x3FF; if (len >= 127) { return; } dwt_write32bitreg(SYS_STATUS_ID,CLEAR_ALLRXGOOD_EVENTS); //Get the receiving time dwt_readrxtimestamp(rx.time.pt); rx.time.value &= MASK_40BIT; //Get received data dwt_readfromdevice(RX_BUFFER_ID,0,len,rx.buf); //Notify observers for (i = 0;i < Len_Observer_Rx;i++) { Observer_Rx[i](rx); } // uint8 resetrx; // //// resetrx = 0xe0; //got LDE done but other flags SFD and PHR are clear - this is a bad frame - reset the transceiver //// dwt_forcetrxoff(); //this will clear all events //// dwt_writetodevice(PMSC_ID, 0x3, 1, &resetrx);//set rx reset //// resetrx = 0xf0; //clear RX reset //// dwt_writetodevice(PMSC_ID, 0x3, 1, &resetrx); dwt_write16bitoffsetreg(SYS_CTRL_ID,0,(uint16)SYS_CTRL_RXENAB); } /* * Send end processing / static void deal_tx_end(void) { union _Dw1000_Time time; uint8_t i = 0; //Get the sending time dwt_readtxtimestamp(time.pt); time.value &= MASK_40BIT; //Notify observers for (i = 0;i < Len_Observer_Tx_End;i++) { Observer_Tx_End[i](time); } }
Observe the received data of the dw1000 module in main.c:
//Add observer to receive data dw1000_register_observer_rx(deal_rx);
Processing function:
void deal_rx(struct _Dw1000_Rx rx) { //deal with... __nop(); __nop(); __nop(); }
Previous article:Full tutorial on developing an optical fingerprint recognition module (FPM10A) based on the STM32 microcontroller
Next article:S3C2440 Mini 2440 DMA mode to achieve Uart serial communication
Recommended ReadingLatest update time:2024-11-16 17:29
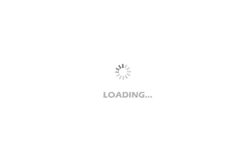
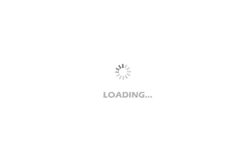
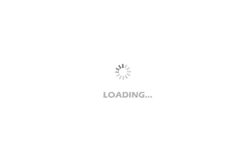
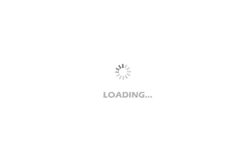
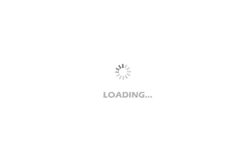
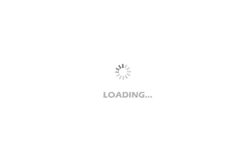
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Can the TF card that comes with SensorTile.box be used?
- EEWORLD University ---- 3D TOF Robot: Obstacle Detection, Collision Avoidance and Navigation
- 【Aigtek Instrument Peripherals】Do you know how to use a dotting machine?
- PADS----ECO problem, as shown in the picture, what is the cause? Please tell me if you know, thank you
- Microwave Solid-State Circuit Design (Second Edition)
- Let's take a look at the electromagnetic waves in Korean
- How terrible is it that an electric motorcycle battery explodes?
- Is there any official routine for developing EFM8 in Simplicity Studio? Where can I download it?
- What is the difference between W(b) and b in assembly language?
- 【Silicon Labs BG22-EK4108A Bluetooth Development Evaluation】+Power-on and Example Program Test