1. Just control the LED on and off, no value is returned
2. Control the LED on and off and return the value
I watched several lectures, all of which were about serial port mode 1. I haven't touched on the others yet, so here I will only use serial port mode 1 to implement these two functions. The part that needs to be calculated in the serial port is to find the initial value of the timer based on the baud rate to be used. The timer uses mode 2, which can automatically load the initial value to avoid errors when the assignment statement loads the initial value.
At 9800bps, timer mode 2, serial port mode 1, and crystal oscillator baud rate of 11.0592MHZ, calculate the values of TH1 and TL1.
The baud rate of mode 1 = (2^SMOD/32) x T1 overflow rate. After the microcontroller is reset, all the power management registers PCON are cleared, and SMOD is also cleared as one of the bits.
The baud rate is already known. So the only thing left is the T1 overflow rate.
Assuming the initial value is X, the timer overflows every time it counts 256-X numbers (the timer is 8 bits, and the maximum is 255. Overflow occurs when 256). The time it takes to count one number is one machine cycle, and the machine cycle = T clock cycle x 12. So the overflow time is = number x each time = (256 - X) * 12/Fosc. Then the base rate is the reciprocal of the overflow time.
So combined with the formula "mode 1 baud rate = (2^SMOD/32)xT1 overflow rate", the formula can be summarized as:
9600 = 2^0 /32 * Fosc / (256 - X)*12 Substitute all known data to get 9600 = 2^0 /32 * 11059200/ (256 - X)*12 =====》》》》 The obtained X is: 253 .
On this basis, if SMOD is set to 1, the baud rate is:
Baud rate = (2^1/32) * 11059200 / (256 - 253) = 2 * [1/32 * 11059200 / (256 - 253)] = 2 * 9600 = 19200. That is, it becomes twice the original.
If the crystal oscillator is replaced with 12MHZ and the initial value is calculated, the obtained X is: 252.744792... infinite decimal. This will cause errors. I always felt that integer crystal oscillators were good before, but now I know why there is a crystal oscillator like 11.0592MHZ.
The initial value is calculated in this way, and the code is posted below.
It only controls the LED on and off, and does not return a value.
There are two ways to achieve this: query and interrupt.
A. Use query first. It feels better to call it judgment because it is implemented by if judgment.
#includeB. Interruption methodvoid main() { //Setting parameters TMOD = 0x20; //Set the working mode of timer 1 to mode 2 TH1 = 0xfd; TL1 = 0xfd; //Load TH1, TL1 TR1 = 1; //Start timer 1 REN = 1; //Enable serial reception bit SM0 = 0; SM1 = 1; //Set the serial port working mode to mode 1 /* * EA = 1; //Global interrupt enable bit * ES = 1; //Serial port interrupt enable bit * The query method is used here to determine the receive interrupt flag, so even if the interrupt enable bit is not enabled, * */ while(1) { //Query method to detect RI if(RI == 1) //RI is the receive interrupt flag. Set to 1 by hardware and cleared to 0 by software { P1 = SBUF; RI = 0; } } }
#includevoid main() { //Setting parameters TMOD = 0x20; //Set the working mode of timer 1 to mode 2 TH1 = 0xfd; TL1 = 0xfd; //Load TH1, TL1 TR1 = 1; //Start timer 1 REN = 1; //Enable serial reception bit SM0 = 0; SM1 = 1; //Set the serial port working mode to mode 1 EA = 1; //Global interrupt enable bit ES = 1; //Serial port interrupt enable bit while(1); //Wait for the interrupt to occur } //Interrupt detection RI void ser() interrupt 4 { P1 = SBUF; RI = 0; }
In addition to the code, it seems that the interrupt enable bit is turned on or not. Because RI is set to 1 automatically by the hardware. Even if the interrupt enable bit is not turned on, it can still be judged by if.
The above two are one-way, here is a two-way one.
/* *Send data to the lower computer through the serial port and display it on the running light of port P1. *At the same time, the MCU returns the received data and displays it on the serial port assistant */ #includeunsigned char flag; void main() { //Setting parameters TMOD = 0x20; //Set the working mode of timer 1 to mode 2 TH1 = 0xfd; TL1 = 0xfd; //Load TH1, TL1 TR1 = 1; //Start timer 1 SM0 = 0; SM1 = 1; //Set the serial port working mode to mode 1 REN = 1; //Enable serial reception bit EA = 1; //Global interrupt enable bit ES = 1; //Serial port interrupt enable bit while(1) { /* At the beginning, the MCU buffer register is empty and no data can be displayed * Receive data from the serial port first, then return the data * Receive data in the interrupt and set the flag to 1. This means that data has been received. * If data is received (flag == 1), it means it is received; otherwise, it means no data is received and no display is made. Continue to determine the flag value */ if(flag == 1) { //send data ES = 0; //Disable serial port interrupt and send data SBUF = P1; //Write data into SBUF register while(!TI); //wait TI = 0; ES = 1; flag = 0; } } } void ser() interrupt 4 { //Receive data P1 = SBUF; flag = 1; RI = 0; }
The flag = 0 in the main function must be there. Otherwise, the serial port assistant will get stuck after just a short while.
There are two more key statements in this example:
P1 = SBUF; //Assign the value in the SBUF register to P1
SBUF = P1; //Write the value of P1 into SBUF
SBUF is written like this: SBUF serial data buffer register, one send buffer register, one receive buffer register. Both share the same address 99H, but are physically two independent registers. So how to distinguish whether it is sending or receiving? Use statements to distinguish.
To control the running lights, you need to send the hexadecimal format.
For example, if I send FB (1111, 1011), the L2 light will be on on my development board. If I send characters, it will be hard to control. If I use the routine in 2 and send "fb" as a character, the microcontroller will return to the serial assistant and display it as "62" in hexadecimal. This, well, I don't know how to calculate it at the moment:P
There are no memorable pictures, just some running lights. However, the running lights now are not the same as before. Now the running lights on the development board can be controlled by me from the computer:D
I just don't know when I will write a host computer next time.
Previous article:Stepper Motor Driver Based on 51 Single Chip Microcomputer
Next article:MCU Tour - Interrupt Flowing Light
Recommended ReadingLatest update time:2024-11-16 19:43
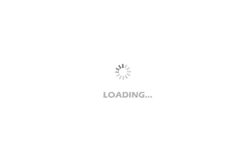
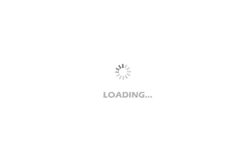
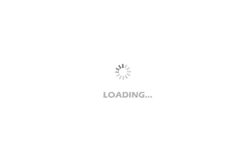
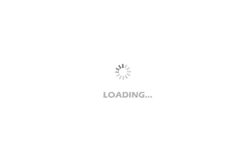
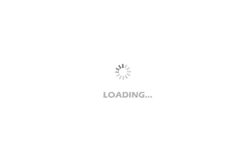
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Traffic Light Control System Based on Fuzzy Control (Single-Chip Microcomputer Implementation)
-
Principles and Applications of Single Chip Microcomputers (Second Edition) (Wanlong)
-
MCU Principles and C51 Programming Tutorial (2nd Edition)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 883 Q What does Q stand for in the device identification?
- Received the prize, I feel the delivery speed is very fast now
- LM358 op amp problem, please give me some advice
- How to Measure Circuit Current Using a Shunt Resistor
- 【TI millimeter wave radar evaluation】+SDK development environment
- PCB News: Zhongjing Electronics plans to acquire 45% of Yuansheng Electronics for RMB 270 million to achieve horizontal integration of PCB
- How to use EPI general mode to communicate with FPGA in TM4c129 series microcontroller
- Before buying a cable fault tester, you must know about its manufacturer. How to find a good time...
- High-speed PCB design technology (Chinese)
- Buck-boost DCDC TPS63810 application in TWS earphones