Program source code
/*-----------------------Include header file area-------------------------*/
#include /*-----------------------Data type definition area-----------------------*/ typedef unsigned char u8; //define type unsigned char alias u8 typedef unsigned int u16; //define type unsigned int alias u16 /*-----------------------User-defined data area---------------------*/ #define FOSC 11059200L //System clock #define Timer_value (65536-(FOSC/12/1000)*1) //Timer value = (timer overflow value - (system clock/12T mode/1000 = timer value for timing 1ms)) * timing time in ms) //The common anode digital tube displays the character array, and the corresponding characters are "0123456789ABCDEF-" code u8 LED_Table[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0x88,0x83,0xc6,0xa1,0x86,0x8e,0xBF,0xFF}; u8 LED_show[8]; //Data array displayed by digital tube u8 key; //Get the key value variable /*-----------------------Port/Pin Definition Area----------------------*/ sbit x1=P1^0; //Port connected to the first row of the matrix sbit x2=P1^1; //Port connected to the second row of the matrix sbit x3=P1^2; //Port connected to the third row of the matrix sbit x4=P1^3; //Port connected to the 4th row of the matrix sbit y1=P1^4; //Port connected to the first column of the matrix sbit y2=P1^5; //Port connected to the second column of the matrix sbit y3=P1^6; //Port connected to the third column of the matrix sbit y4=P1^7; //Port connected to the 4th column of the matrix /*-----------------------Function declaration area---------------------------*/ void delay(u16 ms); //delay function declaration void Timer0_Init(void); //Timer 0 configuration function declaration void LED_Scan(void); //Declaration of digital tube scanning function u8 Get_KEY_value(void); //Get key value function declaration /*-----------------------Main function area-----------------------------*/ void main() { Timer0_Init(); //Timer 0 initialization while(1) // Repeat the while loop program { if(Get_KEY_value()!=0) //The returned key value is not equal to 0, which means a key is pressed { delay(10); //delay to eliminate jitter if(Get_KEY_value()!=0) //Confirm whether the key is actually pressed { key=Get_KEY_value(); //Get the key value } } else //No key is pressed { key=0; //If no key is pressed, key is 0 } LED_show[0]=LED_Table[key/10]; //The first digital tube displays the tens digit of the key value LED_show[1]=LED_Table[key%10]; //The second digital tube displays the unit digit of the key value } } /*-----------------------Timer 0 interrupt function area--------------------*/ void Timer0()interrupt 1 { TH0=Timer_value>>8; //Reset the high 8 bits of the timer value TL0=Timer_value; //Reset the lower 8 bits of the timer value LED_Scan(); //Digital tube scanning } /*---------------------------------------------------------------- Function name: delay() Function: Delay Function parameter: ms is the delay time, the delay range is 0~65535 Return value: None ----------------------------------------------------------------*/ void delay(u16 ms) { u8 i; while(ms--) //loop delay 1ms times { for(i=115;i>0;i--); //delay 1ms } } /*---------------------------------------------------------------- Function Name: Timer0_Init() Function: Timer 0 initialization Function parameters: None Return value: None ----------------------------------------------------------------*/ void Timer0_Init(void) { TMOD=0x01; //Set timer 0 to mode 1 (16-bit counting mode) TH0=Timer_value>>8; //Set the high 8 bits of the timer value TL0=Timer_value; //Set the lower 8 bits of the timer value TR0=1; //Timer 0 counter starts timing ET0=1; //Enable timer 0 interrupt EA=1; //Open the general interrupt } /*---------------------------------------------------------------- Function name: LED_Scan() Function: Digital tube scanning Function parameters: None Return value: None ----------------------------------------------------------------*/ void LED_Scan(void) { static u8 i=0; P0=0xFF; //Display blanking (the blanking level is opposite to the effective level. If the bit selection is enabled first and then the segment selection data is displayed, the blanking is the segment selection, otherwise the blanking is the bit selection) P2=~(0x01< //Enable the (i+1)th digital tube position selection P0=LED_show[i]; //Display the (i+1)th digital tube segment selection data i=(i+1)%2; //Display 2 digital tubes } /*---------------------------------------------------------------- Function name: Get_KEY_value() Function: Get key value Function parameters: None Return value: 0 if no key is entered, 1 to 16 if a key is entered ----------------------------------------------------------------*/ u8 Get_KEY_value(void) { //Detect the first row of buttons x1=0; x2=1; x3=1; x4=1; if(y1==0) return 1; else if(y2==0) return 2; else if(y3==0) return 3; else if(y4==0) return 4; //Detect the second row of buttons x1=1; x2=0; x3=1; x4=1; if(y1==0) return 5; else if(y2==0) return 6; else if(y3==0) return 7; else if(y4==0) return 8; //Detect the third row of buttons x1=1; x2=1; x3=0; x4=1; if(y1==0) return 9; else if(y2==0) return 10; else if(y3==0) return 11; else if(y4==0) return 12; //Detect the fourth row of buttons x1=1; x2=1; x3=1; x4=0; if(y1==0) return 13; else if(y2==0) return 14; else if(y3==0) return 15; else if(y4==0) return 16; return 0; //No key pressed returns 0 } Simulation Circuit
Previous article:51 single-chip microcomputer STC12C5A60S2 timer is used as delay function, and the timer realizes accurate delay
Next article:51 MCU STC89C52 timer interrupt method to scan digital tube and realize stopwatch
Recommended ReadingLatest update time:2024-11-16 10:34
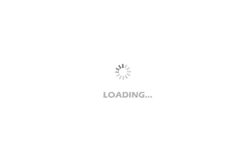
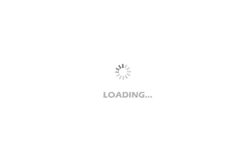
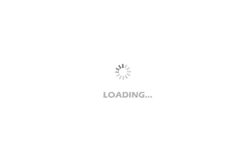
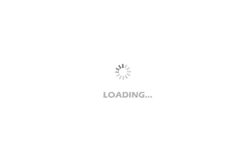
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Chip Manufacturing: A Practical Tutorial on Semiconductor Process Technology (Sixth Edition)
-
Traffic Light Control System Based on Fuzzy Control (Single-Chip Microcomputer Implementation)
-
Principles and Applications of Single Chip Microcomputers (Second Edition) (Wanlong)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Evaluation summary: Domestic FPGA Gaoyun GW1N series development board
- What is IoT technology?
- The Analog Engineer's Circuit Design Cookbook: Amplifiers
- TGF4042 Function Signal Generator Review: Unboxing and Trial
- Wireless Alliance names Wi-Fi 6E for 802.11ax networks using 6GHz spectrum
- Exposing unscrupulous merchants [Jimu Microelectronics Flagship Store]
- esp32,stm32: add machine.I2S to support I2S protocol
- Two AT24C02 read and write examples on the I2C bus
- High power driver amplifier 55453 replacement problem, please recommend
- 【McQueen Trial】Python Programming (3)