1. Simple foreground and background sequential execution of the program. This type of writing is the method used by most people. There is no need to think about the specific architecture of the program. You can directly write the application program through the execution sequence.
2. Time slice polling method, this method is between sequential execution and operating system.
3. Operating system, this method should be the highest level of application programming.
Let’s talk about the advantages, disadvantages and application scope of these three methods respectively. . . . . . . . . . . . .
1. Sequential execution method:
Let's write a sequential execution program model to compare it with the following two methods:
Code:
int main(void)
{
}
2. Time Slice Polling Method
Use 1 timer, which can be any timer. No special instructions are given here. Assuming there are 3 tasks, we should do the following:
1. Initialize the timer. Here we assume that the timer interrupt is 1ms (of course you can change it to 10ms. This is the same as the operating system. If the interrupt is too frequent, the efficiency will be low. If the interrupt is too long, the real-time performance will be poor).
2. Define a value:
Code:
#define TASK_NUM
uint16 TaskCount[TASK_NUM]
uint8
3. Add in the timer interrupt service function:
Code:
void TimerInterrupt(void)
{
}
Code explanation: The timer interrupt service function judges one by one in the interrupt. If the timer value is 0, it means that the timer is not used or the timer has completed the timing and no processing is required. Otherwise, the timer is reduced by one. When it reaches zero, the corresponding flag value is 1, indicating that the timer value of this task has expired.
4. In our application, add the following code where the application timing is required. Let’s take Task 1 as an example:
Code:
TaskCount[0] = 20;
TaskMark[0]
At this point, we only need to determine whether TaskMark[0] is 0x01 in the task
Through the above multiplexing of a timer, we can see that while waiting for a timing to arrive, we can loop to judge the flag bit and execute other functions at the same time.
Loop judgment flag:
So we can think about it, if the loop judges the flag bit, is it the same as the sequential execution program introduced above? A large loop, but this delay is more accurate than the ordinary for loop, and can achieve precise delay.
Execute other functions:
So if we execute other functions when a function is delayed, making full use of CPU time, isn't it similar to the operating system? However, the task management and switching of the operating system is very complicated. Next, we will use this method to build a new application.
The architecture of the time slice polling method:
1. Design a structure:
Code:
//Task structure
typedef struct _TASK_COMPONENTS
{
} TASK_COMPONENTS;
The design of this structure is very important. It uses 4 parameters and the comments are very detailed, so I will not describe it here.
2. The task running mark is displayed. This function is equivalent to the interrupt service function. This function needs to be called in the interrupt service function of the timer. It is separated here for easy transplantation and understanding.
Code:
void TaskRemarks(void)
{
}
Please compare this function carefully to see if it is the same as the timed multiplexing function above.
3. Task processing
Code:
void TaskProcess(void)
{
}
This function is used to determine when to execute a task and implement task management operations. The user only needs to call this function in the main() function and does not need to call and process task functions separately.
At this point, the architecture of a time-slice polling application has been built. Do you think it is very simple? This architecture only requires two functions and a structure. For application purposes, an enumeration variable will be created below.
Now I will talk about how to apply it. Suppose we have three tasks: clock display, key scanning, and working status display.
1. Define a structure variable like the one defined above
Code:
static TASK_COMPONENTS TaskComps[] =
{
};
When defining variables, we have initialized the values. The initialization of these values is very important and is related to the specific execution time priority, etc. You need to master this yourself.
① The general meaning is that we have three tasks, and the following clock display is executed every 1s. Because the minimum unit of our clock is 1s, it is enough to display it once after the second changes.
② Since the key will jitter when it is pressed, and we know that the jitter of a general key is about 20ms, then we usually extend it by 20ms in the sequential execution function. Here we scan once every 20ms, which is a very good result. It achieves the purpose of de-jittering and will not miss the key input.
③ In order to display other prompts and work interfaces after pressing the key, we design it to display once every 30ms. If you think the response is slow, you can make these values smaller. The names behind are the corresponding function names. You must write these functions and the same three tasks in the application.
2. Task List
Code:
//Task list
typedef enum _TASK_LIST
{
} TASK_LIST;
Take a closer look. The purpose of defining this task list here is actually the value of the parameter TASKS_MAX. Other values have no specific meaning and are only for the purpose of clearly showing the relationship between the surface tasks.
3. Write task functions
Code:
void TaskDisplayClock(void)
{
}
void TaskKeySan(void)
{
}
void TaskDispStatus(void)
{
}
// Add other tasks here. . . . . . . .
Now you can write tasks according to your needs.
4. Main function
Code:
int main(void)
{
}
At this point, the architecture of our time slice polling application is complete. You only need to add your own task function where we prompt. Isn't it very simple? Does it have a bit of operating system feeling in it?
3. Operating System
Code:
int main(void)
{
}
Code:
void TaskStart(void* p_arg)
{
#if (OS_TASK_STAT_EN > 0)
#endif
}
It is not difficult to see that the time slice polling method has great advantages, that is, it has the advantages of the sequential execution method and the operating system. The structure is clear, simple, and very easy to understand. . . . . . . . . .
Previous article:UART serial communication between two microcontrollers
Next article:Principle and Application of DS18B20
Recommended ReadingLatest update time:2024-11-23 10:59
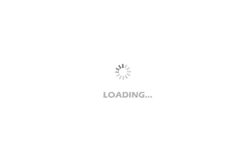
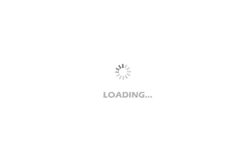
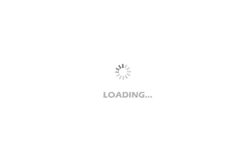
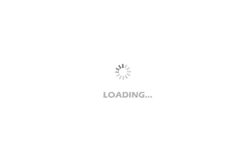
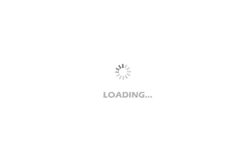
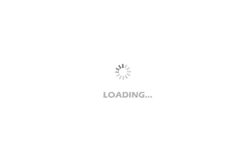
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- [RVB2601 Creative Application Development] Development Board Trial
- LED dot matrix screen, I want to ask you guys, why I only light up one light, but the whole row is lit
- In-depth analysis of T-BOX system solution: wireless connection unit
- 【Beetle ESP32-C3】1. Unpacking materials and lighting (Arduino)
- Online BootLoader method, no need to use C2000hex to get flash program data
- DSP Learning (3) TMS320VC5402 Principle and Application
- Discussion on fast crc8 calculation
- How to avoid pitfalls in GPIO operations?
- Share: Problems with using LM25119
- Please advise on the position of the rectifier bridge and common-mode inductor in power supply design