External interrupt is a very important internal resource of the microcontroller and is widely used. It is the high-speed switch sensor input interface of the microcontroller. It can detect pulse input, receive input signals from infrared remote controls, detect feedback signals from high-speed wheels or circular motion of motors, detect fleeting water drop signals in infusion sets, receive data information from analog serial ports, and so on.
This section will teach you two knowledge points:
The first one: the initialization code of external interrupt and the basic program template of interrupt function.
Second: When there are more than two interrupts in the system, how to set external interrupt 0 as the highest priority to realize the interrupt nesting function.
For details, please see the source code.
(1) Hardware platform:
Based on Zhu Zhaoqi 51 MCU learning board. Use S1 button as the falling edge pulse input of simulated external interrupt 0. Originally, S1 button is directly connected to P0^0 port, so P0^0 port must be connected to P3^2, the dedicated IO port of MCU external interrupt 0, through a jumper. Just remove the two yellow jumpers of P0^0 and P3^2, and connect P0^0 and P3^2 with a line. At this time, every time S1 button is pressed, a falling edge pulse will be generated for P3^2 port, and then the program will automatically jump to the interrupt function and execute once.
(2) Functions to be implemented:
Use the lower 4 digits of the digital tube to record the current falling edge pulse number. Use the S1 button through the jumper to simulate the falling edge input of the external interrupt 0. Each time you press it, the digital tube will display the number of pulses that are accumulated. Because there is jitter when the button is pressed, only a few pulses may be generated when it is pressed once, so you often see three or four data added at a time when you press it once. This experimental phenomenon is normal.
(3) The source code is explained as follows:
#include "REG52.H"
#define const_voice_short 40 //Duration of the buzzer short call
#define const_key_time1 20 //Key debounce delay time
void initial_myself();
void initial_peripheral();
void delay_short(unsigned int uiDelayShort);
void delay_long(unsigned int uiDelaylong);
//74HC595 driving the digital tube
void dig_hc595_drive(unsigned char ucDigStatusTemp16_09, unsigned char ucDigStatusTemp08_01);
void display_drive(); //Drive function for displaying digital tube fonts
void display_service(); //Display window menu service program
//74HC595 driving LED
void hc595_drive(unsigned char ucLedStatusTemp16_09, unsigned char ucLedStatusTemp08_01);
void T0_time(); //Timer interrupt function
void INT0_int(); //External 0 interrupt function
sbit key_gnd_dr=P0^4; //Simulate the ground GND of the independent key, so it must always output a low level
sbit beep_dr=P2^7; //Buzzer driver IO port
sbit dig_hc595_sh_dr=P2^0; //74HC595 program of digital tube
sbit dig_hc595_st_dr=P2^1;
sbit dig_hc595_ds_dr=P2^2;
unsigned char ucDigShow8; //The content to be displayed on the 8th digital tube
unsigned char ucDigShow7; //The content to be displayed on the 7th digital tube
unsigned char ucDigShow6; //The content to be displayed on the 6th digital tube
unsigned char ucDigShow5; //The content to be displayed on the 5th digital tube
unsigned char ucDigShow4; //The content to be displayed on the 4th digital tube
unsigned char ucDigShow3; //The content to be displayed on the third digital tube
unsigned char ucDigShow2; //The content to be displayed on the second digital tube
unsigned char ucDigShow1; //The content to be displayed on the first digital tube
unsigned char ucDigDot8; //Whether the decimal point of digital tube 8 is displayed
unsigned char ucDigDot7; //Whether the decimal point of digital tube 7 is displayed
unsigned char ucDigDot6; //Whether the decimal point of digital tube 6 is displayed
unsigned char ucDigDot5; //Whether the decimal point of digital tube 5 is displayed
unsigned char ucDigDot4; //Whether the decimal point of digital tube 4 is displayed
unsigned char ucDigDot3; //Whether the decimal point of digital tube 3 is displayed
unsigned char ucDigDot2; //Whether the decimal point of digital tube 2 is displayed
unsigned char ucDigDot1; //Whether the decimal point of digital tube 1 is displayed
unsigned char ucDigShowTemp=0; //temporary intermediate variable
unsigned char ucDisplayDriveStep=1; //Dynamic scanning digital tube step variable
unsigned char ucWd1Update=1; //Window 1 update display flag
unsigned char ucWd=1; //Core variable of this program, window display variable. Similar to the variable of the first-level menu. Represents different windows. This program has only one display window
unsigned int uiPluseCnt=0; //Variable for accumulating interrupt pulses in this program
unsigned char ucTemp1=0; //intermediate transition variable
unsigned char ucTemp2=0; //Intermediate transition variable
unsigned char ucTemp3=0; //intermediate transition variable
unsigned char ucTemp4=0; //intermediate transition variable
//Common cathode digital tube character table obtained based on the schematic diagram
code unsigned char dig_table[]=
{
0x3f, //0 Sequence number 0
0x06, //1 Sequence number 1
0x5b, //2 Sequence number 2
0x4f, //3 Sequence number 3
0x66, //4 Sequence number 4
0x6d, //5 Sequence number 5
0x7d, //6 Sequence number 6
0x07, //7 Serial number 7
0x7f, //8 Sequence number 8
0x6f, //9 Sequence number 9
0x00, //No sequence number 10
0x40, //- Sequence number 11
0x73, //P serial number 12
};
void main()
{
initial_myself();
delay_long(100);
initial_peripheral();
while(1)
{
display_service(); //Display window menu service program
}
}
void display_service() //Display window menu service program
{
switch(ucWd) //The core variable of this program, the window display variable. Similar to the variable of the first-level menu. Represents the display of different windows.
{
case 1: //Display the data of the first window. There is only one display window in this system.
if(ucWd1Update==1) //Window 1 needs to be fully updated
{
ucWd1Update=0; //Clear the flag in time to avoid continuous scanning
ucDigShow8=10; //The 8th digital tube displays no
ucDigShow7=10; //The 7th digital tube displays no
ucDigShow6=10; //The 6th digital tube displays no
ucDigShow5=10; //The 5th digital tube displays no
//Decompose the data first
ucTemp4=uiPluseCnt/1000;
ucTemp3=uiPluseCnt%1000/100;
ucTemp2=uiPluseCnt%100/10;
ucTemp1=uiPluseCnt%10;
//Transition the data to be displayed to the buffer variable, making the transition time as short as possible
//The following added if judgment is a slight modification to remove the high bit of 0 in the entire 4-bit data and not display it.
if(uiPluseCnt<1000)
{
ucDigShow4=10; //If less than 1000, thousands will be displayed
}
else
{
ucDigShow4=ucTemp4; //The content to be displayed on the 4th digital tube
}
if(uiPluseCnt<100)
{
ucDigShow3=10; //If it is less than 100, the hundreds place will be displayed
}
else
{
ucDigShow3=ucTemp3; //The content to be displayed on the third digital tube
}
if(uiPluseCnt<10)
{
ucDigShow2=10; //If less than 10, the tens digit will be displayed
}
else
{
ucDigShow2=ucTemp2; //The content to be displayed on the second digital tube
}
ucDigShow1=ucTemp1; //The content to be displayed on the first digital tube
}
break;
}
}
void display_drive()
{
// The following program can also compress the capacity if some arrays and shifted elements are added. However, what Hongge pursues is not capacity, but clear explanation ideas.
switch(ucDisplayDriveStep)
{
case 1: //Display the first position
ucDigShowTemp=dig_table[ucDigShow1];
if(ucDigDot1==1)
{
ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
}
dig_hc595_drive(ucDigShowTemp,0xfe);
break;
case 2: //Display the second digit
ucDigShowTemp=dig_table[ucDigShow2];
if(ucDigDot2==1)
{
ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
}
dig_hc595_drive(ucDigShowTemp,0xfd);
break;
case 3: //Display the third digit
ucDigShowTemp=dig_table[ucDigShow3];
if(ucDigDot3==1)
{
ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
}
dig_hc595_drive(ucDigShowTemp,0xfb);
break;
case 4: //Display the 4th digit
ucDigShowTemp=dig_table[ucDigShow4];
if(ucDigDot4==1)
{
ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
}
dig_hc595_drive(ucDigShowTemp,0xf7);
break;
case 5: //Display the 5th digit
ucDigShowTemp=dig_table[ucDigShow5];
if(ucDigDot5==1)
{
ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
}
dig_hc595_drive(ucDigShowTemp,0xef);
break;
case 6: //Display the 6th digit
ucDigShowTemp=dig_table[ucDigShow6];
if(ucDigDot6==1)
{
ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
}
dig_hc595_drive(ucDigShowTemp,0xdf);
break;
case 7: //Display the 7th position
ucDigShowTemp=dig_table[ucDigShow7];
if(ucDigDot7==1)
{
ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
}
dig_hc595_drive(ucDigShowTemp,0xbf);
break;
case 8: //Display the 8th digit
ucDigShowTemp=dig_table[ucDigShow8];
if(ucDigDot8==1)
{
ucDigShowTemp=ucDigShowTemp|0x80; //Display decimal point
}
dig_hc595_drive(ucDigShowTemp,0x7f);
break;
}
ucDisplayDriveStep++;
if(ucDisplayDriveStep>8) //After scanning 8 digital tubes, restart scanning from the first one
{
ucDisplayDriveStep=1;
}
}
//74HC595 driver function of digital tube
void dig_hc595_drive(unsigned char ucDigStatusTemp16_09, unsigned char ucDigStatusTemp08_01)
{
unsigned char i;
unsigned char ucTempData;
dig_hc595_sh_dr=0;
dig_hc595_st_dr=0;
ucTempData=ucDigStatusTemp16_09; //Send the high 8 bits first
for(i=0;i<8;i++)
{
if(ucTempData>=0x80)dig_hc595_ds_dr=1;
else dig_hc595_ds_dr=0;
dig_hc595_sh_dr=0; //The rising edge of the SH pin sends data to the register
delay_short(1);
dig_hc595_sh_dr=1;
delay_short(1);
ucTempData=ucTempData<<1;
}
ucTempData=ucDigStatusTemp08_01; //Send the lower 8 bits first
for(i=0;i<8;i++)
{
if(ucTempData>=0x80)dig_hc595_ds_dr=1;
else dig_hc595_ds_dr=0;
dig_hc595_sh_dr=0; //The rising edge of the SH pin sends data to the register
delay_short(1);
dig_hc595_sh_dr=1;
delay_short(1);
ucTempData=ucTempData<<1;
}
dig_hc595_st_dr=0; //The ST pin updates the data of the two registers and outputs them to the output pin of 74HC595 and latches them
delay_short(1);
dig_hc595_st_dr=1;
delay_short(1);
dig_hc595_sh_dr=0; //Pull low to enhance anti-interference
dig_hc595_st_dr=0;
dig_hc595_ds_dr=0;
}
void T0_time() interrupt 1 //Timer interrupt function
{
TF0=0; //Clear interrupt flag
TR0=0; //Disable interrupt
display_drive(); //Drive function of digital tube font
TH0=0xfe; //Reinstall initial value (65535-500)=65035=0xfe0b
TL0=0x0b;
TR0=1; //Open interrupt
}
/* Note 1:
* Use the S1 button in Zhu Zhaoqi 51 learning board as the falling edge pulse input of simulated external interrupt 0.
* The S1 button is directly connected to the P0^0 port, so the P0^0 port must be connected to the
* On the MCU external interrupt 0 dedicated IO port P3^2, just remove the two yellow jumpers P0^0 and P3^2, and use a
* Connect P0^0 and P3^2 with a wire. At this time, every time you press the S1 button, P3^2 will be
* Generate a falling edge pulse, and then the program will automatically jump to the following interrupt function and execute once.
* Because there is jitter when the button is pressed, only a few pulses may be generated when it is pressed once, so you often see three or four data added at a time.
* This kind of experimental phenomenon is normal.
*/
void INT0_int(void) interrupt 0 //INT0 external interrupt function
{
EX0=0; //Disable external 0 interrupt This is just my personal programming habit, you can also leave it open
uiPluseCnt++; //Accumulate the number of pulses on the falling edge of external interrupt
ucWd1Update=1; //Window 1 updates the display
EX0=1; //Open external 0 interrupt
}
void delay_short(unsigned int uiDelayShort)
{
unsigned int i;
for(i=0;i
{
; //A semicolon is equivalent to executing an empty statement
}
}
void delay_long(unsigned int uiDelayLong)
{
unsigned int i;
unsigned int j;
for(i=0;i
{
for(j=0;j<500;j++) //Number of empty instructions in the embedded loop
{
; //A semicolon is equivalent to executing an empty statement
}
}
}
void initial_myself() //Initialize the MCU
{
/* Note 2:
* The matrix keyboard can also be used as an independent key, provided that one of the common output lines outputs a low level.
* Simulate the triggering ground of an independent key. In this program, key_gnd_dr is outputted at a low level.
* S1 of Zhu Zhaoqi 51 learning board is an independent key used in this program.
* Connected to the external interrupt dedicated interface P3^2 of the microcontroller to simulate external falling edge pulse input.
*/
key_gnd_dr=0; //Simulate the ground GND of the independent key, so it must always output a low level
beep_dr=1; //Use PNP transistor to control the buzzer, it will not sound when the output is high level.
TMOD=0x01; //Set timer 0 to working mode 1
TH0=0xfe; //Reinstall initial value (65535-500)=65035=0xfe0b
TL0=0x0b;
}
void initial_peripheral() //Initialize the periphery
{
ucDigDot8=0; //No decimal points are displayed
ucDigDot7=0;
ucDigDot6=0;
ucDigDot5=0;
ucDigDot4=0;
ucDigDot3=0;
ucDigDot2=0;
ucDigDot1=0;
EX0=1; //Enable external interrupt 0
IT0=1; //Falling edge triggers external interrupt 0 If it is 0, it means low level interrupt
/* Comment 3:
* Note that this system uses two interrupts, one is a timer interrupt and the other is an external interrupt.
* Therefore, the IP register must be set to make external interrupt 0 the highest priority so that external interrupt 0 can interrupt
* Timed interrupts.
*/
IP=0x01; //Set external interrupt 0 to the highest priority, which can interrupt low-priority interrupt services. Implement interrupt nesting function
EA=1; //Open the general interrupt
ET0=1; //Enable timer interrupt
TR0=1; //Start timing interrupt
}
Closing remarks:
This section describes the basic program template of external interrupts. In the next section, I will talk about an example of a practical application of external interrupts. For more details, please listen to the next section for details: Using external interrupts to simulate serial port data transmission and reception.
Previous article:Section 65: Division of large data
Next article:Section 67: Using external interrupts to simulate serial port data transmission and reception
Recommended ReadingLatest update time:2024-11-16 22:32
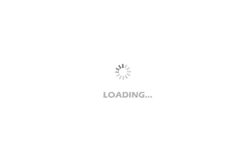
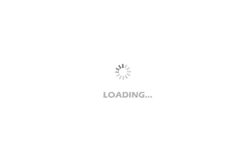
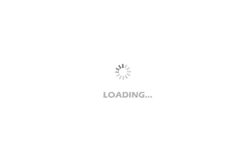
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- After reading this article from the Research Institute, I feel that 6-lane Wi-Fi still has a long way to go.
- Warning for trouble~~~~ la la la~ hurry to the front of the post to find out
- mcgs Kunlun Tongtai Modbus RTU, Modbus TCP communication method Modicon Modbus communication configuration steps
- Proficient in hardware and familiar with software
- This week's highlights
- [Nucleo G071 Review] SYSTICK & Comparison of Two Commonly Used Low Power Modes
- [Top Micro Smart Display Screen Review] 3. Run SciTE Lua script editor to test the screen alarm function
- 【TGF4042 signal generator】+Sweep application in issue 7
- Download and receive gifts | The era of artificial intelligence and the Internet of Things is coming, are you ready?
- 【TGF4042 signal generator】+ Basic function mode test