When using the controller to write a bare board application, the programmer only needs to understand the initialization sequence and content of the controller without understanding the specific details of the initialization to complete the application. Obviously, it can greatly improve work efficiency, and the specific details of the hardware are the most error-prone part of the application, and the use of the controller can greatly reduce the possibility of errors.
ARM is a more powerful single-chip microcomputer. In the process of learning bare metal programming, it is found that ARM integrates more peripherals than ordinary single-chip microcomputers (general single-chip microcomputers only integrate serial ports and four groups of IO ports), contains more GPIOs, and has more registers. The relevant pins are controlled by setting and enabling registers to control related devices. S3C2440 has an internal hardware controller integrated, and various driver protocols are generated by the hardware controller. We only need to configure the registers of the corresponding hardware controller to generate the corresponding driver timing. High-end ARM learning is mainly based on software programming (i.e., understanding). It has rich resources and rarely needs to expand peripherals. The focus of learning is how to configure registers and how to write applications.
Just set the corresponding control registers and read and write the corresponding data registers. The key to learning bare board program development is to understand the principles of various interface protocols, so that you can understand the initialization sequence and content of the controller.
The following is an example of the S3C2440 I2C controller. The following program is based on the interrupt mode. For the explanation of the I2C protocol, you can check my previous blog. The I2C protocol stipulates that after the receiver receives a byte (address or data), it must send an ACK. After the transmitter receives the ACK, it can continue to send data or end data transmission. After the receiver (here we are the S3C2440 I2C controller) receives the ACK, an interrupt will be generated. In the interrupt handler, you can continue to send the program or end the transmission. Understanding the basic principles of the I2C protocol, writing programs is a piece of cake.
- //===================================================================
- // SMDK2440 IIC configuration
- // GPE15=IICSDA, GPE14=IICSCL
- // "Interrupt mode" for IIC block
- //===================================================================
- //******************[ Test_Iic ]**************************************
- void Test_Iic(void)
- {
- unsigned int i,j,save_E,save_PE;
- static U8 data[256];
- Uart_Printf("\nIIC Test(Interrupt) using AT24C08\n");
- save_E = rGPECON; //Save the previous value
- rGPECON |= 0xa00000; //GPE15:IICSDA , GPE14:IICSCL
- pISR_IIC = (unsigned)IicInt; //Set the interrupt processing function
- rINTMSK &= ~(BIT_IIC); //Open IIC interrupt
- //Enable ACK, Prescaler IICCLK=PCLK/16, Enable interrupt, Transmit clock value Tx clock=IICCLK/16
- // If PCLK 50.7MHz, IICCLK = 3.17MHz, Tx Clock = 0.198MHz
- rIICCON = (1<<7) | (0<<6) | (1<<5) | (0xf); //The fourth bit is 1, the SCL line is pulled low, and the transmission is interrupted
- rIICADD = 0x10; //2440 slave address = [7:1]
- rIICSTAT = 0x10; //IIC bus data output enable (Rx/Tx) enable receive/send function
- rIICLC = (1<<2)|(1); // Filter enable, 15 clocks SDA output delay added by junon
- Uart_Printf("Write test data into AT24C08\n");
- for(i=0;i<256;i++)
- Wr24C080(0xa0,(U8)i,i);
- for(i=0;i<256;i++)
- data[i] = 0;
- Uart_Printf("Read test data from AT24C08\n");
- for(i=0;i<256;i++)
- Rd24C080(0xa0,(U8)i,&(data[i]));
- //Line changed 0 ~ f
- for(i=0;i<16;i++)
- {
- for(j=0;j<16;j++)
- Uart_Printf("%2x ",data[i*16+j]);
- Uart_Printf("\n");
- }
- rINTMSK |= BIT_IIC;
- rGPECON = save_E;
- }
- //*************************[ Wr24C080 ]****************************
- void Wr24C080(U32 slvAddr,U32 addr,U8 data)
- {
- _iicMode = WRDATA;
- _iicPt = 0;
- _iicData[0] = (U8)addr;
- _iicData[1] = data;
- _iicDataCount = 2;
- rIICDS = slvAddr; //0xa0
- rIICSTAT = 0xf0; //MasTx, Start host transmitter sends S signal
- //Clearing the pending bit isn't needed because the pending bit has been cleared.
- while(_iicDataCount!=-1);//Wait for data to be sent
- //Start a new transmission, but only send the device address and wait for a response to prove that the last transmission is complete. The next time Wr24C080 starts, it will start again. No data is written in this transmission.
- _iicMode = POLLACK;
- while(1)
- {
- rIICDS = slvAddr;
- _iicStatus = 0x100;
- rIICSTAT = 0xf0; //MasTx,Start
- rIICCON = 0xaf; //Resumes IIC operation.
- while(_iicStatus==0x100); //Wait for data to be sent and the status register to change.
- if(!(_iicStatus&0x1)) //The last bit received is 0 (ACK signal received)
- break; //When ACK is received, determine the status of the last bit
- }
- rIICSTAT = 0xd0; //Stop MasTx condition
- rIICCON = 0xaf; //Resumes IIC operation.
- Delay(1); //Wait until stop condtion is in effect.
- //Write is completed.
- }
- //**********************[ Rd24C080 ] ***********************************
- void Rd24C080(U32 slvAddr,U32 addr,U8 *data)
- {
- _iicMode = SETRDADDR;
- _iicPt = 0;
- _iicData[0] = (U8)addr;
- _iicDataCount = 1;
- rIICDS = slvAddr;
- rIICSTAT = 0xf0; //MasTx,Start
- //Clearing the pending bit isn't needed because the pending bit has been cleared.
- while(_iicDataCount!=-1);
- _iicMode = RDDATA;
- _iicPt = 0;
- _iicDataCount = 1;
- rIICDS = slvAddr;
- rIICSTAT = 0xb0; //MasRx,Start
- rIICCON = 0xaf; //Resumes IIC operation.
- while(_iicDataCount!=-1);
- *data = _iicData[1];
- }
- //-------------------------------------------------------------------------
- void __irq IicInt(void)
- {
- U32 iicSt,i;
- rSRCPND = BIT_IIC; //Clear pending bit
- rINTPND = BIT_IIC;
- iicSt = rIICSTAT;
- if(iicSt & 0x8){} //When bus arbitration is failed.
- if(iicSt & 0x4){} //When a slave address is matched with IICADD
- if(iicSt & 0x2){} //When a slave address is 0000000b
- if(iicSt & 0x1){} //When ACK isn't received
- switch(_iicMode)
- {
- case POLLACK:
- _iicStatus = iicSt;
- break;
- case RDDATA:
- if((_iicDataCount--)==0)
- {
- _iicData[_iicPt++] = rIICDS;
- rIICSTAT = 0x90; //Stop MasRx condition
- rIICCON = 0xaf; //Resumes IIC operation.
- Delay(1); //Wait until stop condtion is in effect.
- //Too long time...
- //The pending bit will not be set after issuing stop condition.
- break;
- }
- _iicData[_iicPt++] = rIICDS; //The last data has to be read with no ack.
- if((_iicDataCount)==0)
- rIICCON = 0x2f; //Resumes IIC operation with NOACK.
- else
- rIICCON = 0xaf; //Resumes IIC operation with ACK
- break;
- case WRDATA:
- if((_iicDataCount--)==0)
- {
- rIICSTAT = 0xd0; //Stop MasTx condition
- rIICCON = 0xaf; //Resumes IIC operation.
- Delay(1); //Wait until stop condtion is in effect.
- //The pending bit will not be set after issuing stop condition.
- break;
- }
- rIICDS = _iicData[_iicPt++]; //_iicData[0] has dummy.
- for(i=0;i<10;i++); //for setup time until rising edge of IICSCL
- rIICCON = 0xaf; //resumes IIC operation.
- break;
- case SETRDADDR:
- // Uart_Printf("[ S%d ]",_iicDataCount);
- if((_iicDataCount--)==0)
- break; //IIC operation is stopped because of IICCON[4]
- rIICDS = _iicData[_iicPt++];
- for(i=0;i<10;i++); //For setup time until rising edge of IICSCL
- rIICCON = 0xaf; //Resumes IIC operation.
- break;
- default:
- break;
- }
- }
Previous article:In-depth analysis of the big-endian and small-endian issues in the S3C2440 startup code
Next article:GPIO (II) C Program
Recommended ReadingLatest update time:2024-11-16 19:48
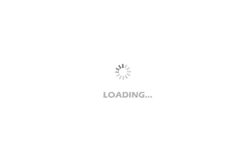
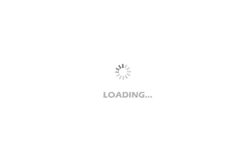
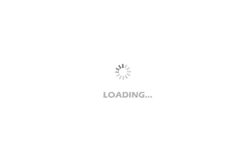
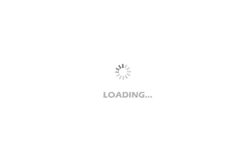
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Is there any solution to adjust the voltage accurately to 0.1V and start continuously and stably? ??? ? Help! ! ! !
- Learn about the internal structure of ST waterproof pressure sensor in this 32-second video
- 【Qinheng CH582】Evaluation Summary
- Introduction to oscilloscope related terms (Part 2)
- Electric curtain chip solution-single chip solution
- TMS320C5402 serial port initialization
- Some new changes that 5G brings to PA
- Experience in using Ti's C28x series DSP (28069) (28377D)
- EETalk: What pitfalls have you encountered during PCB design?
- Harbin Institute of Technology and Harbin Engineering University are banned from using genuine MATLAB software. What do you think about this?