IAR Embedded Workbench and AQ430) integrates C compiler and C language debugger C-SPY. However,
it is difficult to achieve accurate delay in C language, which has always troubled many MSP430
single-chip microcomputer programmers. In the actual project development process, the author encountered many interface devices that require strict timing control,
such as single bus digital temperature sensor DS18820, real-time clock chip PCF8563 (need to use ordinary 1/2C
bus timing), three-wire digital potentiometer AD8402, CF card (Compact Flash Card), etc., which all require μs or even nanons
level accurate delay; while some slow devices only need ms to s level delay. For this reason,
the author proposed a software or hardware accurate delay method suitable for different delay levels, and it has been actually applied with good results
, greatly shortening the development cycle.
1
sleeps in low-power mode when there is no external event trigger. When an external event arrives, an interrupt is generated to activate the CPU and enter the corresponding interrupt service program (
ISR). The interrupt response program only completes two tasks, one is to set the flag of the corresponding event, and the other is to make the MCU
exit the low-power mode. The main program is responsible for switching the MCU between the low-power mode and the event handler,
that is, an infinite loop is set in the main program, and the system enters the low-power mode directly after initialization. After the MCU is awakened, it
determines whether each flag is set. If a single flag is set, the MCU executes the corresponding event handler and
enters the low-power mode after completion; if multiple flags are set at the same time,
the main program judges and processes them in turn according to the pre-arranged message queue. After all events are processed, the MCU
sleeps and the system enters a low-power state (the order of the message queue is set according to the priority of the task importance)
. In this front-end and back-end system, since the main program is an infinite loop, the watchdog must be turned off. Instead of idling,
it is better to use its timer function for hardware delay. Using the MSP430
microcontroller watchdog timer to achieve an arbitrary length of accurate delay not only meets the system's real-time low power requirements,
but also makes up for the shortcomings of using an infinite loop delay that is difficult to determine and takes up a long CPU time. The following example explains
the techniques for completing delays of different lengths in the same WDT ISR.
#pragma vector=WD_r_VECTOR
}
If task 1 requires a 500 ms delay, just execute the following statement where the delay is required:
generates an interrupt request every 250 ms. The clock beat can be changed as needed. When using a 32768 Hz crystal oscillator as the clock source,
a delay base of 1.9ms, 16 ms, 250 ms and 1000 ms can be generated. In the header file msp430xl4x.h,
the WDT configuration macros for these four flip times are defined as: WDT_ADLY_1_9, WDT_ADLY_16, WDT_ADLY_250 and
WDT_ADLY_1000. If DCOCLK is used as the clock source of SMCLK, WDT selects SMCLK = 1 MHz as the clock source,
so that 0.064 ms, 0.5 ms, 8 ms and 32 ms delay bases can be used.
③ Always identify the Delay500ms bit in the DelayTime flag group. If it is in the set state,
it means that the required delay has not arrived. Perform no operation until the delay time is reached, reset Delay500ms in WDTISR, jump out of
the while() loop, and execute the next instruction.
Similarly, if task 2 requires a 30 s delay, WDT interrupt is activated by WDTCTL=WDT_ADLY_1000, and the interrupt
is performed every 1 s. In the WDT ISR, the flag is judged to be set to Delay30s instead of Delay500ms, and the 30 s
delay program branch is executed. Each time the interrupt is performed, the counter nS is increased by 1 until it reaches 30, indicating that the 30 s delay is completed, the counter is cleared, and
the watchdog is stopped (WETCTL=WETHOLD+WDTPW;) to stop the interrupt and reset the delay flag
to notify the task that the delay time is up, and the following instructions can be executed.
In the WDT ISR
, any length of time delay can be realized according to the combination of the delay base and the counter. When designing the system program,
first determine the different delay times required, and then
add the corresponding delay branch in the WDT ISR. The embedded real-time operating system μC/OS-II transplanted to the MSP430
microcontroller uses the watchdog timer to generate clock beats.
For a relatively simple system, only a single delay is required. If the system power consumption is considered,
another method of using the watchdog timer interrupt to complete the delay is introduced. If the delay is 1 s, set the WDT
to interrupt once every 250 ms. When the delay is required, start the watchdog timer and allow its interrupt, and the system enters the low power mode 3 (there are 5
modes in total) to sleep. In the interrupt service program, the delay time is accumulated, and when it reaches 1 s, the CPU is woken up
and the watchdog timer interrupt is stopped. The example code is as follows:
void main(void)
}
#pragrna vector=WDT_VECTOR
__interrupt void WDT_Delay(void)
{
IEII&=~WDTIE;)
}
This method makes full use of the ultra-low power consumption characteristics of the MSP430 series. During the waiting delay, the CPU
does not need to keep checking the flag to know that the delay has ended, but enters the power saving mode. During the waiting process,
only a very short time will accumulate time and make judgments in the interrupt service program. The CPU can be set to
enter different low-power modes LPMx as needed. If the system uses multiple peripheral interrupts
and there are statements to wake up the CPU in other interrupt service programs, this method is no longer applicable.
It is not suitable to use hardware delay for μs-level delays, because frequent in and out interrupts will cause the CPU
to spend a lot of time responding to interrupts and executing interrupt return operations. The hardware delay method is suitable for
long delays above the ms level.
2
In the operation of the digital temperature sensor DS18820, the delays used are: 15 μs, 90 μs, 270 μs, 540 μs
, etc. These delays are short and do not take up too much CPU time,
so they are more suitable for software delay methods. It is easy to control the time of a program written in assembly language.
We know the execution time of each statement,
the execution time of each macro, and the time consumed by each subroutine and the statement called. Therefore, to
compile a more accurate delay program in C language, it is necessary to study the assembly code generated by the C program.
Loop structure delay: The delay time is equal to the product of the instruction execution time and the number of instruction loops. For example,
the following delay program is experimentally analyzed.
while(time一一){};
In main(), call the delay function delayr(n); What is the delay time obtained? It is necessary to
compile and test in the integrated compilation environment IAR Embedded Workbench IDE 3.10A of the MSP430 microcontroller.
Use C430 to write a section of executable code, add a delay function to it, and call it in the main function. Take delay(1OO)
as an example. Set the project options Options, select Driver as Simulator in the Debugger column, and
perform software simulation. In the simulation environment C-SPY Debugger, call up the Disassembly and Register
windows from the View menu. The former displays the assembly program generated by the programming software based on the C language program. In the latter window, open the CPU
Register subwindow and observe the instruction cycle counter CYCLE-COUNTER. It can be seen that delay()
is compiled to obtain the following code segment:
00111A
single-step execution, observe the CYCLECOUNTER, and find that the value of CYCLECOUNTER increases by 1 for each instruction executed, indicating that these 5
instructions each take up 1 instruction cycle, and the loop body while() requires 5 instruction cycles each execution,
plus the function call and function return each take up 3 instruction cycles, delay(100) delays 5×100+6-506
instruction cycles. As long as the instruction cycle is known,
the delay time can be easily calculated. The execution time of the delay function varies due to different loop statements and compilers.
According to the above method, the purpose of flexible programming can be achieved.
The instruction execution speed of MSP430 is the number of cycles used by the instruction. The clock cycle here refers to
the cycle of the main system clock MCLK. After the microcontroller is powered on, if the clock system is not set, the default 800 kHz DCOCLK is
the clock source of MCLK and SMCLK, and LFXTl is connected to a 32768 Hz crystal and works in low-frequency mode (XTS=O) as the clock source of ACLK. The instruction cycle of the CPU
is determined by MCLK, so the default instruction cycle is 1/800 kHz = 1.25 μs. To get an
instruction cycle of lμs, you need to adjust the DCO frequency, that is, MCLK = 1 MHz, just set it as follows: BCSCTLl = XT20FF + RSEL2;
// Make the single instruction cycle lμs
It does not mean that the delay benchmark with the smallest delay of the MSP430 microcontroller software is lμs. When the XT2 = 8 MHz high-frequency oscillator is turned on,
the instruction cycle can reach 125 ns. The MSP430F4XX series of microcontrollers uses the enhanced frequency-locked loop technology FLL+, which
can double the DCO frequency to 40MHz, thereby obtaining the fastest instruction cycle of 25 ns.
The method of calling the delay function is suitable for delays between 100 μs and 1 ms.
Short delays below 100 μs are best achieved through the no-operation statement _NoP()
or any combination thereof. Macro definitions can be used to achieve the required delay. For example, if a delay of 3 μs is required, then: #define
DELAY5US{_NOP();_NOP();_NOP();}
Conclusion The hardware delay solution and software delay method based on the MSP430 on-chip watchdog timer
Previous article:Example of calculating the initial value of a microcontroller timer
Next article:Communication between MCU and 24C02
Recommended ReadingLatest update time:2024-11-23 10:59
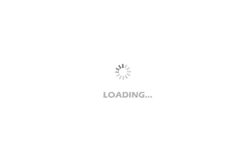
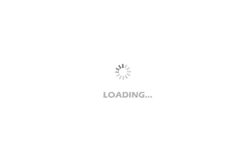
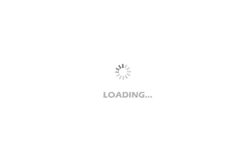
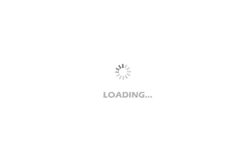
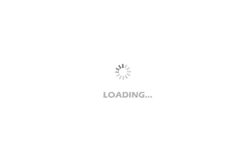
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- [Motor Kit P-NUCLEO-IHM] Part 2 SDK5.0 download and installation and personal suggestions for SDK5.0
- [Contactless face recognition access control system] + 6-EFR32BG22 using GPIO
- EEWORLD University ---- Linear Regulator_Low Dropout Regulator and Switching Power Supply Introduction
- MicroPython programming environment——Pycharm
- Serial port printing problem of domestic AC7801X chip (with program)
- EEWORLD University Hall--Introduction to the system and software and hardware implementation of the vehicle external amplifier
- Last 3 days: Apply for EC-01F-Kit, the official website of Essence, for free.
- [LPC8N04 Review] Unboxing Review
- Have you ever thought about the issue of program efficiency?
- Anyone who has used Eagle, please help me convert Eagle files into PADs files, thank you