Regarding the procedure for configuring SDRAM, since I have been studying it for 2 days, I will review it here at the end.
arm-linux-gcc -c -o head.o head.S
arm-linux-gcc -c -o leds.o leds.c
arm-linux-objdump -D -m arm sdram_elf > sdram.dis
bl disable_watch_dog @ Disable WATCHDOG, otherwise the CPU will restart continuously
bl memsetup @ Set up the storage controller
bl copy_steppingstone_to_sdram @ Copy code to SDRAM
ldr pc, =on_sdram @ Jump to SDRAM and continue execution
ldr sp, =0x34000000 @ Set up the stack
bl main
b halt_loop
@ Write 0 to the WATCHDOG register
mov r1, #0x53000000
mov r2, #0x0
str r2, [r1]
mov pc, lr @ return
@ Copy all Steppingstone 4K data to SDRAM
@ Steppingstone starts at 0x00000000, and SDRAM starts at 0x30000000
mov r1, #0
ldr r2, =SDRAM_BASE
mov r3, #4*1024
ldr r4, [r1], #4 @ Read 4 bytes of data from Steppingstone and add 4 to the source address
str r4, [r2], #4 @ Copy this 4-byte data to SDRAM and add 4 to the destination address
cmp r1, r3 @ Check whether it is completed: Is the source address equal to the unsigned address of Steppingstone?
bne 1b @ If not finished copying, continue
mov pc, lr @ return
@ Set up the memory controller to use peripherals such as SDRAM
mov r1, #MEM_CTL_BASE @ The starting address of the 13 registers of the storage controller
adrl r2, mem_cfg_val @ The starting storage address of these 13 values
add r3, r1, #52 @ 13*4 = 54
ldr r4, [r2], #4 @ Read the setting value and add 4 to r2
str r4, [r1], #4 @ Write this value to the register and add 4 to r1
cmp r1, r3 @ Determine whether all 13 registers have been set
bne 1b @ If not written, continue
mov pc, lr @ return
@ Storage controller 13 register settings
.long 0x22011110 @ BWSCON
.long 0x00000700 @ BANKCON0
.long 0x00000700 @ BANKCON1
.long 0x00000700 @ BANKCON2
.long 0x00000700 @ BANKCON3
.long 0x00000700 @ BANKCON4
.long 0x00000700 @ BANKCON5
.long 0x00018005 @ BANKCON6
.long 0x00018005 @ BANKCON7
.long 0x008C07A3 @ REFRESH
.long 0x000000B1 @ BANKSIZE
.long 0x00000030 @ MRSRB6
.long 0x00000030 @ MRSRB7
0: 43434700 movtmi r4, #14080; 0x3700
4: 5328203a teqpl r8, #58; 0x3a
8: 6372756f cmnvs r2, #465567744; 0x1bc00000
c: 20797265 rsbscs r7, r9, r5, ror #4
10: 202b2b47 eorcs r2, fp, r7, asr #22
14: 6574694c ldrbvs r6, [r4, #-2380]!
18: 30303220 eorscc r3, r0, r0, lsr #4
1c: 2d337138 ldfcss f7, [r3, #-224]!
20: 20293237 eorcs r3, r9, r7, lsr r2
24: 2e332e34 mrccs 14, 1, r2, cr3, cr4, {1}
28: Address 0x00000028 is out of bounds.
0: 00002541 andeq r2, r0, r1, asr #10
4: 61656100 cmnvs r5, r0, lsl #2
8: 01006962 tsteq r0, r2, ror #18
c: 0000001b andeq r0, r0, fp, lsl r0
10: 00543405 subseq r3, r4, r5, lsl #8
14: 01080206 tsteq r8, r6, lsl #4
18: 01140412 tsteq r4, r2, lsl r4
1c: 03170115 tsteq r7, #1073741829; 0x40000005
20: 01190118 tsteq r9, r8, lsl r1
24: Address 0x00000024 is out of bounds.
0: 43434700 movtmi r4, #14080; 0x3700
4: 5328203a teqpl r8, #58; 0x3a
8: 6372756f cmnvs r2, #465567744; 0x1bc00000
c: 20797265 rsbscs r7, r9, r5, ror #4
10: 202b2b47 eorcs r2, fp, r7, asr #22
14: 6574694c ldrbvs r6, [r4, #-2380]!
18: 30303220 eorscc r3, r0, r0, lsr #4
1c: 2d337138 ldfcss f7, [r3, #-224]!
20: 20293237 eorcs r3, r9, r7, lsr r2
24: 2e332e34 mrccs 14, 1, r2, cr3, cr4, {1}
28: Address 0x00000028 is out of bounds.
0: 00002541 andeq r2, r0, r1, asr #10
4: 61656100 cmnvs r5, r0, lsl #2
8: 01006962 tsteq r0, r2, ror #18
c: 0000001b andeq r0, r0, fp, lsl r0
10: 00543405 subseq r3, r4, r5, lsl #8
14: 01080206 tsteq r8, r6, lsl #4
18: 01140412 tsteq r4, r2, lsl r4
1c: 03170115 tsteq r7, #1073741829; 0x40000005
20: 01190118 tsteq r9, r8, lsl r1
24: Address 0x00000024 is out of bounds.
bl memsetup @ Set up the storage controller
bl copy_steppingstone_to_sdram @ Copy code to SDRAM
Keywords:ARM
Reference address:ARM Memory Configuration
First, add some basic knowledge:
Run address->Link address
When executing a program in SRAM or SDRAM, the PC points to this address, so the command should be in this address;
Load address -> store address
The address where the program is stored in NAND FLSAH
Position-independent codes: B, BL, MOV
Position related code: LDR PC, =Label
【About Makefile】
sdram.bin : head.S leds.c
arm-linux-ld -Ttext 0x30000000 head.o leds.o -o sdram_elf
arm-linux-objcopy -O binary -S sdram_elf sdram.bin
clean: rm -f sdram.dis sdram.bin sdram_elf *.o
Here we see this sentence "arm-linux-ld -Ttext 0x30000000 head.o leds.o -o sdram_elf", which means that the program is placed at the address 0x3000 0000. From the storage address of mini2440, we can know that it is the first address of SDRAM.
【head.S】(Configure SDRAM)
@****************************************************** ************************
@File:head.S
@ Function: Set up SDRAM, copy the program to SDRAM, and then jump to SDRAM to continue execution
@****************************************************** ************************
.equ MEM_CTL_BASE, 0x48000000
.equ SDRAM_BASE, 0x30000000
.text
.global _start
_start:
on_sdram:
halt_loop:
disable_watch_dog:
copy_steppingstone_to_sdram:
1:
memsetup:
1:
.align 4
mem_cfg_val:
【Disassembly】
sdram_elf: file format elf32-littlearm
Disassembly of section .text:
30000000 <_start>:
30000000:eb000005bl 3000001c
30000004: eb000010bl 3000004c
30000008:eb000007bl 3000002c
3000000c: e59ff090 ldr pc, [pc, #144] ; 300000a4
30000010 :
30000010: e3a0d30d mov sp, #872415232; 0x34000000
30000014: eb000035bl 300000f0
30000018 :
30000018: eafffffe b 30000018
3000001c :
3000001c: e3a01453 mov r1, #1392508928; 0x53000000
30000020: e3a02000 mov r2, #0 ; 0x0
30000024: e5812000 str r2, [r1]
30000028: e1a0f00e mov pc, lr
3000002c :
3000002c: e3a01000 mov r1, #0 ; 0x0
30000030: e3a02203 mov r2, #805306368; 0x30000000
30000034: e3a03a01 mov r3, #4096; 0x1000
30000038: e4914004 ldr r4, [r1], #4
3000003c: e4824004 str r4, [r2], #4
30000040: e1510003 cmp r1, r3
30000044: 1afffffb bne 30000038
30000048: e1a0f00e mov pc, lr
3000004c :
3000004c: e3a01312 mov r1, #1207959552; 0x48000000
30000050: e28f2018 add r2, pc, #24; 0x18
30000054: e1a00000 nop (mov r0,r0)
30000058: e2813034 add r3, r1, #52; 0x34
3000005c: e4924004 ldr r4, [r2], #4
30000060: e4814004 str r4, [r1], #4
30000064: e1510003 cmp r1, r3
30000068: 1afffffb bne 3000005c
3000006c: e1a0f00e mov pc, lr
30000070 :
30000070: 22011110 .word 0x22011110
30000074: 00000700 .word 0x00000700
30000078: 00000700 .word 0x00000700
3000007c: 00000700 .word 0x00000700
30000080: 00000700 .word 0x00000700
30000084: 00000700 .word 0x00000700
30000088: 00000700 .word 0x00000700
3000008c: 00018005 .word 0x00018005
30000090: 00018005 .word 0x00018005
30000094: 008c07a3 .word 0x008c07a3
30000098: 000000b1 .word 0x000000b1
3000009c: 00000030 .word 0x00000030
300000a0: 00000030 .word 0x00000030
300000a4: 30000010 .word 0x30000010
300000a8: e1a00000 .word 0xe1a00000
300000ac: e1a00000 .word 0xe1a00000
300000b0 :
300000b0: e52db004 push {fp}; (str fp, [sp, #-4]!)
300000b4: e28db000 add fp, sp, #0; 0x0
300000b8: e24dd00c sub sp, sp, #12; 0xc
300000bc: e50b0008 str r0, [fp, #-8]
300000c0: e51b2008 ldr r2, [fp, #-8]
300000c4: e3520000 cmp r2, #0 ; 0x0
300000c8: 03a03000 moveq r3, #0 ; 0x0
300000cc: 13a03001 movne r3, #1 ; 0x1
300000d0: e20310ff and r1, r3, #255; 0xff
300000d4: e2423001 sub r3, r2, #1; 0x1
300000d8: e50b3008 str r3, [fp, #-8]
300000dc: e3510000 cmp r1, #0 ; 0x0
300000e0: 1afffff6 bne 300000c0
300000e4: e28bd000 add sp, fp, #0; 0x0
300000e8:e8bd0800 pop {fp}
300000ec: e12fff1e bx lr
300000f0 :
300000f0: e92d4800 push {fp, lr}
300000f4: e28db004 add fp, sp, #4; 0x4
300000f8: e3a02456 mov r2, #1442840576; 0x56000000
300000fc: e2822010 add r2, r2, #16; 0x10
30000100: e3a03456 mov r3, #1442840576; 0x56000000
30000104: e2833010 add r3, r3, #16; 0x10
30000108: e5933000 ldr r3, [r3]
3000010c: e3c33bff bic r3, r3, #261120; 0x3fc00
30000110: e5823000 str r3, [r2]
30000114: e3a02456 mov r2, #1442840576; 0x56000000
30000118: e2822010 add r2, r2, #16; 0x10
3000011c: e3a03456 mov r3, #1442840576; 0x56000000
30000120: e2833010 add r3, r3, #16; 0x10
30000124: e5933000 ldr r3, [r3]
30000128: e3833b55 orr r3, r3, #87040; 0x15400
3000012c: e5823000 str r3, [r2]
30000130: e3a02456 mov r2, #1442840576; 0x56000000
30000134: e2822014 add r2, r2, #20; 0x14
30000138: e3a03456 mov r3, #1442840576; 0x56000000
3000013c: e2833014 add r3, r3, #20; 0x14
30000140: e5933000 ldr r3, [r3]
30000144: e3833e1e orr r3, r3, #480; 0x1e0
30000148: e5823000 str r3, [r2]
3000014c: e3a02456 mov r2, #1442840576; 0x56000000
30000150: e2822014 add r2, r2, #20; 0x14
30000154: e3a03456 mov r3, #1442840576; 0x56000000
30000158: e2833014 add r3, r3, #20; 0x14
3000015c: e5933000 ldr r3, [r3]
30000160: e3c33020 bic r3, r3, #32; 0x20
30000164: e5823000 str r3, [r2]
30000168: e3a00064 mov r0, #100; 0x64
3000016c:ebffffcfbl 300000b0
30000170: eafffff5 b 3000014c
Disassembly of section .comment:
00000000 <.comment>:
Disassembly of section .ARM.attributes:
00000000 <.ARM.attributes>:
[page]
sdram_elf: file format elf32-littlearm
Disassembly of section .text:
30000000 <_start>:
30000000:eb000005bl 3000001c
30000004: eb000010bl 3000004c
30000008:eb000007bl 3000002c
3000000c: e59ff090 ldr pc, [pc, #144] ; 300000a4
30000010 :
30000010: e3a0d30d mov sp, #872415232; 0x34000000
30000014: eb000035bl 300000f0
30000018 :
30000018: eafffffe b 30000018
3000001c :
3000001c: e3a01453 mov r1, #1392508928; 0x53000000
30000020: e3a02000 mov r2, #0 ; 0x0
30000024: e5812000 str r2, [r1]
30000028: e1a0f00e mov pc, lr
3000002c :
3000002c: e3a01000 mov r1, #0 ; 0x0
30000030: e3a02203 mov r2, #805306368; 0x30000000
30000034: e3a03a01 mov r3, #4096; 0x1000
30000038: e4914004 ldr r4, [r1], #4
3000003c: e4824004 str r4, [r2], #4
30000040: e1510003 cmp r1, r3
30000044: 1afffffb bne 30000038
30000048: e1a0f00e mov pc, lr
3000004c :
3000004c: e3a01312 mov r1, #1207959552; 0x48000000
30000050: e28f2018 add r2, pc, #24; 0x18
30000054: e1a00000 nop (mov r0,r0)
30000058: e2813034 add r3, r1, #52; 0x34
3000005c: e4924004 ldr r4, [r2], #4
30000060: e4814004 str r4, [r1], #4
30000064: e1510003 cmp r1, r3
30000068: 1afffffb bne 3000005c
3000006c: e1a0f00e mov pc, lr
30000070 :
30000070: 22011110 .word 0x22011110
30000074: 00000700 .word 0x00000700
30000078: 00000700 .word 0x00000700
3000007c: 00000700 .word 0x00000700
30000080: 00000700 .word 0x00000700
30000084: 00000700 .word 0x00000700
30000088: 00000700 .word 0x00000700
3000008c: 00018005 .word 0x00018005
30000090: 00018005 .word 0x00018005
30000094: 008c07a3 .word 0x008c07a3
30000098: 000000b1 .word 0x000000b1
3000009c: 00000030 .word 0x00000030
300000a0: 00000030 .word 0x00000030
300000a4: 30000010 .word 0x30000010
300000a8: e1a00000 .word 0xe1a00000
300000ac: e1a00000 .word 0xe1a00000
300000b0 :
300000b0: e52db004 push {fp}; (str fp, [sp, #-4]!)
300000b4: e28db000 add fp, sp, #0; 0x0
300000b8: e24dd00c sub sp, sp, #12; 0xc
300000bc: e50b0008 str r0, [fp, #-8]
300000c0: e51b2008 ldr r2, [fp, #-8]
300000c4: e3520000 cmp r2, #0 ; 0x0
300000c8: 03a03000 moveq r3, #0 ; 0x0
300000cc: 13a03001 movne r3, #1 ; 0x1
300000d0: e20310ff and r1, r3, #255; 0xff
300000d4: e2423001 sub r3, r2, #1; 0x1
300000d8: e50b3008 str r3, [fp, #-8]
300000dc: e3510000 cmp r1, #0 ; 0x0
300000e0: 1afffff6 bne 300000c0
300000e4: e28bd000 add sp, fp, #0; 0x0
300000e8:e8bd0800 pop {fp}
300000ec: e12fff1e bx lr
300000f0 :
300000f0: e92d4800 push {fp, lr}
300000f4: e28db004 add fp, sp, #4; 0x4
300000f8: e3a02456 mov r2, #1442840576; 0x56000000
300000fc: e2822010 add r2, r2, #16; 0x10
30000100: e3a03456 mov r3, #1442840576; 0x56000000
30000104: e2833010 add r3, r3, #16; 0x10
30000108: e5933000 ldr r3, [r3]
3000010c: e3c33bff bic r3, r3, #261120; 0x3fc00
30000110: e5823000 str r3, [r2]
30000114: e3a02456 mov r2, #1442840576; 0x56000000
30000118: e2822010 add r2, r2, #16; 0x10
3000011c: e3a03456 mov r3, #1442840576; 0x56000000
30000120: e2833010 add r3, r3, #16; 0x10
30000124: e5933000 ldr r3, [r3]
30000128: e3833b55 orr r3, r3, #87040; 0x15400
3000012c: e5823000 str r3, [r2]
30000130: e3a02456 mov r2, #1442840576; 0x56000000
30000134: e2822014 add r2, r2, #20; 0x14
30000138: e3a03456 mov r3, #1442840576; 0x56000000
3000013c: e2833014 add r3, r3, #20; 0x14
30000140: e5933000 ldr r3, [r3]
30000144: e3833e1e orr r3, r3, #480; 0x1e0
30000148: e5823000 str r3, [r2]
3000014c: e3a02456 mov r2, #1442840576; 0x56000000
30000150: e2822014 add r2, r2, #20; 0x14
30000154: e3a03456 mov r3, #1442840576; 0x56000000
30000158: e2833014 add r3, r3, #20; 0x14
3000015c: e5933000 ldr r3, [r3]
30000160: e3c33020 bic r3, r3, #32; 0x20
30000164: e5823000 str r3, [r2]
30000168: e3a00064 mov r0, #100; 0x64
3000016c:ebffffcfbl 300000b0
30000170: eafffff5 b 3000014c
Disassembly of section .comment:
00000000 <.comment>:
Disassembly of section .ARM.attributes:
00000000 <.ARM.attributes>:
1) First, we need to know the location of this program. It is located at the first address of NAND FLASH, which is 0x0000 0000. When we start from NAND FLASH, the first 4K code will be automatically copied to SRAM for execution.
Here, I encountered a problem that puzzled me for a long time: the running address of this code is 0x3000 0000, so how can it run at 0x0000 0000?
Answer: This is the position-independent code we mentioned above.
bl disable_watch_dog @ Disable WATCHDOG, otherwise the CPU will restart continuously
These 3 instructions can still be executed and complete the initialization of SDRAM
2) Now that the SDRAM has been initialized, that is, SDRAM can now help the memory process information, our instruction is
ldr pc, =on_sdram @ Jump to SDRAM and continue execution
This is our position-related code, which jumps from SRAM to SDRAM to continue execution and complete the operation;
【About experimental operation】
Oflash is used in the friendly video, but we now have a more powerful minitool tool, so I use minitool to download here, but several debugging failed. The reasons are as follows (the picture is borrowed from 2451, don't worry about these details, automatically replace it with 2440)
[This is the download method that failed to burn before]
As for the cause of the error, I don't know yet.
[The following is the correct burning posture...]
Previous article:ARM Interrupt Controller
Next article:ARM SecureCRT connects Windows and Linux
Recommended ReadingLatest update time:2024-11-16 22:39
Development of vehicle anti-theft device based on embedded technology
With the rapid development of my country's automobile industry, many families have their own private cars, but the number of garages is far from meeting the demand. Therefore, how to effectively prevent car theft is the most concerned issue for car owners. In recent years, science and technology have developed rapid
[Microcontroller]
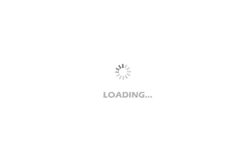
[Embedded] arm-linux-gcc/ld/objcopy/objdump parameter overview
arm-linux-gcc -o only activates preprocessing, compilation, and assembly, that is, it only makes the program into an obj file -Wall specifies to generate all warning messages -O2 The compiler provides a compilation optimization option for the program. Using this option during compilation can improve the exec
[Microcontroller]
ARM instruction classification (details)
instruction: Data processing instructions can only operate on register contents, not memory contents. All data processing instructions can use the suffix s to affect the flag bits Data processing instructions Data transfer instructions Arithmetic and logical operation instructions Comparison Instructions Jump Inst
[Microcontroller]
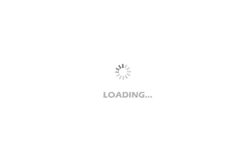
ARM I2C bus interface circuit and timing
ARM I2C bus interface circuit and timing
ARM I2C bus consists of a data line SDA (serial data line) and a clock line SCL (serial clock line). Each circuit module is hung on the SDA and SCL lines of the I2C bus to exchange data with the main chip. The input end of the interface circuit on the I2C bus must be
[Microcontroller]
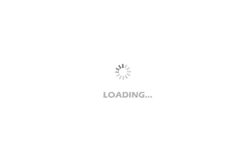
Understanding of ARM's definition of special registers *(volatile unsigned long *))
I used to not understand the (*(volatile unsigned long *)) in ARM programs. Today, I will analyze this type conversion by consulting materials and reading articles written by others. This usage is not only useful in defining internal special registers, but also in defining the addresses of external devices when
[Microcontroller]
Design of smart home monitoring system based on ARM
1 Introduction Information technology has been widely used in all aspects of people's lives. People have higher and higher requirements for their family living environment. Smart homes have emerged. Compared with ordinary homes, smart homes not only have traditional living functions, but also provide comfortable, s
[Microcontroller]
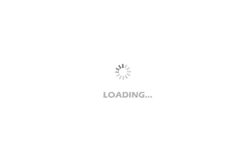
ARM Study Notes 11——GNU ARM Assembly Programming
In GNU ARM assembly programming, the syntax format of each line is as follows: @comment If the statement is too long, you can write it in several lines, and use "" at the end of the line to indicate a line break. There cannot be any characters after "", including spaces and tabs. Parameter description:
[Microcontroller]
Hardware Design of an Embedded Network Video Monitoring System
1. Introduction
The monitoring system using embedded network technology is the latest development trend in the monitoring field. The embedded network monitoring system is a high-tech product that is a combination of the rapid development of electronic technology, computer technology, communication technology
[Microcontroller]
Recommended Content
Latest Microcontroller Articles
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
MoreDaily News
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
Guess you like
- What are the methods of remote communication? Which manufacturers have used specific products?
- Regarding "Circuit Design Based on Operational Amplifiers and Analog Integrated Circuits", there is a concept of "Pole" that is not clear
- RISC-V IDE MRS usage notes (Part 3): Improving floating-point calculation efficiency
- Pan it STM32H750 3 (lan8720 Ethernet test --iperf)
- Another question about the matrix keyboard
- Understanding of MSP430 interrupt mechanism
- Understand some misunderstandings about FPGA learning
- Transmit power measurement method
- My Journey of MCU Development (VI)
- [RVB2601 Creative Application Development] 4 Long press and short press to eliminate the letter A