#define C02MasterAddr 0xa0 //C02 device address
#define C02SlaveAddr 0 //Starting address for reading and writing data
#define LED (1 << 19) //LED IO number
#define LED2 (1 << 20)
#define AA (1 << 2) //Definition of each bit of I2CONSET
#define SI (1 << 3)
#define STO (1 << 4)
#define STA (1 << 5)
#define I2EN (1 << 6)
#define WRMode 0 //Write mode
#define RDMode 1 //Read mode
#define lastbyte 1 //Is it the last byte
uint8 I2C_buf1 = 5;
uint8 I2C_buf2 = 0;
//Delay function
void Delay(uint32 dly)
{
uint32 i;
for(; dly > 0; dly--)
{
for(i = 0; i < 50000; i++);
}
}
//I2C initialization function
void I2C_Init(uint32 Fi2c)
{
if(Fi2c > 400000)
{
Fi2c = 400000;
}
PINSEL0 = (PINSEL0 & (~0xf0)) | 0x50;//Here is to let P02, P03 select the second function, that is, I2C bus
I2SCLH = (Fpclk/Fi2c + 1) / 2;//Set I2C speed to 400K
I2SCLL = (Fpclk/Fi2c) / 2;
I2CONCLR = 0x2c; //Master transmission mode clears STA, AA, SI
I2CONSET = 0x40;//Use I2C bus
// I2CONCLR = STA|AA|SI;
// I2CONSET = I2EN;
}
//I2C bus start function
void I2C_Start(void)
{
I2CONSET = STA;//set start condition
do{}while(0x08 != I2STAT);//A start condition has been transmitted
I2CONCLR = STA;//clear start condition
}
//I2C bus stop function
void I2C_Stop(void)
{
I2CONSET = STO; //set STO condition
I2CONCLR = SI; //clear SI condition
}
//write byte function
void WriteByte(uint8 data)
{
I2DAT = data;// data transmitted
I2CONCLR = SI;//clear SI condition
}
//wirte I2C bus address mode is RDMode or WRMode
void WriteAddr(uint8 Mode)
{
WriteByte(C02MasterAddr + Mode);//transmite the I2C address and R/W mode
if(Mode)
{
do{}while(0x40 != I2STAT);//SLA+R has been transmitted;ACK has been received
}
else
{
do{}while(0x18 != I2STAT);//SLA+W has been transmitted;ACK has been received
}
}
//write data to I2C
void WriteData(uint8 data)
{
WriteByte(data);// data is will transmitted data
do{}while(0x28 != I2STAT);//Data byte in I2DAT has been transmitted; ACK has been received
}
//read i2c byte
uint8 ReadByte(uint8 last)
{
if(last)
{
I2CONCLR = AA;
I2CONCLR = SI;
do{} while(0x58 != I2STAT);//data byte has been received;NOT ACK has been returned
}
else
{
I2CONSET = AA;//transmited ACK
I2CONCLR = SI;
do{}while(0x50 != I2STAT);//data byte has been received;ACK has been returned.
}
return I2DAT;//return read data
}
//write data into 24c02
void WriteC02(void)
{
// uint8 i;//define unsigned char i
I2C_Start();
WriteAddr(WRMode);
WriteData(C02SlaveAddr);//the data stor address
WriteData(I2C_buf1);
I2C_Stop();
}
//read I2C data
void ReadC02(void)
{
// uint8 i;
I2C_Start();
WriteAddr(WRMode);
WriteData(C02SlaveAddr);
I2C_Stop();
I2C_Start();
WriteAddr(RDMode);
I2C_buf2 = ReadByte(lastbyte);
I2C_Stop();
}
int main(void)
{
PINSEL0 = 0x00000000;
PINSEL1 = 0x00000000;
IODIR = LED|LED2;
IOSET = LED|LED2;
I2C_Init(400000);
WriteC02();
Delay(1000);
ReadC02();
if(I2C_buf2 == I2C_buf1)
{
while(1){
IOCLR = LED;//correct LED1 light
Delay(50);
IOSET = LED;
Delay(50);
}
}
else
{
while(1)
{
IOCLR = LED2;//error LED2 light
Delay(50);
IOSET = LED2;
Delay(50);
}
}
return 0;
}
Previous article:ADS_LPC2103 development board SPI 4-bit digital tube test experiment
Next article:First Example of IAR ARM Based on LPC2131: LED Blinking
Recommended ReadingLatest update time:2024-11-16 09:01
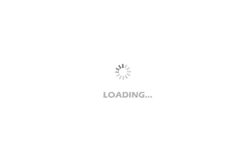
- Popular Resources
- Popular amplifiers
-
Operational Amplifier Practical Reference Handbook (Edited by Liu Changsheng, Zhao Mingying, Liu Xu, etc.)
-
A review of deep learning applications in traffic safety analysis
-
A review of learning-based camera and lidar simulation methods for autonomous driving systems
-
ARM Embedded System Principles and Applications (Wang Xiaofeng)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- What are risc-v chips?
- sensortile box source code
- Lingdongwei MM32 download program error description solution
- Double 12 limited time offer | Tektronix year-end rewards, oscilloscope third-order upgrade!
- Driver chip in Type-c OTG mode - LDR6028S
- The pressure sensor outputs the pressure value in a table
- Application of power amplifier in R&D and testing of high voltage side power supply of current transformer
- C/C++ Memoirs
- 5G RF Front-end Solutions
- Teach you how to simply judge whether the transformer is good or bad