/*This program is an eight-bit common cathode digital tube with two 573 controlled dynamic scans*/
#include
#include
#define uchar unsigned char
#define uint unsigned int
//Note: The function of code is to store the following data in the program memory. If code is not used, it will be placed in the random access memory.
#pragma data:code
const table[]={0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d, 0x07,
0x7f,0x6f,0x77,0x7c,0x39,0x5e,0x79,0x71};//Without decimal point
const table2[]={0xbf,0x86,0xdb,0xcf,0xe6,
0xed,0xfd,0x87,0xff,0xdf};//Decimal point encoding
/*If uchar table[] is used, it will be placed in the data memory. Never use it this way, it will take up more space. */
/*Two 573, segment code PA3, bit code PA4*/
//Connect 18B20 DQ to PC2
#define DQ_IN DDRC&=~BIT(2)
//The previous sentence is to set DQ as input
#define DQ_OUT DDRC|=BIT(2)
//The previous sentence is to set DQ as output
#define DQ_SET PORTC|=BIT(2)
//The previous sentence is to set DQ to high level
#define DQ_CLR PORTC&=~BIT(2)
//The previous sentence is to set DQ to low level
#define DQ_RD PINC&BIT(2)
//The previous sentence is to read data from PC2uchar
disdata[4];
void delay(uint ms)
{
uint i,j;
for(i=ms;i>0;i--)
for(j=220;j>0;j--);
}
void delayus(uint us)
{
while(us--);
}
void show(uchar j,uchar k)
{
DDRA|=BIT(3);
DDRA|=BIT(4);
DDRB=0XFF;
PORTA|=BIT(3);
PORTB=table[j];
PORTA&=~BIT(3);
PORTB
=0XFF; PORTB&
=
~BIT
(k
);
void show_point(uchar j,uchar k)
{
DDRA|=BIT(3);
DDRA|=BIT(4);
DDRB=0XFF;
PORTA|=BIT(3);
PORTB=table2[j];
PORTA&=~BIT(3);
PORTB=0XFF;
PORTB&=~BIT(k);
PORTA|=BIT(4);
PORTA&=~BIT(4);
delay(5);
}
rest_18B20()
{
uchar i ;
DQ_OUT;//Set DQ as output
DQ_SET;//Pull DQ high
delayus(5);
DQ_CLR;//Pull DQ low
delayus(800);
DQ_SET;//Pull DQ high
//delayus(550);
DQ_IN;//Set DQ as input
i=DQ_RD;
delayus(800);
return i;
}
void write_18B20(uchar dat)
{
uchar i;
for(i=0;i<8;i++)
{
DQ_OUT;//Set DQ as output
DQ_CLR;//Pull DQ low
delayus(30);
if(dat&0x01)//Judge whether the current bit is high, if it is high, execute the following statement
{
DQ_SET;//Pull DQ high, let the bus sample to 1
}
else
{
DQ_CLR;//Pull DQ low
}
delayus(80);
DQ_SET;//Release the bus
dat=dat>>1;
}
}
uchar read_18B20()
{
uchar i,value;
for(i=0;i<8;i++)
{
DQ_OUT;//Set DQ as output
DQ_CLR;//Pull DQ low
value=value>>1;
delayus(10);
DQ_SET;//Release the bus and wait for MCU to sample
DQ_IN;//Set DQ as input to sample
if(DQ_RD)
{
value|=0x80;
}
delayus(100);
// DQ_SET;//Release the bus
}
return value;
}
void display(uint tvalue)
{
disdata[0]=tvalue/1000;//hundreds
disdata[1]=tvalue%1000/100;//tens
disdata[2]=tvalue%100/10;//units
disdata[3]=tvalue%10;//decimal places
show(disdata[0],0);
delay(2);
show(disdata[1],1);
delay(2);
show_point(disdata[2],2);
//show(0x80,2);
show(disdata[3],3);
//The following display method can also be
used/* uint i,temp[4];
for(i=0;i<4;i++)
{
temp[3-i]=dat%10;
dat=dat/10;
}
for(i=0;i<4;i++)
{
show(temp[i],i);
delay(2);*/
/* // The following three sentences are used to turn off the display and are used for dynamic display
DDRA|=BIT(3);
DDRA|=BIT(4);
DDRB=0XFF;
}*/
}
void main()
{int L,H;
uint tvalue;
while(1)
{
rest_18B20();
write_18B20(0xcc);//skip reading the serial number
write_18B20(0x44);//start temperature conversion
delayus(50);
//reset again before reading
rest_18B20();
write_18B20(0xcc);//skip reading the serial number
write_18B20(0xbe);//start sampling, read the register
delayus(50);
L=read_18B20();//read the lower eight bits first
H=read_18B20();//read the higher eight bits later
tvalue=H;
tvalue<<=8;
tvalue=tvalue|L;
tvalue=tvalue*0.625;//Expand 10 times
display(tvalue);
}
}
Previous article:AVR on-chip TWI bus (I2C bus protocol)
Next article:Atmega16 and DS1302 digital tube display program
Recommended ReadingLatest update time:2024-11-16 19:46
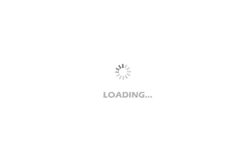
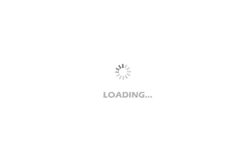
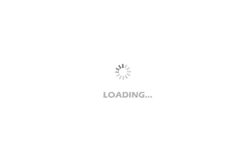
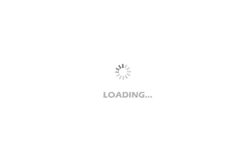
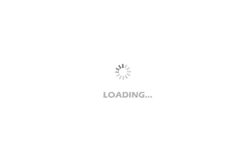
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- IEC61000-4-5 coupling mode
- Brushless servo, no Hall drive, electric vehicle controller (with Hall sine wave)
- [AT-START-F403A Review] 5. Simulate the SPI screen and find a problem
- [RT-Thread Reading Notes] RT-Thread Reading Notes Summary
- Build it yourself? Huawei is openly recruiting "lithography engineers"
- ST announces price increase
- [Smart Wardrobe] Material Unboxing ESP32-S3-BOX
- A valve is used in the water supply of the product. The valve is opened when water is used and closed when water is not used. How to measure the force of the opening and closing valve?
- Taiwan Sun Yat-sen University ASIC Laboratory Comprehensive Script Tutorial
- MSP430 Program Library--Digital Tube Display