typedef unsigned char uchar;
typedef unsigned int uint;
/*****18B20 interface definition********/
#define DQ1 P1OUT |= BIT6
#define DQ0 P1OUT &= ~BIT6
#define DQ_in P1DIR &= ~BIT6
#define DQ_out P1DIR |= BIT6
#define DQ_val (P1IN & BIT6)
/*****Digital tube interface definition********/
#define wei_h P5OUT|= BIT5
#define wei_l P5OUT&= ~BIT5
#define duan_l P6OUT &= ~BIT6
#define duan_h P6OUT |= BIT6
//Digital tube seven-segment code; 0--f
uchar table[16] = {0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,
0x7f,0x6f,0x77,0x7c,0x39,0x5e,0x79,0x71};
uchar table1[16] = {0xbf,0x86,0xdb,0xcf,0xe6,0xed,0xfd,
0x87,0xff,0xef,0xf7,0xfc,0xb9,0xde,0xf9,0xf1}; // a bit
uchar tflag,num=0 ;
int tvalue;
uchar disdata[4];
/***********18B20 part of the program******************/
/************************************************
Function name: DelayNus
function Function: Achieve N microseconds of delay
Parameter: n--delay length
Return value: None
Description: The counting clock of timer A is 1MHz, and the CPU main frequency is 8MHz
, so extremely accurate
us-level delay can be obtained through timer delay
********************************************/
void DelayNus(uint n)
{
CCR0 = n;
TACTL |= MC_1; //Increase count to CCR0
while(!(TACTL & BIT0)); //Wait for
TACTL &= ~MC_1; //Stop counting
TACTL &= ~BIT0; //Clear interrupt flag
}
/*******************************************
Function name: Init_18B20
Function: Reset DS18B20
Parameter: None
Return value: Initialization status flag: 1-failure, 0-success
****************************************/
uchar Init_18B20(void)
{
uchar Error;
DQ_out;
_DINT();
DQ0;
DelayNus(500);
DQ1;
DelayNus(55);
DQ_in;
_NOP();
if(DQ_val)
{
Error = 1; // Initialization failed
}
else
{
Error = 0; // Initialization successful
}
DQ_out;
DQ1;
_EINT();
DelayNus(400);
return Error; // If Error = 1 here, there will be an infinite loop later, indicating that 18B20 may be broken
}
/***********************************************
Function name: Write_18B20Function
: Write a byte of data to DS18B20
Parameter: wdata--Written data
Return value: None
********************************************/
void Write_18B20(uchar wdata)
{
uchar i;
_DINT();
for(i = 0; i < 8;i++)
{
DQ0;
DelayNus(6); //Delay 6us
if(wdata & 0X01) DQ1;
else DQ0;
wdata >>= 1;
DelayNus(50); //Delay 50us
DQ1;
DelayNus(10); //Delay 10us
}
_EINT();
}[page]
/*******************************************
Function name: Read_18B20Function
: Read one byte of data from DS18B20
Parameter: None
Return value: One byte of data read out
****************************************/
uchar Read_18B20(void)
{
uchar i;
uchar temp = 0;
_DINT();
for(i = 0;i < 8;i++)
{
temp >>= 1;
DQ0;
DelayNus(6); //Delay 6us
DQ1;
DelayNus(8); //Delay 9us
DQ_in;
_NOP();
if(DQ_val) temp |= 0x80;
DelayNus(45); //Delay 45us
DQ_out;
DQ1;
DelayNus(10); //Delay 10us
}
_EINT();
return temp;
}
uint Do1Convert(void)
{
uchar i;
uchar temp_low;
uint temp;
do
{
i = Init_18B20();
}
while(i);
//The i here is equal to the previous Error. If Error = 1, an infinite loop will occur, indicating that 18B20 may be broken.
Write_18B20(0xcc); //Send the command to skip reading the product ID number
Write_18B20(0x44); //Send the temperature conversion command
for(i = 20;i > 0;i--)
DelayNus(60000); //Delay for more than 800ms
do
{
i = Init_18B20();
}
while(i);
//The i here is equal to the previous Error. If Error = 1, an infinite loop will occur, indicating that 18B20 may be broken.
Write_18B20(0xcc); //Send the command to skip reading the product ID number
Write_18B20(0xbe); //Send the command to read ROM
temp_low = Read_18B20(); //Read the low bit
temp = Read_18B20(); //Read the high bit
temp = (temp<<8) | temp_low;
if(temp<0x0fff)
tflag=0;
else
{ temp=~temp+1;
tflag=1;
}
tvalue=temp*(0.625); //Expand the temperature value by 10 times and be accurate to 1 decimal place
return tvalue;
}
void display(int dat)
{
disdata[0]=dat/1000;
disdata[1]=dat%1000/100;
disdata[2]=dat%100/10;
disdata[3]=dat%10;
}
/****************Main function********************/
void main(void)
{
/*The following six lines of program close all IO ports*/
P5DIR = 0xff;
=P5OUT = 0xff;P1DIR = 0XFF;P1OUT = 0XFF; P2DIR = 0XFF;
P2OUT = 0XFF; P3DIR = 0XFF;
P3OUT = 0XFF;
P4DIR = 0XFF;P4OUT = 0XFF;
P5DIR = 0XFF;P5OUT = 0XFF;
P6DIR = 0XFF;P6OUT = 0XFF;
uchar i;
WDTCTL=WDTPW+WDTHOLD;
/*------Select system master clock as 8MHz-------*/
BCSCTL1 &= ~XT2OFF; //Turn on XT2 high frequency crystal oscillatordo
{
IFG1
&= ~OFIFG; //Clear crystal failure flagfor
(i = 0xFF; i > 0; i--); //Wait for 8MHz crystal to start oscillating
}
while ((IFG1 & OFIFG)); //Does the crystal failure flag still exist?
BCSCTL2 |= SELM_2 + SELS; //MCLK and SMCLK select high frequency crystal oscillator
/*P6DIR |= BIT6;P6OUT |= BIT6; //Disable level conversion
P5DIR |= BIT5;P5OUT |= BIT5; //Disable level conversion
P6DIR |= BIT7;P6OUT |= BIT7; //Disable buzzer*/
// Set watchdog timer and initialize IO to control digital tube
WDTCTL = WDT_ADLY_1_9;
IE1 |= WDTIE;
//Count clock selects SMLK=8MHz, 1MHz after 1/8 division
TACTL |= TASSEL_2 + ID_3;
//Open global interrupt_EINT
();
//Loop reading displaywhile
(1)
{
display(Do1Convert());
}
}
/*******************************************
Function name: watchdog_timer
Function: Watchdog timer interrupt service function,
dynamic scanning of digital tube
Reference Number: None
Return value: None
********************************************/
#pragma vector = WDT_VECTOR
__interrupt void watchdog_timer(void)
{
P4OUT = table[disdata[num]];
if(num==2) P4OUT = table1[disdata[num]]; //Add decimal point
duan_h;
duan_l;
P4OUT = ~(1<
wei_l;
num++;
if(num == 4) num = 0;
}
Previous article:Clock written with 32768Hz crystal oscillator of MSP430
Next article:MSP430 and DS1302 1602 display
Recommended ReadingLatest update time:2024-11-16 13:54
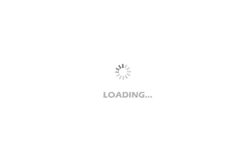
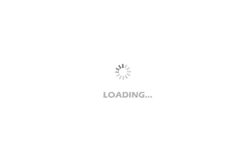
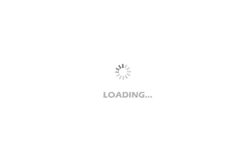
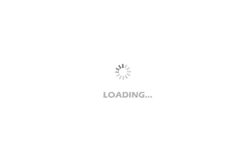
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- How to choose between different chips in TI's Sub-1GHz product line?
- CircuitPython Electronic Chain Bracelet
- "C Programming Magic Book: Based on C11 Standard"
- [Project Source Code] 3 methods to display state machine names in Modelsim simulation based on FPGA
- Temperature sensor + I2C + serial port + PC host (pyserial) example
- MSP430G2553 internal ADC principle and routine description
- Types of PCB substrates
- What is the difference between an AD schematic and a schematic library?
- Review Weekly Report 20220725: Allwinner 40i development board with 100% domestic components is here, Canaan K510 will be available this Thursday~
- Here is the ultrasound information on the national competition list