BootLoader is a small boot program that is executed first after the microcontroller is reset. This program can be used to initialize the hardware and update the "user program". This article mainly discusses the dynamic update of the "user program" through BootLoader.
In the process of using single-chip microcomputers for product development and use, the problem of updating programs is inevitable. Normal program download is achieved by connecting the single-chip microcomputer emulator to the special I/O port of the single-chip microcomputer. In the development stage of the product, program download and debugging can be achieved through the emulator. After the product development is completed, since the single-chip microcomputer has been encapsulated inside the product, if you want to update it, you need to reopen the product shell and connect the data cable. This is almost impossible when the product has been mass-produced or even in the hands of the end user. On the one hand, this is because it is very inefficient and costly, and on the other hand, it also brings a great negative impact on the overall performance of the product to users.
1 Flash operation and program storage area structure and function division
1.1 Flash basic operations and storage structure
C8051F410 only supports 0 operation for Flash, so the sector should be erased before writing data (erasing can only be done on the entire page, and each bit is 1 after the operation is completed). Software writing and erasing FLASH is protected by the FLASH lock and key code function. Before performing FLASH operations, the key code must be written to the FLASH lock and key code register (FLKEY) in sequence: 0xA5, 0xF1. The timing of writing the key code is not important, but it must be written in sequence.
To implement BootLoader, we must first understand the Flash structure of the program storage, as shown in Table 1. C8051F410 has a total of 32k Flash program memory, which is divided into 512 sectors (pages) and can be programmed in the system (IAP). This provides the necessary and sufficient prerequisites for implementing the BootLoader function.

1.2 Functional division of storage area
The BootLoader program and the "user program" are stored in different areas of the Flash, which are divided as follows in this article: The BootLader program occupies addresses 0x6000 to 0x7FFF, of which page 0x7C00 is used to save the entry address of the user program, so that the size of the real BootLoader program cannot exceed 7k (0x6000 to 0x7A00). The "user program" occupies addresses 0x0000 to 0x5FFF, so there are no other special requirements for the writing of the "user program" except that the size cannot exceed 24k.

1.3 Locating the Storage Location of BootLoader
It is very easy to locate the program when developing it using Keil software. Here is the easiest way to do it. Just enter the address range of the program in the BL51 Locate panel of the BootLoader project's settings window, as shown in the figure below.

2 BootLoader program workflow description
2.1 Key process description
Power-on reset: After reset, the microcontroller first executes the jump instruction at address 0x0000 to jump to the entry address of the BootLoader program and performs operations such as turning off the watchdog, setting the crystal frequency, and setting the UART serial port baud rate. In order to make the program update faster, the system clock frequency in this application sets a larger communication baud rate of 115200bps.
Upgrade handshake: According to the pre-defined handshake rules, some data is exchanged with the host computer (usually a computer) through URAT. After receiving the correct reply, the handshake is considered successful, and an indication of readiness to receive data is sent through the serial port. If it is unsuccessful, the user program jump operation is performed.
User program judgment: If it exists, jump to the user program entry address immediately (this is also the most common normal startup process).
Jump to the user program entry: The BootLoader task is completed, and the control of the MCU is handed over to the user program until the next reset to re-enter the BootLoader.
Receiving data: No interrupt function is used in BootLoader, which reduces the remapping operation of the interrupt vector and increases the stability of the program. The query method is used here to realize data reception.
Instruction type analysis: The data frame sent by the host computer has multiple functions. The protocol is specified by the developer of the BootLoader. The main instruction types include: writing data, reading data and uploading, and ending the data transmission process.
Send "xxxx" prompt: Send some operation result information to the host computer through UART, such as "operation successful", "operation failed", etc., to inform the host computer how to proceed to the next step.
2.2 BootLoader Flowchart

3. Program processing of key operations
Data reception: Do not use interrupt functions in the BootLoader program, as this will cause the same interrupt processing function of the "user program" to become invalid. Therefore, the query method is used here to implement UART serial port data reception.
Saving the BootLoader program entry address: After the microcontroller is reset, it always starts execution from the 0x0000 address of the Flash storage area. Here, 3 bytes are used to save a jump instruction. The content of address 0x0000 is 0x02, which is the jump instruction of the machine code. The following two bytes save the address value to jump to. In order to ensure that it can jump to the BootLoader area correctly, it is necessary to save the jump address value before erasing this page, and rewrite these 3 bytes after the erasure is completed. The implementation code is as follows:
BootAddr[0]=FlASH_ByteRead(0x0001);
BootAddr[1]=FLASH_ByteRead(0x0002);
FLASH_PageErase(0X0000); // Erase page 0
FLASH_ByteWrite(0x0000,0x02);//jump instruction 0x02
FLASH_ByteWfite (0x0001, BootAddr [0]); //Write the start address of the bootloader
FLASH_ByteWrite(0x0002, BootAddr[1]);
Saving of the "user program" entry address: The "user program" entry address is marked in the program file and saved in the first 3 address bytes of the program. It is displayed in the generated program Hex file as:
:03000000021ECC11
:0C1ECC00787FE4F6D8FD7581700216A046
The content in the first line indicates that the content in the address 0x0000 and the next two bytes is 0x02ECCC, which means it will jump to the Flash address 0x1ECC to execute the first instruction of the "user program". Here we need to save this address so that the BootLoader program can jump here to run the "user program" after execution. That is, save the 3 bytes originally pointing to the address 0x0000~0x0002 in the "user program" file to a page specified by the BootLoader and save it separately. In this application, it is saved to the first 3 bytes of the 0x7A00 page. The implementation code is as follows:
#define APP_ADDR_PAGE 0x7C00L ∥Entry address of user program...
startAddr=RecData[2]*256+RecData[3];
if((startAddr+i==0)‖(startAddr+i==1)‖(startAddr+i==2))
FLASH_ByteWrite(APP_ADDR_PAGE+i, RecData[5+i]);
startAddr is the address where the data specified in the data frame sent by the host computer should be saved
Protection of BootLoader program area: When updating the "user program", it is necessary to prevent the data sent by the host computer from containing the address segment that is repeated with the address of the BootLoader program storage area. If the BootLoader area is overwritten, the boot program will not be executed correctly after the next reset. The protection of the boot area is achieved through the following program segments:
if(startAddr>=0x6000)//Conflict with BootLoader
SendString("Code overflow! ");
Absolute address jump: When the upgrade is completed or the host computer does not respond to the upgrade handshake after reset, the program jumps to the entry address of the "user program", which is saved at 0x7C00 in Flash.
4. Host computer software development
In order to cooperate with the function realization of BootLoader in the single-chip microcomputer, it is necessary to write a corresponding download program on the computer side to complete the firmware upgrade together. According to the communication protocol of BootLoader, the host computer service program is developed using Delphi. The program is mainly aimed at serial port operations, completing the handshake protocol, user program file reading and packaging in a fixed format, downloading and progress monitoring, etc. The running interface of the program is shown in Figure 4.

5 Conclusion
BootLoader is a basic function that a complete product should have, and it provides a good solution for product program upgrades based on microcontrollers.
The practical application and reliability of the method described in this paper have been well verified through the actual product use of the C8051F410 microcontroller core. At the same time, this method is also applicable to other microcontrollers with similar structures.
When the program has important confidentiality requirements, you can consider encrypting the original Hex file and decrypting it according to the encryption rules during the download process to make the program upgrade more secure and universal.
In order to make the program function more complete, the old version of the "user program" in the microcontroller should be downloaded and saved before updating the program, and then updated. When the newly upgraded program cannot be used, it can be restored to the old version.
Previous article:Design of safety warning system based on infrared radiation technology
Next article:Anti-interference design of pedometer based on MMA8452Q sensor
Recommended ReadingLatest update time:2024-11-16 23:58
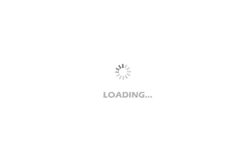
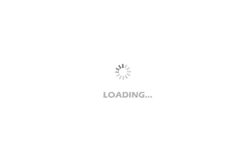
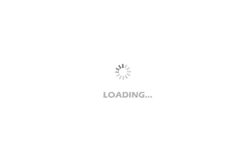
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [NXP Rapid IoT Review] +5. Write a simple project
- What is the function of R1 in the circuit in the figure?
- C2000 MCU, Vienna Rectifier-Based Three-Phase Power Factor Correction Reference Design
- STC89C52RC MCU realizes serial port printing function
- Share a DIY infrared chip with code library for home air conditioner and TV set-top box
- ADI Flow Cytometer Solutions
- A cross-era product, the Red Flag brand - do you have one or two memories that you can recall?
- Simple comparison of Cortex series M0-4
- Application of Aigtek power amplifier in multi-source excitation research of mechanical plate structure damage
- Ultra-low power Bluetooth controlled, cost-effective, dimmable smart lighting solution