/*--------------------
〖Description〗The SPI bus driver source program defaults to a 11.0592Mhz crystal oscillator.
〖File〗93CXX.C ﹫2003/5/12
---------------------*/
/*General 93c06-93c86 series instructions
93c06=93c4693c56=93c6693c76=93c86*/
//dipx can be defined by yourself
#i nclude
#i nclude "intrins.h"
/*-----------------------------------------------------
SPI93cXX series timing function call (normal package)
Calling method: self-defined﹫2001/05/12
Function description: private function, encapsulate each interface definition
-----------------------------------------------------*/
/*#define di_93 dip3
#define sk_93 dip2
#define cs_93 dip1
#define do_93 dip4
#define gnd_93 dip5
#define org_93 dip6*/
sbit cs_93=0xA3;
sbit sk_93=0xA4;
sbit
di_93=0xA5;
sbit do_93=0xA6; sbit org_93=0xA7;
/*
sbit spi_cs = 0xA3;
sbit spi_sk = 0xA4;
sbit spi_di = 0xA5;
sbit spi_do = 0xA6;
sbit spi _org = 0xA7;*/
/*-----------------------------------------------------
SPI93cXX series timing function call (normal package)
Calling method: void high46(void) --- High 8-bit function call
void low46(void) --- Low 8-bit function call ﹫2001/05/12
Function description: Private function, SPI dedicated 93c46 normal package driver program
-----------------------------------------------------*/
void high46(void)
{
di_93=1;
sk_93=1;
_nop_();
sk_93=0;
_nop_();
}
void low46(void)
{
di_93=0;
sk_93=1;
_nop_();
sk_93=0;
_nop_();
}
void wd46(unsigned char dd)
{
unsigned char i;
for (i=0;i<8;i++)
{
if (dd>=0x80)
high46();
else
low46();
dd=dd<<1;
}
}
unsigned char rd46(void)
{
unsigned char i,dd;
do_93=1;
for (i=0;i<8;i++)
{
dd<<=1;
sk_93=1;
_nop_();
sk_93=0;
_nop_();
if (do_93)
dd|=1;
}
return(dd);
}
/*-----------------------------------------------------
SPI93cXX series timing function call (special package)
Calling method: self-definition﹫2001/05/12
Function description: private function, special package interface definition
-----------------------------------------------------
#define di_93a dip5
#define sk_93a dip4
#define cs_93a dip3
#define do_93a dip6
#define gnd_93a dip7
#define vcc_93a out_vcc(2)
sbit cs_93a=P1^0;
sbit sk_93a=P1^1;
sbit di_93a=P1^2;
sbit do_93a=P1^3;
/*-----------------------------------------------------
SPI93cXX series timing function call (special package)
Calling method: void high46a(void) ---high 8-bit function call
void low46a(void) ---Low 8-bit function call﹫2001/05/12Function
description: Private function, SPI dedicated 93c46 special package driver
----------------------------------------------------------
void high46a(void)
{
di_93a=1;
sk_93a=1;_nop_();
sk_93a=0;_nop_();
}
void low46a(void)
{
di_93a=0;
sk_93a=1;_nop_();
sk_93a=0;
_nop_();
}
void wd46a(unsigned char dd)
{
unsigned char i;
for (i=0;i<8;i++)
{
if (dd>=0x80) high46a();
else low46a();
dd=dd<<1;
}
}
unsigned char rd46a(void)
{
unsigned char i,dd;
do_93a=1;
for (i=0;i<8;i++)
{
dd<<=1;
sk_93a=1;_nop_();
sk_93a=0;_nop_();
if (do_93a) dd|=1;
}
return(dd);
}
/*-----------------------------------------------------
SPI93c46 series function call (example)
Calling method: bit write93c56_word(unsigned int address,unsigned int dat) ﹫2001/05/12
Function description: Private function, SPI dedicated
-----------------------------------------------------*/
void ewen46(void)
{
_nop_();
cs_93=1;
high46();
wd46(0x30);
cs_93=0;
}[page]
unsigned char eral_46()
{
data unsigned char temp1;
data unsigned int temp2;
cs_93=0;
sk_93=0;
//spi_org=1;
cs_93=1;
ewen46();
_nop_();
cs_93=1;
_nop_();
high46();
wd46(0x20);
wd46(0x20);
cs_93=0;
_nop_();
cs_93=1;
temp1=1;
temp2=50000;
while(!do_93)
{
temp2=temp2-1;
if(temp2= =0)
{
temp1=0;
break;
}
}
cs_93=0;
return temp1;
}
unsigned int read93c46_word(unsigned char address)
{
unsigned int dat;
unsigned char dat0,dat1;
//gnd_93a=0;
//gnd_93=0;
cs_93=sk_93=0;
//org_93=1;
cs_93=1;_nop_() ;
//address=address<<1;
//address=address>>1;
address=address|0x80;
address=address|0x80;
high46();
wd46(address);
dat1=rd46();
dat0=rd46( );
cs_93=0;
dat=dat1*256+dat0;
return(dat);
}
bit write93c46_word(unsigned char address,unsigned int dat)
{
unsigned char e,time,temp=address;
e=0;
while (e<3)
{
//gnd_93a=0;
//gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
ewen46();
_nop_();
cs_93=1;
_nop_ ();
high46();
address<<=1;//??
address|=0x80;
address>>=1;//??
address|=0x40;
wd46(address);
wd46(dat/256);
wd46 (dat%256);
cs_93=0;
_nop_();
cs_93=1;
time=0;
do_93=1;
while (1)
{
if (do_93==1)
break;
if (time>20)
break;
time++;
}
cs_93=0;
if (read93c46_word(temp)==dat)
{
return(0);
}
e++;
}
return(1);
}
/*----------------------- ------------------------------
SPI93c57 series function call (example)
Calling method: bit write93c57_word(unsigned int address,unsigned int dat ) ﹫2001/05/12
Function description: Private function, SPI only
----------------------------------- ------------------
void ewen57(void)
{
_nop_();
cs_93=1;
dip7=0;
high46();
low46();
wd46(0x60);
cs_93 =0;
}
unsigned int read93c57_word(unsigned int address)
{
unsigned int dat;
unsigned char dat0,dat1;
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
address=address>>1;
high46();
high46();
wd46(address);
dat1=rd46();
dat0=rd46();
cs_93=0;
dat=dat1*256+dat0;
return(dat);
}
bit write93c57_word(unsigned int address,unsigned int dat)
{
unsigned char e;
unsigned int temp=address;
e=0;
while (e<3)
{
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
ewen57();
cs_93=1;
_nop_( );
high46();
low46();
address>>=1;
address|=0x80;
wd46(address);
wd46(dat/256);
wd46(dat%256);
cs_93=0;
_nop_();
cs_93=1;
time=0;
do_93=1;
while (1)
{
if (do_93==1) break;
if (time>20) break;
}
cs_93=0;
if (read93c57_word(temp)= =dat)
{
return(0);
}
e++;
}
return(1);
}
/*----------------------------- ------------------------
SPI93c56 series function call (example)
Calling method: bit write93c56_word(unsigned int address,unsigned int dat) ﹫2001/05/ 12Function
description: Private function, SPI only
----------------------------------------- ------------
void ewen56(void)
{
_nop_();
cs_93=1;
high46();
low46();
low46();
wd46(0xc0);
cs_93=0;
}
unsigned int read93c56_word(unsigned char address)
{
unsigned int dat;
unsigned char dat0,dat1;
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
address=address>>1;
high46();
high46();
low46();
wd46(address);
dat1=rd46();
dat0=rd46();
cs_93=0;
dat=dat1*256+dat0;
return(dat);
}
bit write93c56_word(unsigned char address,unsigned int dat)
{
unsigned char e;
unsigned int temp=address;
e=0;
while (e<3)
{
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
93c56_word(temp
)
==dat) {
return(
0)
;
} e++
;
} return
(
1)
; }
[page] /*-----------------------------------------------------
SPI93c76
and
SPI93c86
series
function
call
(
example
)
Calling
method
:
bit
write93c76_word
(
unsigned
int
address
,
unsigned
int
dat) ﹫2001/05/12Function
description: Private function, SPI dedicated
-----------------------------------------------------
void ewen76(void)
{
_nop_();
cs_93=1;
dip7=1;
high46();
low46();
low46();
high46();
high46();
wd46(0xff);
cs_93=0;
}
unsigned int read93c76_word(unsigned int address)
{
unsigned char dat0,dat1;
gnd_93=0;
cs_93=sk_93=0;
org_93=1;
cs_93=1;
address>>=1;
high46();
high46();
low46();
if((address&0x200)==0x200) high46();
else low46();
if ((address&0x100)==0x100) high46();
else low46();
wd46(address);
dat1=rd46();
dat0=rd46();
cs_93=0;
return(dat1*256|dat0);
}
bit write93c76_word(unsigned int address,unsigned int dat)
{
unsigned char e;
unsigned int temp=address;
e=0;
address>>=1;
while (e<3)
{
gnd_93=0;
cs_93=sk_93=0
; org_93=1;
cs_93=1;
ewen76();
_nop_();
cs_93=1;
high46();
low46();
high46();
if((address&0x200)==0x200) ;
else low46();
if ((address&0x100)==0x100) high46();
else low46();
wd46(address);
wd46(dat/256);
wd46(dat%256);
cs_93=0;_nop_();cs_93=1;
time=0;do_93=1;
while (1)
{
if (do_93==1) break;
if (time>10) break;
}
cs_93=0;
e++;
}
return(1);
}
/*-----------------------------------------------------
Main function call (example)
Calling method: main() ﹫2001/05/12
Function description: Private function, SPI dedicated
-----------------------------------------------------*/
main()
{
bit b;
unsigned int i;
unsigned int j[32];
eral_46();
for(i=0;i<32;i++)
j[i]=read93c46_word(i);
for(i=0;i<32;i++)
write93c46_word(i,0x0910);
i=0;
b=write93c46_word(i
,
0x1213);
j[i]=read93c46_word(
i);
i=1
;
b=write93c46_word(i,0x1122);
j[i]=read93c46_word(i);
i=2
; 344);
j[i]=read93c46_word(i);
i=3;
b=write93c46_word(i,0x1526);
j
[i]=read93c46_word(i);
i=
4
;
Previous article:1-wire bus assembler
Next article:HD44780 read and write C51 program
Recommended ReadingLatest update time:2024-11-16 14:35
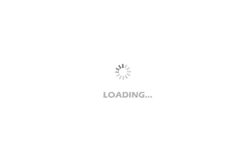
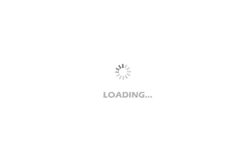
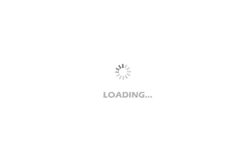
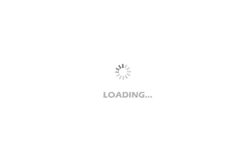
- Popular Resources
- Popular amplifiers
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Digilent Vivado library
-
Teach you to learn 51 single chip microcomputer-C language version (Second Edition) (Song Xuefeng)
-
Raspberry Pi Development in Action (2nd Edition) ([UK] Simon Monk)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University ---- Common Errors and Solutions in DC/DC Converter Design
- 【I-Prober 520】Unboxing and simple review
- EEWORLD University Hall----Live Replay: Maxim supports integrated digital IO technology for industrial systems
- Want to buy second-hand AM335x Starter Kit development board
- Emulating I2C communication using GPIO on C2000
- 【TouchGFX Design】Make a Rubik's Cube
- Two circuits for modeling the behavior of a two-input AND gate
- Circuit protection devices protect mobile devices from ESD
- Let's discuss some gadgets commonly used by RF engineers
- MSP430 interrupt vector table