Program description: This is a complete program design example using 51 single-chip microcomputer to drive DS1302 time module + DS18B20 temperature sensor module + 12864 LCD display. There are four keyboards KEY0 to KEY3. Key0 is used to modify the time. First, it is from second to minute to hour to year to month to day to week. Key1 is to add 1 and key2 is to subtract 1. When in the time modification state, press key3 again to exit and modify the time and date. In the normal state, press key3 and then key2 to turn on the LCD light. Pressing key3 alone will turn off the light.
File 1: DS1302 driver.c
#include
//ds1302
sbit sclk=P0^3;
sbit io=P0^4;
sbit rst=P0^5;
sbit acc0=ACC^0;
sbit acc1=ACC^1;
sbit acc2=ACC^2;
sbit acc3=ACC^ 3;
sbit acc4=ACC^4;
sbit acc5=ACC^5;
sbit acc6=ACC^6;
sbit acc7=ACC^7;
delay()
{
unsigned char i;
i=10;
i=
10;
i=10;
i=10;
i=10;
i=10;}
w_1302(unsigned char dat,unsigned char shu){//write an eight-bit number to DS1302 //rising efficiency ;;;select the write address and then write the data
ACC=dat;//address
sclk=0;
rst=1;//
io=acc0;///
sclk=0;
delay();
sclk=1;
io=acc1;
sclk=0;
delay();
sclk= 1;
io=acc2;
sclk=0;
delay();
sclk
=1
; io=acc3;
sclk
=0;
delay();
sclk=1;
io=acc4;
sclk=0;
delay();
sclk=1;
io=acc5;
sclk=0;
delay();
sclk
=1;
io=acc6;
sclk
=0;
delay();
sclk
=1;
io=acc7;
sclk=0;
delay();
sclk=1;// /
//sclk=0;
ACC=shu; //data
io=acc0;///
sclk=0;
delay();
sclk
=1
; io=acc1;
sclk=0;
delay();
sclk=1;
io= acc2;
sclk=0;
delay();
sclk=1;
io=acc3;
sclk
=0;
delay()
; sclk=1;
io=acc4;
sclk
=0
; delay();
sclk=1;
io=acc5;
sclk=0;
delay();
sclk=1;
io=acc6;
sclk
=0;
delay()
; sclk=1;
io=acc7;
sclk=0;
delay();
sclk=1;///
rst=0 ;
sclk=0;
} ///Complete writing
unsigned char r_1302(unsigned char ADD){
sclk=0;
rst=1;
ACC=ADD; //Address
io=acc0;///
sclk=0;
delay();
sclk=1;
io=acc1;
sclk
=0;
delay();
sclk=1;
io=acc2;
sclk=0;
delay();
sclk=1;
io=acc3;
sclk=0;
delay();
sclk=1;
io=acc4;
sclk
=0;
delay();
sclk=1
; io=acc5;
sclk=0;
delay();
sclk= 1;
io=acc6;
sclk=0;
delay();
sclk
=1;
io=acc7;
sclk
=0;/////
delay();
sclk=1;
delay();
sclk=0;
acc0=io ;
delay();
sclk=1;
delay
();
sclk=0
;
acc1
=io;
delay();
sclk=1; delay(); sclk=0;
acc2=io;
delay();
sclk=1;
delay();
sclk=0;
acc3=io;
delay()
; sclk
=1;
delay();
sclk=0;
acc4=io;
delay();
sclk=1;
delay();
sclk= 0;
acc5=io;
delay();
sclk=1;
delay
();
sclk
=0;
acc6=io
; delay
();
sclk=1;
delay();
sclk=0;
acc7=io;
delay();
rst=0;
sclk=0;
return(ACC);
}
ds1302_c()
{
w_1302(0x80,0x80);
w_1302(0x8e,0x00);
w_1302(0x80,0x50); //;Secondsw_1302
(0x82,0x06); //Minutes=0
w_1302(0x84,0x01); //Hours=0
w_1302(0x86,0x22); //
w_1302(0x88,0x11);//
w_1302(0x8a,0x04);//
w_1302(0x8b,0x07);//
w_1302(0x8e,0x80);
w_1302(0x90,0xa6);
w_1302(0x80,0x00);
}
main(){
rst=0;
ds1302_c();
for(;;){
P2=r_1302(0x81);
}
}
---------------------------------------------------------------------------------------------------------------
File 2: Full version of the light key.c
#include
sbit di = P2^7; ////////Define pin
sbit rw = P2^6;
sbit e = P2^5;
sbit cs1 = P2^4;
sbit cs2 = P2 ^1;
sbit lcd_d = P2^0;
sbit key0 = P2^3;
sbit key1 = P2^2;
sbit key2 = P1^0;
sbit key3 = P1^1;
sbit P07 = P0^7;
sbit P06 = P0^ 6;
sbit P05 = P0^5;
sbit P04 = P0^4;
sbit P03 = P0^3;
//ds1302
sbit sclk=P1^4;
sbit io=P1^3;
sbit rst=P1^2;
sbit acc0= ACC^0;
sbit acc1=ACC^1;
sbit acc2=ACC^2;
sbit acc3=ACC^3;
sbit acc4=ACC^4;
sbit acc5=ACC^5;
sbit acc6=ACC^6;
sbit acc7=ACC^7;
typedef unsigned char byte;
typedef unsigned int word;
sbit KEY=P3^7; //Define port ds1820 according to actual situation
unsigned char key_time=0; //Global variable
//////////////////////////////////////Temperature
//ds18b20 digital temperature sensor module program
void delay1(word useconds)
{
for(;useconds>0;useconds--);
}
//Reset
byte ow_reset(void)
{
byte presence;
KEY = 0; //pull DQ line low
delay1(29); // leave it low for 480us
KEY = 1; // allow line to return high
delay1(3); // wait for presence
presence = KEY; // get presence signal
delay1(25); // wait for end of timeslot
return(presence); // presence signal returned
} // 0=presence, 1 = no part[page]
//Read a byte from the 1-wire bus
read_byte(void)
{
byte i;
byte value = 0;
for (i=8;i>0;i--)
{
value>>=1;
KEY = 0; // pull DQ low to start timeslot
KEY = 1; // then return high
delay1(1); //for (i=0; i<3; i++);
if(KEY)value|=0x80;
delay1( 6); // wait for rest of timeslot
}
return(value);
}
//Write a byte to the 1-WIRE bus
void write_byte(char val)
{
byte i;
for (i=8; i>0; i--) // writes byte, one bit at a time
{
KEY = 0 ; // pull DQ low to start timeslot
KEY = val&0x01;
delay1(5); // hold value for remainder of timeslot
KEY = 1;
val=val/2;
}
delay1(5);
}
//Read temperature
float wendu(void)
{
float tem;
union{
byte c[2];
int x;
}temp;
ow_reset();
write_byte(0xCC); // Skip ROM
write_byte(0xBE); // Read Scratch Pad
temp.c[1]=read_byte(); //lsd
temp.c[0]=read_byte(); // msd
ow_reset();
write_byte(0xCC); //Skip ROM
write_byte(0x44); // Start Conversion
tem=0.0;
if(temp.x & 0x01) tem+=0.0625;temp.x>>=1;
if(temp.x & 0x01) tem+=0.125;temp.x>>=1;
if(temp.x & 0x01) tem+=0.25;temp.x>>=1;
if(temp.x & 0x01) tem+=0.5;temp.x>>=1;
tem+=temp.x;
return term;
}
void cs1_lcd(){ ////////Select
the left
bit a=1;
cs1=a;
cs2=!a;
}
void cs2_lcd(){ ////////Select the right
bit a=1;
cs1=!a;
cs2=a;
}
void busy_lcd(){//////Judge busy
bit a=1;
di=!a;
rw=a;
e=a;
while(P07==a);
}
w_lcd(unsigned b){ ///Write a to 12864
bit a=1;
busy_lcd();
rw =!a;
di =a;
P0=b;
e=a;
e=!a;
}
void dis_lcd(){//Open the display and select the first line to display
bit a=1;
busy_lcd();
rw=!a;
di=!a;
P0=0x3f;
e=a;
e=!a;
P0=0xc0;
e=a;
e=!a;
}
y_lcd(unsigned a){///The y-axis is determined by A,
bit b=1;
busy_lcd();
rw=!b;
di=!b;
P0=a;
P07=b;
P06=!b;
P05=b;
P04=b;
P03=b;
e=b;
e=!b;
}
x_lcd(unsigned a){//The x-axis is determined by a,
bit b=1;
busy_lcd();
rw=!b;
di=!b;
P0=a;
P07=!b;
P06=b;
e=b;
e=!b;
}
void clr_lcd(){///Clear screen
bit a=0;
unsigned x,y,date,i;
cs1_lcd();
date=0x00;
for(i=0;i<2;i++){
for(y=0 ;y<8;y++)
for(x=0;x<64;x++){
x_lcd(x);
y_lcd(y);
w_lcd(date);
}
cs2_lcd();}
}
unsigned char code shu[18][16]={///0 to 9 numbers
{0x00,0xE0,0x10,0x08,0x08,0x10,0xE0,0x00,0x00,0x0F,0x10,0x20,0x20,0x10,0x0F,0x00},//0
{0x00,0x10,0x10,0xF8,0x00,0x00,0x00,0x00,0x00,0x20,0x20,0x3F,0x20,0x20,0x00,0x00},//1
{0x00,0x70,0x08,0x08,0x08,0x88,0x70,0x00,0x00,0x30,0x28,0x24,0x22,0x21,0x30,0x00},//2
{0x00,0x30,0x08,0x88,0x88,0x48,0x30,0x 00,0x00,0x18,0x20,0x20,0x20,0x11,0x0E,0x00},//3
{0x00,0x00,0xC0,0x20,0x10,0xF8,0x00,0x00,0x00,0x07,0x04,0x24,0x24,0x3F,0x24,0x00},///4
{0x00,0xF8,0x08,0x88,0x88,0x08,0x08,0 x00,0x00,0x19,0x21,0x20,0x20,0x11,0x0E,0x00},//5
{0x00,0xE0,0x10,0x88,0x88,0x18,0x00,0x00,0x00,0x0F,0x11,0x20,0x20,0x11,0x0E,0x00},//6
{0x00,0x38,0x08,0x08,0xC8,0x38,0x08,0x 00,0x00,0x00,0x00,0x3F,0x00,0x00,0x00,0x00},//7
{0x00,0x70,0x88,0x08,0x08,0x88,0x70,0x00,0x00,0x1C,0x22,0x21,0x21,0x22,0x1C,0x00},//8
{0x00,0xE0,0x10,0x08,0x08,0x10,0xE0,0x 00,0x00,0x00,0x31,0x22,0x22,0x11,0x0F,0x00},//9
{0x00,0x00,0x00,0xC0,0xC0,0x00,0x00,0x00,0x00,0x00,0x00,0x30,0x30,0x00,0x00,0x00},//:
{0x00,0x00,0x00,0x00,0x80,0x60,0x18,0x 04,0x00,0x60,0x18,0x06,0x01,0x00,0x00,0x00},// /
};
unsigned char code shu1[9][32]={///
{0x02,0x42,0x42,0xFE,0x42,0x42,0xFE,0x02,0x02,0xFA,0x02,0x02,0xFE,0x00,0x00,0x00,0x08,0x08,0x08,0x07,0x84,0x44,0x23,0x18,0x06,0x01,0x3E,0x40,0x43,0x40,0x78,0x00}, // Current 12
{0x00,0x04,0x04,0xC4,0x64,0x9C,0x87,0x84,0x84,0xE4,0x84,0x84,0x84,0x84,0x04,0x00,0x04,0x02,0x01,0x7F,0x00,0x20,0x20,0x20,0x20,0x3F,0x20,0x20,0x20,0x20,0x20,0x00,0x00}, // at 13
{0x00,0xFC,0x44,0x44,0x44,0xFC,0x10,0x90,0x10,0x10,0x10,0xFF,0x10,0x10,0x10,0x00,0x00,0x07,0x04,0x04,0x04,0x07,0x00,0x00,0x03,0x40,0x80,0x7F,0x00,0x00,0x00,0x00,0x00}, //Time 14
{0x00,0xF8,0x01,0x06,0x00,0xF0,0x92,0x92,0x92,0x92,0xF2,0x02,0x02,0xFE,0x00,0x00,0x00,0xFF,0x00,0x00,0x00,0x07,0x04,0x04,0x04,0x04,0x07,0x40,0x80,0x7F,0x00,0x00}, // 15
{0x00,0x00,0x00,0xBE,0x2A,0x2A,0x2A,0xEA,0x2A,0x2A,0x2A,0x2A,0x3E,0x00,0x00,0x00,0x00,0x48,0x46,0x41,0x49,0x49,0x49,0x7F,0x49 ,0x49,0x49,0x49,0x49,0x41,0x40,0x00},//star 16
{0x00,0x04,0xFF,0x54,0x54,0x54,0xFF,0x04,0x00,0xFE,0x22,0x22,0x22,0xFE,0x00,0x00,0x42,0x22,0x1B,0x02,0x02,0x0A,0x33,0x62,0x18,0 x07,0x02,0x22,0x42,0x3F,0x00,0x00},//Issue 17
{0x10,0x21,0x86,0x70,0x00,0x7E,0x4A,0x4A,0x4A,0x4A,0x4A,0x7E,0x00,0x00,0x00,0x00,0x02,0xFE,0x01,0x40,0x7F,0x41,0x41,0x7F,0x 41,0x41,0x7F,0x41,0x41,0x7F,0x40,0x00},//Wen 18
{0x00,0x00,0xFC,0x04,0x24,0x24,0xFC,0xA5,0xA6,0xA4,0xFC,0x24,0x24,0x24,0x04,0x00,0x80,0x60,0x1F,0x80,0x80,0x42,0x46,0x2A,0x12 ,0x12,0x2A,0x26,0x42,0xC0,0x40,0x00},//degree 19
};
unsigned char time1[7]={0x30,0x00,0x16,8,7,2,8};//Seconds, minutes, hours, days, months, weeks, and years respectively. The subroutine is from http://www.51hei.com hkcd moderator and has passed the test.
delay()
{
unsigned char i;
i=10;
i=10;
i=10;
i=10;
i=10;
i=10;
i=10;}
w_1302(unsigned char dat,unsigned char shu){//Write an eight-bit number to DS1302 //Rising efficiency ;;;Select the write address and then write the data
ACC=dat;//address
sclk=0;
rst=1;//
io=acc0;///
sclk=0;
delay();
sclk=1;
io=acc1;
sclk=0;
delay();
sclk= 1;
io=acc2;
sclk=0;
delay();
sclk
=1
; io=acc3;
sclk
=0;
delay();
sclk=1;
io=acc4;
sclk=0;
delay();
sclk=1;
io=acc5;
sclk=0;
delay();
sclk
=1;
io=acc6;
sclk
=0;
delay();
sclk
=1;
io=acc7;
sclk=0;
delay();
sclk=1;// /
//sclk=0;
ACC=shu; //data
io=acc0;///
sclk=0;
delay();
sclk
=1
; io=acc1;
sclk=0;
delay();
sclk=1;
io= acc2;
sclk=0;
delay();
sclk=1;
io=acc3;
sclk
=0;
delay()
; sclk=1;
io=acc4;
sclk
=0
; delay();
sclk=1;
io=acc5;
sclk=0;
delay();
sclk=1;
io=acc6;
sclk
=0;
delay()
; sclk=1;
io=acc7;
sclk=0;
delay();
sclk=1;///
rst=0 ;
sclk=0;
} ///Complete writing
unsigned char r_1302(unsigned char ADD){
sclk=0;
rst=1;
ACC=ADD; //Address
io=acc0;///
sclk=0;
delay();
sclk=1;
io=acc1;
sclk
=0;
delay();
sclk=1;
io=acc2;
sclk=0;
delay();
sclk=1;
io=acc3;
sclk=0;
delay();
sclk=1;
io=acc4;
sclk
=0;
delay();
sclk=1
; io=acc5;
sclk=0;
delay();
sclk= 1;
io=acc6;
sclk=0;
delay();
sclk=1;
io=acc7;
sclk=0;/////
delay();
sclk=1;
delay();
sclk=0;
acc0=io;
delay
();
sclk
=
1; delay(); sclk=0;
acc1=io;
delay();
sclk=1;
delay();
sclk=0;
acc2= io;
delay();
sclk=1;
delay();
sclk
=0;
acc3=io;
delay
()
; sclk=1;
delay();
sclk=0;
acc4=io;
delay();
sclk=1;
delay();
sclk=0;
acc5=io;
delay
();
sclk
=
1; delay(); sclk=0;
acc6=io;
delay();
sclk=1;
delay();
sclk=0;
acc7= io;
delay();
rst=0;
sclk=0;
return(ACC);
}
ds1302_c()
{
w_1302(0x80,0x80);
w_1302(0x8e,0x00);
w_1302(0x80,time1[0]); //;
Secondsw_1302(0x82,time1[1]); //Minute=0
w_1302(0x84,time1[2]); //Hour=0
w_1302(0x86,
time1[3]);
//
Dayw_1302(0x88,time1[4]);//Monthw_1302(0x8a,time1[5]);//Weekw_1302
(0x8c,time1[6]);//Yearw_1302
(0x8e,0x80);
w_1302(0x90,0xb8);
w_1302(0x80,0x00);
}
//x_lcd(unsigned a){//X axis is determined by a //y_lcd(unsigned a){///Y axis is determined by A
//void dis_lcd(){//Open the display and select the first line to display//w_lcd(unsigned b){ ///Write a to 12864
//void busy_lcd(){//////Judge busy //void cs1_lcd(){ ////////Select the left side
//void cs2_lcd(){ ////////Select the right
sided12864_lcd(unsigned char x,y,k){
unsigned char ix,iy,i;
dis_lcd();//Open the displayif
(x>63){
x=x-64;
cs2_lcd();
}
else cs1_lcd();
i=0;
if(k<12){
for(iy=0;iy<2;iy++)
for(ix
=
0;ix<8;ix++){
y_lcd
(
iy+y)
;
(
shu1[k][i]);
i
++
;
}
}
}
[
page
]
time_lcd(){
unsigned char y,k,time,add,i,we1,we2;
add=128;///////must be less than 64-8 and greater than 64
d12864_lcd(0,0,12);//: North
d12864_lcd(16,0,13);//: Beijing
d12864_lcd(31,0,14);//: Time
d12864_lcd(47,0,15);//: Time
k=0x81;
y=0; //On which line to display
for(i=0;i<3;i++){
time=r_1302(k);
time1[i]=time;
add=add-8;;
time=time&0x0f;
d12864_lcd(add,y,time);///Seconds
add=add-8;
time=r_1302(k);
time&=0xf0;
time>>=4;
d12864_lcd(add,y,time);//second ten
digitadd=add-8;;
k+=2;
}
d12864_lcd(40+64,y,10);//:
d12864_lcd(16+64,y,10);//: display two points
//
time1[5]=r_1302(0x8b);
d12864_lcd(64,4,16);//:
stard12864_lcd(64+16,4,17);//:
dater_1302(0x8b);//read day of
the weekd12864_lcd(64+32,4,r_1302(0x8b));//: read day of the week and display
////
y=2;//Display in the fourth lineadd
=56+16;
time=r_1302(0x87);
time1[3]=time;
d12864_lcd(add,y,time&0x0f);//: Display daytime
=r_1302(0x87);
time&=0xf0;
time>>=4;
add-=8;
d12864_lcd(add,y,time); //Display the tens digit of the dayadd-
=8;
add-=8;
time1[4]=time=r_1302(0x89);
d12864_lcd(add,y,time&0x0f);//: Display monthtime
=r_1302(0x89);
time&=0xf0;
time>>=4;
add-=8;
d12864_lcd(add,y,time); //Display the tens digit of the monthadd-
=8;
add-=8;
time1[6]=time=r_1302(0x8d);
d12864_lcd(add,y,time&0x0f);//: display yeartime
=r_1302(0x8d);
time&=0xf0;
time>>=4;
add-=8;
d12864_lcd(add,y,time); //display the tenth digit of the year
//////Read
d12864_lcd(40+16,y,11);//:
d12864_lcd(16+16,y,11);//: display two digits
add-=8;
d12864_lcd(add,y,0);//: 0
add-=8;
d12864_lcd(add,y,2);//: 2
d12864_lcd(0,4,18);//:
d12864_lcd(16,4,19);//: temperature
we1=wendu();
we2=we1/10;
d12864_lcd(32,4,we2);//:
we2=we1-we2*10;
d12864_lcd(40,4,we2);//: display temperature value
cs1_lcd
();
y_lcd(4);
x_lcd(50);
w_lcd(0x18);
x_lcd(51);
w_lcd(0x18);
}
//////////////////////////Display time completed
hei_lcd(unsigned char x,y){ //All black is used for flash mark
char iy,ix,di,i;
di=0x00;
if(x>63){
x=x-64;
cs2_lcd();
}
else cs1_lcd();
i=0;
for(iy=0;iy<2;iy++){
for(ix=0;ix<16;ix++){
y_lcd(iy+y);
x_lcd(ix+x);
w_lcd(di);
}
}
}
key_t(){ //Keyboard key1 plus 1 key2 minus 1
if(!key1){
key_time++;
delay1(20000);
while(!key1);
}
if(!key2){
key_time--;
delay1(20000);
while(!key2);
}
}
void delay11(word useconds)
{
for(;useconds>0;useconds--)
key_t();
}
time_key(){
unsigned char keyd,ktime;
unsigned int year;
word kk;
keyd=0;
kk=10000;///Flash speedif
(!key0){
for(;;){
if(!key0){
keyd=keyd+1;
delay1(500);
if(keyd>8)keyd=1;
key_time=0;//////Clear the stored time every time a setting is changed
key0=1;
while(!key0);
key0=1;
}
/////////////////////////Flash programif
(keyd==1){//=1 enter the second flash and set the second timekey_time
=(time1[0]&0x0f)+((time1[0]&0xf0)>>4)*10; //Transfer the number in the number to key_time decimal
hei_lcd(112,0);//x y
delay11(kk);////// flashing speedif
(key_time>=60)key_time=0;
d12864_lcd(112,0,key_time/10);
d12864_lcd(120,0,key_time-(key_time/10)*10);
time1[0]=(((key_time/10)<<4)&0xf0)+(key_time-(key_time/10)*10); //Convert to BCD code and store in array
delay1(10000);
}
if(keyd==2){//=1 enters the flash and sets the second time at the same time
key_time=(time1[1]&0x0f)+((time1[1]&0xf0)>>4)*10; //Convert the number in the number sister to key_time decimal
hei_lcd(88,0);//x y
delay11(kk);//////Flashing speedif
(key_time>=60)key_time=0;
d12864_lcd(88,0,key_time/10);
d12864_lcd(96,0,key_time-(key_time/10)*10);
delay1(10000);
time1[1]=(((key_time/10)<<4)&0xf0)+(key_time-(key_time/10)*10); //Convert to BCD code and store in array
}
if(keyd==3){//=1 when entering, flash and set the second time
key_time=(time1[2]&0x0f)+((time1[2]&0xf0)>>4)*10; //Convert the number in the number sister to key_time decimal
hei_lcd(64,0);//x y
delay11(kk);//////Flashing speedif
(key_time>=24)key_time=0;
d12864_lcd(64,0,key_time/10);
d12864_lcd(72,0,key_time-(key_time/10)*10);
time1[2]=(key_time/10)<<4+(key_time-key_time/10);
delay1(10000);
time1[2]=(((key_time/10)<<4)&0xf0)+(key_time-(key_time/10)*10); //Convert to BCD code and store in array
}
if(keyd==4){//=1 enter year flash and set second time
key_time=(time1[6]&0x0f)+((time1[6]&0xf0)>>4)*10; //Convert the number in number sister to key_time in decimal
hei_lcd(16,2);//x y
delay11(kk);//////Flashing speed
if(key_time>99)key_time=0;
d12864_lcd(16,2,key_time/10);
d12864_lcd(24,2,key_time-(key_time/10)*10);
time1[6]=(key_time/10)<<4+(key_time-key_time/10);
delay1(10000);
time1[6]=(((key_time/10)<<4)&0xf0)+(key_time-(key_time/10)*10); //Convert to BCD code and store in array
}
if(keyd==5){//=1 enter the month flash and set the second time
key_time=(time1[4]&0x0f)+((time1[4]&0xf0)>>4)*10; //Convert the number in the number sister to key_time in decimal
hei_lcd(40,2);//x y
delay11(kk);//////Flashing speed
if(key_time>=13)key_time=0;
d12864_lcd(40,2,key_time/10);
d12864_lcd(48,2,key_time-(key_time/10)*10);
time1[4]=(key_time/10)<<4+(key_time-key_time/10);
delay1(10000);
time1[4]=(((key_time/10)<<4)&0xf0)+(key_time-(key_time/10)*10); //Convert to BCD code and store in array
}
if(keyd==6){//=1 to enter day flash and set second time at the same time
key_time=(time1[3]&0x0f)+((time1[3]&0xf0)>>4)*10; //Convert the number in number sister to key_time decimal
year=(time1[4]&0x0f)+(((time1[4]&0xf0)>>4)*10)+2000;
switch(time1[4]){ //Calculate leap month
case 1: ktime=31;break;
case 3: ktime=31;break;
case 5: ktime=31;break;
case 7: ktime=31;break;
case 8: ktime=31;break;
case 10: ktime=31;break;
case 12: ktime=31
;break
;
case 6: ktime=30;
break; case
9: ktime=30;break
;
case 11: ktime=30;break;
case 2: if(year%4==0&&year%100!=0||year%400==0) ktime=29;
else ktime=29;break;
}
hei_lcd(64,2);//x y
delay11(kk);//////Flashing speed
if(key_time>ktime)key_time=0;
d12864_lcd(64,2,key_time/10);
d12864_lcd(72,2,key_time-(key_time/10)*10);
time1[3]=(key_time/10)<<4+(key_time-key_time/10);
delay1(10000);
time1[3]=(((key_time/10)<<4)&0xf0)+(key_time-(key_time/10)*10); //Convert to BCD code and store in array
}
if(keyd==7){//=1 enters week flash and sets second time
char iy,ix,di,i;
key_time=time1[5];
di=0x00;
cs2_lcd();
i=0;
for(iy=0;iy<2;iy++){
for(ix=0;ix<8;ix++){
y_lcd(iy+4);
x_lcd(ix+96);
w_lcd(di);
}
di=00;
}
delay11(kk);//////Flashing speedif
(key_time>7)key_time=0;
d12864_lcd(96,4,key_time);
time1[5]=key_time;
delay1(10000);
time1[5]=key_time; //Convert to BCD code and store in array
}
/////////////////////
if(!key3)break;
}
ds1302_c(); ///Change time
}
}
////////////////
lcd_key(){
if(!key3){
if(!key2)lcd_d=1;
else lcd_d=0;
}
}
main(){
ds1302_c();
clr_lcd ();
for(;;){
time_lcd();
time_key();
lcd_key();
}
}
Previous article:PIC microcontroller AD conversion assembly program
Next article:Production of 51 single chip microcomputer experimental board
Recommended ReadingLatest update time:2024-11-16 22:30
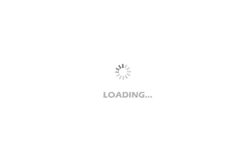
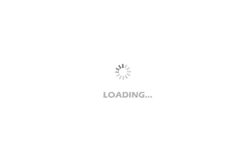
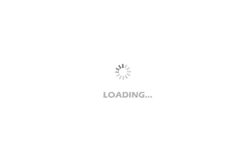
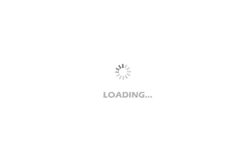
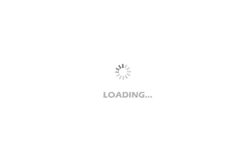
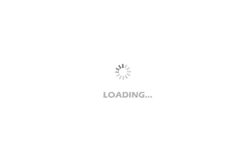
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Common Problems in RF Circuit Design
- Telink Microelectronics Matter Development Guide (I): The Past and Present of Matter
- Example of chip drain damage
- F280049C CAN_ex3 Issue
- [Factory Visit Live Replay] Arrived at the destination - TE Qingdao Factory
- Useful tutorial | How to quickly splice PCB data at unconventional angles?
- Class AB Audio Amplifier
- Max30102 heart rate blood oxygen sensor measures heart rate problem 2
- Execution process before keil main
- Let's also wink