This is the original C language program of the stepper motor with 1602 display speed and direction controlled by infrared remote control. It has been debugged successfully! Some modules were made when doing other experiments before, and now they are directly called, including 1602 LCD display technology, TC9012 infrared decoding, and then the control program of the four-phase stepper motor. I combined these things. If you are interested in this thing or want to do graduation project, you can take a look. If you don’t understand, please go to the microcontroller forum http://www.51hei.com/bbs/ for open discussion. I will give you a detailed answer.
#include
#include
#include
#define uchar unsigned char
#define uint unsigned int
static unsigned int count; //Count
unsigned int hour,minute,second,count;
sbit RS = P2^3;//Pin4
sbit RW = P2^2; //Pin5
sbit E = P2^4;//Pin6
#define Data P0 //Data port
static int step_index; //Step index number, value is 0-7
bit k=0; //Infrared decoding judgment flag, 0 is a valid signal, 1 is invalid
uchar n=0; //Used to control external interrupt
static bit turn; //Stepper motor rotation direction
static bit stop_flag; //Stepper motor stop flag
static int speedlevel; //Stepper motor speed parameter, the larger the value, the slower the speed, the minimum value is 1, the fastest speed
static int spcount; //Stepper motor speed parameter count
void delay(unsigned int endcount); //Delay function, the delay is endcount*0.5 milliseconds
void gorun(); //Stepper motor control step function
uchar data date[4]; //The date array is used to store the original code, inverse code, original code and inverse code of the address
char data Test1[]=" ";
#define IR_RE P3_2
void DelayUs(unsigned char us)//delay us
{
unsigned char uscnt;
uscnt=us>>1;/* Crystal frequency in 12MHz*/
while(--uscnt);
}
/*****************************************************/
void DelayMs(unsigned char ms)//delay Ms
{
while(--ms)
{
DelayUs(250);
DelayUs(250);
DelayUs(250);
DelayUs(250);
}
}
void WriteCommand(unsigned char c)
{
DelayMs(5);//short delay before operation
E=0;
RS=0;
RW=0;
_nop_();
E=1;
Data=c;
E=0;
}
/******************************************************/
void WriteData(unsigned char c)
{
DelayMs(5); //short delay before operation
E=0;
RS=1;
RW=0;
_nop_();
E=1;
Data=c;
E=0;
RS=0;
}
/***********http://www.51hei.comMCU original works******************/
void ShowChar(unsigned char pos,unsigned char c)
{
unsigned char p;
if (pos>=0x10)
p=pos+0xb0; //If it is the second line, the high 4 bits of the command code are 0xc
else
p=pos+0x80; //If it is the second line, the high 4 bits of the command code are 0x8
WriteCommand (p);//write command
WriteData (c); //write data
}
/************************************************************/
void ShowString (unsigned char line,char *ptr)
{
unsigned char l,i;
l=line<<4;
for (i=0;i<16;i++)
ShowChar (l++,*(ptr+i));//Loop display 16 characters
}
/***********************************************************/
void InitLcd()
{
DelayMs(15);
WriteCommand(0x38); //display mode
WriteCommand(0x38); //display mode
WriteCommand(0x38); //display mode
WriteCommand(0x06); //Display cursor position
WriteCommand(0x0c); //Display on and cursor settings
WriteCommand(0x01); //Display clear screen
}
/*--------------------------Delay 1ms subroutine-----------------------*/
delay1000()
{
uint i,j;
for(i=0;i<1;i++)
for(j=0;j<124;j++)
;
}
/*---------------------------Delay 882us subroutine-----------------------*/
delay882()
{
uint i,j;
for(i=0;i<1;i++)
for(j=0;j<109;j++)
;
}
/*--------------------------Delay 2400ms subroutine-----------------------*/
delay2400()
{
uint i,j;
for(i=0;i<3;i++)
for(j=0;j<99;j++)
;
}
void IR_decode()
{
uchar i,j;
while(IR_RE==0);
delay2400();
if(IR_RE==1) //After delaying 2.4ms, if it is high level, it is the new code
{
delay2400(); //Delay 4.8ms to avoid the high level of 4.5msfor
(i=0;i<4;i++)
{
for(j=0;j<8;j++)
{
while(IR_RE==0); //Wait for the first high level of the address code to
arrivedelay882(); //Delay 882ms to judge the pin level at this time
///CY=IR_RE;
if(IR_RE==0)
{
date[i]>>=1;
date[i]=date[i]&0x7f;
}
else if(IR_RE==1)
{
delay1000();
date[i]>>=1;
date[i]=date[i]|0x80;
}
} //1-bit data reception completed
} //32-bit binary code reception completed
}
if (date[2]==0x16)
TR0 = 0;
else if(date[2]==0x14)
{TR0 = 1;
}
else if(date[2]==0x10)
{if (speedlevel>1)
speedlevel--;
else
speedlevel=1;
}
else if(date[2]==0x1A)
{if(speedlevel<5)
speedlevel++;
else
speedlevel=5;
}
else if(date[2]==0x11)
turn=~turn;
[page]
}
void int0() interrupt 0 using 1
{
uint i;
for(i=0;i<4;i++)
{
delay1000();
if(IR_RE==1){k=~k;} //The boot code is 4.5ms at the beginning. If a high level appears within 4ms, the decoding program will be exited
.}
if(k==0)
{
EX0=0; //Disable interrupt when valid signal is detected to prevent interference
IR_decode(); //If a valid signal is received, call the decoding program
//Decoding is successful, call the display program to display the key value
}
EX0=1; //Enable external interrupt to allow new remote control buttons
}
void main(void)
{
InitLcd();//
DelayMs(15);
sprintf(Test1,"STEP MOTOR SPEED");//the first line
ShowString(0,Test1);
ShowChar(0x19,\'r\');
ShowChar(0x1a,\'p\');
ShowChar(0x1b,\ 'm\');
count = 0;
step_index = 0;
spcount = 0;
stop_flag = 0;
P1_0 = 0;
P1_1 = 0;
P1_2 = 0;
P1_3 = 0;
SP=0x60;
EA = 1; //Enable CPU interrupt
TMOD = 0x11; //Set timer 0 and 1 to 16-bit mode 1
ET0 = 1; //Enable timer 0 interrupt
EX0=1;
TH0 = 0xFE;
TL0 = 0x0C; //Set to interrupt every 0.5ms
TR0 = 1; //Start counting
turn=0;
speedlevel=1;
while(1)
{
if(turn==0)
ShowChar(0x14,\'+\');
else
ShowChar(0x14,\'-\');
if(TR0 ==0)
{
ShowChar(0x15 ,\'0\');
ShowChar(0x16,\'0\');
ShowChar(0x17,\'0\');
ShowChar(0x18,\'0\');
}
else
{
if(speedlevel==1 )
{
ShowChar(0x15,\'3\');
ShowChar(0x16,\'0\');
ShowChar(0x17,\'0\');
ShowChar(0x18,\'0\');
}
else if( speedlevel==2)
{
ShowChar(0x15,\'1\');
ShowChar(0x16,\'5\');
ShowChar(0x17,\'0\');
ShowChar(0x18,\'0\');
}
else if(speedlevel==3)
{
ShowChar(0x15,\'1\');
ShowChar(0x16,\'0\');
ShowChar(0x17,\'0 \');
ShowChar(0x18,\'0\');
}
else if(speedlevel==4)
{
ShowChar(0x15,\'0\');
ShowChar(0x16,\'7\');
ShowChar(0x17 ,\'5\');
ShowChar(0x18,\'0\');
}
else if(speedlevel==5)
{
ShowChar(0x15,\'0\');
ShowChar(0x16,\'6\') ;
ShowChar(0x17,\'0\');
ShowChar(0x18,\'0\');
}
} }
}
//
Timer 0 interrupt processing
void timeint(void) interrupt 1 using 0
{
TH0=0xFE;
TL0=0x0C; //When set, interrupt once every 0.5ms
count++;
spcount--;
if(spcount<=0)
{
spcount = speedlevel;
gorun();
}
}
void delay(unsigned int endcount)
{
count=0;
do{}while(count
void gorun()
{
if (stop_flag==1)
{
P1_0 = 0;
P1_1 = 0;
P1_2 = 0;
P1_3 = 0;
return;
}
switch(step_index)
{
case 0: //0
P1_0 = 1;
P1_1
= 0; P1_2
= 0; P1_3 = 0;
break;
case 1: //0
, 1
P1_0 = 1;
P1_1 = 1;
P1_2 = 0;
P1_3 = 0;
break;
case 2: //1
P1_0 = 0;
P1_1
= 1;
P1_2
= 0; P1_3 = 0;
break;
case 3: //1, 2
P1_0 = 0;
P1_1 = 1;
P1_2 = 1;
P1_3 = 0;
break;
case 4: //2
P1_0 = 0;
P1_1
= 0
;
P1_2
= 1; P1_3 = 0;
break;
case 5: //2, 3
P1_0
= 0;
P1_1 = 0;
P1_2 = 1;
P1_3 = 1;
break;
case 6: //3
P1_0 = 0;
P1_1
= 0;
P1_2 = 0; P1_3 =
1;
break;
case 7: //3
, 0 P1_0 = 1;
P1_1 = 0;
P1_2 = 0;
P1_3 = 1;
}
if (turn==0)
{
step_index++;
if (step_index>7)
step_index=0;
}
else
{
step_index--;
if (step_index<0)
step_index=7;
}
}
Previous article:Microcontroller drives buzzer C51 program
Next article:51 single chip microcomputer drives 16×16LED dot matrix to display animated Chinese characters assembly program
Recommended ReadingLatest update time:2024-11-16 16:45
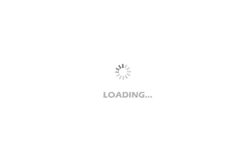
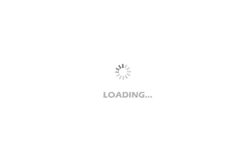
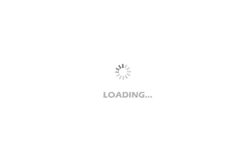
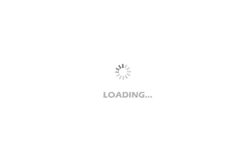
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Principles of Adaptive Filters (5th Edition)
- 10-channel logic analyzer based on VGA display.pdf
- TFTP network burning system
- Introduction to the estimation method of the remaining capacity SOC of lithium batteries
- Advances in radar technology and the development of in-cockpit sensing technology
- Is it so difficult to post a message?
- [RVB2601 Creative Application Development] Ultrasonic pressure-sensitive buttons based on RISC-V processor
- First of all, the 9 boards sent by National Technology have arrived. It's so fast.
- There is no year-end bonus this year.
- Pingtou Ge RRVB2601 Review: Unboxing, Hardware Analysis and Environment Setup