/**************************************************/
/* ds1302 real-time clock C program*/
/*51hei.com member classic works have been tested successfully/
/**************http://www.51hei.com/ziliao/file/ds1302c.rar Click this link to download the code of this example****/
#include < reg52.h>
#include
#define uchar unsigned char
sbit T_CLK = P3^4; /*Real-time clock clock line pin*/
sbit T_IO = P3^3; /*Real-time clock data line pin*/
sbit T_RST = P3^2; /*Real-time clock reset line pin*/
//sbit OE=P3^6;
sbit ACC0=ACC^0;
sbit ACC7=ACC^7;
uchar time[8]=" : : ";
uchar min,hou,day,mon,yea;
void Init1302(void);
void v_W1302(uchar ucAddr, uchar ucDa);
uchar uc_R1302(uchar ucAddr)
;
void v_BurstW1302T(uchar *pSecDa); void v_BurstR1302T(uchar *pSecDa);
void v_BurstW1302R(uchar *pReDa);
void v_BurstR130 2R( uchar *pReDa);
void v_Set1302(uchar *pSecDa);
void v_Get1302(uchar ucCurtime[]);
void donetime(void);
void bcd_int(uchar i);
void bcdtoInt(void);
void SetTime(void);
/** ****************************************
*
* Name: v_RTInputByte
* Description:
* Function: To DS1302 writes 1Byte data
* Call:
* Input: Data written by ucDa
* Return value: None
**************************************** *****************/
void v_WTInputByte(uchar ucDa)
{
uchar i;
//OE=0;
ACC= ucDa;
for(i=8; i>0; i- -)
{
T_IO = ACC0; //* Equivalent to RRC in assembly
T_CLK = 1;
T_CLK = 0;
ACC =ACC>> 1;
}
// OE=1;
}
/********** *****************************************
*
* Name: uchar uc_RTOutputByte
* Description:
* Function: Read 1Byte data from DS1302
* Call:
* Input:
* Return value: ACC
****************************** **********************/
uchar uc_RTOutputByte(void)
{
uchar i;
//OE=0;
for(i=8; i>0; i- -)
{
ACC = ACC>>1; //* Equivalent to RRC in assembly
ACC7 = T_IO;
T_CLK = 1;
T_CLK = 0;
}
//OE=1;
return(ACC);
}
/******** ***********************************************
*
* Name: v_W1302
* Description : Write address first, then write command/data
* Function: Write data to DS1302
* Call: v_RTInputByte()
* Input: ucAddr: DS1302 address, ucDa: data to be written
* Return value: None
******** ************************************************/
void v_W1302(uchar ucAddr, uchar ucDa)
{
//OE=0;
T_RST = 0;
T_CLK = 0;
T_RST = 1;
v_WTInputByte(ucAddr); /* Address, command */
v_WTInputByte(ucDa); /* Write 1Byte data*/
T_CLK = 1;
T_RST = 0;
//OE=1;
} [page]
/**************************** **************************
*
* Name: uc_R1302
* Description: Write address first, then read command/data
* Function: Read a certain address of DS1302 Address data
* Call: v_RTInputByte() , uc_RTOutputByte()
* Input: ucAddr: DS1302 address
* Return value: ucDa : the read data
******************************************************/
uchar uc_R1302(uchar ucAddr)
{
uchar ucDa;
//OE=0;
T_RST = 0;
T_CLK = 0;
T_RST = 1;
v_WTInputByte(ucAddr); /* address, command*/
ucDa = uc_RTOutputByte(); /* read 1Byte data*/
T_CLK = 1;
T_RST =0;
// OE=1;
return(ucDa);
}
/***********************************************
*
* Name: v_BurstW1302T
* Description: Write address first, then write data (clock multi-byte mode)
* Function: Write clock data to DS1302 (multi-byte mode)
* Call: v_RTInputByte()
* Input: pSecDa: Clock data address format: Seconds, minutes, hours, days, months, weeks, years control
* 8Byte (BCD code) 1B 1B 1B 1B 1B 1B 1B 1B
* Return value: None
**********************************************************/
/*void v_BurstW1302T(uchar *pSecDa)
{
uchar i;
v_W1302(0x8e,0x00); //* Control command, WP=0, write operation?
T_RST = 0;
T_CLK = 0;
T_RST = 1;
v_WTInputByte(0xbe); //* 0xbe: Clock multi-byte write command
for (i=8;i>0;i--) //*8Byte = 7Byte clock data + 1Byte control
{
v_WTInputByte(*pSecDa);//* Write 1Byte data
pSecDa++;
}
T_CLK = 1;
T_RST =0;
} */
/*******************************************
*
* Name: v_BurstR1302T
* Description: Write address first, then read command/data (clock multi-byte mode)
* Function: Read DS1302 clock data
* Call: v_RTInputByte() , uc_RTOutputByte()
* Input: pSecDa: Clock data address format: Seconds, hours, minutes, days, months, weeks, years
* 7Byte (BCD code) 1B 1B 1B 1B 1B 1B 1B
* Return value: ucDa: read data
*************************************************/
/*void v_BurstR1302T(uchar *pSecDa)
{
uchar i;
T_RST = 0;
T_CLK = 0;
T_RST = 1;
v_WTInputByte(0xbf); //* 0xbf: clock multi-byte read command
for (i=8; i>0; i--)
{
*pSecDa = uc_RTOutputByte(); //* read 1Byte data
pSecDa++;
}
T_CLK = 1;
T_RST =0;
} */
/**********************************************
*
* Name: v_BurstW1302R
* Description: write address first, then write data (register multi-byte mode)
* Function: write data to DS1302 register (multi-byte mode)
* Call: v_RTInputByte()
* Input: pReDa: register data address
* Return value: None
**********************************************/
/*void v_BurstW1302R(uchar *pReDa)
{
uchar i;
v_W1302(0x8e,0x00); //* Control command, WP=0, write operation?
T_RST = 0;
T_CLK = 0;
T_RST = 1;
v_WTInputByte(0xfe); //* 0xbe: Clock multi-byte write command
for (i=31;i>0;i--) //*31Byte register data
{
v_WTInputByte(*pReDa); //* Write 1Byte data
pReDa++;
}
T_CLK = 1;
T_RST =0;
} */ [page]
/****************************************************
*
* Name: uc_BurstR1302R
* Description: Write address first, then read command/data (register multi-byte mode)
* Function: Read DS1302 register data
* Call: v_RTInputByte() , uc_RTOutputByte()
* Input: pReDa: Register data address
* Return value: None
************************************************/
/*void v_BurstR1302R(uchar *pReDa)
{
uchar i;
T_RST = 0;
T_CLK = 0;
T_RST = 1;
v_WTInputByte(0xff); //* 0xbf: clock multi-byte read command
for (i=31; i>0; i--) //*31Byte register data
{
*pReDa = uc_RTOutputByte(); //* Read 1Byte data
pReDa++;
}
T_CLK = 1;
T_RST =0;
} */
/****************************************************
*
* Name: v_Set1302
* Description:
* Function: Set the initial time
* Call: v_W1302()
* Input: pSecDa: initial time address. The initial time format is: Seconds, hours, minutes, days, months, weeks, and years
* 7Byte (BCD code) 1B 1B 1B 1B 1B 1B 1B
* Return value: None
****************************************************/
/*void v_Set1302(uchar *pSecDa)
{
uchar i;
uchar ucAddr = 0x80;
v_W1302(0x8e,0x00); // Control command, WP=0, write operation?
for(i =7;i>0;i--)
{
v_W1302(ucAddr,*pSecDa); //Seconds, hours, minutes, days, months, weeks, and years
pSecDa++;
ucAddr +=2;
}
v_W1302(0x8e,0x80); // Control command, WP=1, write protection?
} */
/**************************************************
*
* Name: v_Get1302
* Description:
* Function: Read the current time of DS1302
* Call: uc_R1302()
* Input: ucCurtime: Save the current time address. The current time format is: Seconds, Hours, Days, Months, Weeks, Years
* 7Byte (BCD code) 1B 1B 1B 1B 1B 1B 1B
* Return value: None
****************************************************/
/*void v_Get1302(uchar ucCurtime[])
{
uchar i;
uchar ucAddr = 0x81;
for (i=0;i<7;i++)
{
ucCurtime[i] = uc_R1302(ucAddr);//*The format is: Seconds, Hours, Days, Months, Weeks, Years
ucAddr += 2;
}
} */
/**********************************************************
* Name: Init1302
* Description:
* Function: Initialize DS1302
* Call:
* Input:
* Return value: None
**********************************************************/
void Init1302(void)
{
v_W1302(0x8e,0x00); //Control write WP=0
v_W1302(0x90,0xa5);
v_W1302(0x80,0x00);
//Secondsv_W1302(0x82,0x59)
; //Minutesv_W1302(
0x84,0x10); //Hoursv_W1302
(0x86,0x01); //
Dayv_W1302(0x88,0x08); //Monthv_W1302
(0x8a,0x03); //Week
v_W1302(0x8c,0x07); //Year */
v_W1302(0x8e,0x80);
}
/******************************************************
* Name: donetime
* Description:
* Function: Time processing
* Call:
* Input:
* Return value: None
**************************************************************/
void donetime(void)
{
uchar d;
d=uc_R1302(0x81);
time[6]=d/16+48;
time[7]=d%16+48;
d=uc_R1302(0x83);
time[3]=d/16+48;
time[4]=d%16+48;
d=uc_R1302(0x85);
time[0]=d/16+48;
time[1]=d%16+48;
//bcd_int(d);
}
//
/*void bcd_int(uchar i)
{
uchar j;
time[0]=(i&0x0f)+48;
j=i>>4;
time[1]=(j&0x0f)+48;
} */
//
/*void bcdtoInt(void)
{
uchar i;
for(i=0;i<7;i++)
bcd_int(ucCurtm[i]);
} */
//
void SetTime(void)
{
v_W1302(0x8e,0x00);
v_W1302(0x80,0x80);
v_W1302(0x82,min);
v_W1302(0x84,hou);
v_W1302(0x86,day);
v_W1302(0x88,mon);
v_W1302(0x8c,yea);
v_W1302(0x80,0x00);
v_W1302(0x8e,0x80);
}
Previous article:Single chip microcomputer drives 16*16 dot matrix LED Chinese character display C51 program
Next article:Single chip one-button multi-function key recognition technology
Recommended ReadingLatest update time:2024-11-16 16:20
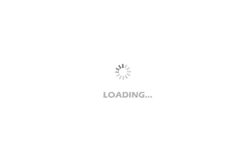
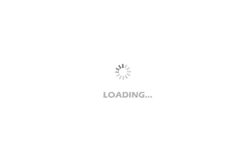
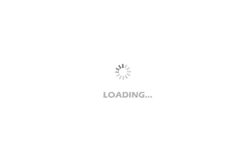
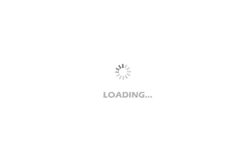
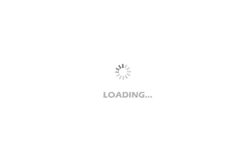
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Talk: Small capital investment
- Where does the EG8010 dead time go?
- Downloads|Selected Courseware & Latest Materials from Tektronix 2019 Semiconductor Seminar
- GaN Power HEMT > 650 V: Parametric Analysis and Comparison with SiC MOSFET
- Voltage controlled current source composed of opa547
- [GUIX①, one of threadX components] guix text button
- Qorvo Technology Video: GaN Solutions for Sub-6 GHz 5G Base Station Applications
- [Jihai M3 core APM32E103VET6S MINI development board] 02. USART online interaction function
- PCB design of RF devices
- [TI star products limited time purchase] TI's latest shopping experience