[
Experimental task
]
Using the characteristic of 24C08 that the stored data does not disappear after power failure, you can make a power failure protection device. First, use the single-chip microcomputer to make an automatic timer of 0-99 seconds. Then randomly turn off the power supply, and after power is turned on, the timer continues to count from the state before power failure.
[
Experimental Principle
]
First, briefly explain the following I2C bus. The I2C bus is a serial data bus with only two signal lines, one is the bidirectional data line SDA, and the other is the clock line SCL. A data byte transmitted on the I2C bus consists of eight bits. The bus has no limit on the number of bytes transmitted each time, but each byte must be followed by an acknowledge bit. Data transmission first transmits the highest bit (MSB), and data transmission is performed in the format shown in Figure 1. First, the host sends a start signal "S" (SDA jumps from high level to low level during the high level of SCL), and then the host sends a byte of data. The first byte of data after the start signal has a special meaning: the upper seven bits are the address of the slave, and the eighth bit is the transmission direction bit. 0 means that the host sends data (write), and 1 means that the host receives data (read). The addressed slave device is set to the corresponding working mode according to the transmission direction bit. The devices of the standard I2C bus have a seven-bit address. All devices connected to the I2C bus receive the first byte after the start signal and compare the received address with their own address. If the address matches, it is the slave that the host wants to visit, and it should send a low level to the SDA line as a response during the ninth clock pulse. In addition to the first byte being a general call address or a ten-bit slave address, the second byte is the data byte. After the data transmission is completed, the host sends a stop signal "P" (SDA jumps from a low level to a high level during the high level of SCL).
AT24C series serial E2PROM has I2C bus interface function, low power consumption, wide power supply voltage (2.5V~6.0V according to different models), working current is about 3mA, static current varies with power supply voltage, 30μA~110μA, AT24C series serial E2PROM parameters are as follows
Model Capacity Device addressing byte (8 bits) Number of bytes loaded at a time
AT24C01 128×8 1010A2A1A0 R/W 4
AT24C02 256×8 1010A2A1A0 R/W 8
AT24C04 512×8 1010A2A1P0 R/W 16
AT24C08 1024×8 1010A2P1P0 R/W 16
AT24C16 2048×8 1010P2P1P0 R/W 16Since
the I2C bus can connect multiple serial interface devices, each device in the I2C bus should have a unique device address. According to the I2C bus rules, the device address is 7-bit data (that is, in theory, 128 devices with different addresses can be connected to an I2C bus system). It and the 1-bit data direction bit constitute a device addressing byte, and the lowest bit D0 is the direction bit (read/write). The highest 4 bits (D7~D4) in the device addressing byte are the device model address. The model addresses of different I2C bus interface devices are given by the manufacturer. For example, the model address of the AT24C series E2PROM is 1010. The lower 3 bits in the device address are the pin addresses A2 A1 A0, corresponding to the D3, D2, and D1 bits in the device addressing byte, which are given by the connected pin level during hardware design.
The read and write operations of the AT24C series E2PROM fully comply with the I2C bus's master-receive-slave-transmit and master-transmit-slave-receive rules.
[
C language source program
]
#include
#include
#include
unsigned char code table[]={0x3f,0x06,0x5b,0x4f,0x66,
0x6d,0x7d,0x07, 0x7f,0x6f,};
unsigned char sec; //Define the count value, every second, sec increases by 1
unsigned int tcnt; //Number of timer interrupts
bit write=0; //Write the flag of 24C08;
sbit gewei=P2^0; //Ones bit selection definition
sbit shiwei=P2^1; //Tens bit selection definition
//////////24C08 read and write driver /////////////////////
sbit scl=P3^4; // 24c08 SCL
sbit sda=P3^5; // 24c08 SDA
void delay1(unsigned char x)
{ unsigned int i;
for(i=0;i
void flash()
{ ; ; }
void x24c08_init() //24c08 initialization subroutine
{scl=1; flash(); sda=1; flash();}
void start() //Start I2C bus
{sda=1; flash(); scl=1; flash(); sda=0; flash(); scl=0; flash();}
void stop() //Stop I2C bus
{sda=0; flash(); scl=1; flash(); sda=1; flash();}
void writex(unsigned char j) //write a byte
{ unsigned char i,temp;
temp=j;
for (i=0;i<8;i++)
{temp=temp<<1; scl=0; flash(); sda=CY; flash(); scl=1; flash();}
scl=0; flash(); sda=1; flash();
}
unsigned char readx() //read a byte
{
unsigned char i,j,k=0;
scl=0; flash(); sda=1;
for (i=0;i<8;i++)
{
flash(); scl=1; flash();
if (sda==1) j=1;
else j=0;
k=(k<<1)|j;
scl=0;}
flash(); return(k);
}
void clock() // I2C bus clock
{
unsigned char i=0;
scl=1; flash();
while ((sda==1)&&(i<255))i++;
scl=0; flash();
}
////////Read a byte of data from address address of 24c02/////
unsigned char x24c08_read(unsigned char address)
{
unsigned char i;
start(); writex(0xa0);
clock(); writex(address);
clock(); start();
writex(0xa1); clock();
i=readx(); stop();
delay1(10);
return(i);
}
//////Write one byte of data to the address of 24c02 info/////
void x24c 08_write(unsigned char address,unsigned char info)
{
EA=0;
start(); writex(0xa0);
clock(); writex(address);
clock(); writex(info);
clock(); stop();
EA=1;
delay1(50);
}
/////////////24C08 read and write driver completed/////////////////////
void Delay(unsigned int tc) //Delay program
{
while( tc != 0 )
{unsigned int i;
for(i=0; i<100; i++);
tc--;}
}
void LED() //LED display function
{
shiwei=0; P0=table[sec/10]; Delay(8); shiwei=1;
gewei=0; P0=table[sec%10]; Delay(5); gewei=1;
}
void t0(void) interrupt 1 using 0 //Timed interrupt service function
{
TH0=(65536-50000)/256; //Assign TH0 TL0
TL0=(65536-50000)%256; //Reload the initial value of the count
tcnt++; //Every 250ust, tcnt increases by one
if(tcnt==20) //When the count reaches 20 times (1 second)
{
tcnt=0; //Recount
sec++;
write=1; //Write to 24C08 once per second
if(sec==100) //Timer is 100 seconds, then start the count from zero
{sec=0;}
}
}
void main(void)
{
TMOD=0x01; //Timer works in mode 1
ET0=1; EA=1;
x24c08_init(); //Initialize 24C08
sec=x24c08_read(2);//Read the saved data and assign it to sec
TH0=(65536-50000)/256; //Assign TH0 TL0
TL0=(65536-50000)%256; //Make the timer interrupt once every 0.05 seconds
TR0=1; //Start timing
while(1)
{
LED();
if(write==1) //Judge whether the timer has counted for one second
{
write=0; //Clear
x24c08_write(2,sec); //Write data sec at address 2 of 24c08
}
}
}
[
Hardware Circuit Diagram
]
Previous article:I2C read and write operation experiment based on single chip microcomputer
Next article:Application experiment of analog-to-digital conversion DAC0832
Recommended ReadingLatest update time:2024-11-16 17:46
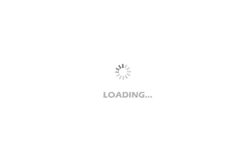
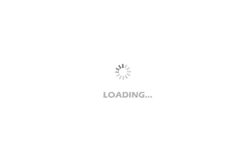
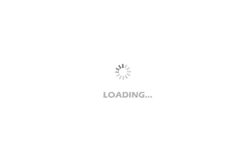
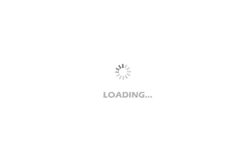
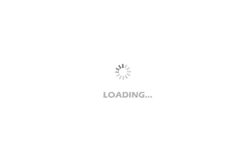
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Compiler Principles C Language Description (Ampel)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Simulation Help Post
- Based in Chengdu, job position: Reliability Engineer
- Use ST's new APP to instantly find the most suitable sensor
- [XMC4800 Relax EtherCAT Kit Review] + Getting started with DAVE, quickly build your own webserver
- [GD32L233C-START Review] + Guess which light will be on next time
- How to convert brd file to ad pcb file
- 【AT32WB415 Review】Serial communication test (including Bluetooth test)
- TI Voltage Reference (VREF) Application Design Tips and Tricks
- What happens if the input voltage of the window comparator is equal to the upper and lower limits?
- Banknote number recognition system based on ARM.pdf