Preface
DS18B2 is generally used in conjunction with a single-chip microcomputer, and there are few reports on the interface between DSP and DS18B20. Therefore, this article introduces in detail the connection method between TMS320LF2407 and DS18B20, and also introduces in detail how to use C language to complete precise software delay, thereby completing the data communication between DS18B20 and TMS320LF2407 based on the single-wire bus protocol.
1 DS18B20 Timing
1. 1 Reset Timing
When resetting DS18B20, it must be reset first before executing other commands. When resetting, the host pulls the data line to a low level and maintains it for 480us to 960us, then releases the data line, and then the pull-up resistor pulls the data line high for 15 to 60us, waiting for DS18B20 to send a presence pulse. The presence pulse is valid for 60 to 240us, and the reset operation is completed.
1.2 Write Timing
When the host writes data to DS18B20, the data line is first set to a high level and then to a low level. The low level should be greater than 1us. Within 15us after the data line becomes a low level, the data line is made high or continues to be low according to writing "1" or writing "0". DS18B20 will sample the data line within 15us to 60us after the data line becomes a low level. The duration of data written to DS18B20 should be greater than 60us and less than 120us, and the time interval between two writes should be greater than 1us.
1.3 Reading Timing
Read time slot When the host reads data from DS18B20, the host first sets the data line to high level, then changes it to low level. The low level should be greater than 1us, and then releases the data line to make it high level. DS18B20 sends data to the data line within 15us when the data line changes from high level to low level. The host can read the data line after 15us.
2 Connection between TMS320LF2407 and DS18B20
DS18B20 has three pins. The wiring diagram between it and TMS320LF2407A is as follows. The VDD pin is connected to 5V voltage to power the sensor . The DQ pin is the data line. When connected to I OP A6 of TMS320LF2407A, it is also connected to a 4.7K pull-up resistor and connected to a 5V power supply so that the data line can be automatically pulled up to a high level in the idle state. The GND pin is grounded.
3 Software Design
3.1 Implementation of Accurate Software Timing
As we all know, the core of TMS320LF2407 belongs to the C2000 series processor , and the processing speed reaches 30MIPs, which means that the time spent on each instruction is only 33ns in theory. However, in reality, due to factors such as the data processing speed and data call of the DSP external circuit, the processing speed often cannot reach this order of magnitude. So how to measure the processing time of each instruction and calculate the precise time of the software delay program? The calculation method is as follows:
1. The C language code of the delay program is:
for(loopindex=0;loopindex
where loopindex is unsigned int, unsigned integer value, N is a constant, calculated according to the required delay time. The specific algorithm is given below.
2. The assembly language generated by the compiler in CCS after compiling this for statement is:
LACL #0h
SACL *, 0
LACL *
SUB N (calculated number of loops)
BCND Address to branch to, GEQ
LACC *, 0
ADD #1h
SACL *, 0
LACL *
SUB N (calculated number of loops)
BCND Address to branch to, LT
The time taken by these assembly statements can be calculated as follows: DELAY = (9N + 4) * single instruction cycle.
3. Test an instruction cycle
The crystal used in the system is 20M, the clock frequency after multiplication is 40M, and the division frequency of timer 1 is 1, which means that the timing cycle of the timer is 25ns. Turn on the timer and run the above assembly statement in single step to see the value increased after each time the timer runs. The test shows that after each single step of running the assembly statement that takes up one instruction cycle, the value of the timer increases by 10. In other words, the time occupied by the instruction of each single instruction cycle is: 25ns*10=250ns, which is 0.25us.
4. The final calculation formula of the delay program is:
DELAY = (9n + 4) * 0.25us.
It can be seen from the formula that when n=0, DELAY=1us, and when n=65535, DELAY=150ms.
3.2 Main procedures
Due to the length of the article, only the reset program, byte reading program and the entire temperature reading main program are given here. The program for writing command words can be written by yourself according to the timing described above.
#define nop() {asm( nop );}
#define DATA_PORT PADATDIR
#define DATA_MODE 0x0040
#define DATA_OUT 0x4000
#define DATA_BIT 0x0040
#define PIN_HIGH() {PADATDIR=PADATDIR|DATA_OUT|DATA_BIT;}
#define PIN_LOW() {DATA_PORT =(PADATDIR|DATA_OUT)&(~DATA_BIT);}
[page]
#define PIN_LEAVE() {DATA_PORT=DATA_PORT&(~DATA_OUT)|DATA_BIT;}
/*
Sensor
Reset procedure*/
unchar reset(void)
{ unchar retval;
unint loopindex="0";
PIN_HIGH();
nop();nop();nop();nop(); /* Delay 1us*/
PIN_LOW();
for(loopindex=0;loopindex<213;loopindex++){;} /* Set the bus to low level and keep it for at least 480us */
PIN_HIGH();
for(loopindex=0;loopindex<26;loopindex++){;} /* Wait for the resistor to pull the bus high and keep it for 15-60us */
PIN_LEAVE(); /* Receive the response signal*/
nop();
if((DATA_PORT & DATA_BIT) == 0x0000)
{ retval = 0; }
else
{ retval = 1; }
PIN_HIGH();
for(loopindex=0;loopindex<106;loopindex++){;} /*Delay 60-240us */
return(retval); /*Return response signal*/
}
/*Read a byte from the sensor*/
void read_byte(unchar *ReadByte)
{
int i;
unchar temp="0";
unint loopindex="0";
PIN_HIGH();
for(i=0;i<8;i++)
{
temp="temp">>1;
PIN_LOW();
nop();nop();nop();nop(); /* Delay 2us */
nop();nop();nop();nop();nop();
PIN_HIGH();
for(loopindex=0;loopindex<6;loopindex++){;} /* Delay 14us */
PIN_LEAVE();
nop();
if((DATA_PORT & DATA_BIT) == 0x0000)
{ temp="temp" & 0x7F; }
else
{ temp="temp" | 0x80; }
PIN_HIGH();
for(loopindex=0;loopindex<26;loopindex++){;} /* Delay 60us */
}
*ReadByte=temp;
return;
}
/* Convert the read temperature into decimal*/
float transform(unchar *T)
{
unchar temp="0";
float temprature="0";
temp=temp|(*(T+1)<<8);
temp=temp|*T;
if((temp&0x0F800)==0x0F800)
{ temprature="0-"((~temp)+1)*0.0625; }
else
{ temprature="temp"*0.
0625; }
return(temprature);
}
/* Main function to read temperature, return decimal temperature value*/
float read_temp()
{
Unchar buff[2];
float temprature="0";
unint loopindex="0";
while (reset()==1){}; /* Reset and wait for slave response*/
write_byte(0xCC); /* Ignore ROM match*/
write_byte(0x44); /* Send temperature conversion command*/
for(loopindex=0;loopindex<65535;loopindex++){;} /* Delay 300ms, wait for
digital-to-analog conversion
*/
for(loopindex=0;loopindex<65535;loopindex++){;}
while(reset()==1){}; /* Reset again, wait for slave response*/
write_byte(0xCC); /* Ignore ROM match*/
write_byte(0xBE); /* Send temperature read command*/
read_byte(buff); /* Read the lower 8 bits of temperature*/
read_byte(buff+1); /* Read the higher 8 bits of temperature*/
temprature="transform"(buff);
PIN_HIGH(); /* Release the bus */
return(temprature);
}
4 Conclusion
DS18B20 is an excellent single bus digital sensor. The hardware design is simple and the operation is reliable. By analyzing the assembly language compiled by C language, the software delay time can be clearly calculated to meet the timing requirements of single-wire bus communication, so that the software design of DSP and DS18B20 communication can be completed excellently.
5 This paper has two innovative points:
1. Compile the corresponding C language delay program through the C compiler to get its corresponding assembly language, and then use the timer to know the time of a single instruction cycle, so as to accurately calculate the delay time of the software delay program.
2. Usually DS18B20 is used in conjunction with a single-chip microcomputer, but this article introduces in detail the combined use of DS18B20 and DSP. The main difference is in the operation of the port and the control of the delay.
Previous article:Electromagnetic compatibility analysis of single-chip computer system for intelligent frame-type circuit breaker
Next article:Noteworthy design principles of single chip microcomputer control board
Recommended ReadingLatest update time:2024-11-16 15:57
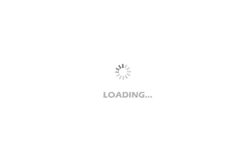
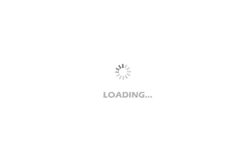
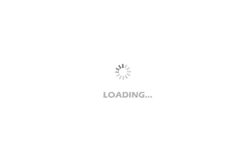
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- msp430f5529 interrupt notes--interrupt nesting
- Wearable ECG monitoring
- General test methods for wireless communication equipment
- I started to enter the Huada MCU again
- Research on Airport Runway Identification Algorithm and Implementation
- Regarding the clamping problem, discuss
- How to drive FT232+stm32?
- CBG201209U201T Product Specifications
- Keil compiles ADuC7029 and reports an error
- Technical Article: Optimizing 48V Mild Hybrid Electric Vehicle Motor Drive Design