Introduction
Due to the limitation of system memory size, it is not realistic to run the desktop X Windows system directly on the ARM platform running Linux. Microwindows is an open source embedded GUI software, which aims to introduce the graphical window environment to small devices and platforms running Linux. As an alternative to the X Windows system, Microwindows can provide similar functions with less RAM and file storage space (100K~600KB), allowing designers to easily add various display devices, mice, touch screens and keyboards. At the same time, Microwindows has very good portability and has been successfully ported to various platforms such as MIPS and ARM.
Microwindows porting to ARM platform
Although there is code supporting ARM processors in the arch directory of Linux, since Linux is implemented on the X86 platform, many aspects do not take into account the particularity of the ARM platform. To port Microwindows to the ARM hardware platform running the ARM-Linux operating system, the following steps are required.
1) Replace the fork() system call. Since ARM-Linux is different from standard Linux, the Microwindows source code developed with the standard Linux kernel as the support target must also be modified accordingly to adapt to the ARM-Linux system. The main problem is that ARM-Linux does not provide a fork() system call, but replaces it with a vfork() call. Therefore, the use of fork() in the ARM-Linux code needs to be modified. Macro definitions can be used to easily replace all fork() calls with vfork(). Modify the Microwindows compilation setting file and use the ARM cross-compiler arm-elf-gcc.
2) Determine the parameters passed to the display driver. Specifically, it is necessary to pass the basic parameters of the display to the device driver when opening the FrameBuffer device /dev/fb0. Modify the following in the fb_open(PSD psd) function in scr_fb.c:
psd->xres=psd->xvirtres=320;
psd->yres=psd->yvirtres=240;
psd->linelen=40;
psd->size=320×320;
3) Compile Microwindows. Establish an ARM cross-compilation environment under Red Hat 9.0, modify the Makefile file, specify the $(CC) compilation parameter as arm-elf-gcc in the installation directory of the cross-compilation environment, and recompile the code to generate a program that can run on the ARM platform. The instruction systems of the ARM series processors are compatible with each other, and the code compiled by arm-elf-gcc can run on various processors based on the ARM core.
Microwindows Chinese localization
In order to make Microwindows support simplified Chinese characters, it is necessary to modify the devfont.c of the engine layer accordingly. The core data structure and operation functions of Microwindows for font operations are defined in the devfont.c file. Since Microwindows adopts an object-oriented design method, as long as a series of data structures and operation functions for simplified Chinese are redefined and registered with the system, the system can be localized. The data structures and functions that need to be redefined are:
static MWFONTPROCS hzk_procs={
MWTF_ASCII, /*routines expect ASCII */
Hzk_getfontinfo,
Hzk_gettextsize,
NULL,
Hzk_destroyfont,
Hzk_drawtext,
Null,
Null,
};
Microwindows graphics programming mechanism
Microwindows adopts a layered design method in principle. Each layer completes a specific function and can be adapted or rewritten for different applications without affecting other layers. At the bottom layer, the drivers of the display, mouse, touch screen, etc. provide access to hardware devices related to interaction; the middle layer is a streamlined graphics engine that provides a variety of basic graphics functions such as line drawing, area filling, polygons, etc.; the top layer provides a rich programming interface function (API) for graphics applications, through which the appearance of the desktop and window can be customized. Currently, Microwindows provides two sets of API interfaces to better adapt to the porting of applications on different platforms, namely, the API that is basically compatible with Win32/Win CE and the Nano-X API that uses the X system.
Device driver layer
The interface of the device driver is defined in the device.h file. The device-independent graphics engine routines provided by the middle layer interact with the hardware device by calling the device driver. This ensures that when the platform hardware device changes, only the corresponding driver needs to be rewritten without modifying the upper layer code. Microwindows provides a FrameBuffer device driver based on the Linux2.4.X kernel. FrameBuffer works through the /dev/fb0 device file in the Linux system, and the display buffer is mapped to the system memory through the mmap() system call. [page]
Device-independent graphics engine layer
The core graphics functions in the Microwindows system are implemented in the graphics engine layer by calling the lower-level hardware device drivers. User applications usually do not directly call the routines of the engine layer, but call the programming interface provided by the top layer. The independence of the core graphics engine routines from the application interface is mainly based on the following considerations: The core routines always reside on the server side in the Client/Server environment. The bitmap and text formats called by these routines are optimized to make the execution faster, so these formats are usually different from those used by the application. In addition, the core routines often use pointers to generate more complex and efficient but less logical code, rather than using the ID numbers commonly used by applications. In the Microwindows source code, the core routines are usually included in the Devdraw.c, Devclip.c, Devmouse.c, Devkbd.c and DevpalX.c files:
Device context
The application must set the device context before calling the graphics drawing API function. Some information, such as the current coordinate system and the current window, remains unchanged for a long time during the execution of the program, so there is no need to pass it to each function called. Therefore, these relatively persistent information can be notified to the system through the setting of the device context. At the same time, many properties such as the current foreground color and the current background color should also be set in the device context. You can get the current device context by calling GetDC. After a series of drawing is completed, call the ReleaseDC function to release the DC object.
Message passing mechanism
The most basic communication mechanism between Microwindows APIs is message passing. A message contains an agreed message number and two parameters: wParam and lParam. The message is stored in the application's message queue and can be obtained by calling the GetMessage() function. When waiting for a message, the application is blocked. Some messages are related to hardware events, such as WM_CHAR for keyboard input and WM_LBUTTONDOWN for the left mouse button pressed. At the same time, the creation and elimination events of windows correspond to WM_CREAT and WM_DESTROY messages respectively. In general, each message corresponds to a window identified by HWND. After receiving the message, the application dispatches the message to the corresponding window for processing by calling DispatchMessage(). When the window is created, the processing functions of various messages corresponding to the window are defined at the same time, so the system knows which window to pass the message to. The message passing mechanism allows the core API to implement various functions, such as window creation, drawing, and movement, by passing messages corresponding to various events. Usually, the relevant window operation messages are processed by the DefWindowsProc function by default, so that the actions of all windows are consistent in behavior. When a window requires special operations, the user can meet the requirements by rewriting the processing program. The functions that can directly process messages include SendMessage, PostMessage, PostQuitMessage, GetMessage, and DispatchMessage.
Creation and elimination of windows
The entry point of a Microwindows application is the WinMain function, not the usual Main(). In the Microwindows API, the most basic display unit is the window, which defines a display area and various message processing functions related to it. Windows can be customized by predefined types, such as buttons, text boxes, etc., and special types can also be defined by the user. Regardless of how the type is defined, the method of creating windows and message communication is the same. The functions related to creating and eliminating windows are RegisterClass, UnRegisterClass, CerateWindowEx, DestroyWindow, GetWindowLong, and SETWindowLong.
Displaying and moving windows
The ShowWindow function allows the device window attribute to be visible or hidden. This attribute can also be implemented by CreateWindowEx during the window creation process. The movement of windows includes changes in the window position or size. When the window position changes, the system sends a WM_MOVE message; when the window size changes, the system sends a WM_SIZE message. Window
drawing
When other windows move and cause a window to be drawn or redrawn, the Microwindows system will send a WM_PAINT message to the relevant window procedure. At this time, it is up to the application to call the graphics operation function to draw the window. Micro windows maintains an UPDATE field for each window, and sends a WM_PAINT message to the window when UPDATE is not empty. For speed considerations, the WM_PAINT message is sent only when there are no other messages in the application queue. This ensures that the application can redraw the window in one step instead of being divided into multiple steps. If you don't want to wait, you can call the UPDATEWindow function to force the window to redraw.
Window area and absolute coordinates
When each window is drawn on the display, it should refer to the absolute coordinates of the pixels on the display. The Microwindows API allows application programmers to use relative coordinates based on the upper left corner of the window in the area of the window that does not include the title bar. This area is called the client area. The GetClientRect function and the GetWindowRect function will return the absolute coordinates of the client area and the window. The ClientTo Screen function and the ScreenToClient function complete the conversion between absolute coordinates and relative coordinates.
Conclusion
By porting Microwindows to the ARM platform, a friendly graphical user interface similar to the X Windows desktop system is implemented in the ARM-based embedded system while maintaining low consumption of system resources. Users familiar with graphical applications can write their own graphical applications on this system. Microwindows will play a greater role in future embedded system designs.
Previous article:Select an operating system for ARM CPU
Next article:Software Simulation of SPI Interface in Embedded Home Gateway
Recommended ReadingLatest update time:2024-11-16 21:36
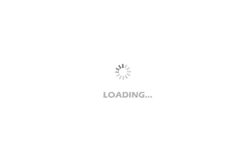
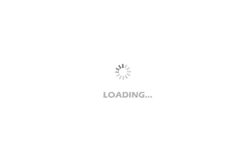
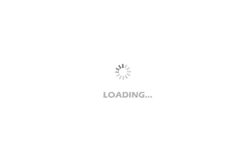
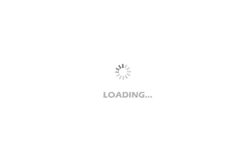
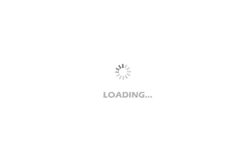
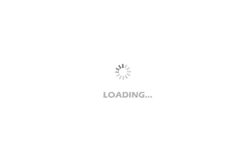
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Electronics Bible: The Art of Electronics by Paul Horowitz
- RSL10 Smart Shot Camera Platform Evaluation Board
- Bootstrap Design of Operational Amplifier Power Supply
- Understand the process of embedded product development
- BQ27542 mass production burning and calibration i2c tool design
- Does ramfifo ip TD5.5.1-64bit not support asynchronous clock?
- EEWORLD University Hall----Live Replay: Application of TI mmWave Sensors in Smart Home
- Download address of 3gpp/3gpp2
- MicroPython Hands-on (04) - Basic Examples of Learning MicroPython from Scratch
- What is 802.11ah or HaLow?