1 Embedded Software Development Process
Refer to the embedded software development process. Step 1: Project creation and configuration. Step 2: Edit source files. Step 3: Project compilation and linking. Step 4: Software debugging. Step 5: Execution file solidification.
In the whole process, users first need to create a project and make preliminary configurations for the project, including configuring the processor and debugging equipment. Edit the project files, including the assembly and C language source programs written by the user, as well as the link script files that need to be written when compiling the project, the storage area image files and command script files that need to be written during the debugging process, and the startup program files for the program running entry during power-on reset.
It is important to understand the last four files, and their functions are explained below.
(1) Link script file: It is effective when the program is compiled. This file describes the information related to the code link positioning, including code segment, data segment, address segment, etc. The linker must use this file to correctly locate the code of the entire system. The link script files for debugging programs in SDRAM, debugging in FLASH, or running after solidification should be distinguished. (Use the extension *.ld in the IDE development environment)
(2) Command script file: It is effective when debugging a program in SDRAM. When the integrated environment is connected to the target, during software debugging, and after the target board is reset, the integrated environment is sometimes required to automatically complete some specific operations, such as resetting the target board, clearing the watchdog, masking the interrupt register, and memory area mapping. These operations can be completed by executing a set of command sequences. The text file that saves a set of command sequences is called a command script file (using the extension *.cs in the IDE development environment).
(3) Storage area image file: It is used when debugging programs in SDRAM. Accessing illegal storage areas during software debugging will cause exceptions on some processors and target boards. If the exceptions are not handled, the software debugging process will not be able to continue. In order to prevent the above problems and adjust the emulator access speed to the most appropriate level, a file called storage area image file (using the extension *.map in the IDE development environment) is provided to describe the properties of each storage area.
During the debugging process of the program, you can choose to use the storage area image file *.map and command script file *.cs to assist in the debugging of the program.
(4) Startup file: It mainly completes some hardware-related initialization work to prepare for the application. Generally, the first step of the startup code is to set up interrupts and exception vectors; the second step is to complete the register configuration required for system startup; the third step is to set up the watchdog and some peripheral circuits designed by the user; the fourth step is to configure the storage area used by the system to allocate address space; the fifth step is variable initialization; the sixth step is to set the stack pointer for each operating mode of the processor; and the last step is to enter the high-level language entry function (Main function).
2 Interrupt Programming
For interrupt debugging, a dynamic processing method similar to vector interrupt can be used, where the fixed address code corresponding to the interrupt is transferred to a fixed address in RAM, and a function pointer is defined to point to the fixed address. The interrupt handling function can then be dynamically changed at any time by replacing the code at the fixed address in RAM.
The specific method is:
(1) Define the interrupt source function pointer at a relative fixed address in RAM and create an interrupt vector table;
void SetInterrupt (U32 vector, void (*handler)())
{
InterruptHandlers[vector] = handler;
}
(2) In the program, call the interrupt handling function of a specific interrupt source;
For example: SetInterrupt(IIC_INT,IICWriteIsr);
/* Declare the IIC interrupt processing function, where IIC_INT is the IIC interrupt source number, and IICWriteIsr is the IIC write interrupt processing function*/
(3) In the IRQ at 0x18 or the FIQ interrupt entry function at 0x1C, obtain the interrupt source, clear the interrupt pending flag, and enter the user's specific interrupt handler through the defined interrupt source function pointer. [page]
void ISR_IrqHandler(void)
{
IntOffSet = (unsigned int)INTOFFSET;
Clear_PendingBit(IntOffSet>>2) ;
(*InterruptHandlers[IntOffSet>>2])();
//Call a specific interrupt handler
}
By adopting a dynamic interrupt handling method, the interrupt response time and program performance are optimized when there are many interrupt sources. In addition, in terms of debugging, this processing method has the advantage of being easy to track and debug, and the interrupt handling function can be easily changed as needed.
3 Interrupt debugging
Software debugging can be done in SDRAM or FLASH. In SDRAM, reading and writing are convenient and the access speed is fast. Generally, software debugging should be done in RAM, but when the RAM space is smaller than the FLASH program space, the program can only be run and debugged in FLASH, or when the user wants to know the actual running status of the program in FLASH, the program can be debugged in FLASH.
When performing interrupt debugging, it should be noted that the interrupt entry is located at address 0x18 or 0x1c in SDRAM or FLASH. The link script file must correctly locate the code of the entire system at the beginning of 0x0, but the link script file and project configuration corresponding to SDRAM or FLASH should be different.
(1) The program runs in SDRAM
Debugging in SDRAM uses the link script file corresponding to SDRAM. The debugging process requires the following steps: compiling and linking the project; connecting the emulator and the circuit board; downloading the program (using the extension *.elf in the IDE development environment); debugging.
Before downloading the program, you must start the command script file to complete some of the above-mentioned specific operations. The command script file is started automatically when the emulator is connected. The storage area mapping should be the same as when the program is running in SDRAM, ensuring that the code of the entire system is correctly located at the beginning of 0x0. The starting address of the download program is also 0x0. After the download is successful, debugging can be carried out.
(2) The program runs in FLASH
Debug in FLASH, use the link script file corresponding to FLASH. The debugging process requires the following steps: compile and link the project; connect the emulator and the circuit board; convert the program format (convert *.elf to *.bin); solidify the *.bin program; debug.
After connecting to the emulator, there is no need to download the program. The storage area mapping is completed by running the startup file in the project itself, and no command script file is required. During the debugging process in this environment, two hardware breakpoints can be set.
(3) The program is transferred from FLASH to SDRAM for execution
In some applications, when the program running speed is emphasized, it is hoped that the program will run in SDRAM. In this case, the program stored in FLASH needs to be moved to a certain space in SDRAM after the system is powered on, and then run automatically. This so-called Bootloader technology is often used in DSP systems.
The debugging process is divided into two steps:
(a) First debug the user program in SDRAM, and then fix the *.bin file to a non-0 sector address space of FLASH;
(b) Debug the Bootloader transfer program written by yourself and fix the Bootloader.bin file to the 0 sector address space of FLASH. After the system is powered on, the transfer program will transfer the program stored in a non-0 sector address space of FLASH in (a) to the same space location in SDRAM debugging, thereby achieving the purpose of running the program in SDRAM.
Also note that because the user's actual program interrupt entry must be located at address 0x18 or 0x1c of FLASH, the Bootloader transfer program should also have the function of jumping to the interrupt entry, that is, to transfer the PC pointer to the interrupt program entry table in the SDRAM space, which is the location where the entire user program is transferred to SDRAM.
Such as: LDR PC, =HandleIRQ
// HandleIRQ is located in the SDRAM space interrupt program entry table
Previous article:How to automatically run a specific program after ARM Linux boots up
Next article:Wireless Communication System of Multifunctional Monitor
Recommended ReadingLatest update time:2024-11-16 22:44
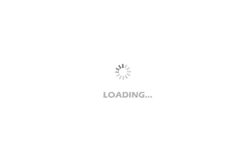
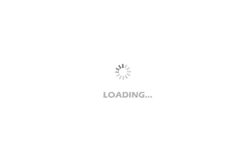
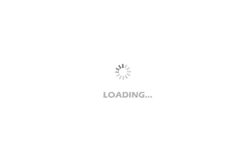
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【TI recommended course】#Power system design tools#
- Question about username
- NeoPixel simulates fluid physics motion
- BMP library management based on FPGA.pdf
- Making some preparations for the flag I set up on the forum this year (first time shopping at the ghost market)
- Open source scientific calculator based on STC32G
- FPGA---DDS Sine Wave Generator
- FPGA/DSP/GPU, accelerating radar signal processing
- Help! Can someone help me analyze a small circuit? Thank you!
- Analysis of the principle of switching power supply