Dynamic scanning display interface circuit and program
In the single-chip system, the dynamic scanning display interface is one of the most widely used display methods in the single-chip system. Its interface circuit connects the same-named ends of the 8 stroke segments ah of all displays together, and the common pole COM of each display is independently controlled by the I/O line. When the CPU sends the glyph code to the field output port, all displays receive the same glyph code, but which display is lit depends on the COM end, which is controlled by the I/O, so we can decide when to display which bit. The so-called dynamic scanning means that we use the time-sharing method to control the COM end of each display in turn, so that each display is lit in turn.
During the scanning process, the lighting time of each display is extremely short (about 1ms). However, due to the persistence of vision and the afterglow effect of the light-emitting diode, although the displays are not actually lit at the same time, as long as the scanning speed is fast enough, it gives the impression of a stable set of display data without flickering.
The following figure shows the dynamic scanning interface on our microcontroller experiment board. The P0 port of 89c51 can inject a large current, so we use a common anode digital tube, and do not use a current limiting resistor, but only use two 1N4004 to step down the voltage to power the digital tube. Only two are used here, and it can actually be expanded. Their common end is controlled by the PNP transistor 8550. Obviously, if 8550 is turned on, the corresponding digital tube can light up, and if 8550 is turned off, the corresponding digital tube cannot light up. 8550 is controlled by P2.7 and P2.6. In this way, we can control the lighting or extinguishing of a certain digital tube by controlling P27 and P26.
The following single-chip microcomputer program uses the digital tube on the experimental board to display 0 and 1.
FIRST EQU P2.7 ; bit control of the first digital tube
SECOND EQU P2.6 ; The second digital tube position control
DISPBUFF EQU 5AH; Display buffer is 5AH and 5BH
ORG 0000H
AJMP START
ORG 30H
START:
MOV SP,#5FH ;Set up the stack
MOV P1,#0FFH
MOV P0,#0FFH
MOV P2, #0FFH ; Initialize, display, LED off
MOV DISPBUFF,#0 ;The first bit displays 0
MOV DISPBUFF+1,#1; The second hold displays 1
LOOP:
LCALL DISP ;Call display program
AJMP LOOP
; The main program ends here
DISP:
PUSH ACC; ACC is pushed onto the stack
PUSH PSW; PSW is pushed onto the stack
MOV A, DISPBUFF; Get the first number to be displayed
MOV DPTR,#DISPTAB ; font table first address
MOVC A,@A+DPTR; Get font code
MOV P0,A ; send the font code to P0 (segment port)
CLR FIRST; open the first display port
LCALL DELAY ; Delay 1 millisecond
SETB FIRST ; Turn off the first bit display (start preparing the second bit data)
MOV A,DISPBUFF+1; Get the second bit of the display buffer
MOV DPTR,#DISPTAB
MOVC A,@A+DPTR
MOV P0,A ; send the second font code to port P0
CLR SECOND ; Open the second display
LCALL DELAY ; Delay
SETB SECOND ; Turn off the second digit display
POP PSW
POP ACC
RET
DELAY: ; Delay 1 millisecond
PUSH PSW
SETB RS0
MOV R7,#50
D1: MOV R6,#10
D2: DJNZ R6,$
DJNZ R7,D1
POP PSW
RET
DISPTAB:DB 28H,7EH,0a4H,64H,72H,61H,21H,7CH,20H,60H
END
It can be seen from the above MCU routine that the dynamic scanning display must be continuously called by the CPU to ensure continuous display.
The above program can realize the display of numbers, but it is not very practical. Why? Here, only two numbers are displayed, and no other work is done. Therefore, the two digital tubes take turns to display for 1 millisecond, which is no problem. In actual work, of course, it is impossible to display only two numbers. Other things must be done. In this way, the time interval between the second call of the display program is uncertain. If the time interval is relatively long, the display will be discontinuous. In actual work, it is difficult to ensure that all work can be completed in a very short time. Moreover, this display program is a bit "wasteful". Each digital tube display takes 1 millisecond, which is not allowed in many combinations. What should we do? We can use the timer. When the timing time is reached, an interrupt is generated, a digital tube is lit, and then it returns immediately. This digital tube will be lit until the next timing time is reached, without calling the delay program. This time can be left for the main program to do other things. When the next timing time is reached, the next digital tube will be displayed, so there is little waste.
Counter EQU 59H ; Counter, through which the display program knows which digital tube is currently displayed
FIRST EQU P2.7 ; bit control of the first digital tube
SECOND EQU P2.6 ; The second digital tube position control
DISPBUFF EQU 5AH; Display buffer is 5AH and 5BH
ORG 0000H
AJMP START
ORG 000BH ;Entry of timer T0
AJMP DISP ;Display program
ORG 30H
START:
MOV SP,#5FH ;Set up the stack
MOV P1,#0FFH
MOV P0,#0FFH
MOV P2, #0FFH ; Initialize, display, LED off
MOV TMOD,#00000001B ; Timer T0 works in mode 1 (16-bit timing/counting mode)
MOV TH0,#HIGH(65536-2000)
MOV TL0,#LOW(65536-2000)
SETB TR0
SETB EA
SETB ET0
MOV Counter,#0 ; Counter initialization
MOV DISPBUFF,#0 ; The first bit always displays 0
MOV A,#0
LOOP:
MOV DISPBUFF+1,A ; The second digit displays 0-9 in turn
INC A
LCALL DELAY
CJNE A,#10,LOOP
MOV A,#0
AJMP LOOP; Any program can be arranged in the middle, this is just a demonstration.
; The main program ends here
DISP: ;Timer T0 interrupt response program
PUSH ACC; ACC is pushed onto the stack
PUSH PSW; PSW is pushed onto the stack
MOV TH0,#HIGH(65536-2000); The timing time is 2000 cycles, about 2170 microseconds (11.0592M)
MOV TL0,#LOW(65536-2000)
SETB FIRST
SETB SECOND ; Turn off display
MOV A,#DISPBUFF ; Display buffer first address
ADD A,Counter
MOV R0,A
MOV A,@R0; Get the corresponding display buffer value according to the counter value
MOV DPTR,#DISPTAB ; font table first address
MOVC A,@A+DPTR; Get font code
MOV P0,A ; send the font code to P0 (segment port)
MOV A,Counter; Get the value of the counter
JZ DISPFIRST ; if it is 0, display the first bit
CLR SECOND ; otherwise display the second digit
AJMP DISPNEXT
DISPFIRST:
CLR FIRST ; Display the first position
DISPNEXT:
INC Counter ;Counter plus 1
MOV A,Counter
DEC A ; If the counter reaches 2, set it back to 0
DEC A
JZ RST COUNT
AJMP DISPEXIT
RSTCOUNT:
MOV Counter,#0 ; The value of the counter can only be 0 or 1
DISPEXIT:
POP PSW
POP ACC
RETI
DELAY: ; Delay 130 milliseconds
PUSH PSW
SETB RS0
MOV R7,#255
D1: MOV R6,#255
D2: NOP
NOP
NOP
NOP
DJNZ R6,D2
DJNZ R7,D1
POP PSW
RET
DISPTAB:DB 28H,7EH,0a4H,64H,72H,61H,21H,7CH,20H,60H
END
From the above MCU program, we can see that the dynamic display is a little more complicated than the static display, but it is worth it. This program has a certain versatility. As long as the value of the port and the value of the counter are changed, more digits can be displayed. The following is a flowchart of the display program.
Previous article:51 MCU Tutorial from Scratch - 24 LED Digital Tube Static Display Interface and Programming
Next article:51 MCU Tutorial from Scratch—— 26 MCU Keyboard Interface Programming
Recommended ReadingLatest update time:2024-11-17 00:45
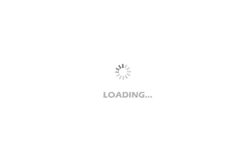
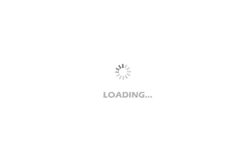
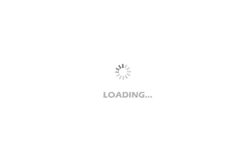
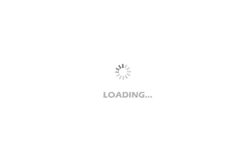
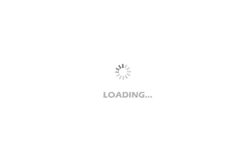
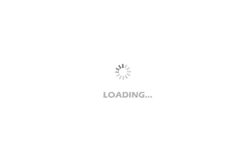
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- MicroPython Hands-on (04) - Basic Examples of Learning MicroPython from Scratch
- What is 802.11ah or HaLow?
- Recruiting senior RF engineers
- [Summary] STM32F107 board data and μC/OS routine software
- [Silicon Labs development kit review] + FreeRTOS + wonderful basic peripherals
- Ultra-low power Bluetooth controlled, cost-effective, dimmable smart lighting solution
- Open source popsicle macro key macropopsicle
- Homemade oscilloscope current probe developed successfully: measuring STM32 FOC motor board
- Altium to KiCad Tool
- GigaDevice GD32W51x 32-bit MCU Basic Instruction User Guide