Achievement purpose:
When a pin is configured as an input, learn how to program a pull-up resistor to simplify the hardware.
How to use software to control sampling frequency and time to achieve anti-interference purpose.
In order to make the program run more stably and prevent it from running away, learn how to use the watchdog.
Circuit and software principle description:
To simplify the code and circuit diagram, this experiment only uses two inputs and two outputs.
(Atmega8 can be expanded to support up to 11 D flip-flops by modifying the software). Under the supervision of the watchdog, Atmega8 periodically scans the sampling levels of PB0 and PB1. If the results of ten consecutive samples are the same, it is considered a valid sample. If one or more of the ten samples are different, it is considered an interference or critical state and will not be processed. This software implements the function of a D-type flip-flop latch: that is, each time the SW is pressed, the corresponding output will flip once.
In order to increase the versatility of the program and facilitate future performance testing or adjustments, the periodic scanning sampling cycle and the effective number of samplings in this program can be easily adjusted. (Just modify the sampling_times and sampling_interval values in the program. This program is defined as a valid input only when the level is the same after 20 scans. The interval between each scan is 50us).
Change the 2 in #define sapleing_way 2 to the number of ways you need, and the new number of ways will be automatically processed without modifying the code.
Question 1:
Why do we need to use sampling_times scans to sample? Only when the results of consecutive sampling_times samplings are consistent is it considered a valid input?
A: It is to increase the anti-interference ability and prevent the uncertainty caused by keyboard jitter when pressing. After the implementation is completed, you can set the sampling number to 1, and you will find that the operation of the D flip-flop will become unreliable.
Question 2:
Why use a watchdog?
A: In actual applications, many unknown situations often occur, which may cause the AVR chip to "run away", that is, the program will go wrong or even freeze. The chip must be reset to solve the problem. The watchdog actually resets the AVR chip regularly. Of course, when designing the program, it is important to place the watchdog instruction in the correct position to ensure that the program will not be reset during execution, and that the program will fall into an infinite loop and reset within the allowed time.

View the C code (the code has detailed comments):
/************************************************************
Experiment 4 (Second Edition): Use Atmega8 to implement the function of D-type trigger latch
Purpose: www.elecfans.com
1. When the pin is set as input, understand how to program the pull-up resistor.
2. How to use software to control the sampling frequency and time to achieve a certain anti-interference purpose
3. In order to make the program run more stable and prevent it from running away, how to use the watchdog?
By armok (2004-09-18) a13809260240@126.com
**************************************************************/
#include
#include
#define sapleing_way 2 //Define how many sampling channels. The maximum value is 8. PB is input and PD is output.
#define sampling_times 20 //Define the number of sampling times. The same sampling values for consecutive times are considered valid sampling.
#define sampling_interval 50 //Define the time interval for each sampling, in units of us.
typedef struct
{ unsigned int v_last; //The result of the last sampling_times sampling value
unsigned int v_current; //The result of the current sampling_times sampling value
unsigned int v[sampling_times]; //Store the continuous sampling values of sampling_times times
unsigned int v_temp; //Store the temporary value for comparison, valid when 1, invalid when 0
} inputStruct;
void delay_nus(unsigned int n); //Delay function, unit is us.
void watchdog_init(void); //Initialize watchdog function
void port_init(void); //Port initialization function
void main(void) //Main function
{
unsigned int i;
unsigned int j;
inputStruct pb_input[sapleing_way];
port_init(); //Initialize port
watchdog_init(); //Initialize watchdog
while (1)
{
//The following for loop stores the sampling results of consecutive sampling_times times in the corresponding array
for (i=0;i
delay_nus(sampling_interval); //Sampling once every sampling_interval
for(j=0;j
pb_input[j].v[i]=PINB&BIT(j);
}
}
//The following for loop determines whether the sampling results of consecutive sampling_times times are valid
for(j=0;j
for (i=1;i
if (pb_input[j].v[i-1]==pb_input[j].v[i]) //If the sampling results of sampling_times times are the same, it is considered valid
pb_input[j].v_temp=1; //The flag that sampling_times times are valid
else //Otherwise, it is discarded and no processing is performed.
{
pb_input[j].v_temp=0; //sampling_times times sampling is invalid, no processing
break;
}
}
//The following if judges the level of PB input, compares it with the result of the previous sampling calculation, and judges whether to flip the corresponding PD
if (pb_input[j].v_temp==1) //sampling_times times sampling is valid, make the following judgment
{
if (pb_input[j].v[0]==0) //The input is low level
pb_input[j].v_current=0;
else
pb_input[j].v_current=1; //The input is high level
if (pb_input[j].v_last==1 && pb_input[j].v_current==0) //If the first ten samples are high level and the current ten are low level, it is regarded as a valid action and the output is executed
PORTD^=BIT(j); //Flip the corresponding PD bit
pb_input[j].v_last=pb_input[j].v_current; //Pass the current result to the previous result and prepare for the next processing
}
} //end for
WDR(); //Clear the watchdog count
} //end while
} // end main()
void delay_nus(unsigned int n)//n microsecond delay function
{
unsigned int i;
for (i=0;i
asm("nop");
}
}
void port_init(void)
{
DDRB=0x00; //Set PB0-7 as input
PORTB=0xFF; //Works with the next sentence
SFIOR&=~BIT(2); //Set the PDU pull-up resistor of SFIOR to be valid. Works with the previous sentence.
DDRD=0xFF; //PD0-7 is output
}
void watchdog_init(void)
{
WDR(); //Watchdog count cleared
WDTCR=0x0F; //Enable watchdog, and use 2048K frequency division, typical overflow time 2.1S at 5V
}
Previous article:1602b LCD display usage example
Next article:Design of multi-chip microcomputer DC power supply control board
Recommended ReadingLatest update time:2024-11-17 01:40
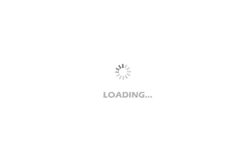
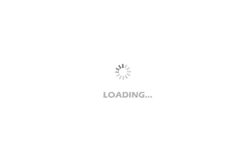
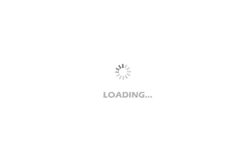
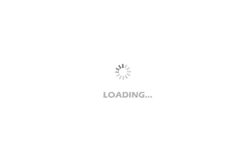
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- Why can't the P0 port of STC8A8K32S4A12 output a high level?
- What other product parameters and characteristics do LED driver chip capacitors have?
- Decomposition
- Serial communication between CC2541 and MSP430
- c6678 sys/bios add hwi interrupt does not trigger, send successfully in bare metal case
- NUCLEO_G431RB review->File structure & ST-Link online debugging experience
- Sugar Glider ⑤ Sugar Glider Health Monitoring System Based on RSL10--Project Introduction
- Problems with using the bq28z610EVM development board
- How to create a transcoding table using HZK16 font in micropython?
- 【TI recommended course】#What is I2C design tool? #