While NI LabVIEW software has long helped engineers and scientists quickly develop functional measurement and control applications, not all new users follow LabVIEW programming best practices. LabVIEW graphical programming is unique because it is immediately apparent whether a user is following coding best practices by just looking at their application. Some users make these mistakes because they do not truly understand the principles behind the data flow of a LabVIEW block diagram, while others simply do not know which features improve the quality of their LabVIEW programming.
This article describes some of the most common programming mistakes made by inexperienced LabVIEW programmers and provides suggestions for adopting correct LabVIEW programming methods.
Figure 1. A typical LabVIEW novice's masterpiece
Overuse of Flat Sequence Structures
Many people who are new to LabVIEW do not fully understand the concepts behind "data flow" execution, which is the foundation of LabVIEW programming. One of the manifestations is that users tend to overuse Flat Sequence structures in the block diagram. Users often rely on Flat Sequence structures to implement the serial execution of code in the block diagram instead of using data flow and wires between nodes.
Figure 2. Users often over-rely on flat sequence structures without fully understanding dataflow programming concepts.
Dataflow programming means that nodes (subVIs, primitives, structures, etc.) on the block diagram will only start executing when all required data inputs arrive. This is very useful for programmers using LabVIEW because independent processes can run in parallel by themselves, while imperative languages require additional settings to implement parallel execution. As computer CPUs continue to increase, LabVIEW can automatically decompose parallel processes and improve code performance without the user having to write any additional code. Forcibly using flat sequential structures to execute program flowcharts will not only limit parallel execution, but also lose this advantage. Limiting unnecessary structures in the program flowchart can help improve overall readability and maintain a more concise flowchart.
Error wires are an efficient way to implement data flow on a block diagram without relying on Flat Sequence structures and can help implement error handling strategies.
When should I use a Flat Sequence structure?
Executing a block diagram with a Flat Sequence structure can help benchmark the performance of your code. By using a Sequence structure with a tick counter inside the frame, you can determine the time taken for code execution between two tick counters. This is not possible with normal dataflow execution.
Figure 3. The Flat Sequence structure and Tick Counter VI can help you benchmark your code.
For more information about dataflow programming, visit the online self-paced training (ni.com/self-paced-training) for the LabVIEW Core Course 1 course, Dataflow. The online self-paced training is available at no charge to customers who have purchased LabVIEW or have a membership to the Standard Service Program (ni.com/SSP).
Incorrect use of local variables
Another common mistake in LabVIEW programming is overusing local variables. A local variable is an area in shared memory that is used to pass data between different parts of a computer program. Local variables are commonly used in text-based programming languages and are very powerful, but they can cause problems if race conditions occur.
For other programming languages, passing data through variables is necessary, while LabVIEW provides a data flow method to move data from one part of the program to another. The inherent parallelism mechanism of LabVIEW determines that users cannot overuse variables because there are usually multiple programs in different locations accessing shared memory at the same time. If variables are overused, a read/write operation will win the "competition" while other operations will lose the "competition", and the operation that loses data will be ignored, so overusing variables in LabVIEW may eventually lead to data loss.
You can safely pass data from one part of a LabVIEW program to another through a variety of methods, including wires, queues, events, notifications, function global variables, and more. Each mechanism is designed for a specific situation, but all have the ability to eliminate race conditions.
For more information about correctly moving data within a LabVIEW program, refer to the online self-paced training at ni.com/self-paced-training for the course “Local Variables” in LabVIEW Core Course 1 and the course “Notifications, Queues, and Events” in LabVIEW Core Course 2.
Ignoring code modularity
Often, new LabVIEW users create "fire and forget" applications to accomplish simple tasks without considering whether the code will be needed in the future. As the programming workload grows, they find themselves rewriting the same code over and over again. If you create a modular subVI that can be reused in other applications while you are programming, you can save a lot of development time.
If you know that a particular section of code will be reused in the same application, or feel that it may be used in future applications, you should take a moment to turn that section of code into a subVI. To make a section of code a subVI, the main things you need to do are add a document, use Terminals, and disable certain VI properties. One of the easiest ways to create a subVI is to highlight a section of code on the block diagram and select Edit >> Create SubVI from the menu bar. This will place the section of code into a separate VI and use Terminals. You will still need to add a description to the icon, add documentation to the block diagram and VI properties, and disable certain VI settings, but Edit >> Create SubVI will give you a good idea of the modularity of your code.
Figure 4. Using the correct approach to modularizing your LabVIEW code can help you save a lot of development time.
One setting that must be turned off when modularizing code is "Allow debugging." You can find this option in the "Execution" category under "VI Properties (File>>VI Properties)." When the code is fully functional and no longer requires debugging features such as execution highlighting, turn off "Allow debugging" in the execution settings and run the VI again. The benefits of this are that the application may run faster due to optimizations performed during the compilation process, and the physical space occupied by the VI on disk is also reduced because the code that enables debugging is turned off.
For more information about code modularization, go to the self-paced online training course "Understanding Modularity" in the LabVIEW Core Course 1 course.
Creating large and cumbersome flowcharts
Many new LabVIEW users will write very cumbersome and large program flowcharts. For some complex applications, we inevitably need to write large program flowcharts, but large program flowcharts can also indicate that the program lacks programming architecture to a certain extent. Without a basic architecture, it is very difficult to maintain the program in the long term, and it will also be very difficult to add new features in the future. Just as only a good framework can build a well-structured house, a good programming architecture can provide a safe and reliable framework for you to build applications.
Almost all programmers will find common frameworks for software architecture useful. In fact, many of the architectures in LabVIEW, such as producer/consumer and state machines, are very similar to those in other programming languages.
Understanding the LabVIEW architecture can reduce development time and increase the scalability of your application. LabVIEW 2012 includes templates and sample projects that make it easier to understand the architecture. Templates explain different architectures and applications. Sample projects are larger applications based on templates that demonstrate how to use the templates to solve real-world challenges. You can add hardware to the sample project or use the sample project as a complete application if you want, and the sample projects are open and well documented so you can customize them for your specific application.
Not paying attention to documentation
Good code documentation is a great way to help others understand your program. Unfortunately, many programmers don't start documenting until the end of the development cycle, after the feature is developed. This leaves very little time to document the code. The right thing to do is to take time to start documenting during the development process. Documentation is also very useful for programmers themselves, especially when they come back to the code after a while and can't remember why they chose certain code in the first place. Programmers often stay up late programming while drinking coffee, which often leads to "temporary amnesia". Documentation can help programmers recall.
In general, the graphical nature of LabVIEW makes it easier to read a program than a text-based program, but effective documentation can reduce the time required to "decode" the program. The easiest way to add documentation comments to a block diagram is to use free labels. You can add comments by double-clicking the left mouse button in a blank area of the block diagram and typing text. Then, use arrow markers to point to the specific code that the free label refers to. If you need to add pictures, you can copy them to the clipboard and paste them into the block diagram. Both pictures of physical systems and mathematical formulas can help to clearly illustrate the context of the code within the block diagram.
Figure 6. Properly architected and well-documented code not only helps others understand your code, it also helps you understand your own code better.
Documenting your code is not just for reusable libraries, it should be for every program. When one person needs to explain something to others, they will have a deeper understanding of the topic. Documentation essentially forces programmers to explain, helping them understand their code better.
Previous article:My LABVIEW rapid development of serial port test software example
Next article:Building the next generation of software-centric automated testing systems
Recommended ReadingLatest update time:2024-11-23 11:17
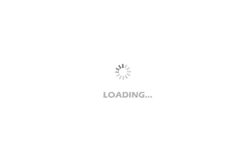
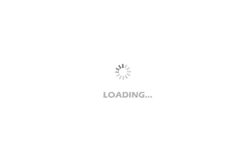
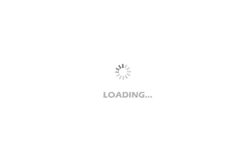
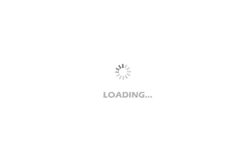
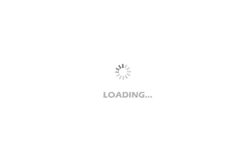
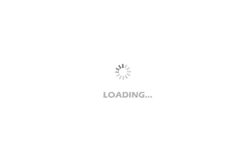
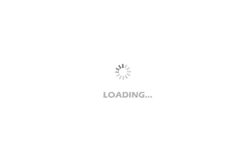
- Popular Resources
- Popular amplifiers
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Arduino Nano collects temperature and humidity data through LabVIEW and DHT11
-
Modern Testing Technology and System Integration (Liu Junhua)
-
Computer Control System Analysis, Design and Implementation Technology (Edited by Li Dongsheng, Zhu Wenxing, Gao Rui)
- New IsoVu™ Isolated Current Probes: Bringing a New Dimension to Current Measurements
- Modern manufacturing strategies drive continuous improvement in ICT online testing
- Methods for Correlation of Contact and Non-Contact Measurements
- Keysight Technologies Helps Samsung Electronics Successfully Validate FiRa® 2.0 Safe Distance Measurement Test Case
- From probes to power supplies, Tektronix is leading the way in comprehensive innovation in power electronics testing
- Seizing the Opportunities in the Chinese Application Market: NI's Challenges and Answers
- Tektronix Launches Breakthrough Power Measurement Tools to Accelerate Innovation as Global Electrification Accelerates
- Not all oscilloscopes are created equal: Why ADCs and low noise floor matter
- Enable TekHSI high-speed interface function to accelerate the remote transmission of waveform data
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- 【AT-START-F425 Review】+u8g2 transplant test
- PCB Design Software Bluetooth Speaker Practice│Physical Rules and Spacing Rules
- Real-time operating system uclinux kernel development manual
- The Definitive Guide to Arduino Robotics
- Electronics Engineer Quick-Start Resources, Super Practical, Suitable for Beginners
- Are those who have seen this almost 40 years old?
- Philips, Nokia, China Mobile and EZ-Card jointly deploy China's first NFC trial in Xiamen
- IPC-6013E-EN_2021 Qualification and Performance Specification or FlexibleRigi...
- TI DSP FFT library functions
- Share a rolling door controller made with CC2541