Top 10 filtering algorithms commonly used in MCU ADC
1. Limiting Filter
1. Methods
-
Determine the maximum deviation value A allowed for two samples based on experience
-
Each time a new value is adopted, the following judgment is made: if the difference between the current value and the previous value is <= A, then this time is valid; if the difference between the current value and the previous value is > A, then this time is invalid and the previous value is used to replace this time.
2. Advantages and Disadvantages
-
Overcome pulse interference, cannot suppress periodic interference, and has poor smoothness.
3. Code
/* A值根据实际调,Value有效值,new_Value当前采样值,程序返回有效的实际值 */
#define A 10
char Value;
char filter()
{
char new_Value;
new_Value = get_ad(); //获取采样值
if( abs(new_Value - Value) > A) return Value; //abs()取绝对值函数
return new_Value;
}
2. Median Filtering
1. Methods
-
Continuously sample N times and arrange by size
-
Take the middle value as the effective value of this time
2. Advantages and Disadvantages
-
It overcomes fluctuation interference and has a good filtering effect on slowly changing measured parameters such as temperature, but is not suitable for rapidly changing parameters such as speed.
3. Code
#define N 11
char filter()
{
char value_buf[N];
char count,i,j,temp;
for(count = 0;count < N;count++) //获取采样值
{
value_buf[count] = get_ad();
delay();
}
for(j = 0;j<(N-1);j++)
for(i = 0;i<(n-j);i++)
if(value_buf[i]>value_buf[i+1])
{
temp = value_buf[i];
value_buf[i] = value_buf[i+1];
value_buf[i+1] = temp;
}
return value_buf[(N-1)/2];
}
3. Arithmetic average filtering
1. Methods
-
Continuously sample N times and take the average
-
When N is large, the smoothness is high and the sensitivity is low.
-
When N is small, the smoothness is low and the sensitivity is high
-
Usually N=12
2. Advantages and Disadvantages
-
It is suitable for systems with random interference, occupies more RAM and has slow speed.
3. Code
#define N 12
char filter()
{
int sum = 0;
for(count = 0;count<N;count++)
sum += get_ad();
return (char)(sum/N);
}
4. Recursive Average Filtering
1. Methods
-
Take N sample values to form a queue, first in first out
-
Take the mean
-
Generally N=4~12
2. Advantages and Disadvantages
-
Good suppression of periodic interference and high smoothness
-
Suitable for high frequency vibration systems
-
Low sensitivity, large RAM usage, severe pulse interference
3. Code
/* A值根据实际调,Value有效值,new_Value当前采样值,程序返回有效的实际值 */
#define A 10
char Value;
char filter()
{
char new_Value;
new_Value = get_ad(); //获取采样值
if( abs(new_Value - Value) > A) return Value; //abs()取绝对值函数
return new_Value;
}
5. Median Average Filter
1. Methods
-
Sample N values and remove the largest and smallest values.
-
Calculate the average value of N-2
-
N = 3~14
2. Advantages and Disadvantages
-
Combines the advantages of median and average
-
Eliminate pulse interference
-
Slow calculation speed and large RAM usage
3. Code
char filter()
{
char count,i,j;
char Value_buf[N];
int sum=0;
for(count=0;count<N;count++)
Value_buf[count]= get_ad();
for(j=0;j<(N-1);j++)
for(i=0;i<(N-j);i++)
if(Value_buf[i]>Value_buf[i+1])
{
temp = Value_buf[i];
Value_buf[i]= Value_buf[i+1];
Value_buf[i+1]=temp;
}
for(count =1;count<N-1;count++)
sum += Value_buf[count];
return (char)(sum/(N-2));
}
6. Limiting average filtering
1. Methods
-
Each sampling data is limited before being sent to the queue
-
take the average
2. Advantages and Disadvantages
-
Combining the advantages of limiting, averaging, and queuing
-
Eliminate pulse interference, occupy more RAM
3. Code
#define A 10
#define N 12
char value,i=0;
char value_buf[N];
char filter()
{
char new_value,sum=0;
new_value=get_ad();
if(Abs(new_value-value)<A)
value_buf[i++]=new_value;
if(i==N)i=0;
for(count =0 ;count<N;count++)
sum+=value_buf[count];
return (char)(sum/N);
}
7. First-order lag filtering
1. Methods
-
Take a=0~1
-
This filtering result = (1-a) * this sampling + a * last result
2. Advantages and Disadvantages
-
Good at periodic interference, suitable for occasions with high fluctuation frequency
-
Low sensitivity, phase lag
3. Code
/*为加快程序处理速度,取a=0~100*/
#define a 30
char value;
char filter()
{
char new_value;
new_value=get_ad();
return ((100-a)*value + a*new_value);
}
8. Weighted Recursive Average Filtering
1. Methods
-
An improvement on the recursive average filter, where different weights are given to data at different times. Usually, the newer the data, the greater the weight. This results in high sensitivity but low smoothness.
2. Advantages and Disadvantages
-
It is suitable for systems with large lag time constants and short sampling periods. It cannot quickly respond to interference with signals with small lag time constants, long sampling periods, and slow changes.
3. Code
/* coe数组为加权系数表 */
#define N 12
char code coe[N]={1,2,3,4,5,6,7,8,9,10,11,12};
char code sum_coe={1+2+3+4+5+6+7+8+9+10+11+12};
char filter()
{
char count;
char value_buf[N];
int sum=0;
for(count=0;count<N;count++)
{
value_buf[count]=get_ad();
}
for(count=0;count<N;count++)
sum+=value_buf[count]*coe[count];
return (char)(sum/sum_coe);
}
9. Anti-jitter filtering
1. Methods
-
Set a filter counter
-
Compare the sampled value with the current effective value
-
If the sample value = the current effective value, the counter is cleared to 0
-
If the sampled value is not equal to the current valid value, the counter is incremented by 1.
-
If the counter overflows, the sampled value replaces the current valid value and the counter is cleared to 0.
2. Advantages and Disadvantages
-
The filtering effect is good for slowly changing signals, but not so good for fast changing signals.
-
Avoid jumping near the critical value. If the interference value is sampled when the counter overflows, filtering cannot be performed.
3. Code
#define N 12
char filter()
{
char count=0,new_value;
new_value=get_ad();
while(value!=new_value)
{
count++;
if(count>=N) return new_value;
new_value=get_ad();
}
return value;
}
10. Limiting and de-jittering filtering
1. Methods
-
Limit first, then debounce
2. Advantages and Disadvantages
-
Combines the advantages of limiting and de-jittering
-
Avoid introducing interference values, and do not use them for fast-changing signals.
3. Code
#define A 10
#define N 12
char value;
char filter()
{
char new_value,count=0;
new_value=get_ad();
while(value!=new_value)
{
if(Abs(value-new_value)<A)
{
count++;
if(count>=N) return new_value;
new_value=get_ad();
}
return value;
}
}
Recommended Reading
Welcome to join the Automotive Electronics WeChat Exchange Group , and have in-depth exchanges with Automotive Industry Engineering, accumulate technical experience, improve technical level, and understand the latest technological progress in the industry.
Reply to any content you want to search in the official , such as problem keywords, technical terms, bug codes, etc., and you can easily get relevant professional technical content feedback . Go and try it!
If you want to see our articles more often, you can go to our homepage, click the "three dots" in the upper right corner of the screen, and click "Set as Star".

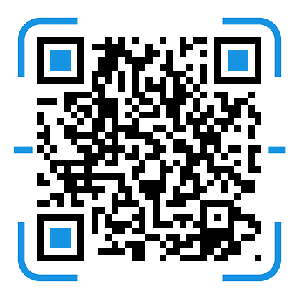
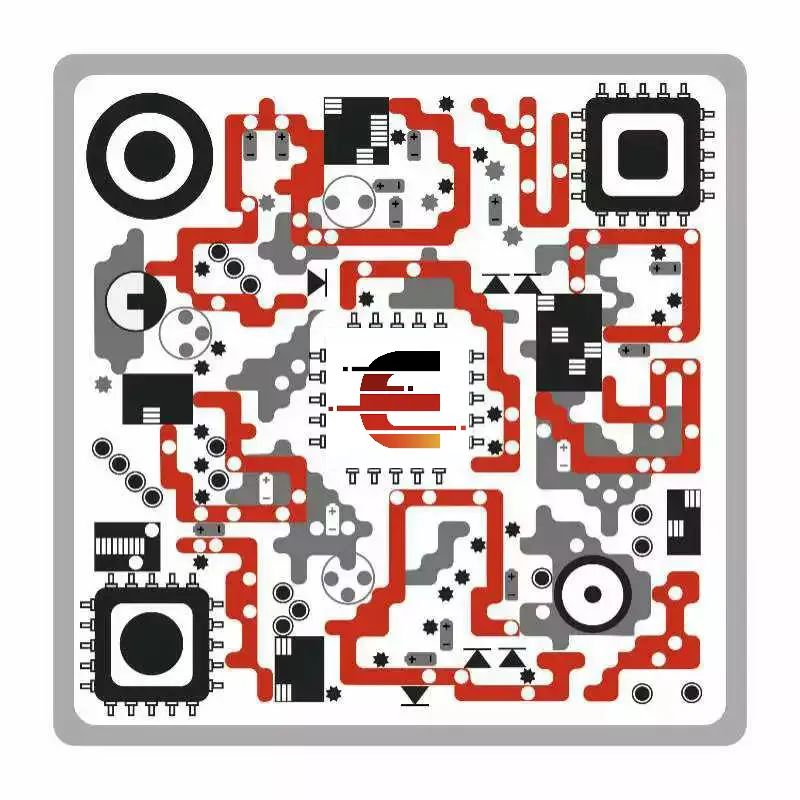
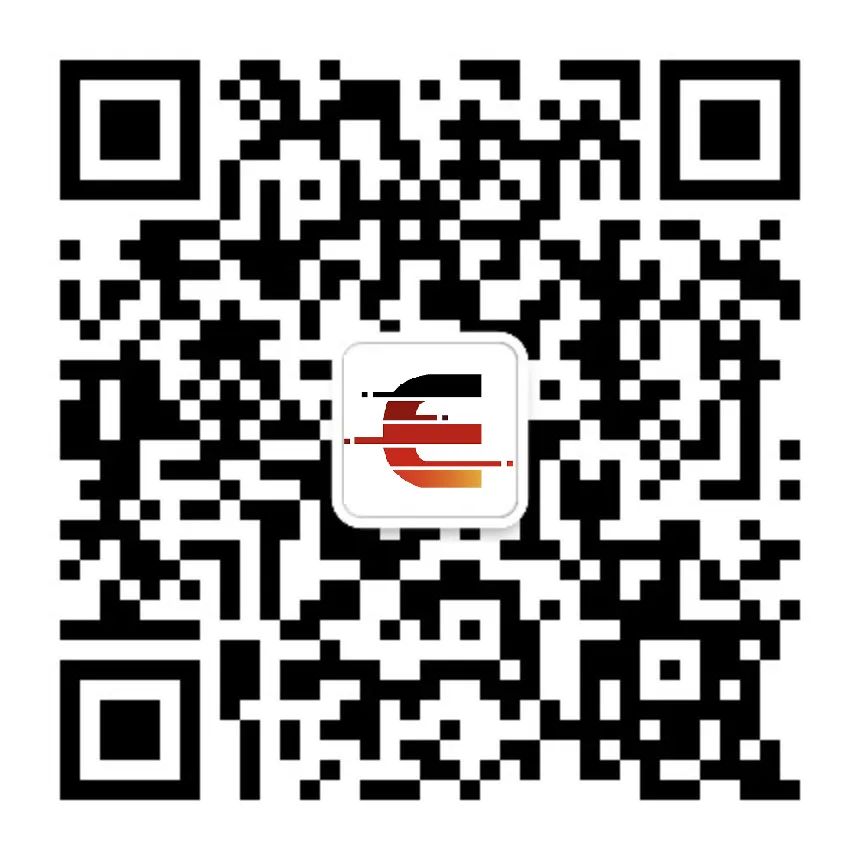