Controlling Hardware with Python - Low Voltage Startup and Reliability Testing
[Copy link]
Reprinted from: "Using Python to control hardware 46-low voltage startup and reliability testing"
During the product development phase, it is sometimes necessary to understand whether the device can still start and work normally when the power supply is low voltage. The following test system can be built:
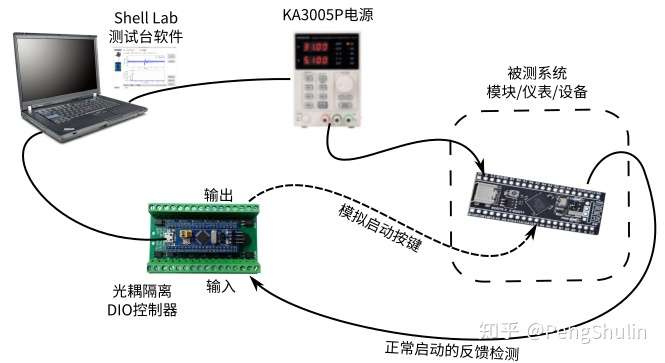
1. Control the programmable power supply to output the measured voltage.
2. Use an optocoupler to isolate the digital IO board and detect the power-on feedback of the system under test. For example, the four experimental boards introduced earlier will have the LED lights flashing at a frequency of 0.5Hz after startup. The control PIN of the LED can be connected to the optocoupler input.
3. If the system under test requires an additional start switch button, the optocoupler output can be connected to the button signal line to simulate the start-up action. If the system is special and needs to press a special function key to detect feedback, this method can also be used.
4. Use Shell Lab software scripts to control the entire test process, or directly write Python scripts under the command line.
The DIO controller in the figure is composed of the simple experiment board + baseboard introduced earlier, which can control 8 optocoupler inputs and 8 optocoupler outputs.
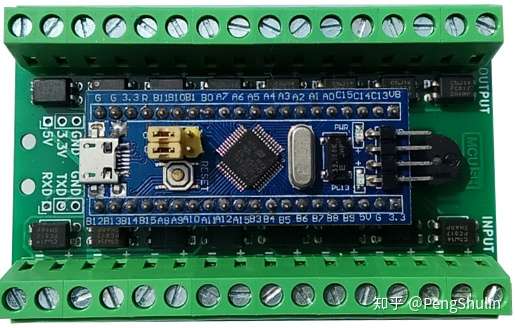
Take the experimental board as an example and test it in the above way:
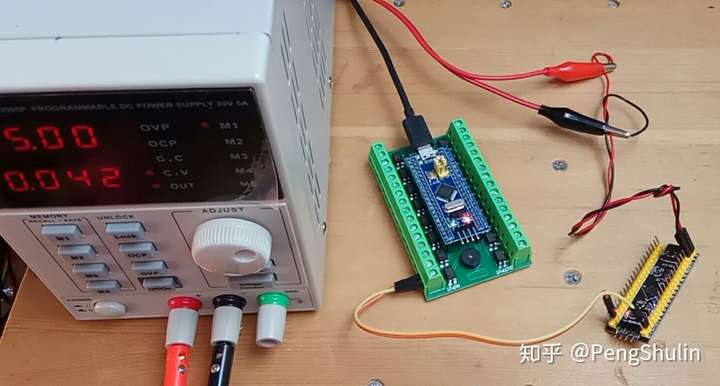
The following code outputs the normal working voltage, switches on and off repeatedly, and detects whether the experimental board can start normally:
VOLTAGE = 5.0 # Volt
CURRENT = 5.0 # Amp
DETECT_PIN = '1.8' # PB8 to detect LED feedback
DETECT_TIMEOUT = 5 # 检测超时 in seconds
DETECT_DEBOUNCE = 0.4 # 去抖动 in seconds
dio = Mcush.Mcush('/dev/ttyACM1')
dio.pinInput(DETECT_PIN)
power = Korad.KA3005P(PORT)
power.output( VOLTAGE, CURRENT )
v, a = power.getSetting()
info( 'Set: %.2f V / %.2f A'% (v, a) )
counter, counter_succ, counter_err = 0, 0, 0
while True:
# power off
power.outputDisable()
time.sleep(0.5) # wait for voltage drop down
# power on
power.outputEnable()
# detect with timeout and debounce
detected = False
t0 = time.time()
while time.time() < t0 + DETECT_TIMEOUT:
t1 = time.time()
while True:
pin = not dio.pinRead(DETECT_PIN)
if pin:
if time.time() > t0 + DETECT_DEBOUNCE:
detected = True
else:
break
if detected:
break
if detected:
counter_succ += 1
else:
counter_err += 1
counter += 1
info( 'Count: %d Succ: %d Err: %d'% (counter,
counter_succ, counter_err) )
The test status count is shown at the bottom:
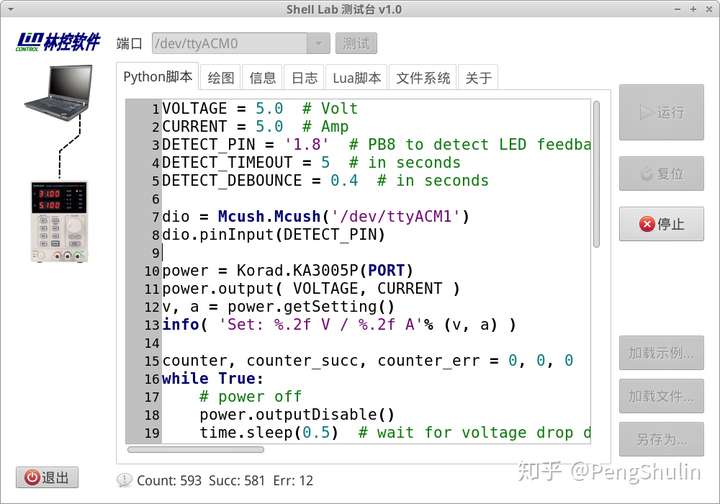
The following script outputs a voltage that increases linearly from low to high to monitor whether it can start working:
VOLTAGE_MIN = 1.0 # Volt
VOLTAGE_MAX = 5.0 # Volt
VOLTAGE_STEP = 20 # steps number
CURRENT = 5.0 # Amp
DETECT_PIN = '1.8' # PB8 to detect LED feedback
DETECT_TIMEOUT = 5 # in seconds
DETECT_DEBOUNCE = 0.4 # in seconds
dio = Mcush.Mcush('/dev/ttyACM1')
dio.pinInput(DETECT_PIN)
power = Korad.KA3005P(PORT)
power.outputDisable()
p = getPlotPanel()
p.addPlot( 'result', 111, label_y='Succ', label_x='Voltage' )
p.setLimit( 'result', top=1.1, left=VOLTAGE_MIN, right=VOLTAGE_MAX, auto=False )
p.setLinestyle( 'result', ['-o'] )
volt_list = linspace(VOLTAGE_MIN, VOLTAGE_MAX, num=VOLTAGE_STEP)
for volt in volt_list:
# output voltage
power.output( volt, CURRENT )
v, a = power.getSetting()
info( 'Set: %.2f V / %.2f A'% (v, a) )
# detect with timeout and debounce
detected = False
t0 = time.time()
while time.time() < t0 + DETECT_TIMEOUT:
t1 = time.time()
while True:
pin = not dio.pinRead(DETECT_PIN)
if pin:
if time.time() > t0 + DETECT_DEBOUNCE:
detected = True
else:
break
if detected:
break
# 绘图输出结果,1-正常 0-异常
p.addData( 'result', int(detected), volt )
# power off
power.outputDisable()
time.sleep(0.5) # wait for voltage drop down
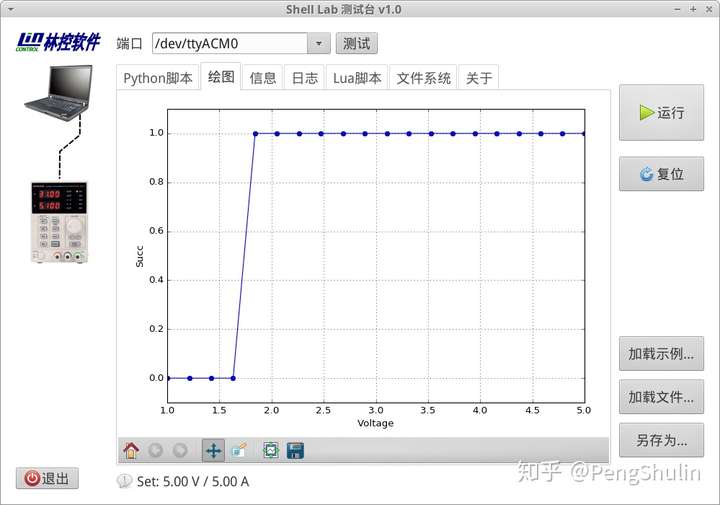
Narrow the range to 1.5V~2.0V and refine it:
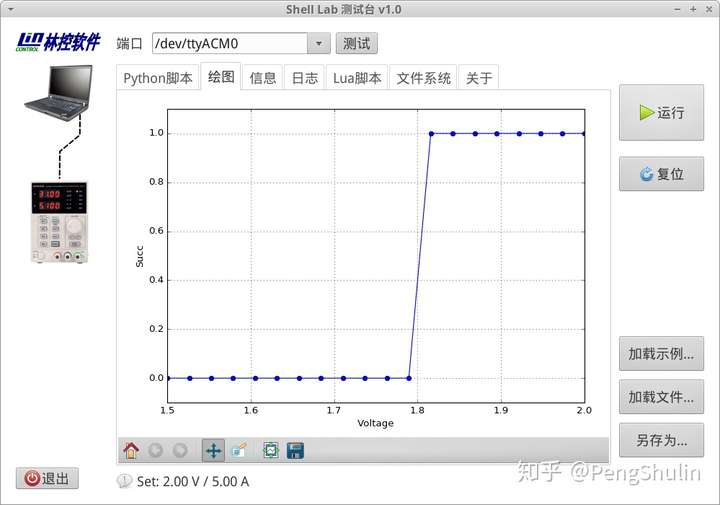
It can be clearly seen that the board cannot start below 1.8V.
This experiment is just for demonstration (the experimental board is usually powered by a stable 5V), and it is more practical in testing battery-powered handheld devices.
|