A potentiometer is a mechanical device that allows you to set the resistance to the desired value, thereby varying the current passing through it. Potentiometers have many applications, but most often they are used as volume controllers in audio amplifiers.
The potentiometer does not control the gain of the signal but it forms a voltage divider and that is why the input signal is attenuated. So in this project, I will show you how to build your digital volume controller using IC PT2258 and interface it with Arduino to control the volume of an amplifier circuit.
Integrated Circuit PT2258
As I mentioned before, the PT2258 is an IC used as a 6-channel electronic volume controller using CMOS technology designed for multi-channel audio-video applications.
The IC provides an I2C control interface, an attenuation range of 0 to -79dB in 1dB/step, and is available in a 20-pin DIP or SOP package.
Some basic features include,
6 input and output channels (suitable for 5.1 home audio systems)
Selectable I2C address (for daisy-chain applications)
High channel separation (for low noise applications)
Signal-to-noise ratio> 100dB
Operating voltage: 5 to 9V
How the PT2258 IC works
This IC sends and receives data from the microcontroller through the SCL and SDA lines. SDA and SCL form the bus interface. These lines must be pulled high through two 4.7K resistors to ensure stable operation.
Before we get into the actual hardware operation, here is a detailed functional description of the IC. If you don’t want to know all this, you can skip this part as all the functional parts are managed by the Arduino library.
Data Validation
When the SCL signal is HIGH, the data on the SDA line is considered stable.
The HIGH and LOW states of the SDA line change only when SCL is LOW.
Start and Stop Conditions
When the start condition is activated
SCL is set to HIGH and
SDA changes from HIGH state to LOW state.
When the stop condition is activated
SCL is set to HIGH and
SDA transitions from low state to high state
NOTE! This information is very useful for debugging signals.
Data Format
Each byte transmitted to the SDA line consists of 8 bits to form a byte. Each byte must be followed by an acknowledge bit.
Acknowledgements
Acknowledgement ensures stable and correct operation. During the acknowledgement clock pulse, the microcontroller pulls the SDA pin high, while the peripheral device (audio processor) pulls the SDA line low (LOW).
The peripheral device (PT2258) is now addressed and it must generate an acknowledge after receiving a byte, otherwise the SDA line will remain high during the ninth (9th) clock pulse. If this happens, the master transmitter will generate a STOP message to abort the transmission.
This eliminates the need for efficient data transfer.
Address Selection
The I2C address of this IC depends on the status of CODE1 (Pin No.17) and CODE2 (Pin No.4).
Logic High = 1
Logic Low = 0
Interface Protocol
The interface protocol consists of the following parts:
A start bit
One chip address byte
ACK = Acknowledge bit
One data byte
Stop bits
After the IC is powered on, it needs to wait for at least 200ms before transmitting the first data bit, otherwise data transmission may fail.
After the delay, the first thing to do is to send "0XC0" over the I2C lines to clear the registers, which ensures normal operation.
The above steps cleared the entire register, now we need to set a value to the register, otherwise, the register stores garbage values and we get a freakish output.
To ensure proper volume adjustment, multiples of 10dB and 1dB codes need to be sent to the attenuator in sequence, otherwise the IC will behave abnormally. The following figure illustrates this more clearly.
Both of the above methods work fine.
To ensure normal operation, please ensure that the I2C data transmission speed does not exceed 100KHz.
This is how you can transmit a byte to the IC and attenuate the incoming signal. The above part is to learn the functionality of the IC but as I said before we will use the Arduino library to communicate with the IC which will manage all the hard code and we just need to make a few function calls.
Schematic
The figure above shows the tested schematic of the PT2258 based volume control circuit. It was taken from the datasheet and modified as required.
For demonstration purposes, the circuit was constructed on a solderless breadboard with the help of the schematic shown above.
Required Components
PT2258 Integrated Circuit – 1
Arduino Nano Controller – 1
Universal Breadboard - 1
Screw Terminal 5mm x 3 – 1
Button – 1
4.7K resistor, 5% - 2
150K resistor, 5% - 4
10k resistor, 5% - 2
10uF capacitor – 6
0.1uF capacitor – 1
Jumper Wires - 10
Arduino Code
#include
#include
#include
Next, open the PT2258.cpp file using your favorite text editor, I used Notepad++.
You can see that the "w" in the wire library is a lowercase letter, which is not compatible with the latest Arduino version. It needs to be replaced with an uppercase "W". That's it.
The complete code for the PT2258 volume controller can be found at the end of this section. The important parts of the program are explained here.
We start the code by including all the necessary library files. The Wire library is used to communicate between the Arduino and the PT2258. The PT2258 library contains all the critical I2C timing information and acknowledgements. The ezButton library is used to interact with the button.
Instead of using the code image below, copy all the code instances from the code file and format them as we have done before in other projects.
#include
#include
#include
Next, make objects for the two buttons and the PT2258 library itself.
PT2258 PT2258;
ezButton button_1(2);
ezButton button_2(4);
Next, define the volume level. This is the default volume level when the IC starts up.
intVolume = 40;
Next, start the UART and set the clock frequency of the I2C bus.
SerialNumber.Start(9600);
Wire.setClock(100000);
It is very important to set the I2C clock otherwise the IC will not work as the maximum clock frequency supported by this IC is 100KHz.
Next, we do some housekeeping using if else statements to ensure that the IC is communicating correctly with the I2C bus.
if (!pt2258.init())
Serial.printIn("PT2258 started successfully");
Other
Serial.printIn("Failed to start PT2258");
Next, we set the debounce delay for the button.
Button_1.setDebounceTime(50);
Button_2.setDebounceTime(50);
Finally, start up the PT2258 IC by setting the default channel volume and pin number.
/* Start PT with default volume and Pin*/
Pt2258.setChannelVolume(volume,4);
Pt2258.setChannelVolume(volume,5);
This marks the end of the Void Setup() section.
In the loop section, we need to call the loop function from the button class; this is the library's specification.
Button_1.loop(); //Library specification
Button_2.loop(); //Library specification
The if part below is to reduce the volume.
/* If the condition is true, then button 1 is pressed */
if (button_1.ispressed())
{
Volume++; //Increase volume counter.
// This if statement ensures that the transaction amount does not exceed 79
if (volume >= 79)
{
Volume = 79;
}
Serial.print("Volume: "); // Print volume level
Serial.printIn(volume);
/* Set the volume of channel 4
In PIN 9 of PT2558 IC
*/
Pt2558.setChannelVolume(volume,4);
/* Set the volume of channel 5
Which is PIN 10 of PT2558 IC
*/
Pt2558.setChannelVolume(volume,5);
}
The if part below is to increase the volume.
// The same goes for button 2
if (button_2.isPressed())
{
Volume - ;
// This if statement ensures that the volume does not drop below zero.
if (volume <= 0)
volume = 0;
Serial.print("Volume: ");
Serial.printIn(volume);
Pt2258.setChannelVolume(volume,4);
Pt2558.setChannelVolume(volume,5);
}
Testing Digital Audio Volume Control Circuits
To test the circuit the following equipment was used
Transformer with 13-0-13 tapping
2 4Ω 20W speakers as load.
Audio source (telephone)
I messed around with the mechanical potentiometer and shorted the two leads with two small jumper cables. Now with the help of two buttons I can control the volume of the amplifier.
#include
#include
#include
PT2258 PT2258; //PT2258 object
ezButton button_1(2); //Button_1 object
ezButton button_2(4); //Button_2 object
integer volume = 40; //Default volume / starting volume
void setup() {
Serial.start(9600); //UART start
Wire.setClock(100000); //Set I2C clock to 100KHz
/* Check
if
MCU
can talk to PT*/
if (!pt2258.init())
Serial.println("PT2258 started successfully");
else
Serial.println("Starting PT2258 failed");
/* Set button debounce delay*/
button_1.setDebounceTime(50);
button_2.setDebounceTime(50);
/* Start PT with default volume and Pin*/
pt2258.setChannelVolume(volume, 4);
pt2258.setChannelVolume(volume, 5);
}
void loop() {
button_1.loop(); //Library Specification
button_2.loop(); //Library Specification
/* If condition is true, then button 1 is pressed */
if (button_1.isPressed())
{
volume++; //Increment volume counter.
// This if statement ensures that the volume does not exceed 79
if (volume >= 79)
{
volume = 79;
}
Serial.print("Volume: "); // Print volume level
Serial.println(volume);
/*Set the volume of channel 4 Which
is pin 9 of the PT2258 IC
*/
pt2258.setChannelVolume(volume, 4);
/*Set the volume of channel 5
Which is pin 10 of the PT2258 IC
*/
pt2258.setChannelVolume(volume, 5);
}
//Same for button 2
if (button_2.isPressed())
{
volume - ;
// This if statement ensures that the volume does not go below zero.
if (volume <= 0)
volume = 0;
Serial.print("Volume: ");
Serial.println(volume);
pt2258.setChannelVolume(volume, 4);
pt2258.setChannelVolume(volume, 5);
}
}
Previous article:How to control the speed of an AC fan using PWM generated by Arduino
Next article:Tutorial on Designing Active Tone Control Circuits
Recommended ReadingLatest update time:2024-11-16 10:32
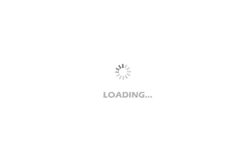
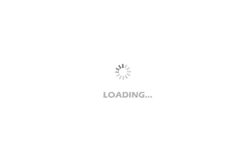
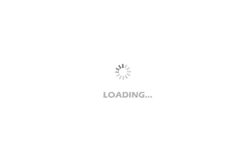
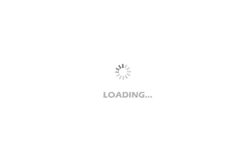
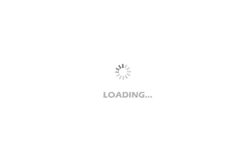
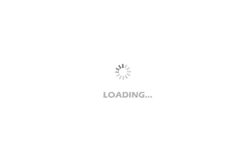
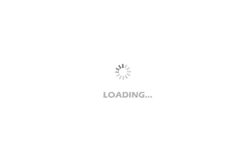
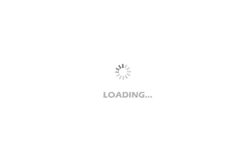
- Popular Resources
- Popular amplifiers
-
Operational Amplifier Practical Reference Handbook (Edited by Liu Changsheng, Zhao Mingying, Liu Xu, etc.)
-
A Complete Illustrated Guide to Operational Amplifier Applications (Written by Wang Zhenhong)
-
Design of isolated error amplifier chip for switching power supply_Zhang Rui
-
DAM medium wave transmitter high frequency power amplifier module test platform_Tian Tian
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Graduation Project: Water Sample Collection at Water Quality Monitoring and Control Station
- [Detailed and practical] Chinese illustration of every parameter of power MOS tube!
- Last day! TI live broadcast with prizes | IoT display solutions using DLP micro-projection technology
- C6000DSP heap and stack
- Potential uses of UWB technology
- Circuit parameters of real op amp
- [Urgent recruitment in Shanghai] RFIC senior design engineer
- Hamburg Records
- About the problem that KEIL MDK can't simulate debugging ARM program
- Flash (STM32) "Big Explanation"