Item name:
Intelligent flowerpot design based on microcontroller
Function:
1. Display the temperature and humidity of the soil in the flower pot and the light intensity outside the flower pot through the display screen;
2. Use the buttons to switch the display interface and set the minimum soil temperature value, minimum humidity value, light intensity value outside the flowerpot, infusion countdown, and soil loosening countdown;
3. Detect soil moisture through soil moisture sensor and soil temperature through DS18B20;
4. Control the heating plate, water pump, infusion, and fill light through relays;
5. Realize soil loosening through stepper motor;
Part of the program:
#include "main.h"
#include "lcd1602.h"
#include "key.h"
#include "ds18b20.h"
#include "adc.h"
#include "motor.h"
#include "timer.h"
/**********************************
Variable definitions
************************************/
uchar key_num = 0; //key scan flag
uchar flag_display = 0; //Display mode flag bit
bit flag_display_cut = 0; //Switch interface flag bit
uint time_num = 0; //10ms count variable
uint temp_value = 0; //Temperature value
uchar temp_min = 10; //Minimum temperature value
uint humi_value = 0; //Humidity value
uchar humi_min = 30; //Minimum humidity value
uint light_value = 0; //Light intensity value
uchar light_min = 50; //Minimum light intensity value
uchar time_songtu = 0; // loosening time
uint time_minus_songtu = 0; //Loose soil countdown time
uchar time_shuye = 0; //Infusion time
uint time_minus_shuye = 0; //Infusion countdown time
extern bit flag_songtu_begin; //Songtu start flag bit
extern bit flag_songtu_finish; //Songtu completion flag bit
extern bit flag_shuye_begin; //Infusion start flag bit
extern bit flag_shuye_finish; //Infusion completion flag bit
/**********************************
function declaration
************************************/
void Delay_function(uint x); //Delay function
void Key_function(void); //Key function
void Monitor_function(void); //Monitoring function
void Display_function(void); //Display function
void Manage_function(void); //processing function
/****
******* Main function
*****/
void main()
{
Lcd1602_Init(); //LCD1602 initialization
Delay_function(50);
lcd1602_clean(); //Clear screen
Delay_function(50);
Ds18b20_Init(); //DS18B20 initialization
Delay_function(50);
Timer0_Init(); //Initialize timer 0
Delay_function(50);
while(1)
{
Key_function(); //Key function
Monitor_function(); //Monitoring function
Display_function(); //Display function
Manage_function(); //processing function
Delay_function(10); //Delay 10ms
time_num++; //10ms interval timing variable +1
if(time_num >= 5000) //The timer accumulates to 5000, and then starts accumulating from 0 again
{
time_num = 0;
}
}
}
/****
******* Delay x ms function
*****/
void Delay_function(uint x)
{
uint m,n;
for(m=x;m>0;m--)
for(n=110;n>0;n--);
}
/****
*******Key function
*****/
void Key_function(void)
{
key_num = Chiclet_Keyboard_Scan(0); //Key scan
if(key_num != 0) //A key is pressed
{
switch(key_num)
{
case 1: //Button 1, switch interface
flag_display++;
if(flag_display >= 6) //A total of 6 interfaces
flag_display = 0;
lcd1602_clean(); //Click once to clear the screen once
break;
case 2:
switch(flag_display)
{
case 0: //When the interface is 0, manually loosen the soil
Motor_Foreward();
break;
case 1: //When the interface is 1, modify the minimum temperature value +1
if(temp_min < 99)
temp_min++;
break;
case 2: //When the interface is 2, modify the minimum humidity value +1
if(humi_min < 99)
humi_min++;
break;
case 3: //When the interface is 3, modify the minimum lighting value +1
if(light_min < 99)
light_min++;
break;
case 4: //When the interface is 4, modify the loosening time +1
time_songtu++;
time_minus_songtu = time_songtu*60;
break;
case 5: //When the interface is 5, modify the infusion time +1
time_shuye++;
time_minus_shuye = time_shuye*60;
break;
default:
break;
}
break;
case 3: //Button 3
switch(flag_display)
{
case 0: //When the interface is 0, manual infusion
RELAY_SHUYE = 0; //Close the infusion relay and start infusion
Delay_function(3000); //Infusion for three seconds
RELAY_SHUYE = 1; //Disconnect the infusion relay and stop the infusion
break;
case 1: //When the interface is 1, modify the minimum temperature value -1
if(temp_min > 0)
temp_min--;
break;
case 2: //When the interface is 2, modify the minimum humidity value -1
if(humi_min > 0)
humi_min--;
break;
case 3: //When the interface is 3, modify the minimum lighting value to -1
if(light_min > 0)
light_min--;
break;
case 4: //When the interface is 4, modify the loosening time by -1
if(time_songtu > 0)
{
time_songtu--;
time_minus_songtu = time_songtu*60;
}
break;
case 5: //When the interface is 5, modify the infusion time by -1
if(time_shuye > 0)
{
time_shuye--;
time_minus_shuye = time_shuye*60;
}
break;
default:
break;
}
break;
case 4: //Button 4, switch interface
flag_display_cut = ~flag_display_cut;
lcd1602_clean(); //Click once to clear the screen once
break;
default:
break;
}
}
}
Previous article:Simple clock design based on 51 microcontroller
Next article:Summary of issues related to crystal oscillators for 51 microcontrollers
Recommended ReadingLatest update time:2024-11-16 12:02
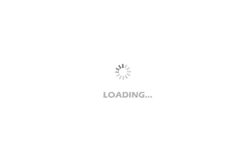
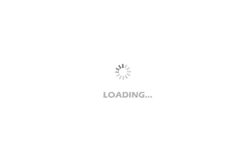
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Traffic Light Control System Based on Fuzzy Control (Single-Chip Microcomputer Implementation)
-
Principles and Applications of Single Chip Microcomputers (Second Edition) (Wanlong)
-
MCU Principles and C51 Programming Tutorial (2nd Edition)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- These tools can help you quickly understand ADI data converter products!
- ADI officially acquired Maxim's old memories
- [Experimental Guide] How to view the number of GPIO added on the FPGA side of the SoC FPGA system
- Looking for a book Yuan Diwen "High Frequency Communication Circuit Design"
- Watch the video, participate in the NXP quiz, and win wonderful gifts!
- Competition Sharing 4: RSL10 Bluetooth SoC BME680 Sensor Data Collection
- [Analog Electronics Course Selection Test] + TI High Precision Laboratory
- Registration has ended | GigaDevice GD32E231 DIY Contest! 100 development boards free to try + great gifts!
- After downloading the program with the emulator, the program cannot be run if the emulator is unplugged
- Here is the DDS information on the national competition list