A variable is a quantity whose value can continuously change during program execution. To use a variable in a program, you must first use an identifier as the variable name and indicate the data type and storage mode used, so that the compilation system can allocate corresponding storage space for the variable. The format for defining a variable is as follows:
In the definition format, except for the data type and variable name table, which are necessary, everything else is optional. There are four storage types: automatic (auto), external (extern), static (statIC) and register (register). The default type is automatic (auto).
The data types here are the same as the definitions of the data types we learned in Lesson 4. After specifying the data type of a variable, you can optionally specify the memory type of the variable. The description of the memory type is to specify the storage area used by the variable in the C51 hardware system and accurately locate it at compile time. Note that the RAM in the AT89C51 chip only has the lower 128 bits, and the upper 128 bits located from 80H to FFH are only useful in the 52 chip and overlap with the special register address. For the address table of the special register (SFR), please see Appendix 2 AT89C51 Special Function Register List
If the memory type is omitted, the system will specify the storage area of the variable according to the default memory type specified by the compilation mode SMALL, COMPACT or LARGE. Variables can be declared in any 8051 storage area regardless of storage mode. However, placing the most commonly used commands such as loop counters and queue indexes in the internal data area can significantly improve system performance. It should also be pointed out that the storage type of the variable has nothing to do with the memory type.
SMALL storage mode places all function variables and local data segments in the internal data storage area of the 8051 system, which makes accessing data very fast, but the address space of SMALL storage mode is limited. When writing small applications, it is good to place variables and data in the data internal data memory because the access speed is fast, but in larger applications, it is better to store only small variables, data or commonly used variables in the data area. (such as cycle count, data index), while large data is placed in other storage areas.
All functions and program variables and local data segments in the COMPACT storage mode are located in the external data storage area of the 8051 system. The external data storage area can have up to 256 bytes (one page). In this mode, the short address of the external data storage area is @R0/R1.
LARGE storage mode The variables and local data segments of all functions and procedures are located in the external data area of the 8051 system. The external data area can be up to 64KB, which requires the use of DPTR data pointers to access data.
I briefly mentioned the methods of defining variables in sfr, sfr16, and sbit before. Let’s take a closer look below.
sfr and sfr16 can directly define the special registers of the 51 microcontroller. The definition method is as follows:
sfr special function register name = special function register address constant;
sfr16 special function register name = special function register address constant;
We can define the P1 port of AT89C51 like this
sfr P1 = 0x90; //Define P1 I/O port, its address is 90H
The sfr key is followed by a name to be defined. You can choose it arbitrarily, but it must comply with the naming rules of identifiers. The name should have a certain meaning. For example, the P1 port can be named P1, which will make the program much easier to read. The equal sign must be followed by a constant, expressions with operators are not allowed, and the constant must be within the address range of the special function register (80H-FFH). For details, please check the related table in the appendix. sfr defines an 8-bit special function register and sfr16 is used to define a 16-bit special function register, such as the T2 timer of 8052, which can be defined as:
sfr16 T2 = 0xCC; //8052 timer 2 is defined here, the address is T2L=CCH, T2H=CDH
When using sfr16 to define a 16-bit special function register, the equal sign is followed by its low-order address, and the high-order address must be located above the physical low-order address. Note that it cannot be used for the definition of timers 0 and 1.
sbit defines bit-addressable objects. Such as accessing a bit in a special function register. In fact, this application is often used, such as accessing the second pin P1.1 in the P1 port. We can define it in the following way:
(1) sbit bit variable name = bit address
sbit P1_1 = Ox91;
This assigns the absolute address of the bit to the bit variable. Like sfr, the bit address of sbit must be between 80H-FFH.
(2) Sbit bit variable name = special function register name ^ bit position
sft P1 = 0x90;
sbit P1_1 = P1 ^ 1; //First define a special function register name and then specify the location of the bit variable name
This method can be used when the addressable bit is located in a special function register
(3) sbit bit variable name = byte address ^ bit position
sbit P1_1 = 0x90^1;
This method is actually the same as 2, except that the address of the special function register is directly expressed as a constant.
The C51 memory type provides a memory type of bdata, which refers to a bit-addressable data memory located in the bit-addressable area of the microcontroller. The data that requires bit-addressability can be defined as bdata, such as:
unsigned char bdata ib; //Define ucsigned char type variable ib in the addressable area
int bdata ab[2]; //Define array ab[2] in the bit-addressable area, these are also called addressable bit objects
sbit ib7=ib^7 //Use the keyword sbit to define a bit variable to independently access one of the addressable bit objects.
sbit ab12=ab[1]^12;
The maximum value of the bit position after the operator "^" depends on the specified base address type, char0-7, int0-15, long0-31.
Let's use the circuit from the previous lesson to practice the knowledge in this lesson. We are also doing a simple marquee experiment, the project is called RunLED2. The procedure is as follows:
sfr P1 = 0x90; //No predefined file is used here,
sbit P1_0 = P1 ^ 0; //Define the special register yourself instead
sbit P1_7 = 0x90 ^ 7; //The predefined file we used before actually has this function
sbit P1_1 = 0x91; //The P1 port and P10, P11, and P17 pins are defined here respectively.
void main(void)
{
unsigned int a;
unsigned char b;
do{
for (a=0;a<50000;a++)
P1_0 = 0; //Light up P1_0
for (a=0;a<50000;a++)
P1_7 = 0; //Light up P1_7
for (b=0;b<255;b++)
{
for (a=0;a<10000;a++)
P1 = b; //Use the value of b to make marquee patterns
}
P1 = 255; //Turn off the LED on P1
for (b=0;b<255;b++)
{
for (a=0;a<10000;a++) //P1_1 flashes
P1_1 = 0;
for (a=0;a<10000;a++)
P1_1 = 1;
}
}while(1);
}
Previous article:Design of data acquisition system based on ADmC812 micro-conversion chip and DSP chip TMS320F206
Next article:Detailed explanation of various instructions of microcontroller
Recommended ReadingLatest update time:2024-11-16 09:40
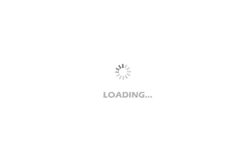
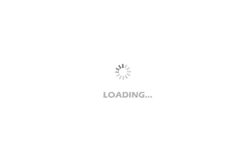
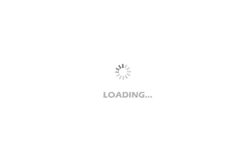
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- How to use the VGA_SYNC_N signal in the VGA of DE1-SOC?
- CCS Tips: Remove the prompt when burning DSP/BIOS
- SEED-DEC6416 is available for purchase, second-hand is also acceptable
- Calculation of stack usage in C2000 DSP
- IRLR024 Output Current
- Bidirectional thyristor circuit wiring problem
- "Goodbye 2019, Hello 2020" + The wind and clouds are free and happy
- A simple question for help
- The power problem of the series resistance of the atomizer heating wire
- [GD32F310 Review] Interrupt test and GPIO usage