This application note describes how to configure the UART of a high-speed microcontroller or ultra-high-speed flash microcontroller to communicate with an SCI-enabled device. It begins with a brief discussion of the differences between SCI and UART modules and ends with a practical example of how to configure an 8051-based Dallas Semiconductor microcontroller UART to communicate with a SCI module.
introduce
The Serial Communications Interface (SCI) is a high-speed serial I/O port that allows synchronous or asynchronous communication between devices. It allows the microcontroller to be connected to a variety of similarly functional peripherals, as well as the standard RS-232 interface. The exact implementation of SCI varies by device manufacturer; many devices support features such as full-duplex communication in asynchronous mode, parity checking, error detection, and programmable character length in <> to <> bits.
All 8051-based Dallas Semiconductor microcontrollers are capable of communicating with SCI-capable devices, even if the SCI functionality is not explicitly listed in the microcontroller's feature list. All of our microcontrollers contain one to three 8051-type UARTs and can be configured to operate in most common SCI modes.
This application note describes how to configure the UART of a high-speed microcontroller or ultra-high-speed flash microcontroller to communicate with a SCI-enabled device. It begins with a brief discussion of the differences between SCI and UART modules and ends with a practical example of how to configure an 8051-based Dallas Semiconductor microcontroller UART to communicate with a SCI module. A code example is provided that demonstrates how to initialize the microcontroller and perform simple tests to ensure the device is communicating correctly.
Characteristics of SCI
As mentioned above, SCI is a high-speed serial interface. It has many similarities to the 8051-style UART on Dallas Semiconductor 8051-based microcontrollers. The following is a list of SCI functions in UART and their counterparts. Users should note that not all SCI modules support all features listed, so users should carefully read the data sheets of SCI-enabled devices to understand how to use them.
feature | SCI | Dallas Semiconductor UART |
asynchronous mode | Works with most implementations | Serial mode 1, 2, 3 |
synchronous mode | Available on some implementations | Serial mode only 0 |
character length | 1 to 9 (if optional character length is supported) | 8 or 9 |
parity | Available on some implementations | Supported by software in 9-bit mode |
Framing error | Yes | Yes |
idle character | Detect idle characters to wake up the device. | The UART cannot detect idle characters, but the UART microprocessor communication mode can be used to signal the UART to treat the next byte as an address/identifier. |
break character | SCI can send and receive interrupt characters (00h). | Interrupt characters can be transmitted by converting the serial port RX pin to logic 0. Receipt of a break character may cause framing errors, depending on the selected character length. |
example
Most SCI modules support asynchronous communication formats, many of which are exclusive. The example here demonstrates how to configure a Dallas Semiconductor 8051-based microcontroller to communicate asynchronously with an SCI-enabled device. In this case, we configure the microcontroller to communicate with the target SCI configured with the following characteristics:
10-bit asynchronous mode; 1 start, 8 data, 1 stop bit
Baud rate: 19200 bps
In order to communicate with this device we will make the following decisions to set up the Dallas Semiconductor microcontroller:
Use serial port 0 for communication
The external clock source is 22.1184MHz
The serial port will be configured in 10-bit asynchronous mode; 1 start, 8 data, 1 stop bit (this is serial port mode 1.
The baud rate generator clock source will be Timer 1 in auto-reload mode (Timer Mode 2).
Since all Dallas Semiconductor 8051-based microcontroller timers default to native divide-by-12 operating mode, this example has the advantage of being applicable to all Dallas Semiconductor devices regardless of the core's clock divisor. This is because the DS5000FP (divide by 12), the DS80C320 (divide by 4), and the DS89C450 (divide by 1) all use the same serial port timings if the timer's higher speed option is not selected. For details on UART operation, see the Serial I/O section of the appropriate user guide.
Since the SCI determines the format of the data, the Dallas Semiconductor microcontroller must next be initialized to the correct baud rate. The 8-bit auto-reload mode (Timer Mode 2) generates the baud rate via a user-selectable timer overflow driven by an external clock source. This adds considerable flexibility to the design and simplifies development since the baud rate can be easily selected in software, allowing multiple baud rates from the same clock source. The formula for determining the baud rate is as follows:
where osc_frequency is the frequency of the external clock source in MHz, TH1 is the 1-bit reload value placed in the Timer 8 MSB SFR, and SMOD_0 (PCON.7) is the serial port 0 multiplier enable bit. Alternatively, if the baud rate and oscillator frequency are known, the value of the 8-bit reload number TH1 can be solved for using the following formula:
Assuming the external clock source is 22.1184MHz, a TH1 value of FDh will produce a target baud rate of 19200 and clear the multiplier bit. For more information on baud rate selection, see the Serial I/O section of the appropriate user guide.
The following short assembly code example demonstrates how to initialize serial port 0 to communicate with an SCI module configured in 10-bit asynchronous mode at 19200 bps. On successful operation, it will echo any characters received. This functionality can be easily removed, making it a universal shell for any user requiring SCI communications applications.
;SCI emulation example
; Simple transmit test to demonstrate how to configure 8051 UART to
; emulate an SCI module. Test code embedded in this example echoes back
; received characters.
org 0h ;Reset vector.
ljmp start
org 23h ;Serial port 0 vector.
ljmp SP0_ISR
org 100h ;Start of code.
start: ;Initialize Serial Port 0 for mode 1, 19200 baud
MOV TMOD, #020h ;Set timer 1 for mode 2 (8-bit auto reload)
MOV SCON0, #050h ;SP0 10-bit asynchronous mode with receive enabled
;Now select the reload value based on baud rate and xtal frequency.
MOV TH1, #0FDh ;19200 baud at 22.11 MHz
;MOV TH1, #0FDh ;9600 baud at 11.059 MHz
;MOV TH1, #0FAh ;9600 baud at 22.11 MHz
SETB TR1 ;Serial port is initialized, now start timer
;Enable Interrupts
MOV IE, #90h ;This example supports interrupt-driven communications, so
; enable global and serial port 0 interrupts.
;Test code in receive interrupt routine echoes back any received characters
; when combined with the loop here.
loop: sjmp loop
SP0_ISR: ;Serial port 0 Interrupt Service Routine
jb RI0, RIO_INT ;Determine if receiver/transmitter was cause of interrupt.
TIO_INT: ;Interrupt was caused by transmission.
;
; Placeholder for transmitter routine
;
CLRTI0
RETI
RIO_INT: ;Interrupt was caused by reception
;
; Placeholder for receiver routine
;
MOV A, SBUF0 ;Test code that echoes back received character
MOV SBUF0, A ; Remove for real code.
CLRRI0
RETI
Summarize
The UART in Dallas Semiconductor's 8051-based microcontrollers can be easily configured to interface with SCI modules in many devices. This popular serial interface can operate in a variety of modes, but the most common is the 11/232-bit asynchronous mode used in RS-10 communications. Allowing Dallas Semiconductor microcontrollers to connect to SCI modules increases overall system flexibility as they can be connected to a wider range of embedded systems.
Although this example focuses on asynchronous mode of operation, the Dallas Semiconductor microcontroller can also be configured to interface with an SCI operating in synchronous mode. The SCI module's similarity to the 8051 UART allows this interface to be accomplished with minimal effort. For more information about synchronous mode (Serial Port Mode 0), see the Serial I/O section of the appropriate user guide.
Previous article:8051 microcontroller interrupt system structure and interrupt control principle
Next article:Design of temperature and humidity acquisition system based on 8051 microcontroller
Recommended ReadingLatest update time:2024-11-22 04:41
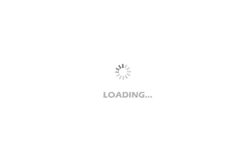
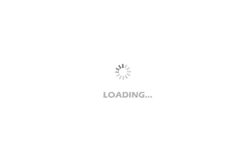
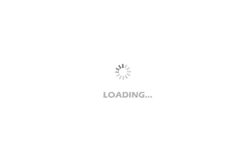
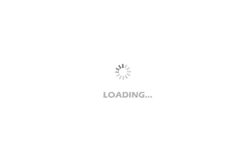
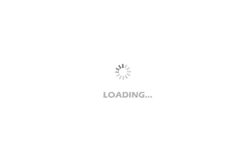
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
STC32G Series MCU Technical Reference Manual
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- Europe's three largest chip giants re-examine their supply chains
- Breaking through the intelligent competition, Changan Automobile opens the "God's perspective"
- The world's first fully digital chassis, looking forward to the debut of the U7 PHEV and EV versions
- Design of automotive LIN communication simulator based on Renesas MCU
- When will solid-state batteries become popular?
- Adding solid-state batteries, CATL wants to continue to be the "King of Ning"
- The agency predicts that my country's public electric vehicle charging piles will reach 3.6 million this year, accounting for nearly 70% of the world
- U.S. senators urge NHTSA to issue new vehicle safety rules
- Giants step up investment, accelerating the application of solid-state batteries
- Guangzhou Auto Show: End-to-end competition accelerates, autonomous driving fully impacts luxury...
- Classification and characteristics of PIC 8-bit microcontrollers (Part 2)
- Application of CAN bus technology in digital servo system
- In 2010, China's analog IC sales reached nearly 200 billion yuan
- EEWORLD University ---- Sitara realizes intelligent servo
- AM5708 platform transplants Ubuntu system and docker container
- Teach you to write microcontroller timer interrupt program
- Compilation warning: last line of file ends without a newline
- casio highway surveying program
- Electronics World Talk!!!
- CC2541 Bluetooth Learning - General I/O Port Interrupt