1. LED lights flash at different frequencies
Next, we take the following LED light flashing code as an example to change the delay length to see the effect of the LED light.
void setup()
{
pinMode(2, OUTPUT);
}
void loop()
{
digitalWrite(2, HIGH);
delay(50); // Wait for xx millisecond(s)
digitalWrite(2, LOW);
delay(50); // Wait for xx millisecond(s)
}
500ms delay flash (1Hz frequency)
200ms delay flash (2.5Hz frequency)
50 ms delay flash (10Hz frequency)
Through three comparative experiments, we found that as the frequency increases, our LED lights slowly begin to feel less flicker. Due to the visual dwell effect of our human eyes, generally a refresh rate greater than 50Hz can meet our requirements.
2. High frequency LED flashing deformation process
We still use this code to fix the frequency at 50Hz, and then keep the period unchanged, that is, the combined time of the high and low levels is equal to 40ms, and then change the duty cycle of the high and low levels (the percentage of the high and low levels in the total cycle), we pass Adjust the length of the high and low level delays to adjust the brightness ratio
Code part:
void setup()
{
pinMode(2, OUTPUT);
}
void loop()
{
digitalWrite(2, HIGH);
delay(10); // Wait for 1000 millisecond(s)
digitalWrite(2, LOW);
delay(10); // Wait for 1000 millisecond(s)
}
50Hz frequency maximum brightness (that is, the delay is 0 when the light is off, and the delay is 20ms when it is on)
50Hz frequency 50% brightness (that is, the delay is 10ms when the light is off, and the delay is 10ms when the light is on)
50Hz frequency 25% brightness (that is, the delay is 15ms when the light is off, and the delay is 5ms when the light is on)
50Hz frequency 10% brightness (that is, the delay is 18ms when the light is off, and the delay is 2ms when the light is on)
We connect the above actions together, that is to say, we make the brightness delay a continuous change. In order to achieve better results in practice, we change the delay to a delay of 200 us, so that the continuous change effect is better.
Code part:
void setup()
{
pinMode(2, OUTPUT);
}
int count = 0;
int PWM_Time = 50;
void loop()
{
digitalWrite(2, HIGH); //LED light off
delayMicroseconds(200-PWM_Time); // Wait for xx millisecond(s)
digitalWrite(2, LOW); // LED light on
delayMicroseconds(PWM_Time); // Wait for xx millisecond(s)
count++;
if(count==50)
{
count = 0;
PWM_Time++;
if(PWM_Time>=200) PWM_Time = 0;
}
}
LED light gradually brightening effect
Let's modify it further and make it a breathing effect.
Code part:
void setup()
{
pinMode(2, OUTPUT);
}
int count = 0; // Because the delay is relatively short, it will change if used directly.
// It's too fast to see the effect. Let's add a counting variable.
int PWM_Time = 0; // LED duty cycle variable
int LED_Togle_Flag = 0; // LED gradually turns on and off flip flag
void loop()
{
if(LED_Togle_Flag)
{
digitalWrite(2, HIGH); //LED light off
delayMicroseconds(200-PWM_Time); // Wait for xx millisecond(s)
digitalWrite(2, LOW); // LED light on
delayMicroseconds(PWM_Time); // Wait for xx millisecond(s)
}
else
{
digitalWrite(2, HIGH); //LED light off
delayMicroseconds(PWM_Time); // Wait for xx millisecond(s)
digitalWrite(2, LOW); // LED light on
delayMicroseconds(200-PWM_Time); // Wait for xx millisecond(s)
}
count++;
if(count==50)
{
count = 0;
PWM_Time++;
//Switch light and dark change logic
if(PWM_Time>=200)
{
PWM_Time = 0;
LED_Togle_Flag = ~LED_Togle_Flag;
}
}
}
LED light breathing light
LED "Meteor Shower"
First, let’s analyze the logic of the meteor shower:
First we need to achieve such an effect, the first one is the brightest, and then the next one is 45% of the brightness of the previous one.
Code part:
// ----------------------------------------------------------------------------
// LED_Rains.ino
//
// Raindrop flow effect implemented by digital pins
// The difference between the raindrop flow effect and the running water light (marquee) is that the raindrop flow effect has a trailing effect, that is, the light that has been lit is slowly extinguished.
//
//Use all pins of UNO to use analog PWM to achieve the effect of raindrop flow, including analog input ports that can also be used as digital outputs
// Each pin is connected to the positive pole of the LED, and the negative pole of the LED is connected to GND.
// ----------------------------------------------------------------------------
const unsigned char leds[] = { A4, A5, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11 }; // All pins are arranged in the order of LED wiring
const unsigned int maxPwm = 100; // To simulate PWM manually, you can define the maximum PWM value yourself, so it is easier to calculate by defining a number of one hundred or one thousand.
unsigned int ledPwm[12] = { 1, 3, 4, 6, 9, 13, 18, 25, 35, 50, 70, 100}; // Store the brightness PWM value of each LED during operation
void setup()
{
for (char i = 0; i < 12; ++i)
{
pinMode(leds[i], OUTPUT);
}
}
void loop()
{
unsigned int i, j;
for (i = 0; i < 12; ++i) // Turn on the light first, then turn off the light when the duty cycle reaches the switching point
{
digitalWrite(leds[i], LOW);
}
for( i=0; i for (j = 0; j < 12; ++j) { if (i == ledPwm[j]) digitalWrite(leds[j], HIGH); } delayMicroseconds(1); } } Static meteor shower effect Code explanation: We first store proportional values in the brightness array ledPwm[12]. Here I calculate it based on a ratio of 70%. For example, the darkest value is 100, then the next darkest value is 100*70% = 70, and so on. Then we light the lights according to the assigned brightness. This part of the code is to light up all the LED lights first for (i = 0; i < 12; ++i) // Turn on the light first, then turn off the light when the duty cycle reaches the switching point { if (ledPwm[i] == 0) continue; digitalWrite(leds[i], LOW); } This part of the code controls the off time according to the brightness of the LED light. We first divide the brightness into 100 parts according to the maximum brightness value "maxPWM". The delay for each part is 1us, and then check the current value in the internal loop. Whether the brightness value reaches the allocated number of copies. If it reaches, it will turn off. If it does not reach, it will continue to stay on. for( i=0; i for (j = 0; j < 12; ++j) { if (i == ledPwm[j]) digitalWrite(leds[j], HIGH); } delayMicroseconds(1); } Obviously, such a static meteor shower still cannot meet our requirements. Next, we will make the meteor shower move first. We need it to move like this LED meteor shower dynamic decomposition diagram Let's try to move it by one bit first. I just need to rearrange the values in the ledPwm[12] array. This is actually an array operation. unsigned int ledPwm[12] = {3, 4, 6, 9, 13, 18, 25, 35, 50, 70, 100, 1}; // Store the brightness PWM value of each LED during operation The effect of LED meteor shower moving one position From the above we know that if we have a way to perform continuous operations on the array, we can achieve the effect of a "meteor shower" flow. The code below is the time counter that comes with Arduino. You can directly read the value inside and use it to assist counting. In fact, you don’t need this. You can count directly in it yourself. extern volatile unsigned long timer0_millis; Complete version of the code: Put all the numerical variables that need to be changed at the front. This is a common practice in writing reusable programs. It can be flexibly adapted to multiple lights, and at the same time, the speed and brightness ratio can be adjusted. // ---------------------------------------------------------------------------- // LED_Rains.ino // // Raindrop flow effect implemented by digital pins // The difference between the raindrop flow effect and the running water light (marquee) is that the raindrop flow effect has a trailing effect, that is, the light that has been lit is slowly extinguished. // //Use all pins of UNO to use analog PWM to achieve the effect of raindrop flow, including analog input ports that can also be used as digital outputs // Each pin is connected to the positive pole of the LED, and the negative pole of the LED is connected to GND. // ---------------------------------------------------------------------------- const unsigned char leds[] = { A4, A5, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11 }; // All pins are arranged in the order of LED wiring const unsigned int maxPwm = 100; // To simulate PWM manually, you can define the maximum PWM value yourself, so it is easier to calculate by defining a number of one hundred or one thousand. const unsigned int initPwm = 100; // The pwm value of the brightest light, that is, the brightness of the first light when moving const unsigned int deltaPwm = 1; // The pwm value of the light slowly extinguishing, how much darker the next light is than the previous light. This is equivalent to an arithmetic queue. Equally different brightnesses don’t feel good, so the next factor of equal proportions is introduced. const unsigned int deltaPercent = 45; // The latter light is darker than the previous light, and its brightness is a few percent of the previous light. Compared with the previous decrease, this is equivalent to a geometric series const unsigned long delayMs = 100; //Movement delay, unit ms const unsigned char ledNum = sizeof(leds) / sizeof(leds[0]); // Number of pins, that is, the number of LEDs unsigned int ledPwm[ledNum]; //Storage the brightness PWM value of each LED during runtime void setup() { for (char i = 0; i < ledNum; ++i) { pinMode(leds[i], OUTPUT); ledPwm[i] = 0; } } extern volatile unsigned long timer0_millis; // Declare the external variable timer0_millis for use in the program. It is actually the return value of millis() - the number of milliseconds the program has been running. Let the LED "meteor shower" move
LED "Meteor Shower" continuous motion
Previous article:Debugging methods for using new IC chips in microcontroller projects
Next article:(3) Another way to learn the basic components of button control LED lights
Recommended ReadingLatest update time:2024-11-22 14:11
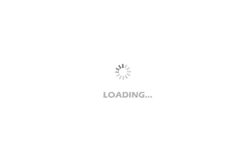
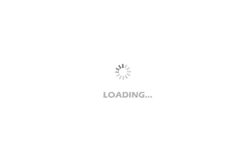
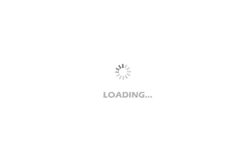
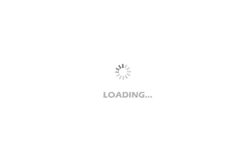
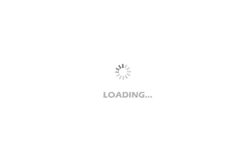
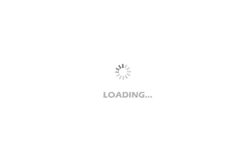
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
-
Intelligent Control Technology of Permanent Magnet Synchronous Motor (Written by Wang Jun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- RAQ #223: How to measure and determine soft-start timing without a soft-start equation?
- RAQ #223: How to measure and determine soft-start timing without a soft-start equation?
- GigaDevice's full range of automotive-grade SPI NOR Flash GD25/55 wins ISO 26262 ASIL D functional safety certification
- GigaDevice's full range of automotive-grade SPI NOR Flash GD25/55 wins ISO 26262 ASIL D functional safety certification
- New IsoVu™ Isolated Current Probes: Bringing a New Dimension to Current Measurements
- New IsoVu™ Isolated Current Probes: Bringing a New Dimension to Current Measurements
- Infineon Technologies Launches ModusToolbox™ Motor Kit to Simplify Motor Control Development
- Infineon Technologies Launches ModusToolbox™ Motor Kit to Simplify Motor Control Development
- STMicroelectronics IO-Link Actuator Board Brings Turnkey Reference Design to Industrial Monitoring and Equipment Manufacturers
- Melexis uses coreless technology to reduce the size of current sensing devices
- 【UFUN Learning】Chapter 5 SD Card Test
- Introduction and working principle of humidity sensitive capacitor (humicap)
- Using STM32 to drive the stepper motor, the motor vibrates severely at high speed. I have tried using subdivision and current feedback, but the effect is not good.
- An electric mosquito swatter actually has so many circuits. Have you learned all of them?
- How to draw the graph of the complex number solution of X^2+1=0 in the coordinate system? Can anyone help me? Thank you.
- Web page control 430 single chip microcomputer
- Bicycle modification series: code table
- Do-it-yourself hand warmer repair
- Subform button display problem
- Huawei's internal hardware development and design process