S3C2440 keyboard module circuit
There are a total of S1, S2, S3, and S4 buttons, which correspond to the four interrupt sources EINT19, EINT2, EINT0, and EINT11 respectively. The interrupt framework and service routine are as follows:
Interrupt response, take out the keyboard number (1-4) that triggered the interrupt and put it in R5
;FileASM_Interrupt.s
;(1)Set the interrupt vector table
Mode_USR EQU 0x50 ; IRQ interrupt is open, FIQ interrupt is closed
Mode_FIQ EQU 0xD1 ; turn off IRQ and FIQ interrupts
Mode_IRQ EQU 0xD2 ; turn off IRQ and FIQ interrupts
Mode_SVC EQU 0xD3 ; turn off IRQ and FIQ interrupts
GET 2440Reg_addr.inc
AREA MyCode, CODE,READONLY
IMPORT initial_keyboard_INT
ENTRY ;Set interrupt vector table
B ResetHandler ;Reset interrupt service routine
B. ; handlerUndef
B. ; SWI interrupt handler
B. ; handlerPAbort
B. ; handlerDAbort
B. ; handlerReserved
B HandlerIRQ ;HandlerIRQ
B. ; HandlerFIQ
;(2)Reset interrupt handler
ResetHandler
BL Clock_Init ; initialize watchdog, clock
;BL MemSetup ;Initialize SDRAM
LDR SP, =SvcStackSpace ;Set management mode stack
MSR CPSR_c, #Mode_IRQ
LDR SP, =IrqStackSpace ; Set IRQ interrupt mode stack
MSR CPSR_c, #Mode_FIQ
LDR SP, =FiqStackSpace ; Set FIQ interrupt mode stack
MSR CPSR_c, #Mode_USR ;Enter user mode
LDR SP, =UsrStackSpace ; Set user and system mode stack
;BL Init_DATA ;Initialize readable and writable data
BL initial_keyboard_INT
LDR R0,=pEINT0;EINT0 address in the interrupt entry transfer table
LDR R1,=EINT0 ;R1 interrupt service routine entry address
STR R1,[R0] ;EINT0 interrupt program entry address is written into the interrupt transfer table
LDR R0,=pEINT2;EINT2 address in the interrupt entry transfer table
LDR R1,=EINT2 ;R1 interrupt service routine entry address
STR R1,[R0] ;EINT2 interrupt program entry address is written into the interrupt transfer table
LDR R0,=pEINT8_23;EINT8_23 address in the interrupt entry transfer table
LDR R1,=EINT8_23 ;R1 interrupt service routine entry address
STR R1,[R0] ;EINT8_23 interrupt program entry address is written into the interrupt transfer table
MAIN_LOOP
NOP
B MAIN_LOOP ; infinite loop, interrupted by IRQ; initialize readable and writable data area
Clock_Init
GET Clock_Init.s ;Initialize watchdog and clock
MemSetup
GET MemSetup.s ;Initialize SDRAM
Init_DATA
GET Init_DATA.s ; Initialize the readable and writable data area
;(3)IRQ interrupt handler
HandlerIRQ
SUB LR,LR, #4 ; Calculate return address
STMFD SP!, {LR} ;Save breakpoint to stack in IRQ mode
LDR LR,= Int_Return ; modify LR and return to Int_Return after executing the EINT8_23 handler.
LDR R0,=INTOFFSET ; Get the number of the interrupt source
LDR R1,[R0]
LDR R2,=Int_EntryTable ;Start address of interrupt transfer table
LDR PC,[R2,R1,LSL#2] ; Check the interrupt transfer table and obtain the handler entry address of EINT8_23, which is equivalent to a subroutine call without parameters, but no breakpoints are saved.
Int_Return ; You must return here after executing the EINT8_23 handler.
LDMFD SP!, {PC }^ ;IRQ interrupt service routine returns, ^ means copying the value of SPSR to CPSR
;EINT0 handler
EINT0
MOV R5,#3
LDR R0,=SRCPND ;Clear several pending registers
LDR R1,[R0]
STR R1,[R0]
LDR R0,=INTPND
LDR R1,[R0]
STR R1,[R0]
MOV PC,LR ; Return to IRQ interrupt service routine from EINT0 handler
;EINT2 handler
EINT2
MOV R5,#2
LDR R0,=SRCPND ;Clear several pending registers
LDR R1,[R0]
STR R1,[R0]
LDR R0,=INTPND
LDR R1,[R0]
STR R1,[R0]
MOV PC,LR ; Return to IRQ interrupt service routine from EINT2 handler
; (4) EINT8_23 handler
EINT8_23
LDR R0,=EINTPEND ;R0 is set to the EINTPEND register address
LDR R1,[R0] ; Read EINTPEND, 24 bits in total, the lowest 4 bits are reserved
CMP R1,#0x080000 ; Determine whether EINT19 interrupt
LDREQ R5,=0x1 ;R5 is the key number
CMP R1,#0x000800 ; Determine whether EINT11 interrupt
LDREQ R5,=0x4
LDR R0,=SRCPND ;Clear several pending registers
LDR R1,[R0]
STR R1,[R0]
LDR R0,=INTPND
LDR R1,[R0]
STR R1,[R0]
LDR R0,=EINTPEND
LDR R1,[R0]
STR R1,[R0]
MOV PC,LR ; Return to IRQ interrupt service routine by EINT8_23 handler
AREA MyRWData, DATA, READWRITE ;Set RW Base=0x33ffe700
Int_EntryTable
GET Int_EntryTable.s ; Interrupt transfer table, 32 entries in total
AREA MyZIData, DATA, READWRITE, NOINIT,ALIGN=8
;Pushdown stack, according to the alignment, the starting address of the segment is 0x33ffe800, and the space of each stack area is 1k
SPACE 0x100 * 4 ; Management mode stack is empty
SvcStackSpace SPACE 0x100 * 4 ;Interrupt mode stack space
IrqStackSpace SPACE 0x100 * 4 ; fast interrupt mode stack space
FiqStackSpace SPACE 0x100 *
UsrStackSpace
END
;File Int_EntryTable.s, defines the interrupt transfer table
;32 channels in total, corresponding to 32 inputs of the arbiter
pEINT0 DCD 0 ; stores the entry address of the EINT0-3 interrupt service routine
pEINT1 DCD 0
pEINT2 DCD 0
pEINT3 DCD 0
pEINT4_7 DCD 0 ; stores the entry address of the EINT4_7 interrupt service routine
pEINT8_23 DCD 0 ; stores the EINT8_23 interrupt service routine entry address——EINT8_23
pINT_CAM DCD 0 ; stores 26 internal interrupt service routine entry addresses
pnBATT_FLT DCD 0
pINT_TICK DCD 0
pINT_WDT_AC97 DCD 0
…
pINT_UART0 DCD 0
pINT_SPI1 DCD 0
pINT_RTC DCD 0
pINT_ADC DCD 0
END
#include "2440Reg_addr.h" //Special register address definition
void initial_keyboard_INT(void){
rGPGCON=((0x10<<22)|(0x10<<6)); //Set GPIO as external interrupt pin
rGPFCON=((0x10|(0x10<<4));//Set GPF as interrupt pin
rGPGUP=0xffff; // No pull-up
rGPFUP = 0xff; // No pull-up
rEINTMASK&=((0x1<<11)|(0x1<<19));//Open EINT11, 19 interrupt
rEXTINT0=0x0; //All are low level interrupts, no filtering is used
rEXTINT1=0x0;
rEXTINT2=0x0;
rINTMSK=0xFFFFFFD5; //Open interrupt
}
Previous article:S3C2440 interrupt analysis (analysis of each interrupt-related register)
Next article:S3C2440——Use URAT0 interrupt mode to send and receive strings
Recommended ReadingLatest update time:2024-11-16 13:44
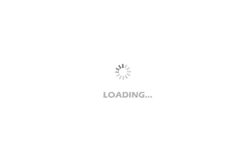
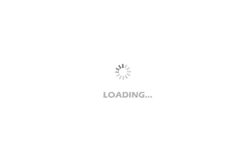
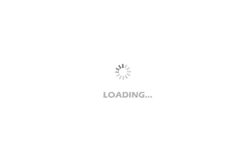
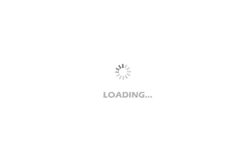
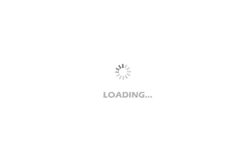
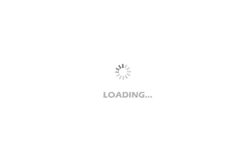
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- DAC analog multiplier, DDS (frequency synthesizer), signal generator
- For beginners of TI 6000 series DSP
- MSP430F6638 MCU interrupt, clock and low power consumption
- How to draw this circuit in dxp
- Microchip Live at 10:00 today: MCU programming is no longer difficult, use MPLAB Code Configurator (MCC) to achieve rapid development
- Application of TMS320DM642 in ATP Technology of Mobile Platform
- [ufun Learning] Unboxing Instructions: What are the preparations before formal learning?
- Raspberry Pi PICO and Firefly Jar
- Today is 520. As boys majoring in science and engineering, how do you surprise your significant other?
- A batch of new Bluetooth speakers are sent for free, and you only need to pay the ten yuan shipping fee