1. Sorting
AREA TEXT,CODE,READWRITE
ENTRY
MOV R0,#100 ;Number of loops
MOV R1,#0 ; Initialize data
LOOP
ADD R1,R1,R0; add the data to get the final data
SUBS R0,R0,#1; loop data R0 minus 1
CMP R0,#0 ; Compare R0 with 0 to see if the loop is finished
BNE LOOP ; Determine whether the loop is finished, if accepted, proceed to the following steps
LDR R2,=RESULT
STR R1,[R2]
RESULT
DCD 0
STOP
B STOP
; Arrangement algorithm: first compare all the data with the first one, and finally take out the smallest data and put it in the first memory unit
;Then start comparing from the second memory unit and put the second smallest data into the second memory unit.
; By this inference, the ten data can be arranged.
AREA TEXT,CODE,READWRITE
ENTRY
LDR R0,=DATA; Get the starting address of DATA data
MOV R1,R0
MOV R5,#9; The number of loops at the beginning is 10, so it should start from 9
MOV R6,R5
COMPARE
ADD R0,R0,#4; The address stored in R0 + 4 is represented as the address of the next number to be compared
SUB R6,R6,#1 ; Loop once and subtract 1
LDR R2,[R1]; Take the data in the register and compare the size
LDR R3,[R0]
CMP R3,R2
MOVCC R7,R2; If the value of the following address is smaller than the previous one, swap their data
MOVCC R2,R3
MOVCC R3,R7
STR R2,[R1] ; store data in the corresponding memory unit
STR R3,[R0]
CMP R6,#0 ; Check if each cycle ends
BNE COMPARE
ADD R1,R1,#4; After each loop, the initial memory address is moved back one unit.
MOV R0,R1 ; Reinitialize the address stored in the register in the previous loop
SUB R5,R5,#1; After each loop, the number of times in the following loops will be reduced by 1
MOV R6,R5
CMP R5,#0 ; Check if all loops are finished
BNE COMPARE
DATA
DCD 9,4,6,7,8,1,3,2,0,5
STOP
B STOP
C language code:
#include
extern void strcopy(char *d, const char *s);
int main()
{
const char *srcstr="abcdefghi";
char dststr[]="ighfedcba";
strcopy(dststr,srcstr);
return 0;
}
ARM assembly code:
STACK_TOPEQU 0x40002000
PRESERVE8
AREA SCopy, CODE, READONLY
EXPORT START
EXPORT strcopy
import main
ENTRY
START
LDR R13,=STACK_TOP
B main
strcopy
LDRB r2, [r1], #1
STRB r2, [r0], #1
CMP r2, #0
BNE strcopy
MOV pc,lr
END
stack_top equ0x40002000
PRESERVE8
export copy
AREA copy,CODE,READONLY
import copystr
export start
ENTRY
start
ldr r13,=stack_top
ldr r0,=src
ldr r1,=dst
BL copystr
src
dcb "abcdefghij"
dst
dcb "helloworld"
end
//C program
#include
void copystr(char *d,char *s)
{
while((*d++=*s++)!='');
}
Previous article:Programmers' Model of ARM Processor
Next article:Build and compile the Android 4.4.4 environment for the 4412 development board
Recommended ReadingLatest update time:2024-11-16 16:42
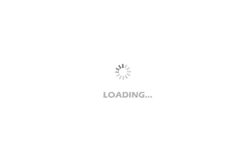
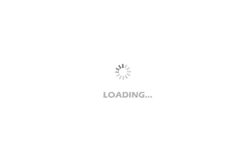
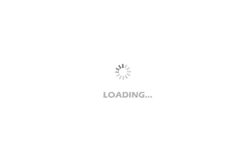
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [ACM32F070 supporting capacitive touch development board target -- Arduino preparation for dual-machine UART communication linkage]
- Award-winning live broadcast: ADI digital active noise reduction headphone solution invites you to listen on August 6th to let technology calm us down
- FOC dual motor development board
- Answer questions to win prizes | ADI Technology Express Issue 1
- Capacitance and inductance recording
- After 12 days, he was taken to quarantine
- What is the prospect of the development of artificial intelligence?
- Looking for a DC-DC module with 0-10V adjustable output current.
- Miscellaneous talks on rectification (VII)
- Sacco Micro benchmarks ST/ON/CJ