There are two main ways to program interrupts for the MC9SDG128B:
The first is to use the symbol "TRAP_PROC". TRAP_PROC tells the compiler that the following function is an interrupt service routine. The compiler will end this function with a special interrupt return instruction (for most processors, this is usually the RTI instruction).
The second is to use the "interrupt" keyword. The "interrupt" keyword is a non-standard ANSI-C keyword, so it is not supported by all ANSI-C compiler vendors. Similarly, the usage of the "interrupt" keyword may change for different compilers. The "interrupt" keyword also prompts the compiler that the following function is an interrupt service routine.
Once the interrupt service function is written, you must associate the interrupt service routine with the interrupt vector table. This is done by initializing the interrupt vector table. You can initialize the interrupt vector table in two ways:
The first is in the PRM file, using the "VECTOR ADDRESS" or "VECTOR" command. The connector provides two commands to initialize the interrupt vector table: VECTOR ADDRESS or VECTOR. You use the VECTOR ADDRESS command to write the address of the interrupt service routine into the interrupt vector table.
The second is to use the "interrupt" keyword. When you write an interrupt service routine, you can directly associate the interrupt service routine with a specific interrupt number in the ANSI-C source file.
int intcount = 0; void timer_init(void) { TSCR2_PR0 = 1; //prescale factor is 128 TSCR2_PR1 = 1; TSCR2_PR2 = 1; intcount++; EnableInterrupts; timer_init(); for(;;) {} The following is an interrupt service routine written using the TRAP_PROC symbol: TSCR2_PR0 = 1; //prescale factor is 128 TSCR2_PR1 = 1; TSCR2_PR2 = 1; intcount++; EnableInterrupts; timer_init(); for(;;) {} The interrupt service routine must be located in the non-banked area. You can use "#pragma CODE_SEG NON_BANKED" to locate the interrupt routine in the non-banked area. At the same time, you must ensure that "sectionNON_BANKED" does not appear in the .prm file. At the end of the interrupt service routine, you need to add "#pragma CODE_SEG DEFAULT", otherwise, the following functions will also be located in the "non-banked" area. So our interrupt service routine must be surrounded by "#pragma CODE_SEG NON_BANKED" and "#pragma CODE_SEG DEFAULT".
The following is the 16-bit free timer overflow interrupt handler for MC9SDG128B that I wrote, which has been debugged:
#include
#include
#pragma LINK_INFO DERIVATIVE "mc9s12dg128b"
TSCR2_TOI = 1; //overflow enable
TFLG2_TOF = 1;
TSCR1_TEN = 1; //timer enable
}
#pragma CODE_SEG NON_BANKED
interrupt 16 void timer_interrupt_handle(void)
{
TFLG2_TOF = 1; //clear interrupt flag
};
#pragma CODE_SEG DEFAULT
void main(void)
{
}
#include
#include
#pragma LINK_INFO DERIVATIVE "mc9s12dg128b"
int intcount = 0;
void timer_init(void)
{
TSCR2_TOI = 1; //overflow enable
TFLG2_TOF = 1;
TSCR1_TEN = 1; //timer enable
}
#pragma CODE_SEG NON_BANKED
#pragma TRAP_PROC
void timer_interrupt_handle(void)
{
TFLG2_TOF = 1;
};
#pragma CODE_SEG DEFAULT
void main(void)
{
}
After the interrupt service program is written, you need to add the following sentence in the .prm file:
VECTOR 16 timer_interrupt_handle
so that the interrupt service program is associated with the corresponding interrupt number. This program has also been debugged.
Previous article:MC9S12XS128 8-bit PWM servo control
Next article:Detailed explanation of s19 file format
Recommended ReadingLatest update time:2024-11-16 10:28
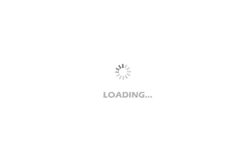
- Popular Resources
- Popular amplifiers
-
Siemens PLC Programming Technology and Application Cases (Edited by Liu Zhenquan, Wang Hanzhi, Yang Kun, etc.)
-
Siemens PLC from Beginner to Mastery with Color Illustrations (Yang Rui)
-
Siemens PLC Project Tutorial
-
Siemens S7-1200-PLC Programming and Application Tutorial (3rd Edition) (Edited by Shi Shouyong)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- stm32f030R8 Chinese reference material
- Recruitment: Executive Vice President of Automotive Electronics (Executive Vice President of New Energy)
- ATmega4809 Curiosity Nano Review + Unboxing and Powering on
- Sell an unused Jiuxintaike oscilloscope and get a Guwei desktop multimeter for free
- MSP430F1232 external 4M crystal oscillator cannot start
- Q: How do you determine the inductance value when selecting a common-mode coil?
- What does BMS device learning and chemical ID mean?
- [NUCLEO-L452RE Review] + Simple Digital Voltmeter
- Gaoyun FPGA various manuals
- [2022 Digi-Key Innovation Design Competition] ESP32S2 Lighting