1 Using LCD1602 to display hours and minutes based on 51 single chip microcomputer
2. Button control hour-minute adjustment
3. Can realize the function of full-time timing (buzzer sounds)
4. Alarm mode
5. Press the button to switch modes (Mode 1: hour-minute display, Mode 2: 60-second countdown)
1. Design ideas:
main body:
The mod value is controlled by external interrupt 0; mod=0, 1.2, 3 correspond to clock mode, adjustment mode, alarm setting mode, and one-minute countdown mode respectively.
detail:
mod0
Through the timing counter, the variable second (s) is increased every second. Every 60 seconds, 1 minute (min) is increased and s is set to 0. Every 60 minutes, 1 hour h is increased. When h>23, h=0; a one-day cycle is performed.
mod1
Key control adds min, h and s to 0
mod2
In addition, set the variables min1 and h1. When min=min1, h=h1, the buzzer will sound.
mod3
Set the variable daojishi=60, through the timing counter, daojishi decreases by 1 every second, when daojishi<0, the buzzer sounds
in addition:
1. Set external interrupt 2 and turn off the buzzer
2. Time can only send one bit at a time
program:
#include //After K1, K3 adds minutes, K4 adds time, K2 adds seconds, K1 enters alarm setting, K2 exits //K2 turns off the alarm typedef unsigned int u16; //declare and define the data type typedef unsigned char u8; #define data8b P1 sbit K1=P3^2; //External interrupt 0 sbit K2=P3^3; //External interrupt 1 sbit K3=P3^0; sbit K4=P3^1; sbit BUZ=P2^4; //Buzzer, 0 beeps sbit RW=P2^5; //Pin 4, data (1) or command (0) sbit RS=P2^6; //Pin 5, read (1) write (0) sbit E=P2^7; //pin 6, enable signal u8 code dat1[]={0X30,0X31,0X32,0X33, 0X34,0X35,0X36,0X37, 0X38,0X39}; void delay(u16 i) //delay function { while(i--); } void open012() //Open interrupt 0,1, timer interrupt 0 { TMOD|=0X01; //Select timer 0 mode, working mode 1 ET0=1; //Enable timer 0 interrupt EA=1; //Open the general interrupt TR0=1; //Turn on the timer EX0=1; //Open external interrupt 0 IT0=1; //Edge trigger mode EX1=1; //Open external interrupt 1 IT1=1; //Edge trigger } void wrm(u8 dat) //write command { delay(1000); RS=0; RW=0; E=0; data8b=dat; E=1; delay(1000); E=0; } void wrd(u8 dat) //write data { delay(1000); RS=1; RW=0; E=0; data8b=dat; E=1; delay(1000); E=0; } void zero() { wrm(0X38); //8-bit data, two lines display, 5*7 wrm(0X0c); //No cursor, turn on display wrm(0X06); //Move the cursor right, but the screen does not move wrm(0X01); //Clear screen wrm(0X80); //Set the data pointer starting point } u8 fg=0,sg=0,bfg=0,bsg=0; u16 i=0; u8 s=0; u8 mod=0; char dingshi; bit bell=0; bit zanting=1; void fangsong() { wrd(dat1[sg/10]); //tens digit of the hour wrd(dat1[sg%10]); //Hour digit wrd(0x3A); //: wrd(dat1[fg/10]); //ten digits wrd(dat1[fg%10]); //dividing into units wrd(0x3A); //: wrd(dat1[(s/10)]); //10 seconds wrd(dat1[(s%10)]); //seconds } void fangsong1() { wrm(0X80); wrd(dat1[sg/10]); //tens digit of the hour wrd(dat1[sg%10]); //Hour digit wrd(0x3A); //: wrd(dat1[fg/10]); //ten digits wrd(dat1[fg%10]); //dividing into units wrd(0x3A); //: wrd(dat1[(s/10)]); //10 seconds wrd(dat1[(s%10)]); //seconds } void chuli() { if(fg==60) { sg++; fg=0; } if(sg==24) { sg=0; } } void main() { u8 shijian; open012(); zero(); chuli(); fangsong(); shijian=100; while(1) { while(mod==0) { EX1=1; //Open external interrupt 1 if(s==60) { fg++; //Convert 60 seconds to 1 minute s=0; } chuli(); if((fg==0)&&(shijian!=sg)) { BUZ=0; shijian=sg; } fangsong1(); if((BUZ==0)&&(bell==0)) { delay(1000); BUZ=1; } if((fg==bfg)&&(sg==bsg)&&(bell==1)) BUZ=0; else BUZ=1; } while(mod==1) { EX1=0; //Disable external interrupt 1 zero(); fangsong(); if(K3==0) { delay(1000); if(K3==0) fg++; } if(K4==0) { delay(1000); if(K4==0) sg++; } if(K2==0) { delay(1000); if(K2==0) s=0; } if(fg>59) { fg=0; } if(sg>23) { sg=0; } if(s>=59) { s=0; } } while(mod==2) //Set the alarm { if(bfg==60) { bsg++; bsg=0; } if(bsg==24) { bsg=0; } zero(); wrd(0x20); wrd(0x20); wrd(0x20); wrd(dat1[(bsg/10)]); //tens digit of the hour wrd(dat1[(bsg%10)]); //Hour digit wrd(0x3A); //: wrd(dat1[(bfg/10)]); //ten digits wrd(dat1[(bfg%10)]); //divide into units if(K3==0) { delay(1000); if(K3==0) bfg++; } if(K4==0) { delay(1000); if(K4==0) bsg++; } bell=1; zero(); } while(mod==3) { while(zanting) { dingshi=60; EX1=1; //Open external interrupt 1 wrm(0X80); wrd(dat1[(dingshi/10)]); //Tens digit of the hour wrd(dat1[(dingshi%10)]); //Hour digit } wrm(0X80); wrd(dat1[(dingshi/10)]); //Tens digit of the hour wrd(dat1[(dingshi%10)]); //Hour digit while(dingshi<0) { wrm(0X80); wrd(dat1[0]); //tens digit of the hour wrd(dat1[0]); //Hour digit BUZ=0; } } } } void time0() interrupt 1 { TH0=0XFC; //Assign initial value to the timer, set the timer to 1ms TL0=0X18; i++; if(i==1000) //ms converted to s { i=0; s++; dingshi--; } } void key1() interrupt 0 //External interrupt 0, adjust time { delay(1000); if(K1==0) { mod++; while(!K1); } if(mod>3) { mod=0; } zero(); } void naozhong() interrupt 2 //Switch the alarm { if(K2==0) { delay(1000); //debounce if(K2==0) { bell=0; BUZ=1; zanting=~zanting; } //Turn off the buzzer while(!K2); //Confirm button is released } } 2. Harvest 1. Become more proficient in the use of timer interrupts and external interrupts The same button can be used to achieve different functions by turning on and off external interrupts. 2. Learn a little bit about register operations 3. Summary of Interrupt Configuration External Interrupt Timer interrupt Serial communication 4. Be more proficient in using Proteus Made a small software development version 5. Learned how to learn a new component (LCD1602) 1. Read the instructions, focusing on the timing diagram, truth table, etc. 2. Write the program according to the timing diagram 3. When neither of the above two conditions is true, look for relevant information 6. Download Principle The principle of microcontroller programming: Microcontroller burning, also known as microcontroller program downloading and burning, is essentially the microcontroller and PC transferring the compiled program to the microcontroller through the interface specified by the chip manufacturer according to the programming protocol specified by the chip manufacturer, and the microcontroller stores the data in its own memory. To understand this principle, you need to know several knowledge points: There are programs inside the MCU, which are fixed in the hardware when it leaves the factory and cannot be modified by the user (this will also be considered as no programs inside it). These programs can call various communication interfaces, internal memory, etc. Downloadable communication interfaces: JTAG, SPI, UART, USB, etc. (There are also many that can be expanded to 485, Ethernet, etc.) Programming protocol: Generally, it will be made public by major manufacturers and can be found in the chip's dedicated technical manual; Memory: There are many types, such as mask, EPROM, EEROM, flash, etc., with different lifespans. Mask can only be used once and must be made by the factory. Flash can be erased and written 10,000 times or more. It can be understood metaphorically like this: the microcontroller is the motherboard of the computer, the program we write is the operating system, the basic program for booting the operating system is loaded into the motherboard, and downloading the program is like installing the system on the computer! 7. Others 1. Understand the operating principles of current electronic watches 2. Learn to use ready-made products (spreadsheets) as references to write programs 3. Understand the importance of communicating with others (inspired by Wang, improved the original program) 3. Later plans 1. Continue to study 32 2. Practice welding skills 3. Continue to learn other peripherals of 51 4. Learn circuits, analog electronics, digital electronics, DXP and other related knowledge according to your interests
Previous article:51 single chip DHT11 temperature and humidity ESP8266WiFi mobile phone APP display design
Next article:LCD display 51 single chip simple calculator
Recommended ReadingLatest update time:2024-11-17 00:03
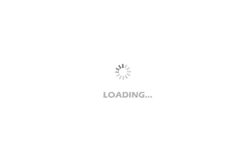
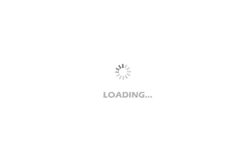
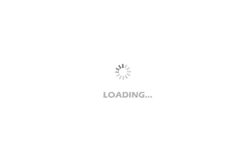
- Popular Resources
- Popular amplifiers
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
stm32+lcd1602 example
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Why is the voltage ringing spike of MOSFET different in boost and buck mode?
- The circuit for discharging the PFC output capacitor is shown in Figure 2.
- Basic syntax of Verilog HDL
- Found a method to import Altium Designer into SIwave
- Thanks to okhxyyo for searching and fish001 for sharing
- Please advise, is it easy to pass the postgraduate examination and graduate, and how useful is the degree certificate?
- About the LAUNCHXL-F28379D Development Kit
- Let me try this feature
- EEWORLD University-What is a transient voltage suppressor (TVS) diode?
- [Xianji HPM6750 Review] RT-Thread SDIO driver and file system