1. SPI module introduction
The Serial Peripheral Interface (SPI) module provides full-duplex, synchronous, serial communication between the microcontroller and peripheral devices. These peripheral devices can include other microcontrollers, analog-to-digital shifters, shift registers, sensors, and memories. The SPI can run at a baud rate of up to 2 divided by the bus clock in master mode and up to 4 divided by the bus clock in slave mode.
The central element of SPI is the SPI shift register. Data is written to the double-buffered transmitter (written to SPIDR) and then transferred to the SPI shift register at the start of the data transfer. After conversion in the data byte, the data is transferred to the double-buffered receiver, where the data can be read (read from SPIDR). The pin multiplexing logic controls the connection between the microcontroller pins and the SPI module. The functional block diagram of SPI is shown in the figure below.
When the SPI is configured as a master SPI, the clock output is routed to the SCK pin, the shifter output is routed to MOSI, and the shifter input is routed out of the MISO pin. When the SPI is configured as a slave SPI, the SCK pin is the clock output, MOSI is the shifter output, and the MISO pin is the shifter input.
In an external SPI system, just connect all the SCK pins to each other, all the MISO pins to each other, and all the MOSI pins to each other. Peripheral devices often use slightly different names for these pins.
AT25040N is an EEPROM memory with a storage capacity of 4K. In this experiment, the SPI interface is used to read and write data. The schematic diagram of the hardware circuit is shown in the figure below.
The SPI interface consists of 4 pins, namely SCK, MOSI, MISO, and CS.
SCK — SPI serial clock
When the SPI is enabled as a slave SPI, this pin is the serial clock input. When the SPI is enabled as a master SPI, this pin is the serial clock output.
MOSI — Master Data Out, Slave Data In
When the SPI is enabled as a master SPI, this pin is the serial data output. When the SPI is enabled as a slave SPI, this pin is the serial data input. When the single-wire bidirectional mode is selected and the master mode is selected, this pin becomes a bidirectional data I/O pin (MOMI).
MISO — Master Data In, Slave Data Out
When the SPI is enabled as a master SPI, this pin is the serial data input. When the SPI is enabled as a slave SPI, this pin is the serial data output. If the single-wire bidirectional mode is selected and the slave mode is selected, this pin becomes a bidirectional data I/O pin (SISO).
CS — Select from
During an SPI transfer, data is sampled (read) and transferred from the MOSI pin on an SCK edge, and the bit value on the MOSI pin is changed after half an SCK cycle. After 8 SCK cycles, the data in the master SPI's shift register has been transferred from the MOSI pin to the slave SPI, and the 8-bit data in the MISO pin is transferred to the master SPI's shift register. At the end of the transfer, the received data byte is transferred from the shifter to the receive data buffer, and SPRF is set, and the display data can be read by reading SPIDR. If there is another data byte waiting in the transmit buffer at the end of the transfer, it is moved to the shifter, SPTEF is set, and a new transfer is started.
Here we introduce the AT25040N communication mode, which is as follows:
Before the microcontroller writes data to AT25040N, it must first write the "Write Enable" command to AT25040N. The command format is as follows:
The format of writing data from the microcontroller to the AT25040N is as follows. The written data consists of at least 3 bytes of commands. The first byte is the "op-code" command. The "A" bit of this byte is the A8 bit in the AT25040N address. Because the AT25040N is an EEPROM with a storage space of 4Kb, it can store 512 bytes of data. Each byte corresponds to an address, which needs to be represented by a 9-bit address, namely "A8-A0". After the "op-code" is "A7-A0", followed by the data to be transmitted.
The format of the data read by the AT25040N microcontroller is as follows: First, the "op-code" command, followed by the lower 8 bits of the read data address, and then the data to be read.
2. Routine Test
In this experiment, we use SPI to write a byte of data into the AT25040 chip, and then read the data out to verify it. The SPI initialization code is shown below.
void INIT_SPI(void)
{
HOLD_dir = 1;
CS_dir = 1;
SPI0CR1 = 0b01010000; // Enable SPI, disable interrupts, clock high valid, phase 0;
SPI0CR2 = 0x00; //SS pin is normal I/O, bidirectional mode;
SPI0BR = 0x70; //Set the SPI clock frequency to 2MHz;
CS = 1;
}
The initialization code sets the SPI to the same mode as the AT25040 chip mode, sets the CS pin to a normal pin, and finally sets the communication frequency through program control.
Below is the code for SPI sending.
void SPI_send(unsigned char data)
{
while(!SPI0SR_SPTEF);
SPI0DRL = data;
}
The code is relatively simple, showing whether the buffer is empty and writing the data to be sent when it is empty.
Below is the code for SPI receiving.
unsigned char SPI_receive(void)
{
unsigned char temp,data;
while(!SPI0SR_SPIF);
temp = SPI0SR;
data = SPI0DRL;
return(data);
}
The receiving code is also relatively simple. It waits for the flag to be set, then clears the flag by reading the flag register, and finally reads the data and returns it.
Below is the code of the main function.
void main(void)
{
DisableInterrupts;
INIT_PLL();
LEDCPU_dir = 1;
INIT_SPI();
INIT_FM25040A();
EnableInterrupts;
LEDCPU = 1;
CS = 0;
SPI_send(0x06);
delay();
CS = 1;
delay();
CS = 0;
SPI_send(0x02);
SPI_send(0x00);
SPI_send('E'); //Store one byte of data
delay();
CS = 1;
longdelay();
CS = 0;
SPI_send(0x03);
SPI_send(0x00);
delay();
SPI_send(0x00);
delay();
temp_SPI = SPI_receive(); //Read data so that the data in the shift register can be stored in SPI0DRL
data_receive = SPI_receive(); //Read data
CS = 1;
if(data_receive == 'E') //Judge whether the data is correct
LEDCPU = 0;
for(;;);
}
In the main function, the program sends a byte of data to AT25040, reads the data, and then determines whether the data is correct. If correct, the LED light is turned on.
You can analyze the code according to the communication protocol of AT25040 above.
Download the program to the microcontroller and run it, and the LED light will turn on.
Previous article:Freescale 16-bit MCU (XIV) - CAN bus module test
Next article:Freescale 16-bit MCU (XII) - IIC module test
Recommended ReadingLatest update time:2024-11-16 16:40
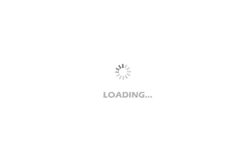
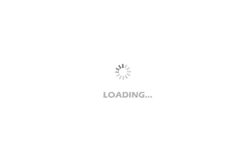
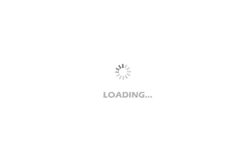
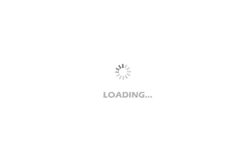
- Popular Resources
- Popular amplifiers
-
Practical Development of Automotive FlexRay Bus System (Written by Wu Baoxin, Guo Yonghong, Cao Yi, Zhao Dongyang, etc.)
-
Automotive Electronics KEA Series Microcontrollers——Based on ARM Cortex-M0+ Core
-
Automotive integrated circuits and their applications
-
ADS2008 RF Circuit Design and Simulation Examples (Edited by Xu Xingfu)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Question about username
- NeoPixel simulates fluid physics motion
- BMP library management based on FPGA.pdf
- Making some preparations for the flag I set up on the forum this year (first time shopping at the ghost market)
- Open source scientific calculator based on STC32G
- FPGA---DDS Sine Wave Generator
- FPGA/DSP/GPU, accelerating radar signal processing
- Help! Can someone help me analyze a small circuit? Thank you!
- Analysis of the principle of switching power supply
- Wireless wifi good books