1. ECC Check
ECC: error Checking and correct, can check errors and correct errors.
Advantages: Extremely fast speed
Disadvantages: can only detect 2-bit errors and can only correct 1-bit errors
If you want to verify this, you need to turn on param.no_tags_ecc=0. The default param.no_tags_ecc=1 does not perform tags verification.
At the same time, the ECC check information should also be added to mkyaffs2image so that the ECC can be read from nand_flash for comparison.
nandmtd2_read_chunk_tags
--> yaffs_unpack_tags2
void yaffs_unpack_tags2(struct yaffs_ext_tags *t, struct yaffs_packed_tags2 *pt, int tags_ecc)
{
enum yaffs_ecc_result ecc_result = YAFFS_ECC_RESULT_NO_ERROR;
if (pt->t.seq_number != 0xffffffff && tags_ecc) {
struct yaffs_ecc_other ecc;
int result;
yaffs_ecc_calc_other((unsigned char *)&pt->t, sizeof(struct yaffs_packed_tags2_tags_only), &ecc);
result = yaffs_ecc_correct_other((unsigned char *)&pt->t,
sizeof(struct yaffs_packed_tags2_tags_only), &pt->ecc, &ecc);
switch (result) {
case 0:
ecc_result = YAFFS_ECC_RESULT_NO_ERROR;
break;
case 1:
ecc_result = YAFFS_ECC_RESULT_FIXED;
break;
case -1:
ecc_result = YAFFS_ECC_RESULT_UNFIXED;
break;
default:
ecc_result = YAFFS_ECC_RESULT_UNKNOWN;
}
}
yaffs_unpack_tags2_tags_only(t, &pt->t);
t->ecc_result = ecc_result; //Save the result after verification, calling its function is to check
yaffs_dump_packed_tags2(pt); // just print, don't care
yaffs_dump_tags2(t); // just print, don't care
}
This part mainly verifies the result in yaffs_packed_tags2* pt, sizeof(yaffs_packed_tags2)=4*7=28
struct yaffs_packed_tags2_tags_only {
unsigned seq_number;
unsigned obj_id;
unsigned chunk_id;
unsigned n_bytes;
};
struct yaffs_ecc_other {
unsigned char col_parity;
unsigned line_parity;
unsigned line_parity_prime;
};
struct yaffs_packed_tags2 {
struct yaffs_packed_tags2_tags_only t;
struct yaffs_ecc_other ecc;
};
Note: This check is only called by nandmtd2_read_chunk_tags, because the data in pt is stored in the OOB area of nand flash, and the data in data area has been checked when reading.
Yaffs also uses the first 28 bytes of the OOB area as data, so this part also needs to be verified. However, nand_flash only verifies the data in the data area, so you need to write your own code to verify the 28 bytes of OOB.
2. Verification Algorithm
2. Generate the column_parity_table table
#include #include unsigned char entry(unsigned char x) { unsigned char b0, b1, b2, b3, b4, b5, b6, b7; unsigned char p4, p2, p1, p4p, p2p, p1p; unsigned char linep; unsigned char result; b0 = (x & 0x01) ? 1 : 0; b1 = (x & 0x02) ? 1 : 0; b2 = (x & 0x04) ? 1 : 0; b3 = (x & 0x08) ? 1 : 0; b4 = (x & 0x10) ? 1 : 0; b5 = (x & 0x20) ? 1 : 0; b6 = (x & 0x40) ? 1 : 0; b7 = (x & 0x80) ? 1 : 0; p4 = b7 ^ b6 ^ b5 ^ b4; p4p = b3 ^ b2 ^ b1 ^ b0; p2 = b7 ^ b6 ^ b3 ^ b2; p2p = b5 ^ b4 ^ b1 ^ b0; p1 = b7 ^ b5 ^ b3 ^ b1; p1p = b6 ^ b4 ^ b2 ^ b0; linep = p1 ^ p1p; result = 0; if(p4) result |= 0x80; if(p4p) result |= 0x40; if(p2) result |= 0x20; if(p2p) result |= 0x10; if(p1) result |= 0x08; if(p1p) result |= 0x04; if(linep) result |= 0x01; //result >>= 2; //if(linep) result |= 0x40; return result; } int main(int argc, char *argv[]) { unsigned i; printf("const unsigned char column_parity_table[] = {"); for(i = 0; i < 256; i++) { if((i & 0xf) == 0) printf("n"); printf("0x%02x, ",entry((unsigned char) i)); } printf("n};n"); } 2.1 Verification steps a. Generate ECC through yaffs_ecc_calc_other, called new_ecc b. XOR new_ecc with old_ecc. If they are different, an error occurs. c. Similar to a binary tree, find the error and make corrections void yaffs_ecc_calc_other(const unsigned char *data, unsigned n_bytes, struct yaffs_ecc_other *ecc_other) //生成新的ECC { unsigned int i; unsigned char col_parity = 0; unsigned line_parity = 0; unsigned line_parity_prime = 0; unsigned char b; for (i = 0; i < n_bytes; i++) { b = column_parity_table[*data++]; col_parity ^= b; if (b & 0x01) { /* odd number of bits in the byte */ line_parity ^= i; line_parity_prime ^= ~i; } } ecc_other->col_parity = (col_parity >> 2) & 0x3f; ecc_other->line_parity = line_parity; ecc_other->line_parity_prime = line_parity_prime; } 3. int yaffs_ecc_correct_other(unsigned char *data, unsigned n_bytes, struct yaffs_ecc_other *read_ecc, const struct yaffs_ecc_other *test_ecc) { //Compare with test_ecc and make corrections if they are different unsigned char delta_col; /* column parity delta */ unsigned delta_line; /* line parity delta */ unsigned delta_line_prime; /* line parity delta */ unsigned bit; delta_col = read_ecc->col_parity ^ test_ecc->col_parity; delta_line = read_ecc->line_parity ^ test_ecc->line_parity; delta_line_prime = read_ecc->line_parity_prime ^ test_ecc->line_parity_prime; if ((delta_col | delta_line | delta_line_prime) == 0) return 0; /* no error */ if (delta_line == ~delta_line_prime && (((delta_col ^ (delta_col >> 1)) & 0x15) == 0x15)) { bit = 0; //bit <0-7>, represents which bit is wrong if (delta_col & 0x20) bit |= 0x04; if (delta_col & 0x08) bit |= 0x02; if (delta_col & 0x02) bit |= 0x01; if (delta_line >= n_bytes) return -1; data[delta_line] ^= (1 << bit); //Found the wrong bit, reverse it return 1; /* corrected */ } if ((hweight32(delta_line) + hweight32(delta_line_prime) + hweight8(delta_col)) == 1) { /* Reccoverable error in ecc */ *read_ecc = *test_ecc; return 1; /* corrected */ } /* Unrecoverable error */ return -1; }
So each result is:
Previous article:About S3C2440 u-boot supports nand hw ecc
Next article:OOB layout of tq210 nand 8-bit HWECC and YAFFS2
Recommended ReadingLatest update time:2024-11-16 12:47
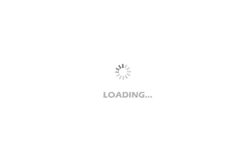
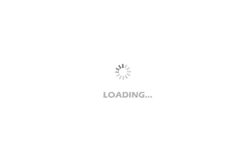
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University - How to use switching chargers to design safe and reliable products with long standby time and powered by micro batteries
- Why can't I download the program on my LPC?
- Tesla exposed a low-level vulnerability: Use Raspberry Pi to DIY car keys, unlocking in just 90 seconds
- Instructions for using 86 box modbus
- Xinhua Dictionary of Power Supply--A brief review of the book "Basics of Power Supply Design"
- MSP430 FAQs: Watchdog and Timer
- Chip supply issues
- Do you have more award-winning power banks? If so, please give me one.
- I want to know what this DSA is and why I don't choose a fixed resistance resistor.
- Review Summary: Tuya Sandwich Wi-Fi & BLE SoC NANO Main Control Board