#################################################################################################
# Common internal parameters of SHELL:
# $# - the total number of arguments passed to the program
# $? —— The exit situation of the previous code or shell program in the shell. If it exits normally, it returns 0, otherwise it returns a non-zero value.
# $* - A string consisting of all the arguments passed to the program.
# $n —— represents the number of parameters, $1 represents the first parameter, $2 represents the second parameter...
# $0 - the name of the current program
# $@ - save all parameters in the form of "parameter 1" "parameter 2" ...
# $$ —— The (process ID number) PID of this program
# $! —— PID of the previous command
# Here is some more explanation about $?, which can be used as additional shell knowledge
# Remember: $? always represents the exit status of the last shell command. When the function is executed, if other commands are executed
# command, then $? no longer indicates the status after the function is executed, but indicates the exit status of other commands.
#-a means (and) both conditions are true at the same time
# -eq means two values are equal
# -gt means n1 is greater than n2, that is, the front is greater than the back
# -lt means n1 is less than n2, that is, the former is less than the latter
# "(" ")" is the escape of parentheses, which are converted into ordinary parentheses "()" to wrap the conditional expression
# The expression in if[...] means: If the number of parameters passed to mkconfig ($#) is equal to 2 and the first variable is
# "-A"($1), an error message will be displayed. What are $0, $1, $2,...? Let's see how the pseudo target make TQ210_config is executed in Makefile.
###########################################################################
# Specify the interpreter, using -e is equivalent to #!/bin/bash
#!/bin/sh -e
# Script to create header files and links to configure
# U-Boot for a specific board.
#
# Parameters: Target Architecture CPU Board [VENDOR] [SOC]
#
# (C) 2002-2006 DENX Software Engineering, Wolfgang Denk # ############################################################################ # Create a new configuration file by default # When executing make TQ210_config, print out the board name TQ210 APPEND=no # Default: Create new config file BOARD_NAME="" # Name to print in make output # Some internal parameters of SHELL are described at the beginning, and no more will be said later! ############################################################################ # When executing make TQ210_config, this process will call the mkconfig file in the uboot root directory. # There will be 6 parameters passed in, as follows # $0 $1 $2 $3 $4 $5 $6 $# # mkconfig TQ210 arm s5pv210 TQ210 samsung s5pv210 6 # BOARD_NAME ARCH CPU BOARD VENDOR SOC # $# -gt 0 means execution when $# is not 0. Obviously, it will not be executed here. # The effect of shift is to make $1=$2, $2=$3, $3=$4..., and the original $1 will be lost. Therefore, the effect of the while loop is, # Process the options passed to the mkconfig script in sequence (--, -a, -n, -t, *). Since we did not pass any options to mkconfig, # items, so the code in the while loop has no effect. while [ $# -gt 0 ] ; do case "$1" in --) shift ; break ;; -a) shift ; APPEND=yes ;; -n) shift ; BOARD_NAME="${1%%_config}" ; shift ;; *) break ;; esac done ########################################################################### # If BOARD_NAME is empty, do nothing; otherwise, assign it to $1, i.e. TQ210 # This sentence assigns BOARD_NAME = TQ210 [ "${BOARD_NAME}" ] || BOARD_NAME="$1" ########################################################################### # Check the number of variables passed in, if less than 4 then exit, if more than 6 then also exit [ $# -lt 4 ] && exit 1 [ $# -gt 6 ] && exit 1 ########################################################################### # The shell prints "Configuring for TQ210 board..." echo "Configuring for ${BOARD_NAME} board..." # # Create link to architecture specific headers # ########################################################################### # If the source top-level directory (SRCTREE) and the directory storing the compiled files (OBJTREE) are different, # The directory (OBJTREE) where the compiled files are stored creates two files include and include2 # Enter the include2 directory and delete the asm folder # Create a soft link asm, link to ${SRCTREE}/include/asm-arm # Then go to the parent directory of the current directory and enter the include folder # Delete asm-arm and asm folders # Create an asm-arm folder in the current directory and create a soft link asm pointing to asm-arm if [ "$SRCTREE" != "$OBJTREE" ] ; then mkdir -p ${OBJTREE}/include mkdir -p ${OBJTREE}/include2 cd ${OBJTREE}/include2 rm -f asm ln -s ${SRCTREE}/include/asm-$2 asm LNPREFIX="../../include2/asm/" cd ../include rm -rf asm-$2 rm -f asm mkdir asm-$2 ln -s asm-$2 asm ########################################################################### # If the source top-level directory (SRCTREE) is the same as the directory storing the compiled files (OBJTREE), # Enter the include directory, delete the asm directory, and create a soft link asm pointing to asm-arm in the current directory # else cd ./include rm -f asm ln -s asm-$2 asm be ########################################################################### # Delete asm-arm/arch. Establishing a soft link actually operates on the target of the link. rm -f asm-$2/arch ########################################################################### # if [ -z "$6" -o "$6" = "NULL" ] ; then ln -s ${LNPREFIX}arch-$3 asm-$2/arch else ln -s ${LNPREFIX}arch-$6 asm-$2/arch be # create link for s3c24xx SoC if [ "$3" = "s3c24xx" ] ; then rm -f regs.h ln -s $6.h regs.h rm -f asm-$2/arch ln -s arch-$3 asm-$2/arch be # create link for s3c64xx SoC if [ "$3" = "s3c64xx" ] ; then rm -f regs.h ln -s $6.h regs.h rm -f asm-$2/arch ln -s arch-$3 asm-$2/arch be # create link for s5pc1xx SoC if [ "$3" = "s5pc1xx" ] ; then rm -f regs.h ln -s $6.h regs.h rm -f asm-$2/arch ln -s arch-$3 asm-$2/arch be # create link for s5pc11x SoC if [ "$3" = "s5pc11x" ] ; then rm -f regs.h ln -s $6.h regs.h rm -f asm-$2/arch ln -s arch-$3 asm-$2/arch be # create link for s5pv210 SoC ######################################################## # TQ210 uses s5pv210, so this code will be executed # Delete the regs.h file, # Create a link file regs.h pointing to s5pv210.h # Delete asm-arm/arch, # Create a soft link asm-arm/arch pointing to arch-s5pv210 if [ "$3" = "s5pv210" ] ; then rm -f regs.h ln -s $6.h regs.h rm -f asm-$2/arch ln -s arch-$3 asm-$2/arch be # create link for s5p64xx SoC if [ "$3" = "s5p64xx" ] ; then rm -f regs.h ln -s $6.h regs.h rm -f asm-$2/arch ln -s arch-$3 asm-$2/arch be # create link for s5p644x SoC if [ "$3" = "s5p644x" ] ; then rm -f regs.h ln -s $6.h regs.h rm -f asm-$2/arch ln -s arch-$3 asm-$2/arch be ########################################################################### # If the architecture is arm architecture, delete the current directory (the current directory is the top-level directory of U-boot source code/include) # Create a soft link in the asm-arm/proc directory ln -s proc-armv asm-arm/proc if [ "$2" = "arm" ] ; then rm -f asm-$2/proc ln -s ${LNPREFIX}proc-armv asm-$2/proc be # create link for s3c64xx-mp SoC if [ "$3" = "s3c64xx-mp" ] ; then rm -f regs.h ln -s $6.h regs.h rm -f asm-$2/arch ln -s arch-$3 asm-$2/arch be # # Create include file for Make # ########################################################################### # Generate make header file config.mk # ARCH=arm,CPU=s5pv210,BOARD=TQ210, add to config.h # > config.mk is forcibly overwritten to create config.mk and then import the data into config.mk # Add VENDOR=samsung,SOC=s5pv210 to config.mk echo "ARCH = $2" > config.mk echo "CPU = $3" >> config.mk echo "BOARD = $4" >> config.mk [ "$5" ] && [ "$5" != "NULL" ] && echo "VENDOR = $5" >> config.mk [ "$6" ] && [ "$6" != "NULL" ] && echo "SOC = $6" >> config.mk # # Create board specific header file # ################################################################### # Create the specified configuration header file, default APPEND=no (there is an assignment at the beginning of this file) # Execute else and create a new config.h file if [ "$APPEND" = "yes" ] # Append to existing config file then echo >> config.h else > config.h # Create new config file be ################################################################### # In config it will show /* Automatically generated - do not edit */ # #include echo "/* Automatically generated - do not edit */" >>config.h echo "#include # Return success status exit 0
Previous article:TQ210——S5PV210 uboot top-level config.mk analysis
Next article:TQ210 —— S5PV210 uboot top-level Makefile analysis
Recommended ReadingLatest update time:2024-11-16 11:53
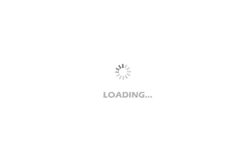
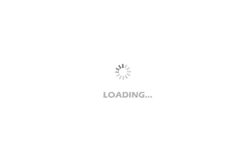
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Types of defects in the EMC packaging forming process
- Application of millimeter wave radar technology in corner radar
- Smart Camera Structural Design--Physical Assembly
- Answer questions to win prizes | Prevent machine failures before they happen, and monitor the health status of large machines to help you
- Chat casually@
- GD32L233C-START Review——01. Unboxing and Development Kit
- About c0_init_calib_complete of ddr4
- Connectivity Primer: Solutions for the IoT Space
- [Allwinner V853 heterogeneous multi-core AI intelligent vision development board evaluation] Compile openWrt
- MSP430F5529 generates PWM waves with CCS