/************************************************************************
Purpose: To establish a SimpleSoft test program for PS/2 (software query and read data received by PS/2)
Target system: Based on AVR microcontroller
Application software: ICCAVR
Version: Version 1.0
*************************************************************************/
/*01010101010101010101010101010101010101010101010101010101010101010101
----------------------------------------------------------------------
Version update record:
----------------------------------------------------------------------
Experimental content:
Press the PC keyboard, observe the data read by the microcontroller, and use the LED of the PA/PB port for indication.
----------------------------------------------------------------------
Hardware Connection:
Use a short-circuit cap to short-circuit the LED indicator lights of the PA/PB ports;
Plug the PC keyboard into the PS2 port.
Use a shorting cap to short PB0 and PS2.SDA.
Use a shorting cap to short PB1 and PS2.SCK
----------------------------------------------------------------------
Precautions:
(1) If you have loaded a library function, please copy the "ICC_H" folder under the "library function" in the root directory of the CD to the D drive.
(2) Please read the “Must-Read” and related materials carefully.
----------------------------------------------------------------------
10101010101010101010101010101010101010101010101010101010101010101010101010101010*/
#include
#include "D:ICC_HCmmICC.H"
#include "D:ICC_HPS2.H"
#define PRESS_DATA_DDR DDRA
#define PRESS_DATA_PORT PORTA
#define SHIFT_DATA_DDR DDRD
#define SHIFT_DATA_PORT PORTD
#define SET_SDA sbi(PORTB,0)
#define CLR_SDA cbi(PORTB,0)
#define GET_SDA gbi(PINB,0)
#define OUT_SDA sbi(DDRB,0)
#define IN_SDA cbi(DDRB,0)
#define SET_SCK sbi(PORTB,1)
#define CLR_SCK cbi(PORTB,1)
#define GET_SCK gbi(PINB,1)
#define OUT_SCK sbi(DDRB,1)
#define IN_SCK cbi(DDRB,1)
#define DELAY() {NOP();NOP();NOP();NOP();}
bool rcvF = 0; //Whether the character flag is received
uint8 keyVal; //key value
/*--------------------------------------------------------------------
Function name: PS2
Function:
Precautions:
Tips:
enter:
return:
--------------------------------------------------------------------*/
void check(void)
{
static uint8 rcvBits = 0; //Number of receptions, number of interruptions
OUT_SCK; //Set "SCK_DDR" to output
DELAY();
SET_SCK; //"SCK_PORT" outputs "1"
DELAY();
IN_SCK; //Set "SCK_DDR" as input
DELAY();
if(!GET_SCK)
{
if((rcvBits>0) && (rcvBits<9))
{
keyVal=keyVal>>1; //Data is in LSB format
//IN_SDA; //When there are attribute settings for the keyboard, pay attention to input and output switching
//DELAY();
if(GET_SDA)
keyVal=keyVal|0x80;
}
rcvBits++;
while(!GET_SCK); //Wait for PS/2CLK to be pulled high
if(rcvBits>10)
{
rcvBits=0; //Receiving 11 times means receiving a frame of data
rcvF=1; //Indicates that a character has been entered
}
}
}
/*--------------------------------------------------------------------
Function name: PS2
Function:
Precautions:
Tips:
enter:
return:
--------------------------------------------------------------------*/
void keyHandle(uint8 val)
{
uint8 i;
static bool isUp=0; //Button release action flag
static bool shift=0; //Shift key pressed flag
rcvF = 0;
PRESS_DATA_PORT = val; //status indication
if(!isUp)
{
switch(val)
{
case 0xF0 : // a release action
isUp = 1;
break;
case 0x12 : // Left shift
shift = 1;
break;
case 0x59 : // Right shift
shift = 1;
break;
default:
if(!shift) // If shift not pressed
{
/*
for(i=0; unshifted[i][0]!=val && unshifted[i][0]; i++)
;
The above writing method is more flexible, but KEIL is not a UNIXC standard and cannot be used.
*/
for(i=0; unshifted[i][0]!=val && i<59; i++)
;
if (unshifted[i][0] == val)
; //Status indication
}
else // If shift pressed
{
/*
for(i=0; unshifted[i][0]!=val && unshifted[i][0]; i++)
;
The above writing method is more flexible, but KEIL is not a UNIXC standard and cannot be used.
*/
for(i=0; shifted[i][0]!=val && i<59; i++)
;
if(shifted[i][0] == val)
SHIFT_DATA_PORT = val; //status indication
}
}
}
else
{
isUp=0;
switch(val)
{
case 0x12 : // Left SHIFT
shift = 0;
break;
case 0x59 : // Right SHIFT
shift = 0;
break;
}
}
}
/*--------------------------------------------------------------------
Function name: PS2
Function:
Precautions:
Tips:
enter:
return:
--------------------------------------------------------------------*/
void main(void)
{
PRESS_DATA_DDR = 0XFF;
SHIFT_DATA_DDR = 0XFF;
IN_SDA;
while(1)
{
check();
if(rcvF)
keyHandle(keyVal);
}
}
Previous article:AVR PS/2 Hardware Interrupt Control Program
Next article:PS/2 Subroutines
Recommended ReadingLatest update time:2024-11-16 11:48
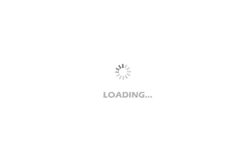
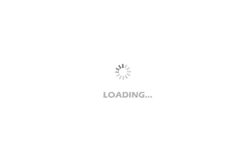
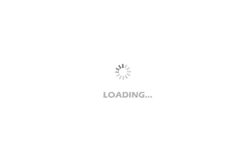
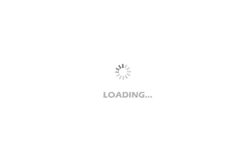
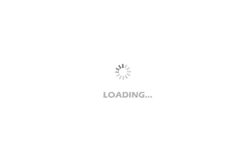
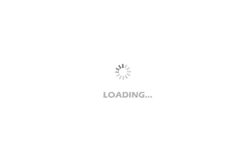
- Popular Resources
- Popular amplifiers
-
Network Operating System (Edited by Li Zhixi)
-
Microgrid Stability Analysis and Control Microgrid Modeling Stability Analysis and Control to Improve Power Distribution and Power Flow Control (
-
MATLAB and FPGA implementation of wireless communication
-
Introduction to Internet of Things Engineering 2nd Edition (Gongyi Wu)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- DIY mini desktop clock using RTT Studio (Part 1) | Create a HelloWorld project based on the STM32 chip
- Share two children's poems, guess how old the author is
- Let’s talk about digital filtering algorithms. Reply to get points!
- GaN power amplifiers revolutionize radar design
- This is an equalizer. I bought it but never used it. I want to share it with you.
- Join the thread and have a chat: If I give you a motor board, what would you want to do?
- 【China Academy of Electronics Science】Recruitment of embedded/algorithm engineers
- Create a healthy life and achieve powerful tools
- Help with the synchronization of the power supply LMZ34002
- How to distinguish diode overcurrent failure and overvoltage failure?