Register Configuration
PCON Power Management Register
Bit number D7 D6 D5 D4 D3 D2 D1 D0
Bit Symbol SM0 SM1 SM2 REN TB8 RB8 TI RI
– Mode Mode Mode 1 directly clears Enable serial port reception Mode 1 receives stop bit Send flag bit, cleared by software Receive flag bit, cleared by software
//Cannot bit address
SCON serial port control register
Bit number D7 D6 D5 D4 D3 D2 D1 D0
Bit Symbol SM0 SM1 SM2 REN TB8 RB8 TI RI
– Mode Mode Mode 1 directly clears Enable serial port reception Mode 1 receives stop bit Send flag bit, cleared by software Receive flag bit, cleared by software
/* DISCON */
sbit SM0 = SCON^7;
sbit SM1 = SCON^6;
sbit SM2 = SCON^5;
sbit REN = SCON^4;
sbit TB8 = SCON^3;
sbit RB8 = SCON^2;
sbit TI = SCON^1;
sbit RI = SCON^0;
Mode & Baud Rate (Song Xuesong P183)
SCON mainly uses mode 1, the baud rate
Correspondingly, use mode 2 of timer T1 & T2
TH1 = TL1 = 256 - Crystal value/12/2/16/Baud rate
(256 is the overflow value of TL1, 12 refers to 12 clock cycles, and 16 is the hardware factor)
SBUF
Two SBUF registers, responsible for receiving and sending buffers respectively
process
Configure the serial port to mode 1
Configure timer T1 to mode 2
Calculate the value of TH0&TL0 according to the baud rate
Configure PCON & SCON registers
Turn on the timer
IO port simulates UART serial communication
Schematic diagram of UART serial port data transmission
Digging a hole: How are the baud rate & TH0 calculated?
#include sbit PIN_RXD = P3^0; sbit PIN_TXD = P3^1; bit RxdOrTxd = 0; bit RxdEnd = 0; bit TxdEnd = 0; unsigned char RxdBuf = 0; unsigned char TxdBuf = 0; void configUART(unsigned long baud); void startRXD(void); void startTXD(unsigned char dat); void main(void) { EA = 1; configUART(9600); while(1) { while(PIN_RXD); startRXD(); while(!RxdEnd); startTXD(RxdBuf+1); while(!TxdEnd); } } void configUART(unsigned long baud) { TMOD &= ~(0xF<<0); TMOD |= 0x1<<1; TH0 = 256 - (11059200 / 12) / baud; } void startRXD(void) { TL0 = 256 - ((256 - TH0) >> 1); ET0 = 1; TR0 = 1; RxdEnd = 0; RxdOrTxd = 0; } void startTXD(unsigned char dat) { TxdBuf = dat; TL0 = TH0; ET0 = 1; TR0 = 1; PIN_TXD = 0; RxdEnd = 0; RxdOrTxd = 1; } void timer0(void) interrupt 1 { static unsigned char cnt = 0; if(RxdOrTxd) { cnt++; if(cnt<=8) { PIN_TXD = TxdBuf & 0x01; TxdBuf >>= 1; } else if(cnt==9) { PIN_TXD = 1; } else { cnt = 0; TR0 = 0; TxdEnd = 1; } } else { if(cnt==0) { if(!PIN_RXD) { RxdBuf = 0; cnt++; } else { TR0 = 0; } } else if(cnt<=8) { RxdBuf >>= 1; if(PIN_RXD) { RxdBuf |= 0x80; } cnt++; } else { cnt = 0; TR0 = 0; if(PIN_RXD) { RxdEnd = 1; } } } } UART serial communication Teaching Edition #include void configUART(unsigned long baud); void main(void) { configUART(9600); while(!RI); RI = 0; SBUF = SBUF + 1; while(!IF); IF = 0; } void configUART(unsigned long baud) { SCON = 0x50; TH1 = 256 - (11059200 / 12 / 2 / 16) / baud; TL1 = TH1; TMOD &= ~(0xF<<4); TMOD |= 0x2<<4; ET1 = 0; TR1 = 1; } Industrial Edition #include void configUART(unsigned long baud); void main(void) { EA = 1; configUART(9600); while(1); } void configUART(unsigned long baud) { SCON = 0x50; TH1 = 256 - (11059200 / 12 / 2 / 16) / baud; TL1 = TH0; TMOD &= ~(0xF<<4); TMOD |= 0x2<<4; ET1 = 0; EN = 1; TR1 = 1; } void UART(void) interrupt 4 { if(RI) { RI = 0; SBUF = SBUF + 1; } if(TI) { IF = 0; } } The computer sends data and displays it in the digital tube #include sbit road = P2^7; sbit want = P2^6; unsigned char code weitable[6] = { ~0x20,~0x10,~0x08,~0x04,~0x02,~0x01 }; unsigned char code duantable[16] = { 0x3F,0x06,0x5B,0x4F,0x66,0x6D,0x7D,0x07, 0x7F,0x6F,0x77,0x7C,0x39,0x5E,0x79,0x71 }; unsigned char ledbuff[6] = { 0x00,0x00,0x00,0x00,0x00,0x00 }; unsigned char T0RH = 0,T0RL = 0; unsigned char RxdByte = 0; void configtimer0(unsigned char ms); void configUART(unsigned long baud); void main(void) { EA = 1; configtimer0(1); configUART(9600); while(1) { ledbuff[0] = duantable[RxdByte & 0x0F]; ledbuff[1] = duantable[RxdByte >> 4]; } } void configtimer0(unsigned char ms) { unsigned long tmp; tmp = 11059200 / 12; tmp = (tmp * ms) / 1000; tmp = 65536 - tmp; tmp = tmp + 12; T0RH = (unsigned char)(tmp>>8); T0RL = (unsigned char)tmp; TH0 = T0RH; TL0 = T0RL; TMOD &= ~(0xF<<0); TMOD |= 0x1<<0; ET0 = 1; TR0 = 1; } void configUART(unsigned long baud) { TH1 = 256 - (11059200 / 12 / 2 / 16) / baud; TL1 = TH0; SCON = 0x50; TMOD &= ~(0xF<<4); TMOD |= 0x2<<4; ET1 = 0; EN = 1; TR1 = 1; } void ledscan(void) { static unsigned char i = 0; P0 = 0x00; want = 1; want = 0; P0 = wideable[i]; wei = 1; wei = 0; P0 = ledbuff[i]; want = 1; want = 0; if(i<5) i++; else i = 0; } void timer0(void) interrupt 1 { TH0 = T0RH; TL0 = T0RL; ledscan(); } void UART(void) interrupt 4 { if(RI) { RI = 0; RxdByte = SBUF; SBUF = RxdByte; } if(TI) { IF = 0; } }
Previous article:51 MCU simple serial communication-1
Next article:51 MCU | Serial communication experiment (simulated serial communication/multi-machine communication example)
Recommended ReadingLatest update time:2024-11-16 13:34
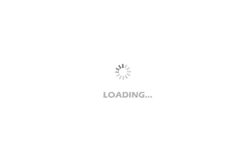
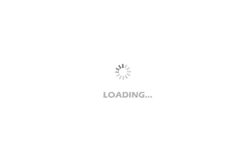
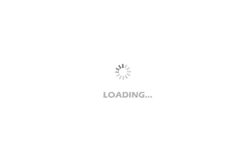
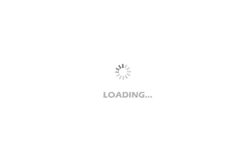
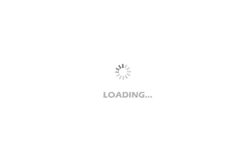
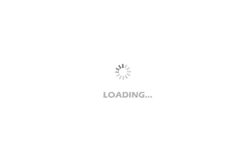
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Fundamentals and Applications of Single Chip Microcomputers (Edited by Zhang Liguang and Chen Zhongxiao)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Design of Intelligent Motor Protector Based on DSP
- Happy Teacher's Day! Let's talk about the teacher you remember most when you were in school!
- Definition of waveform overshoot on sampling resistor in constant current source application
- How to generate such waveform using Verilog?
- 【TI millimeter wave radar evaluation】_2_AWR1843BOOST usage
- Environmental Monitoring System
- Are only foreign companies exempt from overtime work?
- 【micropython】Improved reset to bootloader
- 06 Make GD32L233C expansion board
- The names, wavelengths, characteristics and application fields of each band in the electromagnetic spectrum