In general microcontroller textbooks, there are simple instructions on how to use buzzers. Here we will explain in detail how to write buzzers for different buzzers and different chips.
However, when developing the project, I found that the use of the buzzer in the textbook had great limitations.
In the textbooks, the length of the buzzer is controlled by a busy loop such as delay. However, in reality, this function not only wastes a lot of CPU resources, but also delays the running of other functions. If there are some time-related functions, it will cause time confusion.
In addition, during the buzzer sounding, no operations other than interrupts can be performed. Strictly speaking, except for the infinite loop of the main function, any other busy loop that idles the CPU must be used with caution and the time cannot be too long. Generally speaking, busy loops at the millisecond level are already intolerable.
In addition, this writing method cannot flexibly configure advanced functions such as long or short beeps, and how many times the beeps occur at a certain interval.
However, after checking, I found that turning the buzzer on and off are two different processes.
Therefore, I implemented the buzzer on/off functions and other related functions.
The function of the buzzer on function is to turn on the buzzer, set the time (long beep, short beep, medium beep), and set the number of times.
The function of the buzzer off function is to query the setting value of the on function to decide when to turn off the buzzer, when to turn on the buzzer again, and when to stop turning on the buzzer.
Local global variables control the number of times, and timer global variables control the time.
From the above description, it can be determined that the opening function can be used at will, while the closing function must be looped in the main function to query the setting value.
The following is an example of a function.
1. Necessary initialization
///////////////////Buzzer duration variable declaration//////////////////////
typedef enum //Open time
{
BEEP_SHORT = SEC_01, //100ms
BEEP_MIDDLE = SEC_03, //300ms
BEEP_LONG = SEC_04 //400ms
} BEEP_LAST;
typedef enum //Close interval
{
BEEP_FAST = SEC_03,
BEEP_SLOW = SEC_05
} BEEP_SHUT;
static uint8_t tmp_beep_cnt; //Buzzer count temporary storage
static BEEP_LAST tmp_beep_on; //Buzzer turns on temporary storage
static BEEP_SHUT tmp_beep_period; //temporary storage of buzzer period
2. Buzzer on
/****************************************************************************/
/* Function: buzzer sounds
* describe:
* Parameters: duration of opening, duration of closing (one ring is optional), number of rings (>0)
* return:
* Note: The number cannot be written as 0 */
/****************************************************************************/
void beep_on(BEEP_LAST last, BEEP_SHUT off, uint8_t times)
{
BEEP_CSR |=1<<5; //Turn on the buzzer
freq.beep_on = last; //beep continues
freq.beep_period = last + off; //One on and off cycle
tmp_beep_on = last;
tmp_beep_period = last + off;
if(times > 0)
tmp_beep_cnt = times - 1;
else
tmp_beep_cnt = 0;
}
3. Buzzer off
/****************************************************************************/
/* Function: turn off the buzzer
* Description: Detection time flag, turn it off when it is 0, and reduce the number by 1
* Parameters:
* return:
* Note: This is inside the main loop */
/****************************************************************************/
void beep_off(void)
{
if (freq.beep_on == 0) //If the duration is up, turn off
{
BEEP_CSR &=~(1<<5); //Close
if (tmp_beep_cnt) //The buzzer is turned off, but the count is still there, wait for the conditions to be met to turn it back on
{
if (freq.beep_period == 0) //The buzzer period has expired
{
BEEP_CSR |=1<<5; //Turn on the buzzer
freq.beep_on = tmp_beep_on; //Assign the last duration
freq.beep_off = tmp_beep_period; //Re-count the buzzer period
s_beep_cnt--; //Number of times minus 1
}
}
}
}
Fourth, timer processing, interrupt once every 10ms.
/*Buzzer related timing*/
if (freq.beep_on)
freq.beep_on--; // duration of buzzer on
if(freq.beep_period)
freq.beep_period--; //Buzzer period
Previous article:51 single chip microcomputer - play music with buzzer
Next article:51 single chip microcomputer buzzer "East and West"
Recommended ReadingLatest update time:2024-11-16 12:03
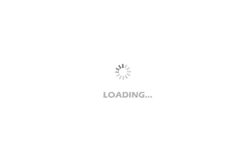
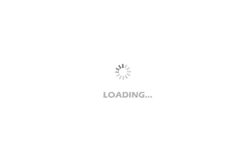
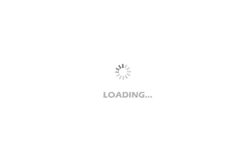
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
Multi-port and shared memory architecture for high-performance ADAS SoCs
-
Teach you to learn 51 single chip microcomputer-C language version (Second Edition) (Song Xuefeng)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Live streaming portal is now open | How TI can develop AI camera applications faster, simpler and at a lower cost
- Thermal imaging reverse upgrade
- Basic circuit diagram of op amp and op amp debugging
- What kind of operation circuit is U2?
- ECO ACTION FAILED
- Wireless street light project——SX1278 debugging
- [GD32E503 Review] Play with GD32E503-uCOSIII operating system transplantation with Demo
- Learning FPGA Universal Logic Verification Platform.pdf
- Tuya Sandwich Wi-Fi & BLE SoC NANO main control board WBRU + Ubuntu development
- 【AT-START-F403A Review】+DHT22 temperature and humidity detection and display