I will not go into details about the concepts of display resolution, pixels, and clarity.
Let's talk about the unfamiliar concept of pitch: it refers to the distance between the centers of two pixels, usually in millimeters by default. For example, p10 refers to an LED dot matrix with a pitch of 10 mm.
LED P00-P07 is directly connected to the processor, but because the processor has limited pins, the following 74HC595 is needed (the processor uses 3 pins to generate 8 parallel signals through this chip, which is equivalent to using 8 pins)
If J13 is jumpered with OE and GND, HC595 will not work. If there is no voltage, jumpered with OE and VCC, it can work.
74HC595 is a shift register. 74HC595 converts serial signals into parallel signals. P34 is the processor input serial signal, P36 is the serial clock, and P35 is the working clock. Then this chip processes and generates 8 parallel signals connected to D0-D7 of the LED matrix.
Here this chip can also control a row of LEDs by connecting the J14 jumper cap in the figure. One end of the LED is high voltage and the other end is 8 serial high and low voltages output by HC595 to light up the LED.
void Hc595SendByte(u8 dat)
{
u8 i = 0, j = 0;
SCK = 0; // Set SCK to the initial state
RCK = 0; // Set RCK to the initial state
for (i=0; i<8; i++) //Use the for loop to send the digital signal 8 times, which is a serial signal
{
SER = dat >> 7;
dat <<= 1;
SCK = 1;
j++; // Delay code, equivalent to nop instruction
j++; // Delay code, equivalent to nop instruction
SCK = 0;
}
RCK = 1;
j++; // Delay code, equivalent to nop instruction
j++; // Delay code, equivalent to nop instruction
}
The principle of LED dot matrix display is similar to the dynamic display of digital tube
First, select each LED pixel to be lit and then refresh it repeatedly.
Link: https://pan.baidu.com/s/1-RGR0v9WUH7LWmOaUTpSbQ
Extraction code: pmw2
This is the font extraction software, which helps you select the LED tubes that need to be lit
Note: You need to run it as an administrator
The font is generated by the CD: tool software/88 font extraction software/LEDDOT V0.2.exe
Note that in the menu bar settings of the mold taking software, the settings are:
Font display mode: single line
Font extraction method: column by column
Font extraction format: C51 format
void main(void)
{
MatrixDisplay(gZhu);
}
void MatrixDisplay(u8 *zimo)
{
u8 i = 0;
while (1)
{
for(i=0;i<8;i++)
{
MATRIX_PORT = gLineCode[i]; // bit selection
Hc595SendByte(zimo[i]); // Send segment selection data
Hc595SendByte(0x00); // Blanking
}
}
}
void Hc595SendByte(u8 dat)
{
u8 i = 0, j = 0;
SCK = 0; // Set SCK to the initial state
RCK = 0; // Set RCK to the initial state
for (i=0; i<8; i++)
{
SER = dat & (0x01);
dat >>= 1;
SCK = 1;
j++; // Delay code, equivalent to nop instruction
j++; // Delay code, equivalent to nop instruction
SCK = 0;
}
RCK = 1;
j++; // Delay code, equivalent to nop instruction
j++; // Delay code, equivalent to nop instruction
}
/*
// Since the module software can only select horizontal and vertical directions, but cannot select vertically from top to bottom or from bottom to top
// The actual test mode of the modulus software is suitable for sending LSB first instead of MSB
void Hc595SendByte(u8 dat)
{
u8 i = 0, j = 0;
SCK = 0; // Set SCK to the initial state
RCK = 0; // Set RCK to the initial state
for (i=0; i<8; i++)
{
SER = dat >> 7;
dat <<= 1;
SCK = 1;
j++; // Delay code, equivalent to nop instruction
j++; // Delay code, equivalent to nop instruction
SCK = 0;
}
The order of sending serial codes here is different from the previous one. This requires actual testing because this order may cause the actual words on the LED to be reversed.
**********************************************************************
* Header file contains
**********************************************************************
*/
#include #include /* ********************************************************************** * Local macro definition ********************************************************************** */ typedef unsigned char u8; // Renaming type u8 simplifies code writing typedef unsigned int u16; #define MATRIX_PORT P0 // Dot matrix LED negative port /* ********************************************************************** * Local global variables ********************************************************************** */ sbit SCK = P3^6; // SCK rising edge shift sbit RCK = P3^5; // RCK rising edge serial output register latch sbit SER = P3^4; // SER pin sends byte data in // Select the value of each element in the array. Select one column u8 gLineCode[]={0xfe,0xfd,0xfb,0xf7,0xef,0xdf,0xbf,0x7f}; /* Font * The font is generated by the CD: tool software/88 font extraction software/LEDDOT V0.2.exe * Note that in the menu bar settings of the mold taking software, the settings are: * Font display mode: single line * Font extraction method: column by column * Font extraction format: C51 format */ u8 gZhu[] = {0x73,0xD6,0x58,0xFF,0x58,0x54,0x52,0x12}; // Chinese character Zhu u8 gSi[] = {0x0,0x4,0x1C,0x24,0x7E,0x4,0x0,0x0}; // number 4 u8 gK[] = {0x0,0x0,0x7E,0x38,0x66,0x0,0x0,0x0}; // Font K /* ********************************************************************** * Function prototype declaration ********************************************************************** */ void Hc595SendByte(u8 dat); void MatrixDisplay(u8 *zimo); /************************************************************************ * Function name: main * Function: Main function * Parameter list: None * Function output: None *************************************************************************/ void main(void) { MatrixDisplay(gZhu); } /************************************************************************ * Function name: MatrixDisplay * Function: Refresh the font to dot matrix in a loop * Parameter list: zimo - array of fonts to be displayed * Function output: None *************************************************************************/ void MatrixDisplay(u8 *zimo) { u8 i = 0; while (1) { for(i=0;i<8;i++) { MATRIX_PORT = gLineCode[i]; // bit selection Hc595SendByte(zimo[i]); // Send segment selection data Hc595SendByte(0x00); // Blanking } } } /************************************************************************ * Function name: Hc595SendByte * Function: Send a byte through 74HC595 serial shift * Parameter list: dat - byte data to be sent * Function output: None *************************************************************************/ void Hc595SendByte(u8 dat) { u8 i = 0, j = 0; SCK = 0; // Set SCK to the initial state RCK = 0; // Set RCK to the initial state for (i=0; i<8; i++) { SER = dat & (0x01); dat >>= 1; SCK = 1; j++; // Delay code, equivalent to nop instruction j++; // Delay code, equivalent to nop instruction SCK = 0; } RCK = 1; j++; // Delay code, equivalent to nop instruction j++; // Delay code, equivalent to nop instruction } /* // Since the module software can only select horizontal and vertical directions, but cannot select vertically from top to bottom or from bottom to top // The actual test mode of the modulus software is suitable for sending LSB first instead of MSB void Hc595SendByte(u8 dat) { u8 i = 0, j = 0; SCK = 0; // Set SCK to the initial state RCK = 0; // Set RCK to the initial state for (i=0; i<8; i++) { SER = dat >> 7; dat <<= 1; SCK = 1; j++; // Delay code, equivalent to nop instruction j++; // Delay code, equivalent to nop instruction SCK = 0; } RCK = 1; j++; // Delay code, equivalent to nop instruction j++; // Delay code, equivalent to nop instruction }
Previous article:The 8x8 dot matrix of the single chip microcomputer allows the number 0 to be displayed in a cycle from right to left
Next article:LED dot matrix of single chip microcomputer
Recommended ReadingLatest update time:2024-11-16 14:52
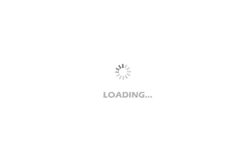
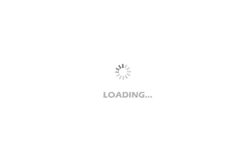
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Application of switch Hall sensor DRV5032 in TWS headset design
- Some learning experiences of NBIoT
- EEWORLD University Hall ---- Digital Signal Processing Beihang Wang Jun
- Application analysis of CC1310 low power consumption advantage
- I am currently using powerSTEP01 to drive a 86mm stepper motor. Does anyone have a driver for this chip?
- C6000 Program Optimization Process and Method
- "Analog input signal" protection circuit
- Simple---a TMS320F28035 key driver
- Reference Design for EtherCAT Slave without DDR
- Crystal Oscillator PPM Small Parameters, Big Effect