void main()//main function
{
delay1ms(1000);
lcd_init();//LCD display initialization
init_play();
while(1)
{
EA=0; //Since DS18B20 has high timing requirements and is affected by interrupts, turn off the general interrupt first
read_temp();//Read temperature
ds1820disp();//Display
CT_init();//Timer counter initialization
EA=1;//Open general interrupt
EX0=1;//Allow external interrupt
IT0=1;//Set external interrupt mode to falling edge trigger
P3=0xff;
t0=(u*65536+x)*(12/22.1);//Calculate pulse time width (unit: ms)
f=1000000/(2*t0);//Calculate frequency
if(f>8000&&tflag==1)
init_play1(); //Display Frozen
else init_play2(); //Display Not frozen
}
}
This is the main function. I initially suspect that the interruption of measuring frequency affects the reading of data by the temperature sensor. How to solve it?
Method 1: If the real-time requirement of reading frequency is not high, just turn this on and off, take turns, and there is no other trick.
First measure the temperature for 1 second, then measure the frequency for 1 second, and then measure the temperature for 1 second?
Method 2: I will give you a reference program, which is also single-bus data acquisition (clock 11.0592).
Do not turn off interrupts in your main loop. When calling to read temperature, there is a checksum to determine the validity of the data (if there is an interrupt in the middle of the temperature reading program, the data may be invalid).
#include
#include "public.h"
sbit DS2401Bus = P3^5;
//DS2401.c
// First reset the single bus:
// The bus remains low for more than 480us; all devices on the bus will reset
// The master releases the bus and enters receive mode
// The slave waits for 15-60us, then pulls the bus low for 60-240us to generate a response pulse
// Write 0x33H command: Only suitable for single node (read ROM[0x33] command)
// All write (0 or 1) time slots require at least 60us and at least 1us recovery time is required between two independent write time slots
// Both write time slots start with the master pulling the bus low
// Write 1 time slot: After pulling the bus low, the master must release the bus within 15us, and the bus is pulled to a high level by a 5k pull-up resistor
// Write 0 time slot: After pulling the bus low, the master only needs to keep the low level for at least 60us during the entire time slot
// -- During the period of 15-60us after the start of the write time slot, the single-bus device samples the bus level state (0 or 1)
// Read time slot
// The device transmits data to the host only when the host issues a read time slot. So after the host issues a read data command, a read time slot must be generated immediately
. // All read time slots require at least 60us and at least 1us of recovery time is required between two independent read time slots.
// Each read time slot is initiated by the host, which pulls the bus down for at least 1us.
// The single-bus device starts sending 0 or 1 on the bus only
after the host initiates a read time slot. // The data sent by the slave remains valid for 15us after the start time slot.
// Therefore, the host must release the bus during the read time slot and sample the bus status within 15us after the start of the time slot.
//
#pragma ot(4,SPEED)
void delay_500us(void)
{
unsigned char data i;
for(i = 0; i < 56; i++);
}
void delay_250us(void)
{
unsigned char data i;
for(i = 0; i < 28; i++);
}
void delay_90us(void)
{
unsigned char data i;
for(i = 0; i < 9; i++) ;
}
void delay_60us(void)
{
unsigned char data i;
for(i = 0; i < 6; i++) ;
}
unsigned char InitDS2401(void) //Reset DS2401
{
unsigned char tmp;
DS2401Bus = 0; // Output: 0; the bus remains at a low level for more than 480us
delay_500us(); // 498us
DS2401Bus = 1; // Release the bus, and the 5k pull-up resistor pulls the bus to a high level
delay_60us(); // 64us, wait for the device to send a response pulse
tmp = DS2401Bus; // Check the response pulse
delay_250us(); // 255us, delay more than 240us
if(tmp) // Read data
return 0;
else
return 1;
}
void WriteDS2401(unsigned char d)//Write 2401 command
{
unsigned char i;
for(i=0;i<8;i++) {
DS2401Bus = 0; // After pulling the bus low, the host must release the bus within 15us_nop_
(); _nop_(); _nop_(); // Each nop is estimated to take 1.085us
if(d & 1) { // Output: data bit
DS2401Bus = 1;
}
d >>= 1;
delay_60us(); // At least keep 60us
DS2401Bus = 1; // Pull the bus to a high level, at least 1us recovery time is required_nop
_();_nop_();_nop_();
}
}
unsigned char ReadDS2401() //Read 2401 data
{
unsigned char i,d;
for(i=0;i<8;i++) {
d >>= 1;
DS2401Bus = 0; // At least pull the bus low for 1us
_nop_();_nop_();_nop_();
DS2401Bus = 1; // Release bus_nop
_();_nop_();_nop_();_nop_();_nop_();
if(DS2401Bus == 1) d |= 0x80; // Read data
delay_90us(); // 90us
}
return d;
}
unsigned char crctest() //Perform CRC check
{
unsigned char i1, i2, crc=0;
for(i1=0; i1<8; i1++) {
crc ^= DS2401IDBuf[i1];
for(i2=0; i2<8; i2++) {
if (crc & 0x01)
crc = (crc >> 1) ^ 0x8C;
else
crc >>= 1;
}
}
return (crc);
}
unsigned char ReadRS2401ID() //Read out DS2401's
{
unsigned char i;
if (!InitDS2401()) return(0);
WriteDS2401(0x33);
for(i=0;i<8;i++) DS2401IDBuf[i]=ReadDS2401();
if (crctest() != 0) return(0);
return(1); //CRC check successful
}
Previous article:51 MCU Smart Home Remote Control
Next article:51 MCU + K9F2080U0A to make MP3 circuit diagram and source program
Recommended ReadingLatest update time:2024-11-16 11:51
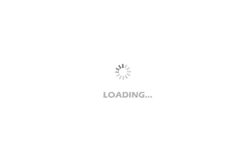
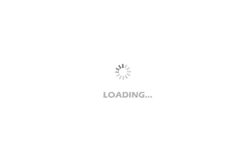
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- ADI Live Show starts at 10:00 this morning | Multi-parameter optical water quality analysis platform
- Qorvo Online Design Conference丨5G Solutions Supporting NAD and Telematics Unit Applications
- AT24C1024 full capacity read and write NXPLPC11XX source program
- MLX90614 infrared temperature sensor manual and forehead temperature gun MCU reference design
- How to enable ADC group and DMA in STM32F030
- Talk about ARM architecture and programming model
- The problem of the transistor emitter voltage in the figure
- Practical case of switching power supply EMI rectification
- Principle and application of relay protection in power system
- I used a better board, but I didn’t expect DDR4 to be…???