Series Function
The functions are defined in arch/arm/mach-s3c2410/gpio.c, and the related macros are defined in include/asm-arm/arch-s3c2410/regs-gpio.h
(1) void s3c2410_gpio_setpin(unsigned int pin, unsigned intto);
Set the output value of the corresponding GPIO port, for example:
pin=S3C2410_GPG2, to=0, then set the output value of S3C2410_GPG2 to 0;
pin=S3C2410_GPG2, to=1, then set the output value of S3C2410_GPG2 to 1.
Function definition:
viewplain
void s3c2410_gpio_setpin(unsigned int pin, unsigned int to)
{
void __iomem *base = S3C2410_GPIO_BASE(pin);
unsigned long offs = S3C2410_GPIO_OFFSET(pin);
unsigned long flags;
unsigned long dat;
local_irq_save(flags);
dat = __raw_readl(base + 0x04);
dat &= ~(1 << offs);
dat |= to << offs;
__raw_writel(dat, base + 0x04);
local_irq_restore(flags);
}
EXPORT_SYMBOL(s3c2410_gpio_setpin);
(2) unsigned int s3c2410_gpio_getpin(unsigned intpin);
Get the value of the corresponding GPIO port.
Function definition:
viewplain
unsigned int s3c2410_gpio_getpin(unsigned int pin)
{
void __iomem *base = S3C2410_GPIO_BASE(pin);
unsigned long offs = S3C2410_GPIO_OFFSET(pin);
return __raw_readl(base + 0x04) & (1<< offs);
}
EXPORT_SYMBOL(s3c2410_gpio_getpin);
(3) void s3c2410_gpio_cfgpin(unsigned int pin, unsigned int function);
Set the working mode of the corresponding GPIO port, input, output, interrupt, etc.
Function definition:
viewplain
void s3c2410_gpio_cfgpin(unsigned int pin, unsigned int function)
{
void __iomem *base = S3C2410_GPIO_BASE(pin);
unsigned long mask;
unsigned long con;
unsigned long flags;
if (pin < S3C2410_GPIO_BANKB) {
mask = 1 << S3C2410_GPIO_OFFSET(pin);
} else {
mask = 3 << S3C2410_GPIO_OFFSET(pin)*2;
}
local_irq_save(flags);
con = __raw_readl(base + 0x00);
con &= ~mask;
con |= function;
__raw_writel(con, base + 0x00);
local_irq_restore(flags);
}
EXPORT_SYMBOL(s3c2410_gpio_cfgpin);
(4) unsigned int s3c2410_gpio_getcfg(unsigned intpin);
Get the working mode of the corresponding GPIO port, including input, output, interrupt, etc.
Function definition:
viewplain
unsigned int s3c2410_gpio_getcfg(unsigned int pin)
{
void __iomem *base = S3C2410_GPIO_BASE(pin);
unsigned long mask;
if (pin < S3C2410_GPIO_BANKB) {
mask = 1 << S3C2410_GPIO_OFFSET(pin);
} else {
mask = 3 << S3C2410_GPIO_OFFSET(pin)*2;
}
return __raw_readl(base) & mask;
}
EXPORT_SYMBOL(s3c2410_gpio_getcfg);
(5) voids3c2410_gpio_pullup(unsigned int pin, unsigned intto);
Set the level of the corresponding GPIO port, for example:
pin=S3C2410_GPG2, to=0, then pull down S3C2410_GPG2, that is, set the value of S3C2410_GPG2 to 0;
pin=S3C2410_GPG2, to=1, then pull up S3C2410_GPG2, that is, set the value of S3C2410_GPG2 to 1.
Function definition:
viewplain
void s3c2410_gpio_pullup(unsigned int pin, unsigned int to)
{
void __iomem *base = S3C2410_GPIO_BASE(pin);
unsigned long offs = S3C2410_GPIO_OFFSET(pin);
unsigned long flags;
unsigned long up;
if (pin < S3C2410_GPIO_BANKB)
return;
local_irq_save(flags);
up = __raw_readl(base + 0x08);
up &= ~(1L << offs);
up |= to << offs;
__raw_writel(up, base + 0x08);
local_irq_restore(flags);
}
EXPORT_SYMBOL(s3c2410_gpio_pullup);
(6) unsigned int s3c2410_modify_misccr(unsigned int clear,unsigned int change);
Miscellaneous settings, set register MISCCR, see the function definition for details.
Function definition:
viewplain
unsigned int s3c2410_modify_misccr(unsigned int clear, unsigned int change)
{
unsigned long flags;
unsigned long misccr;
local_irq_save(flags);
misccr = __raw_readl(S3C2410_MISCCR);
misccr &= ~clear;
misccr ^= change;
__raw_writel(misccr, S3C2410_MISCCR);
local_irq_restore(flags);
return misccr;
}
EXPORT_SYMBOL(s3c2410_modify_misccr);
(7) int s3c2410_gpio_getirq(unsigned intpin);
Get the interrupt number corresponding to the corresponding GPIO port.
Function definition:
viewplain
int s3c2410_gpio_getirq(unsigned int pin)
{
if (pin < S3C2410_GPF0 || pin > S3C2410_GPG15_EINT23)
return -1;
if (pin < S3C2410_GPG0 && pin > S3C2410_GPF7)
return -1;
if (pin < S3C2410_GPF4)
return (pin - S3C2410_GPF0) + IRQ_EINT0;
if (pin < S3C2410_GPG0)
return (pin - S3C2410_GPF4) + IRQ_EINT4;
return (pin - S3C2410_GPG0) + IRQ_EINT8;
}
EXPORT_SYMBOL(s3c2410_gpio_getirq);
(8) int s3c2410_gpio_irqfilter(unsigned int pin, unsigned int on, unsigned int config);
Interrupt filter configuration, see the function definition for details.
Function definition:
viewplain
int s3c2410_gpio_irqfilter(unsigned int pin, unsigned int on,
unsigned int config)
{
void __iomem *reg = S3C2410_EINFLT0;
unsigned long flags;
unsigned long val;
if (pin < S3C2410_GPG8 || pin > S3C2410_GPG15)
return -1;
config &= 0xff;
pin -= S3C2410_GPG8_EINT16;
reg += pin & ~3;
local_irq_save(flags);
val = __raw_readl(reg);
val &= ~(0xff << ((pin & 3) * 8));
val |= config << ((pin & 3) * 8);
__raw_writel(val, reg);
val = __raw_readl(S3C2410_EXTINT2);
val &= ~(1 << ((pin * 4) + 3));
val |= on << ((pin * 4) + 3);
__raw_writel(val, S3C2410_EXTINT2);
local_irq_restore(flags);
return 0;
}
EXPORT_SYMBOL(s3c2410_gpio_irqfilter);
Previous article:Make the mnt folder of the arm development board visible as a mount in the Linux virtual machine
Next article:ARM for ADS1.2
Recommended ReadingLatest update time:2024-11-16 13:45
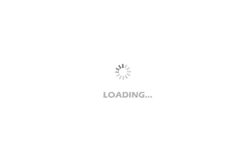
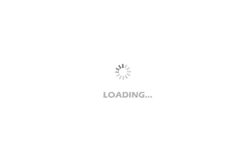
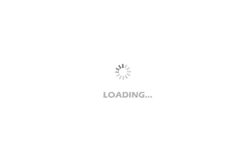
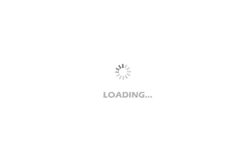
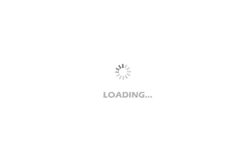
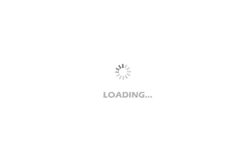
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Product Design Guide
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- This circuit for detecting mobile phones is no longer usable, right?
- STM32 stepper motor trapezoidal acceleration and deceleration program
- What is the way to distinguish whether series resonance is good or bad?
- Improved Snowy Night Christmas Tree Program
- National Geographic China and "Beautiful China - China's Most Beautiful Places Selection Event"
- stm32 does not recognize chip ID using keil+stlink
- I would like to recommend a domestic MCU Gaojing GOF to replace STM32F103C8T6
- Switch-Mode Power Supply Current Sensing: How to Place the Sense Resistor
- (Bonus 4) GD32L233 Evaluation - Obtaining and Printing the Main Frequency of System Resources
- Wanted STM32H750