II. C/C++ Options
Among the following five options, C/C++ is the most important, so some functions need to be emphasized. Looking at the option title "C/C++", it is mainly aimed at C/C++, which is similar to the next option "Asm".
No. 1: Preprocessor Symbols
The main thing here is to predefine functions, which is equivalent to #define xxxx in the program. I predefine STM32F10X_HD above, so there is no need to define it in the stm32f10x.h file.
Part 2: Language / Code Generation
Language/code Generation Language code generation can be understood as compiling, linking, and finally generating code. This part of the function is more important for code optimization. Beginners do not need to understand it too much, but those who have higher requirements for code size, running speed, etc. need to understand it in depth.
Execute only Code: Generate only execution code;
[Set compiler command line: --execute_only]
Generate execution code only prevents the compiler from generating any data access code sections.
Optimize: Optimize options, there are four options from Level0 to Level3.
[Set compiler command line: -Onum]
For beginners and online debugging, it is recommended to use Level 0, that is, no optimization, so that the execution effect is the same as the code. If configured to Level 3, online debugging may optimize some places and fail to breakpoint.
Optimize for Time: Optimize time, that is, optimize the time-consuming parts of the code.
[Set compiler command line: -Otime] --split_sections
For example, some algorithms have a large amount of code and take a long time to run (for example, 2 seconds). If you check this function, you will find that the running time is significantly reduced (perhaps less than 1 second).
Split Load and Store Multiple: Load and store multiple splits.
[Set compiler command line: --split_ldm]
Non-aligned data is accessed multiple times. When more than 4 LMD/STM instructions are generated, the LMD and STM instructions are split to reduce the interrupt delay.
One ELF Section per Function: Optimize each function ELF segment (it is recommended to check both).
[Set compiler command line: --split_sections]
Each function will generate an ELF segment. Check this option to allow optimization of each ELF segment. This option can reduce potential shared addresses, data and strings between functions.
To put it simply: the code size ROM can be reduced (memory RAM will not be reduced).
For example, the storage size after compilation is compared before and after checking this option:
Before checking:
Program Size: Code=2540 RO-data=336 RW-data=40 ZI-data=1024
After checking:
Program Size: Code=908 RO-data=320 RW-data=40 ZI-data=1024
Strict ANSI C: Standard (strict) ANSC.
[Set compiler command line: --strict]
That is to say: during compilation, it is strictly checked according to the ANSI C standard.
Enum Container always int: Enumeration is always int type
[Set compiler command line: --enum_is_int]
It is easy to understand that the member variable type when we enumerate is int.
Plain Char is Signed: Plain Char is Signed.
[Set compiler command line: --signed_chars]
Code example: char a[] = "abcd"; that is, mark "abcd" as character type.
Read-Only Position Independent: Generates an independent code space for constants.
[Set compiler command line: --apcs=/ropi]
For example: we define font variables as constants, and checking this option will place these font variables in a separate code space.
Read-Write Position Independent: Generates an independent code space for readable and writable code.
[Set compiler command line: --apcs=/rwpi]
Warnings: Warnings
[No Warnings set compiler command line: -W]
No Warnings: There will be no warning prompts or outputs;
All Warnings: All warning prompts and outputs.
Thumb Mode: Thumb mode.
Specifies to set the file or folder (group) to Thumb mode.
[Note: This mode is the default in the project, which means it cannot be selected]
No Auto Includes: Do not automatically add header files (usually not checked).
If you do not check this option, the compiler will search for the .h files in your project in the Keil installation path.
For example: we define uint8_t in the stdint.h file, but there is usually no stdint.h file in our project directory. At this time, the compiler will look for the stdint.h file in the Keil path.
C99 Mode: C99 standard mode.
[Set compiler command line: --c99]
C speech has multiple versions of standards, such as C89, C90, C99, etc.
No. 3: Include Paths
The include path is a must-know item when using Keil (and similar) software. The include path specifies the location of the files used in our project so that the compiler can find the corresponding files. It is a must-know item for both beginners and advanced software engineers.
This option has been described in the previous article "Keil (MDK-ARM) Series Tutorial (I)_Detailed Process of Creating a New Software Project". It is relatively easy to understand and simple.
Section 4: Misc Controls
Specifies that there is no separate dialog control. For example, error messages are displayed in Japanese. [Not commonly used]
No. 5: Compiler control string
This is the name of the compiler execution, showing the current compiler command line instructions.
In the above "2nd place: Language code generation" there is a bracket [Set the compiler command line:]
The names inside are shown here.
III. Asm Option
From the layout and structure of this option, we can know that it is similar to the previous option "C/C++", but this one is for Asm. Therefore, this section will only briefly describe it without emphasizing it. You can refer to the previous section for comparison. The 3rd, 4th, and 5th are the same as the C/C++ option, so they are not described here.
No. 1: Conditional Assembly Control Symbols
Specify assembly conditions, which is similar to the preprocessing in the C/C++ options in the previous section.
Part 2: Language / Code Generation
Similar to the previous chapter.
Read-Only Position Independent: Generates an independent code space for constants.
Read-Write Position Independent: Generates an independent code space for readable and writable code.
Thumb Mode: Thumb mode.
Split Load and Store Multiple: Load and store multiple splits.
Execute only Code: Generate only execution code;
No Auto Includes: Do not automatically add header files (usually not checked).
IV. Linker Options
This option is Linker Link, which is the linker configuration option. You can modify, edit and view the linked files. The 1st and 2nd are the key points. The 3rd and 4th are the same as the C/C++ options, so they are not discussed here.
The first place: Use Memory Layout from Target Dialog to load the Target page of the dialog box using a scatter file (Use Memory Layout from Target Dialog)
Make RW Sections Position Independent: Make the RW segments independent.
[Set compiler command line: --rwpi]
When enabled: The variable area (including RW and ZI) has independent addresses.
When disabled: The variable area (including RW and ZI) is located at an absolute memory address.
Make RO Sections Position Independent: Make RO sections independent.
[Set compiler command line: --ropi]
When enabled: Constant and Code Region (RO) have separate addresses.
When disabled: Constants and code regions (RO) are located at absolute memory addresses.
Don't Search Standard Libraries: Do not search the standard library.
[Set compiler command line: --noscanlib]
Disables scanning of the default compiler runtime library.
Report 'might fail' Conditions as Errors: Report 'might fail' conditions as errors.
[Set compiler command line: --strict]
Conditions that could result in failure are reported as errors, not warnings.
X/O Base: X/O base address.
[Set compiler command line: --xo_base=address]
R/O Base: R/O base address.
[Set compiler command line: --ro_base=address]
R/W Base: R/W base address.
[Set compiler command line: --rw_base=address]
disable Warnings: Police warnings.
[Set compiler command line: --diag_suppress]
Part 2: Scatter File
Here you can load, view and edit scattered files. Click the three dots "..." at the back to load the file; click "Edit..." to view and edit the corresponding file.
V. Debug options
This option is more important, and is mainly used for (software simulation, hardware online) debugging. Since the configuration interface of software simulation and hardware online debugging is basically the same, and now we basically use hardware online debugging, therefore, only the hardware online debugging interface is selected for description.
Step 1: Select hardware online debugging
There is no need to say much about the choice of download debugger, mainly to talk about the "Setting" later. Many people often use J-Link to download debugger, and when debugging STM32, you can use the four-wire SWD mode. If you use J-Link for SWD debugging. At this time, you need to select the "SW" mode in "Setting", as follows:
Step 2: Select hardware online debugging
Load Application at Startup: Load the application at startup.
Run to main(): The program executes to the main() function.
When entering debug mode, the program automatically runs to the main function.
Initialization File: Load and edit initialization file.
This can be used in some cases, such as debugging code in RAM. You can refer to my article: STM32 internal RAM online debugging configuration method and detailed instructions (based on Keil development tools).
Part 3: Restore Debug Session Settings
Here, reset settings means to restore settings. If checked, click "Reset" to restore to the previous state. Including: breakpoints, watch windows, performance analyzer, memory window, toolbox, system viewer, etc.
No. 4: DLL file (best default)
The configuration here belongs to Keil's own configuration and it is best not to modify it.
CPU/Driver DLL - Parameter: CPU driver files and parameters.
Dialog DLL - Parameter: Dialog box DLL file and parameters.
Part 5: Managing component description files
Manage Component Viewer Description Files
There is generally no need to manage here.
VI. Utilities option
This chapter contains common options and is relatively simple.
Previous article:Keil (MDK-ARM) series tutorial (three)_project target option configuration (I)
Next article:Keil (MDK-ARM) series tutorial (V)_Configuration (I)
Recommended ReadingLatest update time:2024-11-16 19:32
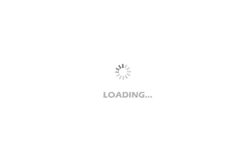
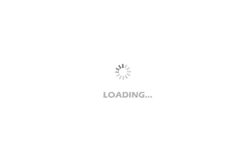
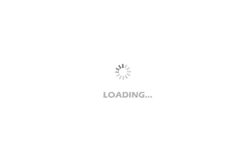
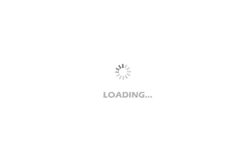
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Just to meet you, Fuchang Electronics WeChat recruitment is now online
- Begging
- EEWORLD University ---- FPGA design and FPGA application based on Verilog HDL (Intel official tutorial)
- Showing off the goods + the first wave of development boards
- RF Circuit Engineering Design
- What is the role of the pull-up resistor? How to choose the value of the pull-up resistor
- How to obtain additional information of Pingtouge scenario-based Bluetooth MESH
- I have encountered a problem with the iTOP4418 development board. Is there any big guy who can help me?
- FLASH cannot be downloaded
- [GD32E231 DIY Contest] 3. Timer + button (supports long press and short press) + LED