To understand RO, RW and ZI you need to first understand the following:
1. Composition of ARM program:
The "ARM program" mentioned here refers to the program being executed in the ARM system, not the bin image file saved in ROM. Please pay attention to the difference.
An ARM program consists of 3 parts: RO, RW and ZI. RO is the instruction and constant in the program; RW is the initialized variable in the program; ZI is the uninitialized variable in the program.
From the above 3 points, it can be understood that RO is readonly, RW is read/write, and ZI is zero
. 2. Composition of ARM image file
The so-called ARM image file refers to the bin file burned into ROM, also called image file. It is called Image file below.
Image files contain RO and RW data. The reason why image files do not contain ZI data is that ZI data is all 0, so there is no need to include it. Just clear the area where ZI data is located before running the program. Including it will waste storage space.
Q: Why must RO and RW be included in the Image? } Prog2: Code RO Data RW Data ZI Data Debug =====================================================
A: Because the instructions and constants in RO and the variables initialized in RW cannot be created out of nothing like ZI.
3. The execution process of the ARM program
From the above two points, we can know that the image file burned into the ROM is not exactly the same as the ARM program in actual operation. Therefore, it is necessary to understand how the ARM program reaches the actual running state from the image in the ROM. In fact
, the instructions in RO should at least have the following functions:
1. Move RW from ROM to RAM, because RW is a variable and variables cannot exist in ROM.
2. Clear all RAM areas where ZI is located, because the ZI area is not in the Image, so the program needs to clear the corresponding RAM area according to the ZI address and size given by the compiler. ZI is also a variable. Similarly: variables cannot exist in ROM. In the
initial stage of program operation, the C program can access variables normally only after the instructions in RO complete these two tasks. Otherwise, only code without variables can be run.
After all the above, you may still be confused. What are RO, RW and ZI? Below I will give a few examples to explain what RO, RW and ZI mean in C.
1. RO
Look at the following two programs. There is a statement between them, which is to declare a character constant. Therefore, according to what we said before, there should only be one byte difference in RO data (character constant is 1 byte).
Prog1:
#include
void main(void)
{
;
#include
const char a = 5;
void main(void)
{
;
}
The compiled information of Prog1 is as follows:
=====================================================
948 60 0 96 0 Grand Totals
Total RO Size(Code + RO Data) 1008 ( 0.98kB)
Total RW Size(RW Data + ZI Data) 96 ( 0.09kB)
Total ROM Size(Code + RO Data + RW Data) 1008 ( 0.98kB)
=======================================================
The information compiled by Prog2 is as follows:
=====================================================
Code RO Data RW Data ZI Data Debug
948 61 0 96 0 Grand Totals
===================================================
Total RO Size(Code + RO Data) 1009 ( 0.99kB)
Total RW Size(RW Data + ZI Data) 96 ( 0.09kB)
Total ROM Size(Code + RO Data + RW Data) 1009 ( 0.99kB)
===================================================
From the information after the above two programs are compiled, we can see that:
The RO of Prog1 and Prog2 contains two types of data: Code and RO Data. The only difference between them is that the RO Data of Prog2 has one more byte than that of Prog1. This is consistent with the previous speculation.
If an instruction is added instead of a constant, the result should be a difference in the size of the Code data.
2. RW
Again, look at the two programs again. The only difference between them is an "initialized variable". According to what has been said before, the initialized variable should be counted in RW, so the size of RW should be different between the two programs.
Prog3:
#include
void main(void)
{
;
}
Prog4:
#include
char a = 5;
void main(void)
{
;
}
The information after Prog3 is compiled is as follows:
======================================================
Code RO Data RW Data ZI Data Debug
948 60 0 96 0 Grand Totals
=========================================================
Total RO Size(Code + RO Data) 1008 ( 0.98kB)
Total RW Size(RW Data + ZI Data) 96 ( 0.09kB)
Total ROM Size(Code + RO Data + RW Data) 1008 ( 0.98kB)
======================================================
The information compiled by Prog4 is as follows:
======================================================
Code RO Data RW Data ZI Data Debug
948 60 1 96 0 Grand Totals
=========================================================
Total RO Size(Code + RO Data) 1008 ( 0.98kB)
Total RW Size(RW Data + ZI Data) 97 (0.09kB)
Total ROM Size(Code + RO Data + RW Data) 1009 (0.99kB)
==================================================
It can be seen that there is only one byte difference between Prog3 and Prog4 in RW Data, which is caused by an initialized character variable "a".
3. ZI
Looking at the two programs again, the difference between them is an uninitialized variable "a". From the previous understanding, it can be inferred that the only difference between the two programs should be the size of ZI.
Prog3:
#include
void main(void)
{
;
}
Prog4:
#include
char a;
void main(void)
{
;
}
The information after Prog3 is compiled is as follows:
========================================================
Code RO Data RW Data ZI Data Debug
948 60 0 96 0 Grand Totals
============================================================
Total RO Size(Code + RO Data) 1008 ( 0.98kB)
Total RW Size(RW Data + ZI Data) 96 ( 0.09kB)
Total ROM Size(Code + RO Data + RW Data) 1008 ( 0.98kB)
==========================================================
The information compiled by Prog4 is as follows:
========================================================
Code RO Data RW Data ZI Data Debug
948 60 0 97 0 Grand Totals
============================================================
Total RO Size(Code + RO Data) 1008 ( 0.98kB)
Total RW Size (RW Data + ZI Data) 97 ( 0.09kB) Total ROM Size
(Code + RO Data + RW Data) 1008 ( 0.98kB )
=== ... Appendix: Program compilation command (assuming the C program is named tst.c): armcc -c -o tst.o tst.c armlink -noremove -elf -nodebug -info totals -info sizes -map -list aa.map -o tst.elf The compiled information of tst.o is in the aa.map file. ROM mainly refers to: NAND Flash, Nor Flash RAM mainly refers to: PSRAM, SDRAM, SRAM, DDRAM
Previous article:A red cross appears in MDK, but the program compiles normally without errors
Next article:Description of the FLASH size occupied by the bin file generated after MDK compilation
Recommended ReadingLatest update time:2024-11-16 15:56
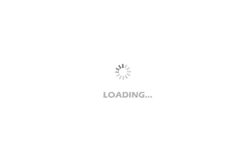
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Problems with ADS1220
- Why do smart water meters use disposable 17450 (or other models) disposable lithium batteries? Why not use rechargeable batteries?
- 【Development and application based on NUCLEO-F746ZG motor】15. Mathematical model - voltage equation and electromagnetic torque equation
- Playing with Zynq Serial 42——[ex61] Image Laplacian Sharpening Processing of OV5640 Camera
- Snake moving in a twisting motion
- Qorvo's leading RF solutions
- Shanghai Hangxin ACM32F070 development board + serial port
- I'm a newbie and would like to ask for the code of an electronic combination lock!
- Purgatory Legend-Generate War.pdf
- In FPGA design, timing is everything