Since the speed of STC microcontroller is faster than 8051, it belongs to 1 clock/machine cycle high-speed microcontroller. The high speed is of course its advantage, but for transplanting traditional 8051 programs, it is necessary to modify the timing to realize a certain function.
The following program is a simple driver for ds18b20. It was downloaded from the Internet. After a slight modification, it was debugged in STC12C5A32S2. Finally, the temperature is read and returned as an unsigned int. The lower 12 bits are the temperature data. The actual temperature value can be obtained by performing calculations in the main program. Hehe~~
/***********ds18b20 subroutine****************************/
/***********ds18b20 delay sub-function (crystal oscillator 11.0592MHz)*******/
void delay_18B20(unsigned int i)
{
while(i--);
}
/**********ds18b20 initialization function*************************/
void Init_DS18B20(void)
{
unsigned char x=0;
DQ = 1; //DQ reset
delay_18B20(80); //Slight delay
DQ = 0; //MCU pulls DQ low
delay_18B20(800); //Precise delay greater than 480us
DQ = 1; //Pull the bus high
delay_18B20(140);
x=DQ; delay_18B20(200);
}
/***********ds18b20 reads a byte**************/
unsigned char ReadOneChar(void)
{
uchar i=0;
uchar dat = 0;
for (i=8;i>0;i--)
{
DQ = 0; // give pulse signal
dat>>=1;
DQ = 1; // give pulse signal
if(DQ)
dat|=0x80;
delay_18B20(40); //40
}
return(dat);
}
/*************ds18b20 writes a byte****************/
void WriteOneChar(uchar dat)
{
unsigned char i=0;
for (i=8; i>0; i--)
{
DQ = 0;
DQ = dat&0x01;
delay_18B20(50); //50
DQ = 1;
dat>>=1;
}
}
/**************Read the current temperature of ds18b20************/
unsigned int ReadTemp(void)
{
unsigned char a=0;
unsigned char b=0;
unsigned int temp_value=0;
Init_DS18B20();
WriteOneChar(0xCC);
WriteOneChar(0x44);
delay_18B20(1000);
Init_DS18B20();
WriteOneChar(0xCC);
WriteOneChar(0xBE);
delay_18B20(1000);
a=ReadOneChar(); //Read the low bit of the temperature value
b=ReadOneChar(); //Read the high bit of the temperature value
temp_value = b<<8;
temp_value |= a;
return temp_value;
}
Previous article:STC15F2K60S2 reads DS18B20 temperature serial port display
Next article:STC15F2K60S2 serial communication program
Recommended ReadingLatest update time:2024-11-16 20:52
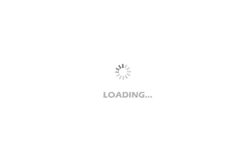
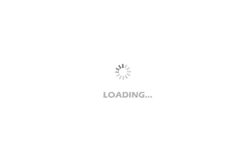
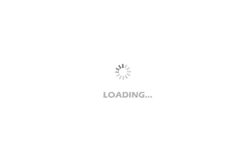
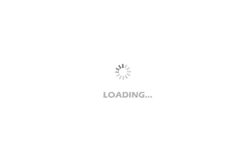
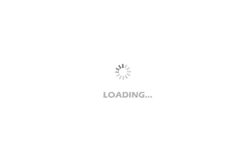
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- DSP C6000 Peripherals
- Can 5 GHz iFEM really speed up the launch of Wi-Fi 6 home mesh products?
- Operational Amplifier
- [RVB2601 Creative Application Development] Environmental Monitoring Terminal 05- Temperature and Humidity Collection and Display
- Principles of Radar (5th Edition)
- Current Sensing Using Nanopower Op Amps
- Looking for a microcontroller model
- Is the STM32 library function HAL_UART_Receive blocking?
- 【DIY Creative LED V2】Complete program
- The Engineer's Way of Quanhui, the author of "FPGA Timing Constraints and Analysis"