Sometimes I always wonder, what is the best way to learn? Just like learning USB, is it enough to just learn how to apply it, or do I have to go deep into the protocol content and the underlying driver? I often tell others that I know something, but in fact I only know a little bit, and I only know some applications to fool others. So I always comfort myself: I just need to make some applications!!!
The following introduces the two files usb_endp.c and usb_istr.c of the STM32 USB project.
First is usb_endp.c. This file is very simple. It just defines several endpoint input and output functions. My project only has it.
uint8_t USB_Receive_Buffer[REPORT_COUNT]; //Endpoint receives data buffer REPORT_COUNT=64
uint8_t USB_Send_Buffer[REPORT_COUNT]; //Endpoint sends data buffer
volatile uint8_t USB_Received_Flag=0; //USB received data flag
/****************************************************** ****************************
* Function Name: EP1_IN_Callback.
* Description: Endpoint 1 input callback function
* Input : None.
* Output : None.
* Return : None.
*************************************************** *******************************/
void EP1_IN_Callback(void)
{
}
/****************************************************** ****************************
* Function Name: EP1_OUT_Callback.
* Description: Endpoint 1 output callback function
* Input : None.
* Output : None.
* Return : None.
*************************************************** *******************************/
void EP1_OUT_Callback(void)
{
USB_SIL_Read(EP1_OUT,USB_Receive_Buffer); //Read the data of output endpoint
USB_Received_Flag=1; //Flag of received data
}
/****************************************************** ****************************
* Function Name: EP2_IN_Callback.
* Description : Endpoint input callback function.
* Input : None.
* Output : None.
* Return : None.
*************************************************** *******************************/
void EP2_IN_Callback(void)
{
}
Next, we will divide usb_istr.c. This c file mainly registers some endpoint response functions, such as the endpoint input and output callback function above, and the interrupt processing of the ISTR interrupt status register, as follows:
__IO uint16_t wIstr; /* Used to save the value read from the ISRT register*/
__IO uint8_t bIntPackSOF = 0; /* Number of start of frame packets (SOFs) received between two consecutive data packets */
/*Define an array of function pointers pointing to pointers. The function pointers point to 7 endpoint input service programs respectively*/
void (*pEpInt_IN[7])(void) =
{
EP1_IN_Callback,
EP2_IN_Callback,
EP3_IN_Callback,
EP4_IN_Callback,
EP5_IN_Callback,
EP6_IN_Callback,
EP7_IN_Callback,
};
/*Define an array of function pointers pointing to pointers. The function pointers point to 7 endpoint output service programs respectively*/
void (*pEpInt_OUT[7])(void) =
{
EP1_OUT_Callback,
EP2_OUT_Callback,
EP3_OUT_Callback,
EP4_OUT_Callback,
EP5_OUT_Callback,
EP6_OUT_Callback,
EP7_OUT_Callback,
};
#ifndef STM32F10X_CL
/****************************************************** ****************************
* Function Name : USB_Istr
* Description: ISTR interrupt event interrupt service routine
* Input : None.
* Output : None.
* Return : None.
*************************************************** *******************************/
void USB_Istr(void)
{
wIstr = _GetISTR(); //Read the value of the interrupt status register (ISTR)
#if (IMR_MSK & ISTR_CTR) //Correct transmission interrupt CTR flag
if (wIstr & ISTR_CTR &wInterrupt_Mask)
{
/* servicing of the endpoint correct transfer interrupt */
/* clear of the CTR flag into the sub */
CTR_LP(); //Call the correct transmission interrupt service routine
#ifdef CTR_CALLBACK
CTR_Callback(); //When CTR_CALLBACK is defined, CTR_Callback is called, like a hook function, to do something when a CRT interrupt occurs
#endif
}
#endif
/*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-* -*-*-*-*-*-*-*-*-*-*/
#if (IMR_MSK & ISTR_RESET) //Reset (RESET) interrupt flag
if (wIstr & ISTR_RESET & wInterrupt_Mask) //The interrupt flag read is the RESET interrupt flag, and the RESET interrupt is enabled
{
_SetISTR((uint16_t)CLR_RESET); //Clear RESET interrupt flag
Device_Property.Reset(); //Call the reset function
#ifdef RESET_CALLBACK
RESET_CALLBACK(); //When RESET_CALLBACK is defined, call RESET_CALLBACK, like a hook function, to do something when a RESET interrupt occurs
#endif
}
#endif
/*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-* -*-*-*-*-*-*-*-*-*-*/
#if (IMR_MSK & ISTR_DOVR) //Packet buffer overflow (DOVR) interrupt flag
if (wIstr & ISTR_DOVR & wInterrupt_Mask) //The interrupt flag read is the DOVR interrupt flag, and the DOVR interrupt is enabled
{
_SetISTR((uint16_t)CLR_DOVR); //Clear DOVR interrupt flag
#ifdef DOVR_CALLBACK
DOVR_Callback(); //When DOVR_CALLBACK is defined, DOVR_Callback is called, just like a hook function, to do something when a DOVR interrupt occurs
#endif
}
#endif
/*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-* -*-*-*-*-*-*-*-*-*-*/
#if (IMR_MSK & ISTR_ERR) //Error (ERR) interrupt flag
if (wIstr & ISTR_ERR & wInterrupt_Mask) //The interrupt flag read is the ERR interrupt flag, and the ERR interrupt is enabled
{
_SetISTR((uint16_t)CLR_ERR); //Clear ERR interrupt flag
#ifdef ERR_CALLBACK
ERR_Callback(); //When ERR_CALLBACK is defined, ERR_Callback is called, like a hook function, to do something when an ERR interrupt occurs
#endif
}
#endif
/*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-* -*-*-*-*-*-*-*-*-*-*/
#if (IMR_MSK & ISTR_WKUP) //Wake-up (WKUP) interrupt flag
if (wIstr & ISTR_WKUP & wInterrupt_Mask) //The interrupt flag read is the WKUP interrupt flag, and the WKUP interrupt is enabled
{
_SetISTR((uint16_t)CLR_WKUP); //Clear ERR interrupt flag
Resume(RESUME_EXTERNAL); //Call the wake-up function
#ifdef WKUP_CALLBACK
WKUP_Callback(); //When WKUP_CALLBACK is defined, WKUP_Callback is called, just like a hook function, to do something when a WKUP interrupt occurs
#endif
}
#endif
/*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-* -*-*-*-*-*-*-*-*-*-*/
#if (IMR_MSK & ISTR_SUSP) //Suspend (SUSP) interrupt flag
if (wIstr & ISTR_SUSP & wInterrupt_Mask) //The interrupt flag read is the SUSP interrupt flag, and the SUSP interrupt is enabled
{
/* check if SUSPEND is possible */
if (fSuspendEnabled) // Check if it can be suspended
{
Suspend(); //If it is possible to suspend, call the suspend function
}
else
{
/* if not possible then resume after xx ms */
Resume(RESUME_LATER); //If suspend is not allowed, resume after xx ms
}
/* clear of the ISTR bit must be done after setting ofCNTR_FSUSP */
_SetISTR((uint16_t)CLR_SUSP); //Clear SUSP interrupt flag
#ifdef SUSP_CALLBACK
SUSP_Callback(); //When SUSP_CALLBACK is defined, SUSP_Callback is called, just like a hook function, to do something when a SUSP interrupt occurs
#endif
}
#endif
/*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-* -*-*-*-*-*-*-*-*-*-*/
#if (IMR_MSK & ISTR_SOF) //Start of frame (SOF) interrupt flag
if (wIstr & ISTR_SOF & wInterrupt_Mask) //The interrupt flag read is the SOF interrupt flag, and the SOF interrupt is enabled
{
_SetISTR((uint16_t)CLR_SOF); //Clear SOF interrupt flag
bIntPackSOF++; //Count the total number of SOFs received
#ifdef SOF_CALLBACK
SOF_Callback(); //When SOF_CALLBACK is defined, SOF_Callback is called, like a hook function, to do something when a SOF interrupt occurs
#endif
}
#endif
/*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-* -*-*-*-*-*-*-*-*-*-*/
#if (IMR_MSK & ISTR_ESOF) //Expected frame start (ESOF) interrupt flag, when the expected SOF frame start is not received, the interrupt is triggered
if (wIstr & ISTR_ESOF & wInterrupt_Mask) //The interrupt flag read is the SOF interrupt flag, and the ESOF interrupt is enabled
{
_SetISTR((uint16_t)CLR_ESOF); //Clear ESOF interrupt flag
/* resume handling timing is made with ESOFs */
/* request without change of the machine state */
Resume(RESUME_ESOF); //Resume ESOF processing time
#ifdef ESOF_CALLBACK
ESOF_Callback(); //When ESOF_CALLBACK is defined, ESOF_Callback is called like a hook function to do something when an ESOF interrupt occurs
#endif
}
#endif
} /* USB_Istr */
Previous article:stm32NVIC and external interrupt
Next article:Introduction to STM32 interrupts
Recommended ReadingLatest update time:2024-11-17 02:54
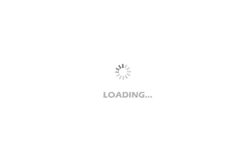
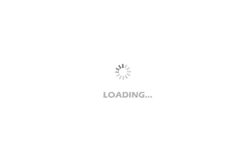
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- [ESP32 Learning] Reading Hall Sensor
- Lock-in Amplifier Design
- What is transparent transmission and point-to-multipoint transmission? What are their classic applications and advantages?
- How to build an undervoltage protection circuit using discrete components
- [Mill MYB-YT507 development board trial experience] + some problems in the compilation experience
- Is there any AMS1117 with 12V output?
- How to configure the pins used by LCD as GPIO?
- 【GD32E231_DIY】②DS1302 real-time clock module information
- Like this BOOST boost topology, if a lithium battery 4.2V & 1000mA is used, the load current is a momentary signal (no need to...
- [NXP Rapid IoT Review] + When connecting to a device via mobile app, the message "Unable to verify the device's hardware credentials" appears