This program is used in the development board to generate sounds of different frequencies. The overall program is relatively simple, mainly using two timers. The code and my comments are as follows.
/*********************************************************
Program function: Use fixed frequency square wave to drive buzzer, a total of 16 tones;
While emitting different tones, the LED lights up to indicate the
The number of the current tone (1~16)
----------------------------------------------------------
Dip switch setting: turn BUZZER to ON and the rest to OFF
Test instructions: Listen to the tone change of the buzzer. At the same time, the LED also has corresponding indications
**********************************************************/
#include
typedef unsigned char uchar;
uchar step = 0xff;
/****************************Main function****************************/
void main( void )
{
flying i;
WDTCTL = WDTPW + WDTHOLD; //关狗
/*The following six lines of code close all IO ports*/
P1DIR = 0XFF;P1OUT = 0XFF;
P2DIR = 0XFF;P2OUT = 0XFF;
P3DIR = 0XFF;P3OUT = 0XFF;
P4DIR = 0XFF;P4OUT = 0XFF;
P5DIR = 0XFF;P5OUT = 0XFF;
P6DIR = 0XFF;P6OUT = 0XFF;
P6DIR |= BIT2;P6OUT |= BIT2; //Disable level conversion
/*------Select the system main clock as 8MHz-------*/
BCSCTL1 &= ~XT2OFF; //Turn on XT2 high frequency crystal oscillator
do
{
IFG1 &= ~OFIFG; // Clear crystal oscillator failure flag
//IFG1 is the interrupt register OFIFG is the crystal oscillator startup failure interrupt flag
for (i = 0xFF; i > 0; i--); //Wait for 8MHz crystal to start oscillating
}
while ((IFG1 & OFIFG)); //Does the crystal oscillator failure flag still exist?
//The above step is mainly to wait for the crystal oscillator to work normally
BCSCTL2 |= SELM_2 + SELS; //MCLK and SMCLK select high frequency crystal
TACCTL0 |= CCIE; //Enable compare interrupt
TACTL |= TASSEL_2 + ID_3 ; //Count clock selection SMLK=8MHz, 1/8 division is 1MHz
TBCCR0 = 4096*2 - 1; //cycle two seconds
//Time calculation: 32768/8*2+1 Note that a watch crystal is used
TBCCTL0 |= CCIE;
TBCTL |= TBSSEL_1 + ID_3 + MC_1; //时钟源ACLK/8,up mode
P6DIR |= BIT7; //Buzzer corresponds to IO 6.7 and is set as output
P2DIR = 0xff; //Indicates the corresponding status
P2OUT = 0xff;
_UNITE();
LPM1;
}
/*******************************************
Function name: Timer_A
Function: interrupt service function of timer A, driven here
Buzzer sounds
Parameters: None
Return value: None
********************************************/
#pragma vector=TIMERA0_VECTOR
__interrupt void Timer_A (void)
{
P6OUT ^= BIT7; // Toggle P6.7
}
/*******************************************
Function name: Timer_B
Function: Interrupt service function of timer B, change here
Buzzer sound frequency
Parameters: None
Return value: None
********************************************/
#pragma vector=TIMERB0_VECTOR
__interrupt void Timer_B (void)
{
if(step == 0xff) //The initial value of step is 0xff,
TACTL |= MC_1; //TimerA needs to be set to up-counting mode. This can be set during initialization. I don't understand why it is placed here.
step++;
switch(step)
{
case 0: TACCR0 = 5000; P2OUT = ~1; break; // 100Hz
//P2OUT uses LED to display the corresponding value, just for demonstration, no practical significance
case 1: TACCR0 = 2500; P2OUT = ~2; break; // 200Hz
case 2: TACCR0 = 1250; P2OUT = ~3; break; // 400Hz
case 3: TACCR0 = 625; P2OUT = ~4; break; // 800Hz
case 4: TACCR0 = 500; P2OUT = ~5; break; // 1KHz
case 5: TACCR0 = 250; P2OUT = ~6; break; // 2KHz
case 6: TACCR0 = 167; P2OUT = ~7; break; // 3KHz
case 7: TACCR0 = 125; P2OUT = ~8; break; // 4KHz
case 8: TACCR0 = 100; P2OUT = ~9; break; // 5KHz
case 9: TACCR0 = 83; P2OUT = ~10; break; // 6KHz
case 10: TACCR0 = 71; P2OUT = ~11; break; // 7KHz
case 11: TACCR0 = 63; P2OUT = ~12; break; // 8KHz
case 12: TACCR0 = 56; P2OUT = ~13; break; // 9KHz
case 13: TACCR0 = 50; P2OUT = ~14; break; // 10KHz
case 14: TACCR0 = 33; P2OUT = ~15; break; // 15KHz
case 15: TACCR0 = 25; P2OUT = ~16; break; // 20KHz
case 16: step = 0xff; // Continue to add, the same effect as clearing, loop playback
}
}
Previous article:MSP430 Learning Notes 5- Playing Music with Buzzer
Next article:MSP430 Study Notes 3-PWM Generation
Recommended ReadingLatest update time:2024-11-16 20:49
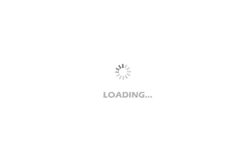
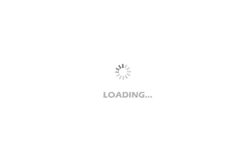
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Sampling period
- Ask for help, recommendation, keywords
- It's holiday time, but we're still working overtime every day
- Tuya Sandwich Wi-Fi & BLE SoC NANO Main Control Board WBRU+ Unboxing
- Why should we have an oscilloscope
- Why use WDM-PON in 5G fronthaul
- 【Iprober 520 Current Probe】Evaluation Report (II) Basic Performance Test
- MicroPython Hands-on (28) - Yeelight for the Internet of Things
- Basic operations and precautions for exporting Gerber files from PCB design (Part 1)
- Allegro cannot be installed due to VC2005. Is there any way to fix this?