P1~P6 each group has 8 I/O ports, P3, P4, P5, P6 have I/O and other on-chip peripheral functions, each group has 4 registers. In addition to the above functions, P1 and P2 also have interrupt capabilities, each group has 7 registers.
2. Px port
1. P1, P2 ports
(1) PxDIR input/output direction register (x represents 1, 2)
The eight independent bits define the input and output directions of the eight pins Px7~Px0.
0 Input mode, read only.
1 Output mode, readable and writable.
All 8 bits are reset and set to 0 after PUC.
Eg: P1DIR = 0x0F;
Here, 0x0F is represented in hexadecimal, and the corresponding binary is 0000 1111, that is, the upper 4 bits of P1DIR are set to 0, and the lower 4 bits are set to 1, that is, P1.7, P1.6, P1.5, P1.4 (the upper 4 bits of P1) are set to input mode; and P1.3, P1.2, P1.1, P1.0 (the lower 4 bits of P1) are set to output mode.
(2) PxIN input register
Each bit corresponds to an input port, such as: the second bit corresponds to Px.2, which records the data input to the corresponding bit, 0 or 1. The input register is a read-only register, and the user cannot write to it, but can only read data from it.
For example:
char a;
a = P1IN;
Assign the data input by P1 to a, or read one or several bits of it.
eg: if((P1IN&0x01) == 1) a = b;
This sentence means that if the lowest bit of P1IN is 1, that is, the input of P1.0 is 1, b will be assigned to a; the '&' means the bitwise AND operation, that is, the 8-bit data in P1IN and 0x01 are bitwise ANDed.
(3) PxOUT output register
This register is the output buffer register of the I/O port. Each bit corresponds to an output port, such as: the second bit corresponds to Px.2. When the user writes data to the corresponding bit, the corresponding port will output the corresponding data.
For example:
P1OUT = 0x01;
Set the lowest position of P1OUT to 1 and the other positions to 0, that is, P1.7, P1.6, P1.5, P1.4, P1.3, P1.2, and P1.1 output 0, and P1.0 outputs 1;
P1OUT = BIT0;
By consulting the header file of the MSP430 microcontroller, we can know that BIT0 is 0x01. This sentence is equivalent to the previous one. The reason for writing it this way is to make the program easier to understand and more concise.
(4) PxIFG interrupt flag register
0 means no interrupt request
1 indicates an interrupt request
The interrupt flags PxIFG.0~PxIFG.7 share an interrupt vector and are multi-source interrupts. When a rising edge or falling edge appears on the corresponding I/O port, the corresponding flag bit will be set. If the interrupt is enabled and the system total interrupt is enabled, an interrupt will be generated and the interrupt handler will be executed.
Note: 1. After PxIFG.0~PxIFG.7 are set, they will not be reset automatically. You must use software to determine which I/O has an interrupt event and reset the corresponding flag.
2. The external interrupt event must be maintained for no less than 1.5 times the MCLK time to ensure that the interrupt request is accepted and the corresponding interrupt flag is set.
(5) PxIE interrupt enable register
0 Disable interrupts
1 Enable interrupts
Only a transition can cause an interrupt request, while a static level cannot.
(6) PxIES interrupt trigger edge selection register
0 A rising edge sets the corresponding flag
1 Falling edge sets the corresponding flag
(7) PxSEL function selection register
0 Select pin as I/O function
1 Select pin as peripheral module function
There are also abundant peripheral modules in the microcontroller, which usually need to communicate with the outside world. However, the microcontroller has limited pins, so the method of multiplexing P1 and P2 pins is used to achieve this.
For example:
P5SEL |= 0x10; // P5.4 is used as MCLK output
2. Ports P3, P4, P5, P6
(1) Ports P3, P4, P5, and P6 have the same functions as P1 and P2 except that they do not have interrupt capability, including input/output functions and peripheral module functions.
(2) Since ports P3, P4, P5, and P6 do not have interrupt capabilities, they do not have interrupt-related registers. Each port group has four registers: PxDIR input/output direction register, PxIN input register, PxOUT input register, and PxSEL function selection register.
3. Port COM and S
Used to realize direct interface with LCD, only MSP430F4XX series of microcontrollers have it.
———————————————————————————————————————
#include "msp430x26x.h"
void main( void )
{
// Stop watchdog timer to prevent time out reset
WDTCTL = WDTPW + WDTHOLD; //Stop the watchdog, WDTPW is the watchdog write command, WDTHOLD is the watchdog stop bit.
P1DIR = 0x0f; //PxDIR direction register, 0 is input mode, 1 is output mode.
//After all PUCs are reset. When used as input, it can only be read; when used as output, it can be read and written.
P1OUT = 0xff;
P1IE |= 0xf0; // P1.4, 5, 6, 7 interrupt enable (0 disables interrupt, 1 enables interrupt)
P1IES |= 0xf0; // P1.4, 5, 6 IO port edge interrupt trigger mode, lower edge is valid (0 rising edge is valid, 1 falling edge is valid)
P1IFG &= 0x00; // P1.4, 5, 6 clear IO interrupt flag
P2DIR |= BIT3+BIT4;
P2OUT = 0xff;
P2IE |= BIT0+BIT1;
P2IES |= 0x00;
P2IFG &= 0x00;
_EINT(); //Enable general interrupt
LPM4; // (LOW POWER MODE) Enter low power mode 4, at this time the microcontroller power consumption is the lowest
}
//************************************************ ***************
//P1 port interrupt service routine
#pragma vector=PORT1_VECTOR
__interrupt void p1int(void)
{
if((P1IFG&0xf0)==0x10) P1OUT=0xf1;
else if((P1IFG&0xf0)==0x20) P1OUT=0xf2;
else if((P1IFG&0xf0)==0x40) P1OUT=0xf4;
else if((P1IFG&0xf0)==0x80) P1OUT=0xf8;
P1IFG &= 0x00;
}
#pragma vector=PORT2_VECTOR
__interrupt void p2_port(void)
{
if((P2IFG&0x0f)==0x01) P2OUT=0x04;
else if((P2IFG&0x0f)==0x02) P2OUT=0x08;
P2IFG &= 0x00;
}
Previous article:A comprehensive look at the MSP430 MCU timer
Next article:Keil Memory Model Selection Problem
Recommended ReadingLatest update time:2024-11-16 23:57
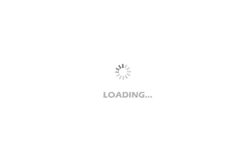
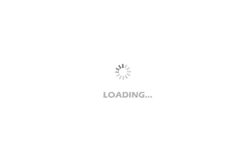
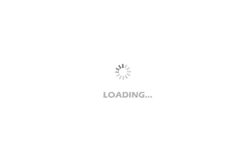
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [Sipeed LicheeRV 86 Panel Review] 5-Porting Python3 to the Development Board
- GD32F103RC burning problem
- How much processor overhead does Tracealyzer require to trace an RTOS?
- [RVB2601 Creative Application Development] Environmental Monitoring Terminal 03-Using LVGL to Design the Interface
- Weekly evaluation information delivered to you, keep up to date with new evaluation trends!
- Why does the value of the ad7747 ready register remain unchanged?
- Advantages of Integrated Current Sensing
- ROM, RAM, SRAM, DRAM, RRAM, FRAM, flash, there are so many types, how to distinguish them
- Novice Question
- Milliohm meter-host computer design based on [EVAL-ADICUP360]